GSAP With React

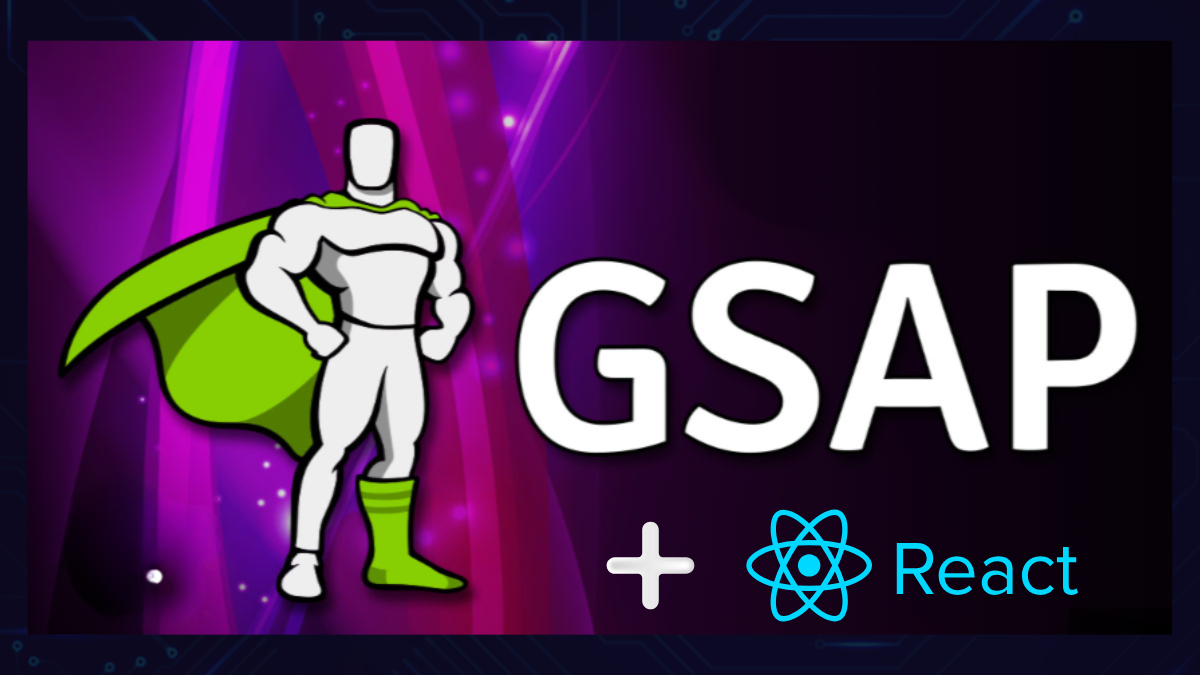
Introduction to GSAP
GreenSock Animation Platform (GSAP) is a powerful JavaScript library for creating smooth, high-performance animations. When combined with React, it enables developers to create seamless, professional-grade animations. This blog will guide you through using the custom useGSAP
hook in a React project to enhance your animations.
Why Use GSAP?
Highly performant, even for complex animations.
Smooth transitions and effects.
Control over timelines, easing, and staggering.
Compatibility with modern browsers.
What is useGSAP
(hook)?
The useGSAP
hook is not an official GSAP feature but a community-driven pattern that leverages React hooks to integrate GSAP animations smoothly within React components. By using a custom hook like useGSAP
, we can encapsulate GSAP animation logic in a reusable way, keeping our components clean and maintainable.
Implementation
1. Setting Up a React Project :-
Before we dive into animations, let’s set up a new React project:
npx create-react-app gsap-react
cd gsap-react
npm install gsap
2. Creating the useGSAP
Custom Hook :-
let’s create a custom hook to simplify how we use GSAP within our components.
import { useEffect, useRef } from 'react';
import { gsap } from 'gsap';
const useGSAP = (animation, dependencies = []) => {
const elementRef = useRef(null);
useEffect(() => {
if (elementRef.current) {
animation(elementRef.current);
}
}, dependencies);
return elementRef;
};
export default useGSAP;
Explanation:-
This hook accepts an animation function and an array of dependencies.
It uses
useRef
to reference the DOM element.The
useEffect
hook ensures that the animation runs when dependencies change.
3. Using the Hook in a Component :-
Let’s create a component that uses our useGSAP
hook to animate a box:
BoxComponent.jsx
:-
import React from 'react';
import useGSAP from './useGSAP';
import { gsap } from 'gsap';
const BoxComponent = () => {
const boxRef = useGSAP((el) => {
gsap.to(el, { x: 200, duration: 2, ease: 'power2.out' });
});
return (
<div
ref={boxRef}
style={{ width: '100px', height: '100px', backgroundColor: 'lightblue' }}
>
Animate Me
</div>
);
};
export default BoxComponent;
Explanation:-
The
useGSAP
hook is used to animate the box when the component mounts.We pass a function to
useGSAP
that animates the element to move 200 pixels to the right.
4. Adding Scroll Animations :-
Let’s extend the functionality by adding a scroll-triggered animation using
ScrollTrigger
from GSAP:
npm install gsap@3.11.5
ScrollAnimation.js
:
import React from 'react';
import { gsap } from 'gsap';
import { ScrollTrigger } from 'gsap/ScrollTrigger';
import useGSAP from './useGSAP';
gsap.registerPlugin(ScrollTrigger);
const ScrollAnimation = () => {
const scrollRef = useGSAP((el) => {
gsap.fromTo(
el,
{ opacity: 0 },
{
opacity: 1,
scrollTrigger: {
trigger: el,
start: 'top 80%',
end: 'bottom 30%',
toggleActions: 'play none none reverse',
},
}
);
});
return (
<div ref={scrollRef} style={{ height: '300px', marginTop: '100vh' }}>
Scroll to see me fade in!
</div>
);
};
export default ScrollAnimation;
5. Bringing It All Together :-
Now, let’s use both components in our main application:-
App.js
:
import React from 'react';
import BoxComponent from './BoxComponent';
import ScrollAnimation from './ScrollAnimation';
const App = () => {
return (
<div>
<h1>GSAP Animations with React</h1>
<BoxComponent />
<ScrollAnimation />
</div>
);
};
export default App;
6. Running the Application :-
To see the animations in action:
npm start
Open your browser at http://localhost:3000/
, and you should see the animations happening as intended!
Subscribe to my newsletter
Read articles from Yashaswi Sahu directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
