Day 2: JavaScript Fundamentals and Best Practices

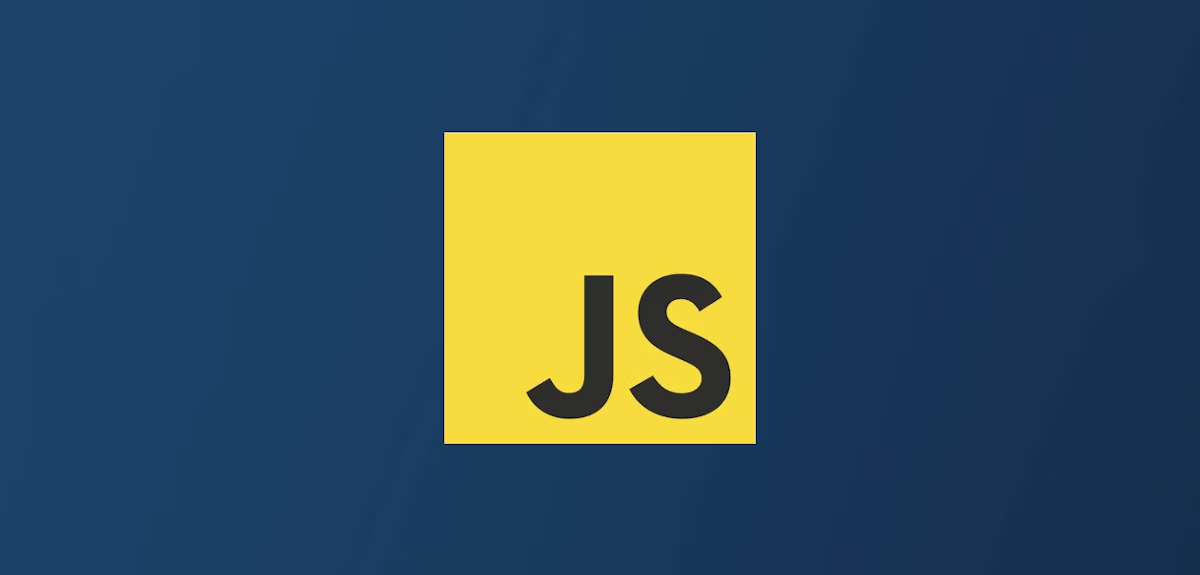
Introduction:
In today’s learning session, I explored some important aspects of JavaScript, including MIME types, strict mode, targeting legacy browsers, JavaScript output techniques, and various methods of accessing HTML elements. This post will cover key topics such as script MIME types, JavaScript best practices, targeting legacy browsers, which are essential for building modern web applications.
Key Highlights of the Day:
MIME Types for JavaScript:
JavaScript can be served with different MIME types depending on how it is used. For example, when used with HTML in browsers, the MIME type is usually "text/javascript." However, JavaScript with a Babel compiler or a module system requires different MIME types.Strict Mode in JavaScript:
JavaScript is not strictly typed by default, which can lead to unexpected behaviors. Enabling "strict mode" forces developers to write cleaner, error-free code by following stricter standards.Targeting Legacy Browsers:
Developers can target legacy browsers by enclosing modern JavaScript code inside HTML comments. This technique allows old browsers to safely ignore newer code.JavaScript Methods for Accessing HTML Elements:
JavaScript provides several methods to target HTML elements, such as document.getElementById(), document.getElementsByClassName(), and document.querySelector().
Deep Dive into Today’s Concepts:
1. Understanding MIME Types for JavaScript:
JavaScript can be served with different MIME types depending on the environment in which it’s being executed. The most common MIME type is text/javascript, but there are others for specific use cases:
text/javascript: Standard MIME type for JavaScript.
text/babel: Used when JavaScript is compiled using Babel.
text/module: Used when working with JavaScript modules.
These MIME types define how the browser interprets the JavaScript code.
