About React: A Beginner's Introduction

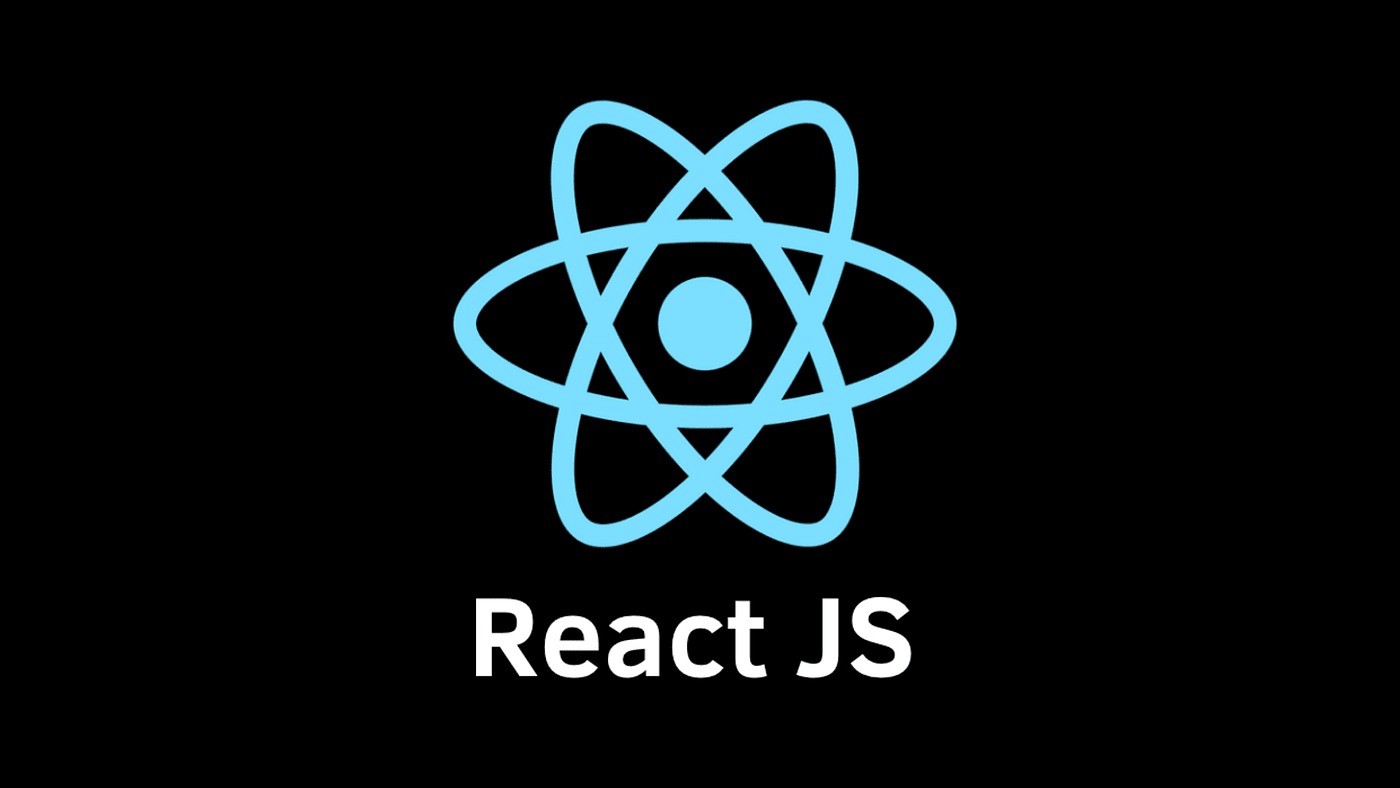
Introduction
React has become one of the most popular JavaScript libraries for building user interfaces, especially single-page applications. Created by Facebook, it’s known for making interactive and efficient UIs easier to develop. In this post, I'll introduce you to the core ideas behind React and why so many developers love using it.
What is React?
React is a JavaScript library for building reusable, component-based UIs. Unlike traditional HTML and JavaScript setups, where you handle every DOM change manually, React allows developers to build UI components that update automatically when the app’s data changes.
React is also focused on speed and efficiency. By using a "virtual DOM" (more on that below), React minimizes costly operations and helps web apps run faster.
Key Concepts of React
Here are some essential concepts you’ll encounter when working with React:
1. Components
Components are the building blocks of any React application. Think of a component as a self-contained piece of the UI, like a button, a list, or even an entire page. Components allow you to divide the UI into independent, reusable pieces.
// Example of a simple React component
function Welcome() {
return <h1>Hello, World!</h1>;
}
2. JSX
JSX (JavaScript XML) is a syntax extension for JavaScript that allows you to write HTML-like code directly in your JavaScript files. It’s an essential part of React because it makes defining UI elements easy and readable.
const element = <h1>Hello, React!</h1>;
3. State
State represents data that can change within a component. When the state of a component changes, React re-renders the component, updating the UI automatically. For example, if you have a counter component, the state would store the current count value.
import { useState } from 'react';
function Counter() {
const [count, setCount] = useState(0);
return (
<div>
<p>Count: {count}</p>
<button onClick={() => setCount(count + 1)}>Increase</button>
</div>
);
}
4. Props
Props (short for "properties") allow you to pass data from one component to another. This is essential for building components that are reusable and dynamic. For example, you can create a button component and pass it different labels via props.
function Button(props) {
return <button>{props.label}</button>;
}
Why Use React?
React has become incredibly popular for several reasons:
Reusability: Components can be reused across the app, saving time and reducing code duplication.
Efficiency: The virtual DOM in React allows efficient updating, making apps faster.
Developer-Friendly: JSX and component-based structure make the code more organized and easier to understand.
Conclusion
React is an excellent choice for building modern, fast, and dynamic web applications. Although it might feel overwhelming at first, mastering the basics—like components, state, and props—will help you build exciting projects with ease. I hope this quick intro inspires you to explore React further!
Subscribe to my newsletter
Read articles from Pragya Rajput directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Pragya Rajput
Pragya Rajput
Passionate about web development and always eager to learn new technologies. With a focus on the MERN stack and a curiosity for front-end and back-end development, I enjoy building interactive, user-friendly applications. Join me as I share my journey, insights, and tips on mastering the world of coding!