Easy React Animation with GSAP
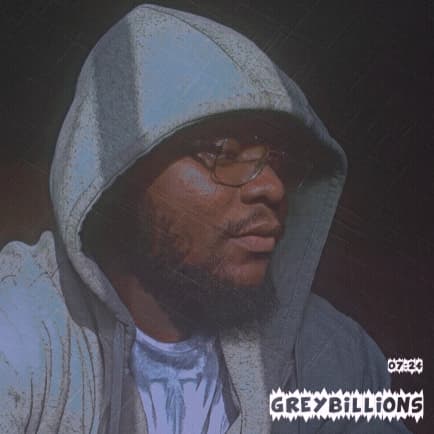
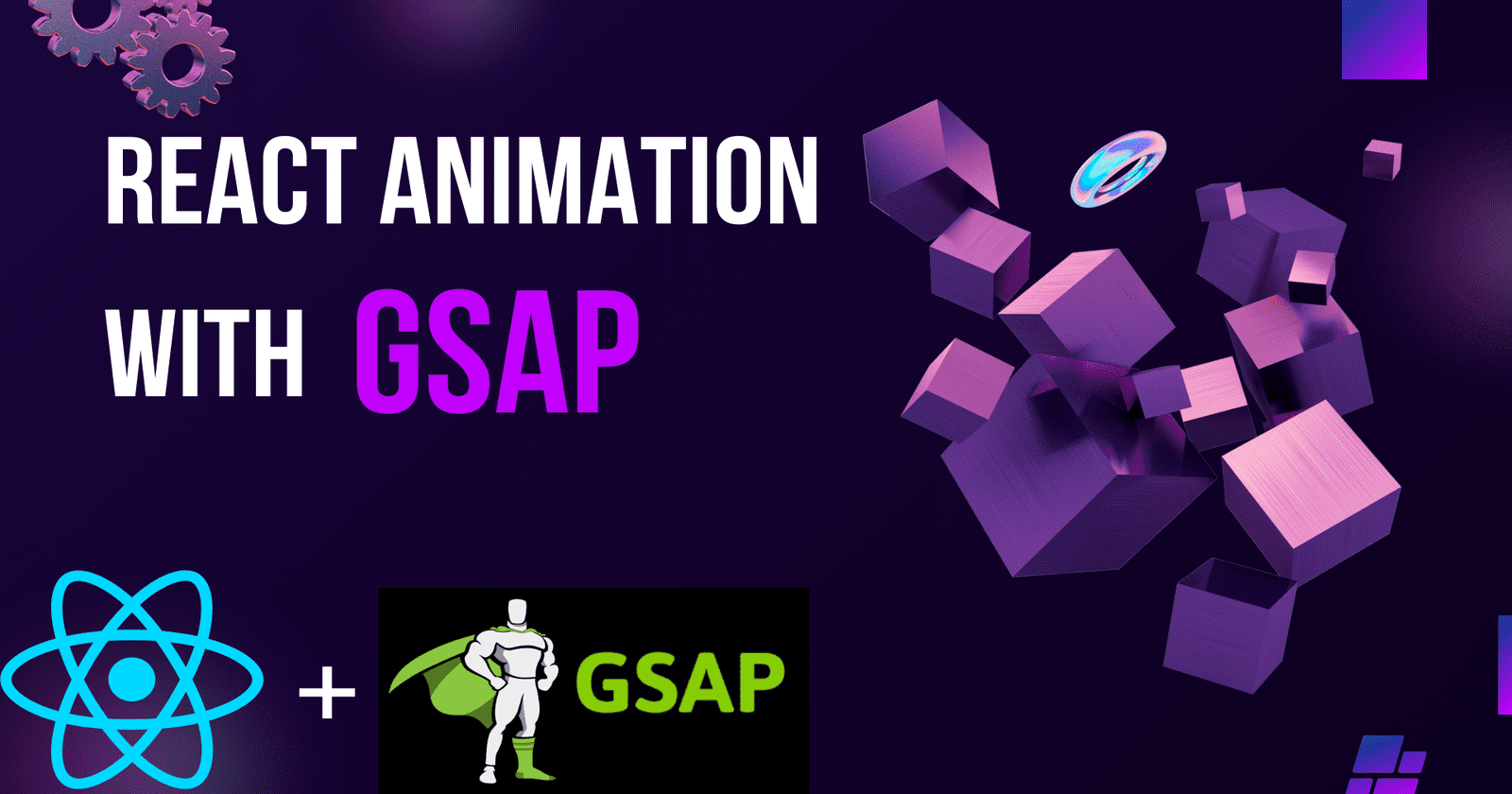
Are you tired of static web applications? Want to stand out from the v0.dev bot crowd with eye-catching animations? Popular websites like Awwwards, Dribbble, and Behance use GSAP to create engaging user interfaces.
