How to Use Python for DevOps: Essential Skills and Tools for Beginners (Part-2)
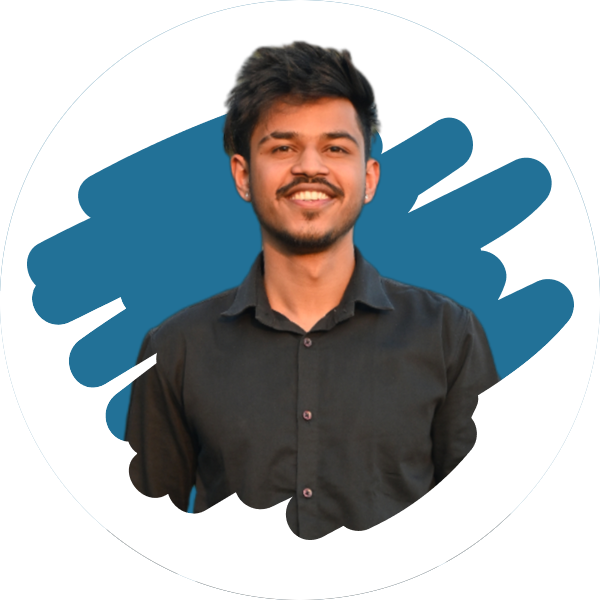
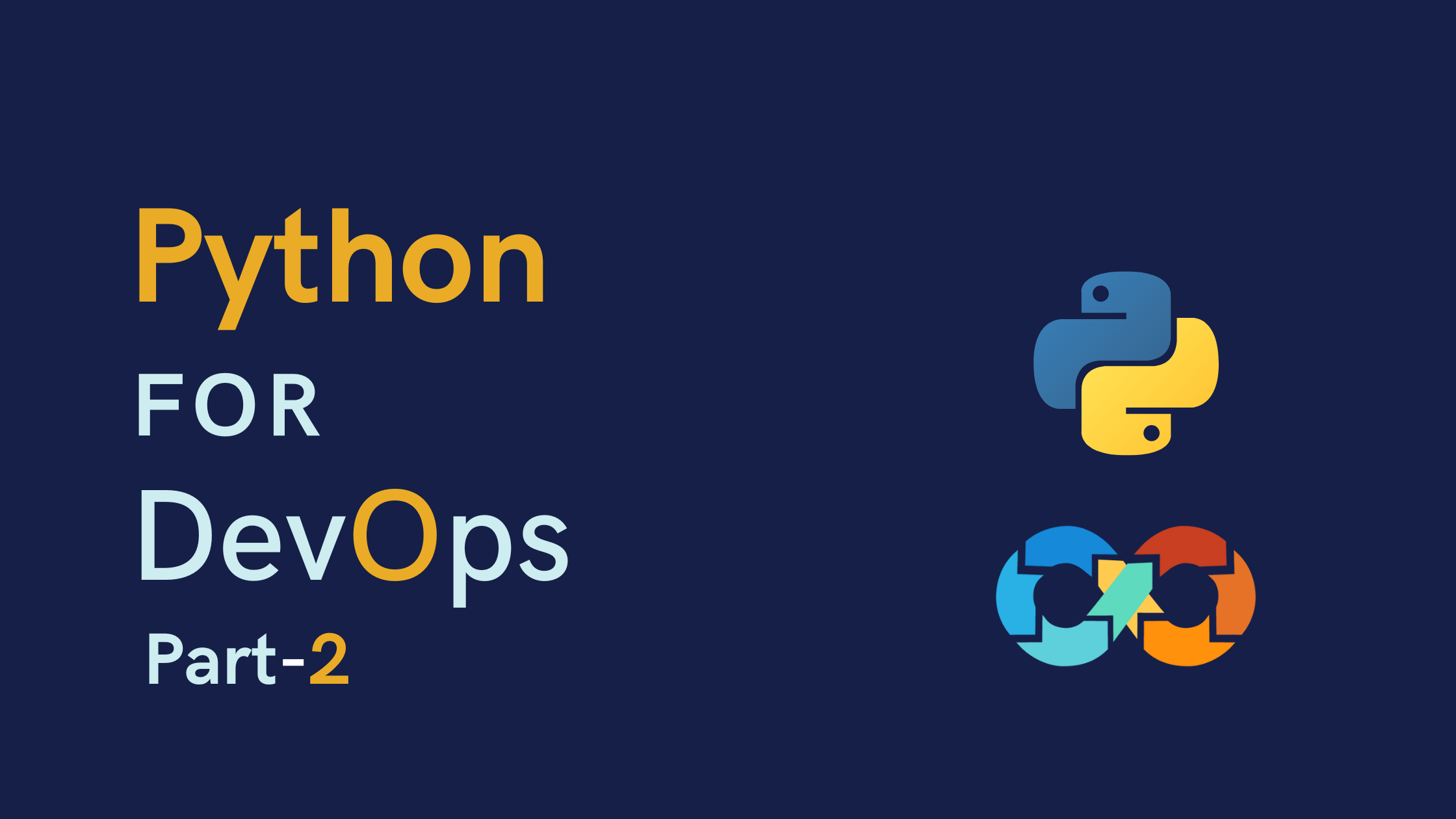
๐ก Introduction
Welcome back to our journey through Python for DevOps! ๐ In Part 1, we explored the foundational Python concepts that every DevOps engineer should know, including data types, functions, variables, and more. If you missed it, catch up here: How to Use Python for DevOps โ Essential Skills and Tools for Beginners (Part 1).
