Chapter 7: Mastering CSS Selectors and Advanced Styling Techniques
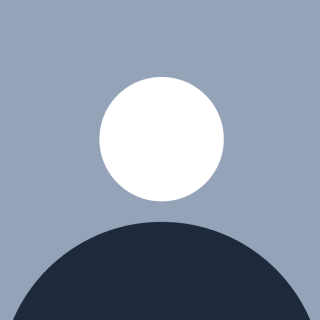
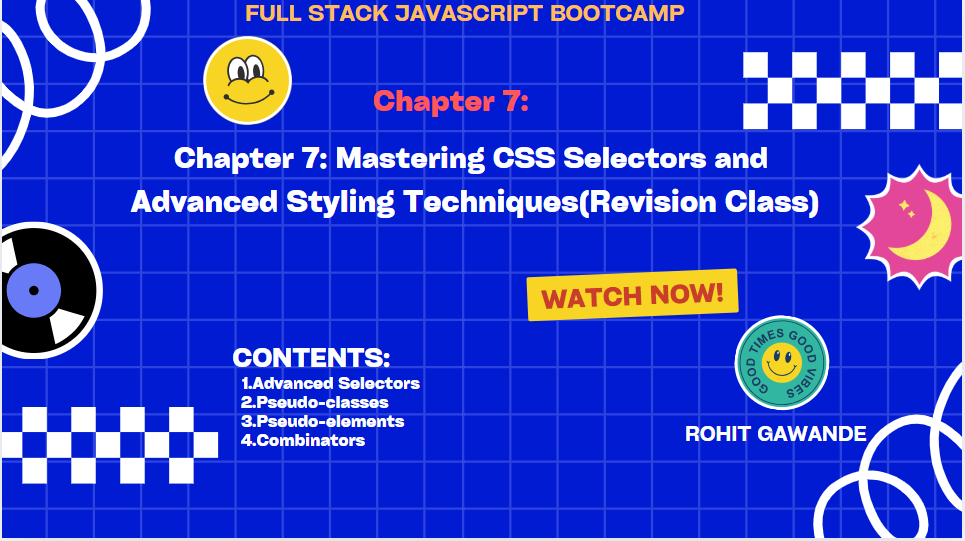
1. Introduction
Overview of CSS Selectors
CSS selectors are the foundation of styling web pages. They allow developers to target specific HTML elements for styling, ensuring precision and efficiency in web development. Selectors range from simple ones like targeting element types (p
, div
) to more advanced combinations involving pseudo-classes (:hover
) and pseudo-elements (::before
).
Importance of Mastering Selectors for Efficient Web Development
A strong understanding of CSS selectors enables developers to:
Write clean, maintainable CSS.
Avoid unnecessary redundancy by targeting specific elements.
Optimize page performance by reducing overuse of general rules.
Handle dynamic states of elements (e.g., hover, focus) effectively.
Scope of This Chapter
This chapter will cover:
Selectors: Basic selectors like universal, type, class, and ID.
Pseudo-classes: Styling based on element states.
Pseudo-elements: Targeting parts of elements, such as the first line or first letter.
Combinators: Defining relationships between elements for advanced styling.
2. Recap of Basic Selectors
Universal Selector (*
)
The universal selector targets all elements on a page. While it is a powerful tool, it should be used cautiously to avoid unintended styling across the site.
Example:
* {
margin: 0;
padding: 0;
}
This removes the default margin and padding from all elements.
Type Selector
The type selector targets HTML elements directly by their tag name. It is simple yet effective for general styling.
Example:
p {
font-size: 16px;
line-height: 1.5;
}
This applies the specified styles to all <p>
elements on the page.
Class and ID Selectors
Class Selector: Targets elements with a specific class attribute. Classes are reusable across multiple elements.
Example:
.card {
border: 1px solid #ddd;
border-radius: 8px;
}
ID Selector: Targets a single, unique element by its ID. IDs are not reusable.
Example:
#header {
background-color: #f4f4f4;
padding: 20px;
}
Comparison:
Feature | Class Selector (.class ) | ID Selector (#id ) |
Reusability | Can be used on multiple elements | Used only for a single element |
Specificity | Lower specificity | Higher specificity |
3. Box Model Refresher
The box model is fundamental to CSS layout. It describes how an element's size and spacing are calculated, consisting of margins, borders, padding, and content.
Margin
The margin is the outermost layer of the box model, providing space between the element and its neighbors.
Example:
.container {
margin: 20px;
}
Common Pitfall: Collapsing margins occur when vertical margins of adjacent elements combine. Use padding or borders to prevent this.
Padding
Padding is the space between the content and the element's border, creating "breathing room" within the element.