By the end of this article, you'll know how to install and set up GSAP
, create both basic and complex animations, and use useGSAP()
hooks to enhance your React projects.
Let’s get started!
Prerequisite
To follow this guide on animating React components, ensure you have basic knowledge of the following:
React Fundamentals
JavaScript Essentials
Tailwind CSS
Development Setup: Have a code editor (VScode recommended), Node.js, npm, a React project setup, and Tailwind CSS installed.
With these prerequisites, you're all set to start animating React components using GSAP
!
What is GSAP?
GSAP, or the GreenSock Animation Platform, is a well-known JavaScript library for creating high-performance web animations. It is praised for its smooth, efficient, and versatile animations.
It is widely used in both simple websites and complex interactive applications.
To master GSAP, it's crucial to understand Tweens and Timelines:
Tween
In GSAP, tweens are the fundamental elements of animation. Over time, they smoothly change an object's properties, such as position, scale, or opacity.
Think of a Tween as the engine driving smooth animations. You select the targets (the objects you want to animate), set a duration, and decide which properties to change.
The Tween calculates and updates these properties at each step, creating a smooth transition.
Examples of tweens used for simple animations:
gsap.to()
gsap.from()
gsap.fromTo()
gsap.to(".box", { rotation: 360, x:800, duration:2 }):
This code snippet rotates the element with the class .box
by 360 degrees and moves it 800 pixels to the right in 2 seconds.
Note: "x" is a shortcut for a translateX() transform, just like "y" is for translateY() transform.
Here is the output:
Let's take a closer look:
The image above shows that there are three main components: a method, a target, and the vars (short for variables) that contain the animation properties.
Let's explore each part in more detail:
Method
There are four types of methods in GSAP:
gsap.to()
: Animates an element from its current state to a new state.gsap.from()
: Begins the animation from a specified state and moves to the element's original state.gsap.fromTo()
: Let you set both the starting and ending states for precise control.gsap.set()
: Used to position or style elements initially, getting them ready for animation.
Target
These are the elements you want to animate. You specify targets using CSS selectors, DOM elements, or even variables and arrays of elements.
For example:
//using id or class
gsap.to(".box", { rotation: 360 });
//using CSS selector
gsap.to("div > .box", { rotation: 180 });
//using a variable
Let box = document.querySelector(".box")
gsap.to("box", { scale: 3 });
//using arrays
Let box = document.querySelector(".box")
Let text = document.querySelector(".title")
gsap.to([ box, text ], { x: 300, opacity: 1 });
In summary, targets specify the elements you want to animate.
Variables
In GSAP, vars are the properties that define how an animation behaves. They include the end-state properties, duration, and easing of the animation.
For example:
gsap.to(".box", {
//vars
x: 200,
opacity: 0.5,
duration: 1,
ease: "power2.out"
});
Vars are the settings that define what the animation does and how it behaves.
Timeline
A timeline creates and organizes multiple animations (tweens) in a sequence. With timelines, you can easily manage complex animations' order, timing, and flow.