In this Part 2, weโll take things a step further. Weโll dive into more advanced topics like control flow, loops, working with files, handling errors, and using Python libraries for DevOps automation. Plus, weโll wrap it all up with a hands-on Python project to apply what weโve learned!
Letโs continue building our Python skills and see how they can supercharge your DevOps workflows.
๐ก Conditional Statements in Python
Conditional statements form the backbone of decision-making in Python. They allow us to execute specific blocks of code based on whether certain conditions are met. Python provides three main keywords for conditional logic: if
, elif
(else if), and else
.
if
Statement
The if
statement executes a block of code if the condition evaluates to True
.
x = 10
if x > 5:
print("x is greater than 5")
elif
Statement
The elif
statement is used to check additional conditions when the if
condition is False
. You can use multiple elif
statements for a series of conditions.
x = 10
if x > 15:
print("x is greater than 15")
elif x > 5:
print("x is greater than 5 but not greater than 15")
else
Statement
The else
statement executes a block of code when all the previous conditions are False
.
x = 3
if x > 5:
print("x is greater than 5")
else:
print("x is not greater than 5")
๐ก Lists and Tuples in Python
Lists
A list is one of Python's most versatile data structures. It allows you to store a collection of items that can include numbers, strings, or even other lists. Lists are mutable, meaning you can modify their contents after creation.
Example: Creating and Manipulating a List
my_list = [1, 2, 3, 'apple', 'banana']
# Accessing elements
print(my_list[0]) # Output: 1
# Adding an element
my_list.append('cherry')
# Removing an element
my_list.remove('apple')
# Getting the length of the list
print(len(my_list))
# Slicing
print(my_list[1:3]) # Output: [2, 3]
# Checking for an item
is_present = "banana" in my_list # True
# Sorting (works for lists with elements of the same type)
numbers = [4, 2, 8, 6]
numbers.sort()
print(numbers) # Output: [2, 4, 6, 8]
Tuples
Tuples are similar to lists but immutable, meaning their contents cannot be changed after creation. Tuples are often used to group related data or return multiple values from a function.
Example: Creating and Using a Tuple
my_tuple = (1, 2, 3, 'apple', 'banana')
# Accessing elements
print(my_tuple[0]) # Output: 1
# Getting the length of the tuple
print(len(my_tuple))
# Concatenation
new_tuple = my_tuple + (4, 5)
print(new_tuple) # Output: (1, 2, 3, 'apple', 'banana', 4, 5)
# Unpacking a tuple
def get_coordinates():
return (3, 4)
x, y = get_coordinates()
print(x, y) # Output: 3, 4
๐ก Differences Between Lists and Tuples
Feature | List | Tuple |
Mutability | Mutable | Immutable |
Syntax | [] (square brackets) | () (parentheses) |
Performance | Slightly slower | Faster |
Use Cases | General-purpose storage | Fixed or read-only data |
Iteration | Slower due to mutability | Faster |
Memory Usage | Higher | Lower |
๐ก Loops in Python
Loops are essential in programming as they allow us to automate repetitive tasks efficiently. Python offers two primary types of loops: for
loops and while
loops, each suited for specific scenarios.
for
Loop
A for
loop is used to iterate over a sequence (such as a list, tuple, string, or range) and execute a block of code for each element in the sequence. It automatically ends after all the elements in the sequence are processed.
