Working with LocalStorage in JavaScript
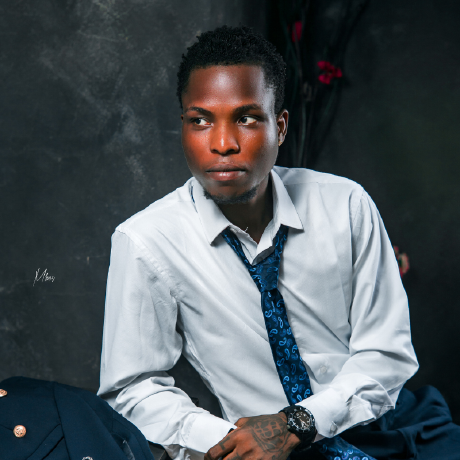
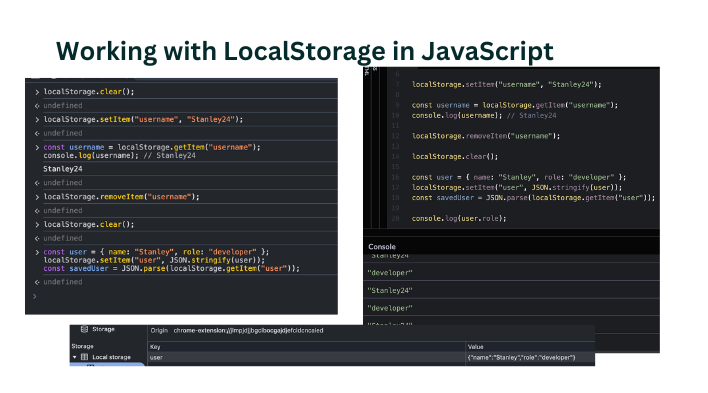
LocalStorage revolutionizes development by enabling apps to store data directly in a browser. This allows for persistent, seamless, and personalized user experiences. Today, we will delve into LocalStorage, its practical applications, and projects demonstrating its effectiveness.
What is LocalStorage?
LocalStorage is a component of the Web Storage API that allows persistent storage of key-value pairs in a user's browser.
Capacity: Up to 5MB of data.
Data Type: Stores data as strings (other types require JSON serialization).
Persistence: Data doesn’t expire, unlike SessionStorage.
How to Use LocalStorage in JavaScript
Set a Key-Value Pair:
localStorage.setItem("username", "Stanley24");
Retrieve a Value:
const username = localStorage.getItem("username"); console.log(username); // Stanley24
Delete a Key:
localStorage.removeItem("username");
Clear All Data:
localStorage.clear();
Store Complex Data (Objects/Arrays):
const user = { name: "Stanley", role: "developer" }; localStorage.setItem("user", JSON.stringify(user)); const savedUser = JSON.parse(localStorage.getItem("user"));
Practical Use Cases
Saving User Preferences: Persist settings like dark mode or language.
Shopping Cart: Save cart items across sessions.
Progress Tracking: Store tutorial or to-do list progress.
Form Autosave: Prevent data loss during accidental reloads.
Linked Projects
To-Do List with LocalStorage
Coming Soon: Shopping Cart Feature
- Retain cart data across sessions for seamless e-commerce functionality.
Coming Soon: Dark Mode Toggle
- Save theme preferences to offer a consistent look and feel.
Best Practices and Limitations
Best Practices:
Keep data sizes minimal.
Use
JSON.stringify()
andJSON.parse()
for complex objects.Avoid storing sensitive data like passwords.
Limitations:
Storage Limit: 5MB per domain.
Security Risks: Vulnerable to XSS attacks; never store sensitive information.
Synchronous Nature: Large operations can impact page performance.
Conclusion
LocalStorage is a powerful tool for enhancing user experience by storing and managing data directly in the browser. It offers endless possibilities for modern web development, from saving user preferences to enabling dynamic functionalities.
This marks Day 23 of our journey in JavaScript! 🚀
Explore our linked projects, apply your knowledge, and build smarter web applications today. Let’s grow, share, and build an incredible community together!
👉 Follow for more insights and updates as we continue this journey. Your support helps us keep learning and sharing. Join the conversation, and let’s grow our network!
Subscribe to my newsletter
Read articles from Stanley Owarieta directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
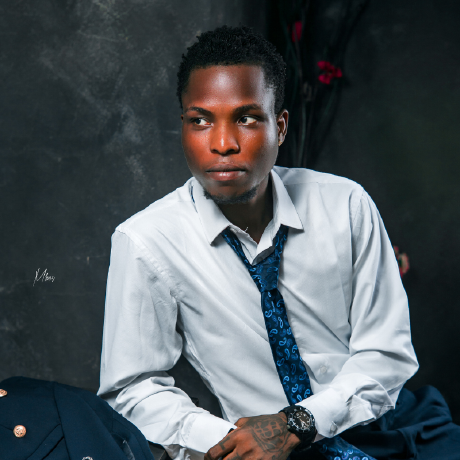
Stanley Owarieta
Stanley Owarieta
I help tech businesses and startups create engaging, SEO-driven content that attracts readers and converts them into customers. From in-depth blog posts to product reviews, I ensure every content is well-researched, easy to understand, and impactful. As a programming learner with HTML, CSS, and JavaScript knowledge, I bring a unique technical perspective to my writing. If you're looking for content that ranks and resonates, let's connect! 📩 Open to collaborations! Message me or email me at freelance@stanleyowarieta.com