Timelines provide features that simplify and add flexibility to managing animations. They let you create a sequence of multiple animation steps, playing each one in the order you specify.
Additionally, timelines make it easy to overlap animations or add staggered delays, creating smoother and more dynamic visual effects.
To create a timeline, use gsap.timeline()
as shown below:
//create the timeline
gsap.timeline()
//initialize a timeline
const tl = gsap.timeline()
//adding tweens to the timeline
tl.to(".box", { x: 800, duration: 2.5, rotate: 360 });
//chaining tween methods to timeline
tl.to('#box1', { x: 800, duration: 2.5, rotate: 360 })
.to('#box2', { x: 800, duration: 3, scale: 1.5 })
.to('#box3', { x: 800, duration: 3, rotate: -360 });
The result of the chained tween method is:
You see how they animate one after another? Very cutesy, mindful, and demure.
What is the useGSAP() hook?
Let's examine the useGSAP
hook and understand its importance.
The useGSAP
hook is not a built-in feature of the GSAP or React libraries. Instead, it is a custom React hook that developers create to manage GSAP animations in a React project.
This hook allows for reusable GSAP animation logic and integrates seamlessly with React's lifecycle methods, making it easier to trigger animations when components mount, unmount, update, or respond to user interactions.
It lets you animate DOM elements, SVGs, three.js, canvas, or WebGL, providing endless creative possibilities.
useGSAP()
serves as an alternative to useEffect()
or useLayoutEffect()
.
Installing GSAP
To use GSAP in a React project, you need to install it first. You can do this by using the @gsap/react
library specifically designed for React projects.
To install GSAP, open your terminal and navigate to your React project. Then, run the following command:
#install gsap library/gsap react package
npm install gsap @gsap/react
#run the project
npm run dev
You can now import GSAP into your component using this syntax:
import gsap from 'gsap'
import { useGSAP } from '@gsap/react';
Now, you're ready to use it in your React app! You can begin animating elements in your components with tweens.
Basic Animations with GSAP & useGSAP
Creating animations with GSAP is easy and highly customizable.
Here is a simple example of how to use gsap
and useGSAP
effectively:
import gsap from 'gsap';
import { useGSAP } from '@gsap/react';
const App = () => {
useGSAP(() => {
gsap.to('#hero-title', {
y: -150,
duration: 2.5,
opacity: 1,
ease: 'power2.inOut'
})
}, []);
return (
<div className='w-full h-[100vh] flex flex-col items-center justify-center'>
<div
className='w-full h-full flex flex-col gap-6 items-center justify-center'
>
<h1 id="hero-tile" className='text-4xl font-bold opacity-0'>Hello World</h1>
</div>
</div>
);
};
export default App;
The result of the code snippet above:
Using useGSAP
, you can define animations clearly and efficiently, making sure they work smoothly within the React lifecycle. This approach is perfect for creating dynamic, interactive user interfaces with minimal code.
GSAP ScrollTrigger Plugin
Developers can load only the plugins they need, which keeps GSAP lightweight and efficient while still offering advanced animation features when necessary.
The ScrollTrigger plugin is a powerful tool for creating animations that activate or are controlled by scrolling.
It lets you sync animations with the scroll position of a page or container, enabling effects like parallax, pinning, scrubbing, and revealing content as users scroll.
To use the ScrollTrigger plugin, import it from gsap
.
For example:
import gsap from 'gsap';
import ScrollTrigger from 'gsap/ScrollTrigger';
gsap.registerPlugin(ScrollTrigger);
If you look closely at the last line of the code snippet above, you'll see the plugin being registered. Registering GSAP plugins is like adding special tools to your animation toolkit, ensuring your code is bug-free.
By registering a plugin, you enable GSAP to access its features.