Example:
fruits_list = ["apple", "banana", "mango", "grapes", "pineapple", "oranges", "pear"]
for fruit in fruits_list:
if fruit == "apple":
continue # Skip the iteration when fruit is "apple"
print(fruit)
# Output: banana, mango, grapes, pineapple, oranges, pear
Here, the continue
statement skips the current iteration and moves to the next one.
while
Loop
A while
loop repeats a block of code as long as a given condition is True
. The loop will stop executing when the condition becomes False
.
Example:
x = 0
while x <= 5:
if x == 3:
break # Exit the loop when x equals 3
print(x)
x += 1
# Output: 0, 1, 2
In this example, the break
statement exits the loop completely when the condition is met.
๐ก DevOps Use-Cases for for
Loops
1. Provisioning Multiple Servers
Automate tasks like installing monitoring agents on multiple servers:
servers=("server1" "server2" "server3")
for server in "${servers[@]}"; do
configure_monitoring_agent "$server"
done
2. Deploying Configurations
Deploy configurations to different environments (development, staging, production):
environments=("dev" "staging" "prod")
for env in "${environments[@]}"; do
deploy_configuration "$env"
done
3. Backup and Restore Operations
Automate backups for multiple databases:
databases=("db1" "db2" "db3")
for db in "${databases[@]}"; do
create_backup "$db"
done
4. Monitoring and Reporting
Monitor resource utilization across multiple servers:
servers=("server1" "server2" "server3")
for server in "${servers[@]}"; do
check_resource_utilization "$server"
done
5. Managing Cloud Resources
Resize multiple cloud instances:
instances=("instance1" "instance2" "instance3")
for instance in "${instances[@]}"; do
resize_instance "$instance"
done
๐ก DevOps Use-Cases for while
Loops
1. CI/CD Pipeline Monitoring
Wait for a Kubernetes deployment to complete:
while kubectl get deployment/myapp | grep -q 0/1; do
echo "Waiting for myapp to be ready..."
sleep 10
done
2. Log Analysis and Alerting
Monitor logs continuously for errors and send alerts:
while true; do
if tail -n 1 /var/log/app.log | grep -q "ERROR"; then
send_alert "Error detected in the log."
fi
sleep 5
done
3. Service Health Monitoring and Auto-Recovery
Check service health and restart unhealthy services:
while true; do
if ! check_service_health; then
restart_service
fi
sleep 30
done
๐ก Dictionaries and Sets in Python
Python provides powerful data structures like dictionaries and sets to handle and organize data efficiently. These structures are particularly valuable in scenarios like managing configurations, optimizing retrieval, and performing mathematical operations on data sets.
๐ก Dictionaries
A dictionary is a collection of key-value pairs, where each key must be unique. Dictionaries are implemented as hash tables, offering fast access to values using their keys.
Creating a Dictionary
my_dict = {'name': 'John', 'age': 25, 'city': 'New York'}
Accessing and Modifying Data
print(my_dict['name']) # Output: John
my_dict['age'] = 26 # Modify value
my_dict['occupation'] = 'Engineer' # Add new key-value pair
Removing Elements
del my_dict['city'] # Remove key-value pair
Checking for Key Existence
if 'age' in my_dict:
print('Age is present in the dictionary')
Iterating Through Keys and Values
for key, value in my_dict.items():
print(key, value)
Use Case: Server Configurations
Dictionaries are especially useful for managing configurations.
server_config = {
'server1': {'ip': '192.168.1.1', 'port': 8080, 'status': 'active'},
'server2': {'ip': '192.168.1.2', 'port': 8000, 'status': 'inactive'},
'server3': {'ip': '192.168.1.3', 'port': 9000, 'status': 'active'}
}
# Function for retrieving server status
def get_server_status(server_name):
return server_config.get(server_name, {}).get('status', 'Server not found')
# Example usage
server_name = 'server2'
status = get_server_status(server_name)
print(f"{server_name} status: {status}")
# Output: server2 status: inactive
๐ก Sets
A set is an unordered collection of unique elements, ideal for eliminating duplicates and performing mathematical operations like union, intersection, and difference.