Example:
.button {
padding: 10px 20px;
}
Key Difference from Margin:
Feature | Margin | Padding |
Placement | Outside the border | Inside the border |
Affects size | No | Yes (increases element size) |
Border
The border wraps around the padding and content, visually defining the element's boundaries.
Example:
.box {
border: 2px solid #333;
border-radius: 5px;
}
Borders can be customized with styles (solid
, dashed
, dotted
), widths, and colors.
Background Properties
Background properties allow you to set colors, images, or gradients for an element.
Example:
.hero {
background: linear-gradient(to right, #ff7e5f, #feb47b);
color: white;
padding: 40px;
}
Tip: Use background shorthand to combine properties for cleaner code.
Height and Width
Height and width define the size of an element. Use relative units (%
, em
, rem
, vh
, vw
) for responsiveness.
Best Practices for Responsive Design:
Avoid fixed units like
px
for layouts; use%
orvw
.Use
max-width
instead ofwidth
for better flexibility.
Example:
.image {
max-width: 100%;
height: auto;
}
This ensures the image scales proportionally within its container.
These sections set the stage for understanding more advanced CSS techniques, including pseudo-classes, pseudo-elements, and combinators, which will be covered in the subsequent sections.
4. Child and Sibling Selectors
Selectors in CSS define rules for styling elements, often based on their relationships in the DOM. Here, we’ll explore Child Selectors and Sibling Selectors using your provided examples.
Child Selector (>
):
The child selector is used to target elements that are direct children of a specific parent element. This is helpful when you want to apply styles to only the immediate descendants, ignoring any nested elements.
Your Example:
<style>
div > p {
color: blue;
}
</style>
This rule applies the style only to <p>
elements that are direct children of a <div>
. Let’s see it in a complete HTML example.
Output:
The two
<p>
elements inside the<div>
will turn blue.The
<p>
outside the<div>
and the deeply nested<p>
remain unaffected.
Adjacent Sibling Selector (+
):
The adjacent sibling selector applies styles to the next immediate sibling of an element.
Your Example:
<style>
div + p {
color: blue;
}
</style>
Here, the rule targets only the first <p>
that immediately follows a <div>
.
Output:
The
<p>
right after the<div>
is styled blue.All other
<p>
elements remain unaffected.
General Sibling Selector (~
):
The general sibling selector applies styles to all sibling elements that follow a specific element.
Your Example:
<style>
div ~ p {
color: blue;
}
</style>
This rule styles every <p>
that follows a <div>
as a sibling.
Output:
All
<p>
elements after the<div>
are styled blue.Any
<p>
elements inside the<div>
are unaffected.
Comparison Table for Selectors
Selector | Targets | Example | Output |
div > p | Direct child <p> of <div> | <div><p></p></div> | Styles only direct children. |
div + p | Next immediate sibling <p> | <div></div><p></p> | Styles the first <p> after <div> . |
div ~ p | All sibling <p> of <div> | <div></div><p></p><p></p> | Styles all <p> after <div> . |
Why Use These Selectors?
Precision: Apply styles only where needed.
Flexibility: Handle dynamic layouts efficiently.
Scalability: Avoid redundant class definitions for sibling or child elements.
5. Pseudo-selectors
Pseudo-selectors are used in CSS to define the style of elements based on their state or specific part. Let’s dive into some commonly used pseudo-selectors with your examples.
a. Hover (:hover
)
Explanation:
The :hover
pseudo-selector allows you to style an element when the user places the mouse pointer over it. It’s widely used for interactive elements like links, buttons, or images.
Your Example:
a:hover {
background-color: yellow;
color: red;
}
When a user hovers over an anchor (<a>
), its background will turn yellow, and the text will turn red.
Complete Example:
Output:
When the user hovers over the link, it will have a yellow background and red text.
Removing the mouse pointer restores its original style.
b. Focus (:focus
)
Explanation:
The :focus
pseudo-selector applies styles when an element, typically a form field, is in focus. This happens when a user clicks on the field or navigates to it using the keyboard (like pressing the Tab
key).
Your Example:
input:focus {
border: 2px solid blue;
}
When an <input>
field gains focus, a blue border will appear around it.
Complete Example:
Output:
Clicking inside the input field highlights it with a blue border.
Clicking outside or on another field removes the focus style.
c. Visited (:visited
)
Explanation:
The :visited
pseudo-selector styles links that have already been visited by the user. It’s useful for distinguishing previously clicked links.
Your Example:
a:visited {
color: gray;
}
Any anchor (<a>
) previously visited by the user will have gray text.
Complete Example:
Output:
After clicking a link, its color will change to gray.
