Essential AWS Services Every Developer Should Know

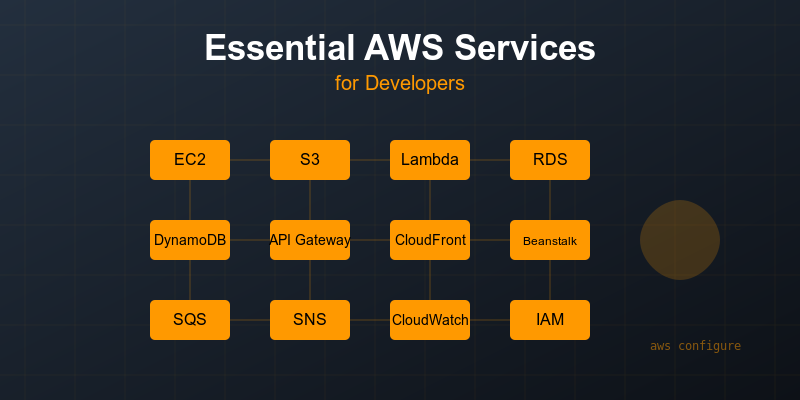
Introduction
Hey fellow developers! Let's break down the most crucial AWS services you'll need in your development journey. I'll explain each service, its core features, and when to use it. No fluff - just practical knowledge.
1. Amazon EC2 (Elastic Compute Cloud)
What: Virtual servers in the cloud
Key Features:
bashCopy# Instance types example
t2.micro # Free tier, good for learning
t3.medium # Production workloads
c5.xlarge # Compute-intensive tasks
When to Use:
Hosting web applications
Running development environments
Processing batch jobs
Running containerized applications
Cost-Saving Tips:
Use Spot Instances for non-critical workloads
Schedule dev instances to shut down after work hours
Right-size your instances based on CloudWatch metrics
2. Amazon S3 (Simple Storage Service)
What: Object storage service
Common Operations:
javascriptCopy// S3 operations example
const AWS = require('aws-sdk');
const s3 = new AWS.S3();
// Upload file
await s3.putObject({
Bucket: 'my-bucket',
Key: 'file.txt',
Body: 'Hello World'
}).promise();
// Download file
const data = await s3.getObject({
Bucket: 'my-bucket',
Key: 'file.txt'
}).promise();
Best Use Cases:
Static website hosting
Application assets
Data backup
Content distribution source
3. Amazon RDS (Relational Database Service)
What: Managed relational databases
Supported Engines:
PostgreSQL
MySQL
MariaDB
Oracle
SQL Server
Aurora
Key Features:
yamlCopy# Example RDS configuration
Database:
Engine: postgres
Version: 13.4
InstanceClass: db.t3.micro
MultiAZ: true
AutoBackup: true
BackupRetention: 7
4. AWS Lambda
What: Serverless compute service
Example Function:
pythonCopydef lambda_handler(event, context):
# Process API request
return {
'statusCode': 200,
'body': 'Hello from Lambda!'
}
Perfect For:
API endpoints
Data processing
Scheduled tasks
Event-driven processes
5. Amazon DynamoDB
What: NoSQL database service
Key Concepts:
javascriptCopy// Table design example
const table = {
TableName: 'Users',
KeySchema: [
{ AttributeName: 'userId', KeyType: 'HASH' },
{ AttributeName: 'timestamp', KeyType: 'RANGE' }
],
ProvisionedThroughput: {
ReadCapacityUnits: 5,
WriteCapacityUnits: 5
}
};
Best For:
High-scale applications
Real-time data processing
Session management
Gaming leaderboards
6. Amazon CloudFront
What: Content Delivery Network (CDN)
Use Cases:
Static asset delivery
Dynamic content acceleration
Video streaming
Security at the edge
7. Amazon API Gateway
What: Managed API service
Example Configuration:
yamlCopy# API Gateway definition
paths:
/users:
get:
integration:
type: AWS_PROXY
uri: arn:aws:lambda:region:function:GetUsers
post:
integration:
type: AWS_PROXY
uri: arn:aws:lambda:region:function:CreateUser
8. AWS Elastic Beanstalk
What: Platform as a Service (PaaS)
Supported Platforms:
Node.js
Python
Java
.NET
Go
Ruby
Docker
9. Amazon SQS (Simple Queue Service)
What: Managed message queuing service
Code Example:
pythonCopy# Send message
sqs.send_message(
QueueUrl='queue_url',
MessageBody='Task data',
DelaySeconds=0
)
# Receive message
messages = sqs.receive_message(
QueueUrl='queue_url',
MaxNumberOfMessages=1
)
10. Amazon SNS (Simple Notification Service)
What: Pub/sub messaging service
Common Uses:
Application alerts
Email notifications
SMS notifications
Push notifications
Development Tools
AWS CLI Essential Commands:
bashCopy# S3 operations
aws s3 cp file.txt s3://my-bucket/
aws s3 sync . s3://my-bucket/
# EC2 operations
aws ec2 describe-instances
aws ec2 start-instances --instance-ids i-1234567890abcdef0
# Lambda operations
aws lambda list-functions
aws lambda invoke --function-name MyFunction output.txt
Development Setup Checklist:
Install AWS CLI
Configure credentials
Set up IAM users and roles
Enable MFA
Install SDK for your language
Set up local AWS profiles
Best Practices
Security:
Use IAM roles instead of access keys
Enable MFA for all users
Follow the principle of least privilege
Regularly rotate credentials
Use AWS Secrets Manager for sensitive data
Cost Management:
Set up billing alerts
Use the AWS Cost Explorer
Leverage the AWS Free Tier
Clean up unused resources
Use cost allocation tags
Performance:
Use CloudWatch for monitoring
Set up automated scaling
Use caching where possible
Optimize database queries
Use appropriate instance types
Integration Examples
Common Architecture Pattern:
plaintextCopyWeb App -> API Gateway -> Lambda -> DynamoDB
-> S3 (static assets)
-> CloudFront (CDN)
Debugging Tools
CloudWatch Logs
X-Ray for tracing
CloudTrail for API activity
VPC Flow Logs
CloudWatch Metrics
Getting Started Steps
Create an AWS Account
Set up billing alerts
Create an IAM admin user
Install development tools
Start with basic services (S3, EC2)
Gradually explore advanced services
Resources for Learning
AWS Free Tier
AWS Documentation
AWS Workshops
AWS Training and Certification
AWS re:Invent videos
Remember: Always start small, understand the pricing model, and scale as needed. AWS can be overwhelming at first, but focus on services that solve your immediate problems.
#AWS #CloudComputing #DevOps #Programming
Subscribe to my newsletter
Read articles from Gedion Daniel directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Gedion Daniel
Gedion Daniel
I am a Software Developer from Italy.