Example Syntax:
<script type="text/javascript"> </script>
<script type="text/babel"> </script>
<script type="module"> </script>
2. Exploring Strict Mode in JavaScript:
Strict mode enforces a more disciplined version of JavaScript, helping developers avoid common coding pitfalls. For instance, with strict mode enabled, attempting to use an undeclared variable will result in an error.
How to enable strict mode:
By adding "use strict"; at the beginning of a script or function, strict mode is activated.
Example:
<script>
"use strict";
x = 10; // This will throw an error because x is not declared.
document.write("x=" + x);
</script>
3. Targeting Legacy Browsers:
In certain cases, developers need to ensure that newer JavaScript features work with older browsers. One method is to enclose modern JavaScript inside HTML comments, which older browsers will ignore.
Syntax:
<script type="text/javascript">
<!--
"use strict";
function PrintPage() {
window.print();
}
-->
</script>
Challenge with this technique:
- It can slow down page rendering and isn’t reusable across multiple pages.
4. Methods for Accessing HTML Elements:
JavaScript provides several ways to access HTML elements, from using basic DOM navigation to advanced CSS selectors. These methods allow JavaScript to interact dynamically with the page.
- Using DOM hierarchy: JavaScript can access HTML elements directly by navigating through the DOM (Document Object Model) structure.
Example:
window.document.images[0].src = "public/images/shoe.jpg";
window.document.images[0].width = 200;
- Using element "name" attribute: Elements can be accessed by their name attribute, which is particularly useful in forms.
Example:
<img name="pic" />
<script>
pic.src = "public/images/shoe.jpg";
</script>
- Using element id attribute: The id attribute is unique and can be used to select a specific element.
Example:
document.getElementById("pic").src = "public/images/shoe.jpg";
- Using CSS selectors: JavaScript can select elements using CSS selectors, providing more flexibility.
Example:
document.querySelector("img").src = "public/images/shoe.jpg";
document.querySelector("#btnRegister").value = "Register";
Code Snippets/Project Work:
Here’s a practical example of a simple JavaScript function that interacts with HTML elements:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Delete Confirmation</title>
<script type="text/javascript">
function DeleteClick() {
flag = confirm("Delete\nRecord will be deleted");
if (flag == true) {
document.querySelector("h2").innerHTML = "<font color=red>Delete Confirmed</font>";
document.querySelector("p").innerHTML = "<i><font color=red>Record Deleted Successfully..</font></i>";
} else {
alert("You canceled..");
}
}
</script>
</head>
<body>
<button onclick="DeleteClick()">Delete</button>
<h2></h2>
<p></p>
</body>
</html>
- Explanation:
The above example uses confirm() for a confirmation dialog. Depending on the user’s response, the page content is updated dynamically using innerHTML.
Challenges and How I Overcame Them:
Challenge 1: Understanding and implementing strict mode.
- Solution: I practiced writing JavaScript code with and without strict mode to understand its impact on error detection and debugging.
Challenge 2: Targeting legacy browsers with embedded scripts.
- Solution: I explored different methods of supporting older browsers, such as using HTML comments to hide newer JavaScript features from legacy browsers.
What I Learned:
Key Takeaway 1: JavaScript’s flexibility with MIME types allows developers to write code that works across different environments, such as with or without a compiler like Babel.
Key Takeaway 2: Strict mode is an invaluable tool for ensuring code quality and adherence to best practices.
Key Takeaway 3: There are multiple ways to interact with HTML elements in JavaScript, each with its use cases, from basic DOM navigation to CSS selectors.
Reflections and Closing Thoughts:
Today’s session helped solidify my understanding of core JavaScript features and best practices. I’m excited to continue learning about how JavaScript interacts with the web and how it can be used to create dynamic, responsive applications.
Subscribe to my newsletter
Read articles from Aditya Singh directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Aditya Singh
Aditya Singh
Passionate BTech student on a journey to explore and master technology. I love learning new skills, tackling challenges, and sharing my knowledge. Currently diving deep into software development, cloud computing, and everything in between!