Here's a simple example of using ScrollTrigger to animate an element when you scroll:
import gsap from 'gsap';
import { useGSAP } from '@gsap/react';
import ScrollTrigger from 'gsap/src/ScrollTrigger';
gsap.registerPlugin(ScrollTrigger);
const GsapExample = () => {
useGSAP(() => {
gsap.to('#box', {
scrollTrigger: {
trigger: '#box',
start: 'top 90%',
},
x: 400,
opacity: 1,
duration: 3,
ease: 'power2.inOut',
});
}, []);
return (
<div>
<div className='h-[100vh] bg-blue-500 flex justify-center items-center'>
<p className='text-7xl'>Section 1</p>
</div>
<div className='h-[100vh] flex items-center'>
<div id='box' className='w-80 h-80 opacity-0 bg-red-500 rounded'></div>
</div>
<div className='h-[100vh] bg-blue-500 flex justify-center items-center'>
<p className='text-7xl'>Section 3</p>
</div>
</div>
);
};
export default GsapExample;
Output:
You can customize when an animation starts and ends using trigger points (trigger
, start
, end
), sync animations with the scroll position using scrub
, and pin elements in place as the user scrolls with pin
.
Debugging tools like markers
help visualize trigger points during development. You can also add interactivity with callbacks such as onEnter
and onLeave
.
GSAP Stagger Property
The GSAP stagger
option allows you to animate multiple elements one after another with a slight delay between each.
This creates impressive visual effects, like items moving in sequence, cascading animations, or smooth wave-like motions.
For example:
import gsap from 'gsap';
import { useGSAP } from '@gsap/react';
const GsapExample = () => {
useGSAP(() => {
gsap.to('#box', {
y: -300,
opacity: 1,
duration: 3,
stagger: { // implemeting the stagger effect
amount: 2,
from: 'beginning',
},
ease: 'power2.inOut',
});
}, []);
return (
<div className='h-[100vh] w-full flex flex-col items-center justify-center'>
<h1 className='text-4xl text-center mb-4'>GSAP Stagger</h1>
<div className='h-[70vh] w-full flex items-end justify-center'>
{Array.from({ length: 8 }).map((_, index) => (
<div
id='box'
key={index}
className='box w-14 h-14 mr-2 opacity-0 bg-red-500 rounded'
></div>
))}
</div>
</div>
);
};
export default GsapExample;
The code snippet above animates 8 red boxes, created dynamically with a loop, using a staggered effect. Each box moves up by 300 pixels (y: -300
), fades in (opacity: 1
), and finishes the animation in 3 seconds with smooth easing (ease: 'power2.inOut'
).
The stagger
option spreads the animations evenly over 2 seconds, starting from the first box (from: 'beginning'
). The animation starts when the component mounts.
Here’s the result of the code:
The stagger
property makes it easy to animate multiple elements one after another by adding a delay between each animation.
Conclusion
GSAP is highly flexible, making it suitable for various projects like Vanilla JS, React, Vue, Angular, or Webflow. It's popular among animators, developers, and designers for creating visually appealing and efficient animations.
It can manage everything from simple hover effects to complex interactive animations. Try out its features and practice often to enhance your skills. With GSAP, the only limit is your imagination.
Further resources
Happy Coding!✨
Subscribe to my newsletter
Read articles from Graham Boyle directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
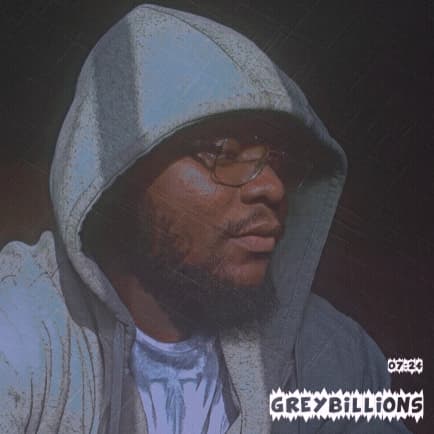
Graham Boyle
Graham Boyle
A Front-end Engineer and Technical Writer