How to Detect and Prevent SQL Injection in RESTful APIs

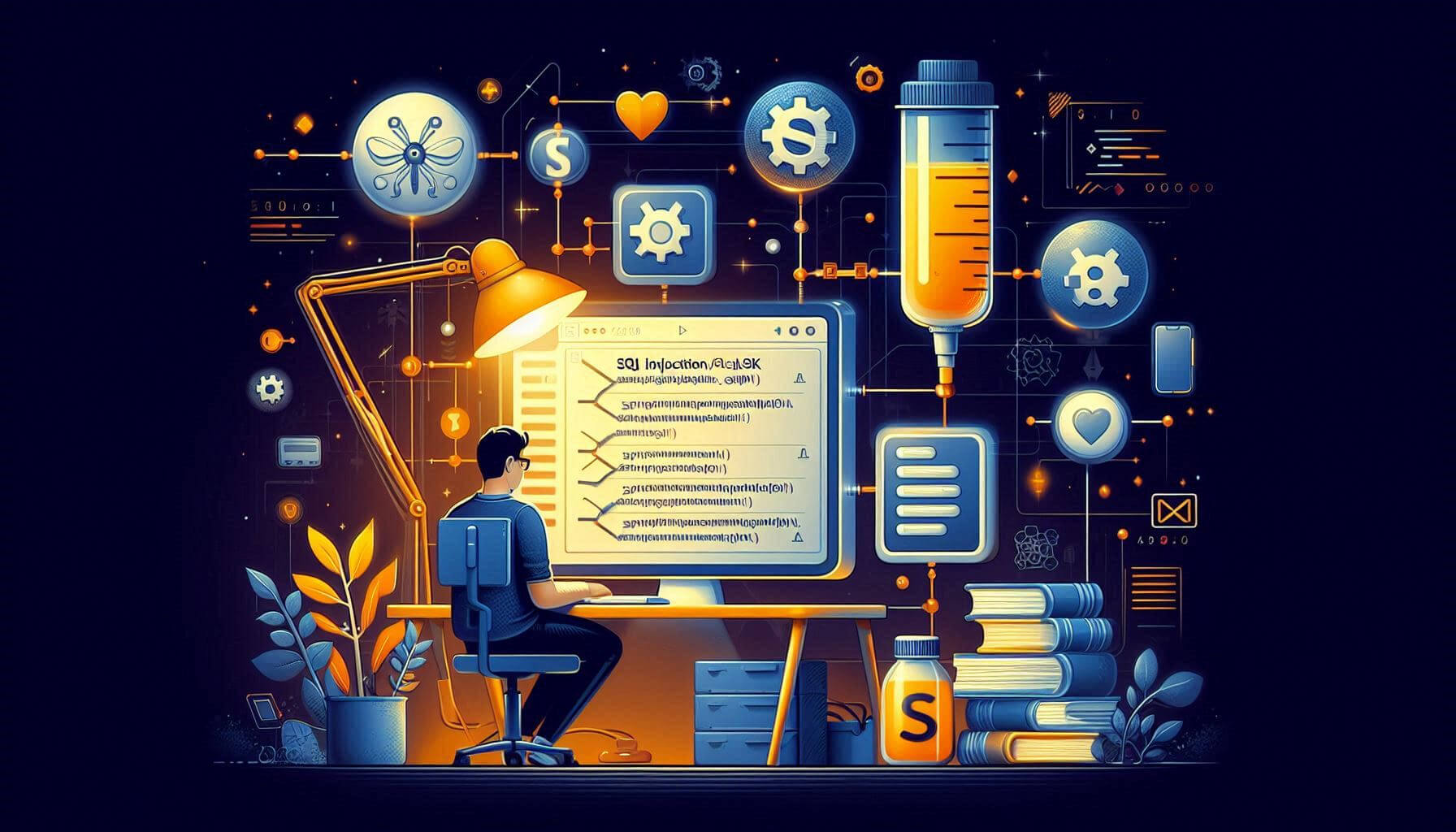
RESTful APIs are a crucial component in modern web applications. However, they are often the target of SQL Injection (SQLi) attacks, which exploit vulnerabilities in database query execution. This blog explores how SQLi can affect RESTful APIs, demonstrates a sample exploit, and highlights ways to detect and prevent such vulnerabilities.
Table of Contents
What is SQL Injection in RESTful APIs?
Common Vulnerabilities Leading to SQLi
Coding Example: SQL Injection Exploit and Fix
How to Detect SQLi with Free Tools
Best Practices to Prevent SQLi in RESTful APIs
What is SQL Injection in RESTful APIs?
SQL Injection (SQLi) occurs when malicious SQL queries are injected into API endpoints, manipulating backend databases. For instance, attackers may exploit poorly sanitized input fields to extract sensitive data or bypass authentication.
Common Vulnerabilities Leading to SQLi
Lack of input validation and sanitization
Exposing direct database queries in API endpoints
Missing parameterized queries or prepared statements
Improper handling of user inputs
Coding Example: SQL Injection Exploit and Fix
Here’s a simple example to demonstrate SQL Injection in a RESTful API endpoint.
Vulnerable Code Example
javascript
// Express.js-based API with an SQL vulnerability
app.post('/api/login', async (req, res) => {
const { username, password } = req.body;
const query = `SELECT * FROM users WHERE username = '${username}' AND password = '${password}'`;
const result = await db.execute(query); // Vulnerable to SQLi
if (result.length) res.status(200).send('Login Successful');
else res.status(401).send('Unauthorized');
});
Fixed Code Example
javascript
// Secure API endpoint using parameterized queries
app.post('/api/login', async (req, res) => {
const { username, password } = req.body;
const query = 'SELECT * FROM users WHERE username = ? AND password = ?';
const result = await db.execute(query, [username, password]); // Secured with parameters
if (result.length) res.status(200).send('Login Successful');
else res.status(401).send('Unauthorized');
});
How to Detect SQLi with Free Tools
SQLi vulnerabilities can be tricky to identify without proper tools. Our Website Security Checker can quickly scan your web application for potential security issues, including SQLi.
Best Practices to Prevent SQLi in RESTful APIs
Use Parameterized Queries: Always use parameterized queries or prepared statements to handle user inputs securely.
Implement Input Validation: Sanitize inputs to prevent malicious queries from being executed.
Apply the Principle of Least Privilege: Limit database user permissions to reduce exposure to SQLi risks.
Perform Regular Security Testing: Use tools like our Website Vulnerability Checker to proactively find and fix vulnerabilities.
Enable Web Application Firewalls (WAFs): Deploy WAFs to block known SQLi attack patterns.
Conclusion
SQL Injection in RESTful APIs is a serious threat that requires proactive measures to mitigate. By understanding how attacks occur, implementing secure coding practices, and using tools like our Website Security Checker, you can safeguard your APIs against SQLi.
Do you want to identify SQLi vulnerabilities in your web application? Try our free tool here today!
Subscribe to my newsletter
Read articles from Pentest_Testing_Corp directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Pentest_Testing_Corp
Pentest_Testing_Corp
Pentest Testing Corp. offers advanced penetration testing to identify vulnerabilities and secure businesses in the USA and UK, helping safeguard data and strengthen defenses against evolving cyber threats. Visit us at free.pentesttesting.com now to get a Free Website Security Check.