Beginner of Coding: A Journey from Zero to OCaml

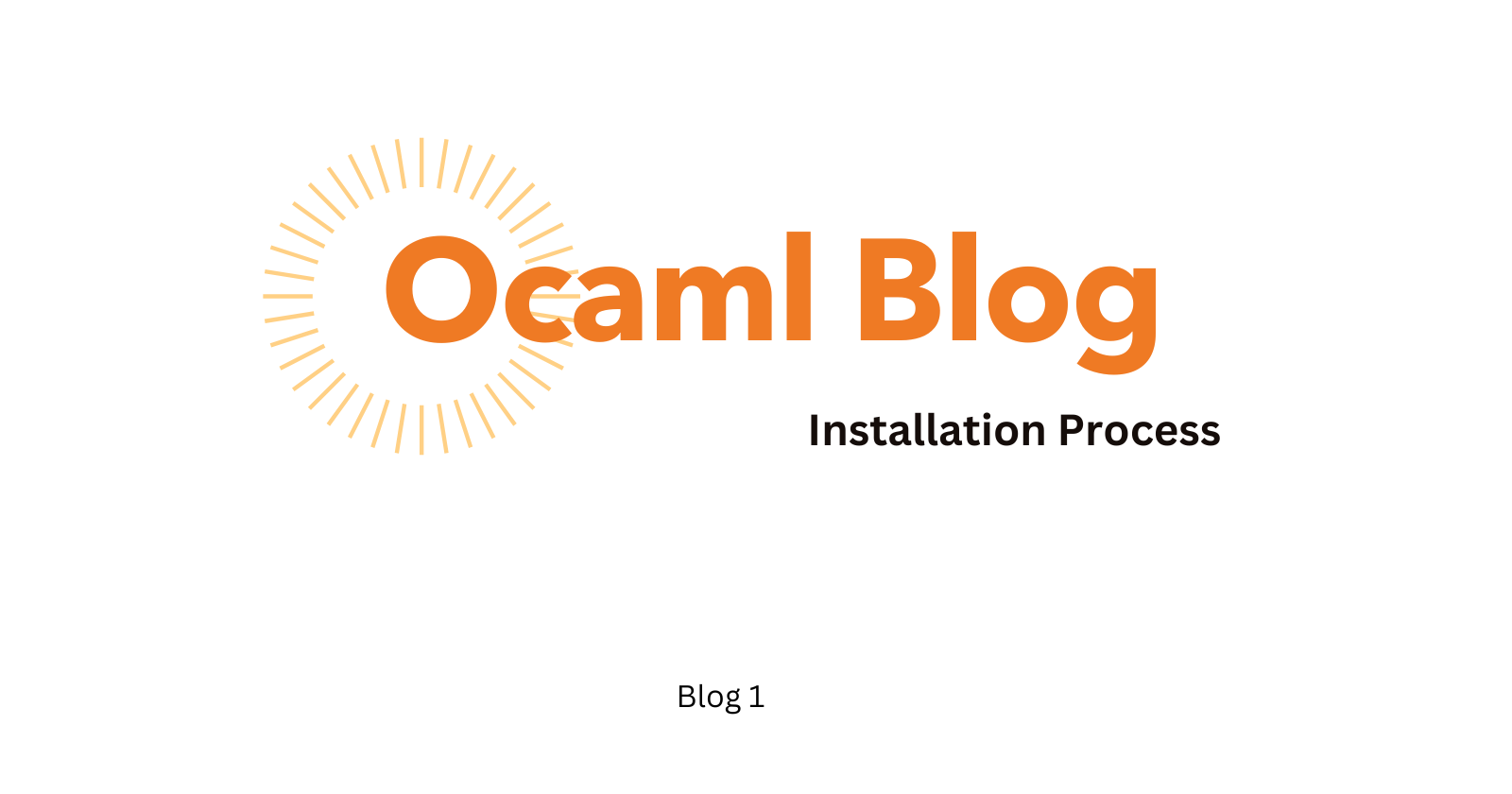
Welcome to this blog series dedicated to absolute beginners—people who have no idea what coding is or how to get started. Don’t worry, we’re going to break it all down step by step, and by the end of this, you’ll have the basic knowledge to write your first line of code!
So, let’s start with the most fundamental question: What is coding?
To understand coding, we first need to know the job that requires this skill. Imagine you’re sitting in front of a computer, and you need to tell it what to do. It might be something simple, like asking it to say “Hello, World” on the screen, or wait until it receives a number, or maybe save a file for you. This is where coding comes in—it’s the skill that lets you communicate with a machine and tell it what to do.
But here’s the catch: computers don’t understand human language. It’s true! Although you might think your voice assistants like ‘Google Assistant’ or ‘Siri’ understand you, in reality, they don’t speak our language. Instead, they communicate using something called machine language.
You see, machine language is a system based on binary numbers, which consist of only 0s and 1s. Everything in a computer—from numbers to images—is represented using this binary system. For example:
0 = 0
1 = 1
2 = 10
3 = 11
4 = 100
...and so on.
This system works well because machines can easily handle binary—it’s just two states, like a lightbulb being either on or off. So, while a machine might not understand the number "5" as you do, it would understand "101", or "on off on"!
Now, you might ask:
“If machines don’t understand human language, and only understand binary, does that mean coding is just typing 0s and 1s until my hands break?”
First of all, great question! But no, you don’t have to deal with binary on a daily basis. This brings us to an important concept in coding: abstraction.
The Magic of Abstraction
In simpler terms, abstraction means hiding the complexity and making things easier for us. Let’s consider an example: When we were children, we used to count numbers with lines like this:
I, II, III, IIII, IIIII, IIIIII... This system is called the unary number system. It’s simple but not efficient, right?