Unvisited links retain their default style.
d. Active (:active
)
Explanation:
The :active
pseudo-selector styles elements when they are being activated. For links, this is the moment between clicking and releasing the mouse button.
Your Example:
a:active {
color: red;
}
When a user clicks on an anchor (<a>
), its text will temporarily turn red.
Complete Example:
Output:
Clicking and holding the link changes its text to red.
Releasing the click restores the original style.
Comparison Table for Pseudo-selectors
Pseudo-selector | State Triggered | Example | Effect |
:hover | When mouse hovers over an element | a:hover | Changes style on hover. |
:focus | When an element gains focus | input:focus | Styles form elements in focus. |
:visited | After a link is visited | a:visited | Changes color of visited links. |
:active | While an element is being activated | a:active | Temporarily changes style on click. |
When to Use These Pseudo-selectors?
Enhance UX: Guide users with interactive visual cues.
Accessibility: Make focused elements more distinguishable.
Customization: Style elements based on their state, improving engagement.
6. Pseudo-Element Selectors
Pseudo-elements in CSS are used to style specific parts of an element or insert content before or after an element. They target virtual elements that aren't in the document structure but are generated by CSS.
a. Before and After (::before
, ::after
)
Explanation:
The ::before
and ::after
pseudo-elements allow you to insert content before or after an element's actual content, respectively. You can use these to add decorative icons, text, or styles that complement the element.
Your Example for ::before
:
.warning::before {
content: "⚠️ Warning: ";
color: red;
}
This example inserts a warning icon and the word "Warning: " before any element with the class .warning
.
Complete Example:
Output:
The text "⚠️ Warning: " will appear before the actual message.
The warning icon and the "Warning:" text will be red, followed by the original message.
Using ::after
:
.success::after {
content: "✔️ Task Complete!";
color: green;
}
In this example, the text "✔️ Task Complete!" will be added after elements with the class .success
.
Complete Example:
Output:
The text "✔️ Task Complete!" will appear after the message "The operation was successful."
The inserted text will be green.
b. First Letter (::first-letter
) and First Line (::first-line
)
Explanation:
The ::first-letter
and ::first-line
pseudo-elements target the first letter and the first line of text within an element, respectively. These pseudo-elements allow you to apply distinct styles to these specific parts of the element.
Your Example for ::first-letter
:
p::first-letter {
font-size: 200%;
color: red;
}
This will make the first letter of any paragraph (<p>
) significantly larger and red in color.
Complete Example for ::first-letter
:
Output:
- The first letter of the paragraph will appear in red and will be twice as large as the regular text.
Example for ::first-line
:
p::first-line {
font-weight: bold;
}
This will make the first line of any paragraph bold.
Complete Example for ::first-line
:
Output:
- The first line of the paragraph will be bolded.
7. Combinators
Combinators define the relationship between selectors. They allow you to target elements based on their position or relationship to other elements.
a. Descendant Selector (' '
or ' '
)
Explanation:
The descendant selector targets any element that is a descendant of another element, regardless of its depth in the hierarchy. It's a space between selectors.
Your Example:
div p {
color: purple;
}
This will select all <p>
elements that are inside a <div>
and change their text color to purple.
Complete Example:
Output:
The
<p>
inside the<div>
will be purple.The
<p>
outside the<div>
will remain unaffected.
b. Child Selector (>
)
Explanation:
The child selector targets only the direct children of an element. It’s represented by a greater-than (>
) symbol.
Example:
This was already explained earlier in our example, where we target direct children.
c. Adjacent Sibling Selector (+
)
Explanation:
The adjacent sibling selector selects an element that is immediately preceded by another element, represented by the plus (+
) sign.
Example:
Previously explained, you can target an element that directly follows another.
d. General Sibling Selector (~
)
Explanation:
The general sibling selector targets all sibling elements that follow a particular element. It’s represented by the tilde (~
) symbol.
Example:
Previously explained, this targets all siblings of a specific element.
Comparison Table for Combinators:
Combinator | Description | Example | Effect |
Descendant (' ' ) | Selects all descendants of a given element | div p { color: purple; } | All <p> elements inside <div> will be purple. |
Child (> ) | Selects direct children of a given element | div > p { color: purple; } | Only the direct <p> children of <div> will be purple. |
Adjacent Sibling (+ ) | Selects the element immediately following another | h2 + p { color: blue; } | The first <p> after an <h2> will be blue. |
General Sibling (~ ) | Selects all siblings that follow a given element | h2 ~ p { color: blue; } | All <p> elements after <h2> will be blue. |
When to Use Combinators?
Targeting Nested Elements: Use descendant or child selectors to style elements inside specific containers.
