Understanding JavaScript Classes: A Beginner's Guide

Table of contents
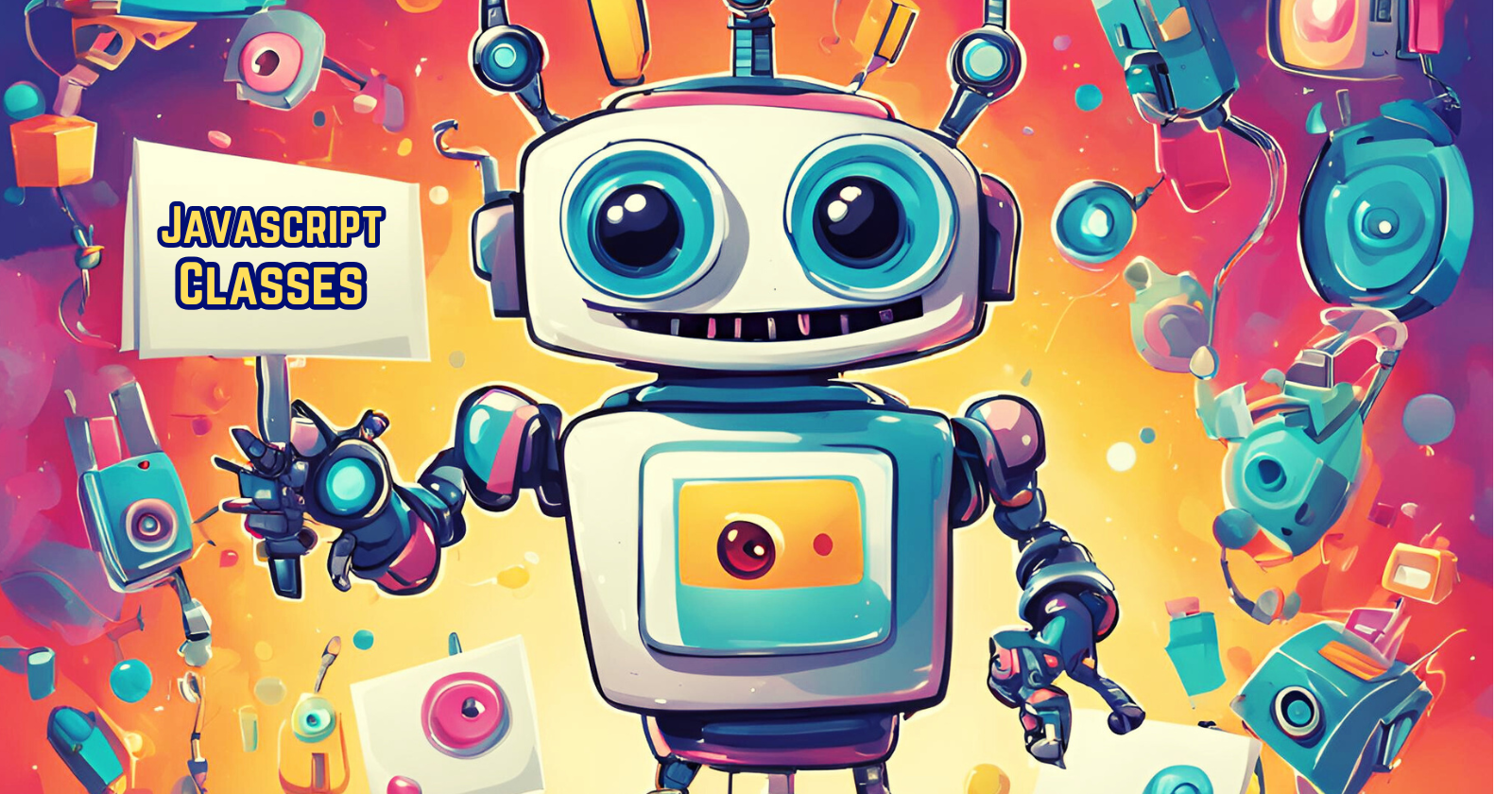
In simple terms, a class in JavaScript is like a recipe or blueprint for creating objects. It bundles together the data (called properties) and actions (called methods) that those objects will have.
The object I mention here is different from the data structure called object. Here, object is just an entity in the code made from the blueprint we provide during the class definition.
An example……
class Person {
constructor(name, age) {
this.name = name; // property
this.age = age; // property
}
greet() { // method
console.log(`Hello, my name is ${this.name} and I am ${this.age} years old.`);
}
}
The $
sign in the greet
method is part of a template literal in JavaScript, a feature that allows embedding expressions inside strings .This helps us to execute expressions and insert variables without using “+“(concatenation).Also, we haven’t used quotes here because when we use $
we must use ``
(backticks) instead.
Key Components of a Class
Constructor: The
constructor
is a special method used to initialize the object's properties when a new instance is created. [instance refers to object]Methods: Methods are functions defined within a class. They define the behavior of the objects created from the class ==>
greet()
Properties: Properties are the data attributes of the objects ==>
name, age
.
Every object created from a class will have its own copy of the properties but will share the methods defined in the class.
Why do we use this
keyword in the constructor?
The purpose of this
keyword in the constructor is to refer to the object that owns the method.
const person1 = new Person('Hopper', 45);
const person2 = new Person('Joyce', 40);
person1.greet(); // Output: Hello, my name is Hopper and I am 45 years old.
person2.greet(); // Output: Hello, my name is Joyce and I am 40 years old.
Here, we are creating two persons (objects) using the class Person as our blueprint. We enter name and age as the data and use the method greet()
we had defined inside.
Summary:
Class: The blueprint (Person).
Object: The actual person (e.g.,
person1
andperson2
).Constructor: Initializes the object's properties.(
name
andage
)Method: Functions (like
greet()
) that define behavior for the objects.
"Stay tuned for more reads through my web development journey. I hope to see you in the next post too! That's all from me for now... see ya! ✋"
The knowledge shared in this blog is based on my learnings from “Complete Web development + Devops Cohort” by Harkirat Singh. You can check out the course here:go to Harkirat's course website.
Subscribe to my newsletter
Read articles from Hitesh Venugopalan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Hitesh Venugopalan
Hitesh Venugopalan
Hey there! I'm a college student majoring in Computer Science, and this blog is my way of documenting my journey from having scattered bits of programming knowledge to building meaningful projects. Join me as I dive into the world of web development (for now), and watch as I explore other exciting paths in tech along the way. I invite you to follow along, learn with me, and share your insights or guidance when I need it. Whether you’re a beginner or a seasoned developer, there’s something here for everyone as we voyage through the ever-evolving world of coding. P.S. I may not still be a beginner when you stumble across this blog, but you’ll get to see where it all began and how far I've come!