So, as we grew older, we started using symbols like 1, 2, 3, 4, 5, and so on, which made counting a lot easier. Similarly, in coding, we don’t need to deal with a bunch of 0s and 1s. Instead, we use programming languages to simplify things.
Programming languages allow us to communicate with computers in a more human-friendly way. They can be divided into various levels based on how much abstraction they provide. These levels are:
Machine language (001100)
Low-level language (Assembly)
Mid-level language (C)
High-level language (Python)
At each level, the abstraction becomes more sophisticated, allowing us to write code that is more understandable to humans and more manageable to write.
What Do We Know So Far?
Here’s a quick summary of what we’ve learned so far:
The job of a programmer is to talk to a machine and tell it what to do.
Machines understand machine language (binary, 0s and 1s).
We use programming languages to simplify this communication.
When we write code, it has to follow certain rules. These rules are the syntax of the programming language, and they are in exchange for not having to write a ton of 0s and 1s.
Compiling and Executing Code
When we write code, we usually save it in files so we can use it later. These files are different from regular files like .pdf or .txt. When you want the computer to execute your code, we need to compile and execute it.
Compiling means the computer will read your code, understand the instructions, and convert them into machine language (binary). The compiled instructions are saved into a file with an extension like
.exe
or.out
.Executing means running the compiled file, telling the computer to follow the commands inside it.
Key Terms to Know
Let’s quickly cover some essential terms that will help you as you start coding:
Terminal: A place where you can type commands to the computer. Think of it like a chat window where you give instructions.
Commands: These are pre-written instructions the computer understands and can perform when you ask it to.
Code Editor: A special software that helps you write code easily, with features like syntax highlighting and code completion.
OCaml: A programming language we’ll be covering in this blog series.
Setting Up OCaml
Before we jump into the fun part of coding, let's get your system set up. Depending on your operating system, the installation process might vary. Don’t worry, I’ll guide you through it step-by-step for Windows, Linux, and Mac users.
For Windows Users:
If you're using Windows, you'll need to install the Windows Subsystem for Linux (WSL). This allows you to run a Linux environment directly within Windows, which is necessary for installing OCaml.
To guide you through the process, I recommend watching this video tutorial: Install WSL on Windows. The video walks you through installing WSL and setting up an Ubuntu environment.
Once you have WSL set up, follow these steps in your Ubuntu terminal to complete the OCaml installation:
Update and upgrade your system:
sudo apt update sudo apt upgrade -y
Install necessary tools:
sudo apt install -y zip unzip build-essential
This installs tools for file compression and building software.
Install OPAM (OCaml package manager):
sudo apt install opam
This is the package manager for OCaml. It helps you install, manage, and update OCaml and related tools.
Initialize OPAM:
opam init --bare -a -y
Install OCaml’s Utop and OUnit2 for testing:
opam install -y ocaml utop ounit2
ocaml
: This is the core OCaml programming language. Installing this ensures that you have OCaml itself set up on your system, which is the language you will be coding in.Why?: Without this, you wouldn’t have OCaml installed, and you wouldn’t be able to write or run OCaml code.
utop
: This is an interactive OCaml shell. It allows you to run OCaml code interactively, which is extremely useful for experimenting with small pieces of code or testing ideas without having to write a complete program.Why?:
utop
provides an easy and fast way to experiment with OCaml code directly in the terminal, making the learning process smoother.ounit2
: This is a testing framework for OCaml. It helps you write unit tests for your code to ensure that everything works correctly.Why?: Writing tests for your code is an important part of software development. With
ounit2
, you can test your OCaml programs to check for bugs or errors and make sure everything behaves as expected.
For Linux Users:
For Linux users, the process is pretty straightforward. You can install OCaml directly through the package manager on your system.
Update and upgrade your system (this ensures your system is up to date):
sudo apt update sudo apt upgrade -y
Install the required build tools:
sudo apt install -y zip unzip build-essential
Install OPAM (the OCaml package manager):
sudo apt install opam
Initialize OPAM (this prepares the OPAM environment for installing OCaml):
opam init --bare -a -y
Install OCaml and other useful tools like Utop (an interactive OCaml shell) and OUnit2 (for unit testing):
opam install -y ocaml utop ounit2
This will install OCaml along with the tools needed to start coding right away.
For Mac Users:
For Mac users, you can install OCaml through Homebrew, which is a popular package manager for macOS.
Install Homebrew (if you don’t already have it installed): Open the terminal and run:
/bin/bash -c "$(curl -fsSL https://raw.githubusercontent.com/Homebrew/install/HEAD/install.sh)"
Install OPAM (OCaml package manager) using Homebrew:
brew install opam
Initialize OPAM:
opam init --bare -a -y
Install OCaml and other useful tools like Utop and OUnit2:
opam install -y ocaml utop ounit2
This will install OCaml and the necessary tools to start coding.
Online Option for All Users:
If you prefer not to set up OCaml on your local machine, or if you don’t have access to a computer, you can use online OCaml compilers. One great option is the Try OCaml Online Compiler. This allows you to write, compile, and run OCaml code directly from your browser without any installation required.
What's Next?
Once you’ve completed the installation process for your operating system, you're ready to start coding with OCaml. In the next blog, we will write our very first OCaml program together!
If you encounter any issues or have questions about the installation, feel free to ask in the comments or reach out to me directly. Let's get coding!
Subscribe to my newsletter
Read articles from Kushal Karmakar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Kushal Karmakar
Kushal Karmakar
Love to share new lessons as I learn them. CSE student at MAKAUT, WB.