Sibling Relationships: Use adjacent or general sibling selectors to apply styles to elements that follow each other.
10. Practical Projects (Focusing on Learned Topics)
Project 1: Blog Layout Styling with Selectors
Objective:
Use child, sibling, and pseudo-element selectors to create a blog layout. You'll practice styling different sections of the layout using the concepts you've learned so far.
Approach:
Header Section: Use pseudo-elements like
::before
to add decorative text or icons before the header title.Main Content: Use descendant and child selectors to style blog posts and any nested elements inside the main content.
Sidebar: Use sibling selectors to style the sidebar and its elements relative to the content section.
Example:
Key CSS Techniques:
::before
is used to add a small icon before the text in the header and sidebar.Child selectors (
>
) target direct children like.post
inside.content
.Sibling selectors (
+
) are used to create space between the sidebar and content.
Project 2: Form Styling with Pseudo-classes
Objective:
Create a form with styling applied using pseudo-classes like :focus
, :hover
, and :active
to enhance user interaction.
Approach:
Input Fields: Use
:focus
to highlight form fields when they are clicked or selected.Submit Button: Use
:hover
to change the button style when the user hovers over it.Active Link: Style the link or button in the form to show a different color when clicked.
Example:
Key CSS Techniques:
:focus
is used to highlight the input fields when focused.:hover
changes the button color on hover.:active
changes the button color when clicked.
Project 3: Interactive Navigation Links
Objective:
Design a simple navigation menu using the :hover
, :visited
, and :active
pseudo-classes to provide an interactive experience for the user.
Approach:
Links Styling: Use
:visited
to style visited links differently.Hover Effects: Apply
:hover
to change the color or background when hovering over navigation links.Active State: Style the active link to show which page is currently being viewed.
Example:
Key CSS Techniques:
:hover
changes the background color of the links when the user hovers.:visited
changes the color of the links that have already been visited.:active
applies a style when the link is actively clicked.
Conclusion:
These projects focus solely on the CSS selectors and pseudo-classes you've learned: pseudo-elements, pseudo-classes (:hover
, :focus
, :visited
, :active
), and combinators. By implementing these concepts in practical projects, you'll be able to strengthen your understanding of how CSS can enhance web design and user interaction.
12. Conclusion
Recap of Key Concepts
CSS offers a rich set of tools to style web elements. Let’s quickly recap some of the key concepts we've explored:
Selectors: These allow you to target specific HTML elements for styling. Key types include element, class, ID, and attribute selectors.
Pseudo-Classes: They target an element’s state, such as
:hover
,:focus
,:visited
, and:active
, allowing you to add interactive behaviors.Pseudo-Elements: They are used to style parts of an element, such as
::before
and::after
, which can be used to insert content before or after an element.Combinators: These include descendant, child, adjacent sibling, and general sibling selectors, which define the relationship between elements for styling.
Related Posts in My Series:
Chapter 1:Mastering the Web: A Journey Through HTML and Beyond
Other Series:
Connect with Me
Stay updated with my latest posts and projects by following me on social media:
LinkedIn: Connect with me for professional updates and insights.
GitHub: Explore my repository and contributions to various projects.
LeetCode: Check out my coding practice and challenges.
Your feedback and engagement are invaluable. Feel free to reach out with questions, comments, or suggestions. Happy coding!
Rohit Gawande
Full Stack Java Developer | Blogger | Coding Enthusiast
Subscribe to my newsletter
Read articles from Rohit Gawande directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
Rohit Gawande
Rohit Gawande
🚀 Tech Enthusiast | Full Stack Developer | System Design Explorer 💻 Passionate About Building Scalable Solutions and Sharing Knowledge Hi, I’m Rohit Gawande! 👋I am a Full Stack Java Developer with a deep interest in System Design, Data Structures & Algorithms, and building modern web applications. My goal is to empower developers with practical knowledge, best practices, and insights from real-world experiences. What I’m Currently Doing 🔹 Writing an in-depth System Design Series to help developers master complex design concepts.🔹 Sharing insights and projects from my journey in Full Stack Java Development, DSA in Java (Alpha Plus Course), and Full Stack Web Development.🔹 Exploring advanced Java concepts and modern web technologies. What You Can Expect Here ✨ Detailed technical blogs with examples, diagrams, and real-world use cases.✨ Practical guides on Java, System Design, and Full Stack Development.✨ Community-driven discussions to learn and grow together. Let’s Connect! 🌐 GitHub – Explore my projects and contributions.💼 LinkedIn – Connect for opportunities and collaborations.🏆 LeetCode – Check out my problem-solving journey. 💡 "Learning is a journey, not a destination. Let’s grow together!" Feel free to customize or add more based on your preferences! 😊