Creating a Set
my_set = {3, 45, 53, 6, 3} # Duplicates are ignored
print(my_set) # Output: {3, 6, 45, 53}
Adding and Removing Elements
my_set.add(99) # Add an element
my_set.remove(3) # Remove an element
Set Operations
set1 = {1, 2, 3, 4}
set2 = {3, 4, 5, 6}
union_set = set1.union(set2) # {1, 2, 3, 4, 5, 6}
intersection_set = set1.intersection(set2) # {3, 4}
difference_set = set1.difference(set2) # {1, 2}
๐ก When to Use Sets in DevOps
Sets are useful for tasks like maintaining a unique list of servers, comparing configurations, or identifying common elements between environments.
Example: Removing Duplicate Server IPs
server_ips = [ '192.168.1.1', '192.168.1.2', '192.168.1.1', '192.168.1.3']
unique_ips = set(server_ips)
print(unique_ips) # Output: {'192.168.1.1', '192.168.1.2', '192.168.1.3'}
Example: Finding Common Issues Across Logs
log1_errors = {'ErrorA', 'ErrorB', 'ErrorC'}
log2_errors = {'ErrorB', 'ErrorC', 'ErrorD'}
common_errors = log1_errors.intersection(log2_errors)
print(common_errors) # Output: {'ErrorB', 'ErrorC'}
๐ก Using Boto3 to Automate AWS EC2 Instance Creation
In this section, we'll walk through setting up a Python script using Boto3 to automate the creation of an EC2 instance.
Python Script: aws-instance-creation.py
Below is the Python script:
import boto3
# Function to create an EC2 instance
def create_ec2_instance():
try:
# Create a session using your configured credentials
ec2_client = boto3.client('ec2', region_name='us-east-1') # Update the region as needed
# Parameters for the new EC2 instance
instance_params = {
'ImageId': 'ami-0c02fb55956c7d316', # Update with the desired AMI ID
'InstanceType': 't2.micro', # Update the instance type as needed
'KeyName': 'my-key-pair', # Update with your existing key pair name
'MinCount': 1,
'MaxCount': 1,
'SecurityGroupIds': ['sg-0abcd1234efgh5678'], # Update with your security group ID
'SubnetId': 'subnet-0abcd1234efgh5678', # Update with your subnet ID
}
# Create the instance
response = ec2_client.run_instances(**instance_params)
# Extract and print the instance ID
instance_id = response['Instances'][0]['InstanceId']
print(f"Instance created successfully! Instance ID: {instance_id}")
return instance_id
except Exception as e:
print(f"An error occurred: {e}")
if __name__ == "__main__":
# Call the function to create an EC2 instance
create_ec2_instance()
๐ก Steps to Use the Script
1. Set Up AWS CLI
Make sure your AWS CLI is configured with the necessary credentials and permissions:
aws configure
Enter your Access Key ID and Secret Access Key.
Set the default region to match the
region_name
parameter in the script (us-east-1
in this example).
2. Replace Placeholders
Update the placeholders in the script with actual values from your AWS environment:
ImageId: Use the AMI ID available in your chosen region.
KeyName: Replace with the name of an existing key pair in your AWS account.
SecurityGroupIds: Provide the security group ID for the instance.
SubnetId: Replace with the appropriate subnet ID.
3. Install Boto3
Ensure Boto3 is installed in your Python environment:
pip3 install boto3
4. Run the Script
Save the script as aws-instance-creation.py
and run it:
python aws-instance-creation.py
If everything is configured correctly, the script will create an EC2 instance and display the instance ID upon success.
Example Output
Instance created successfully! Instance ID: i-0abcd1234efgh5678
๐ก Conclusion
In this blog, we explored Python's core concepts like if-else statements, dictionaries, sets, and loops, showcasing their use in real-world DevOps scenarios such as server provisioning, monitoring, and automation. We tied these together with a practical AWS EC2 instance automation script using Boto3, demonstrating Python's power in managing cloud resources efficiently.
With these foundational skills, you're now equipped to tackle dynamic workflows and streamline DevOps processes. Stay tuned for more advanced topics in upcoming blogs! ๐
๐ For more informative blog, Follow me on Hashnode, X(Twitter) and LinkedIn.
Till then, Happy Coding!!
Subscribe to my newsletter
Read articles from Pravesh Sudha directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
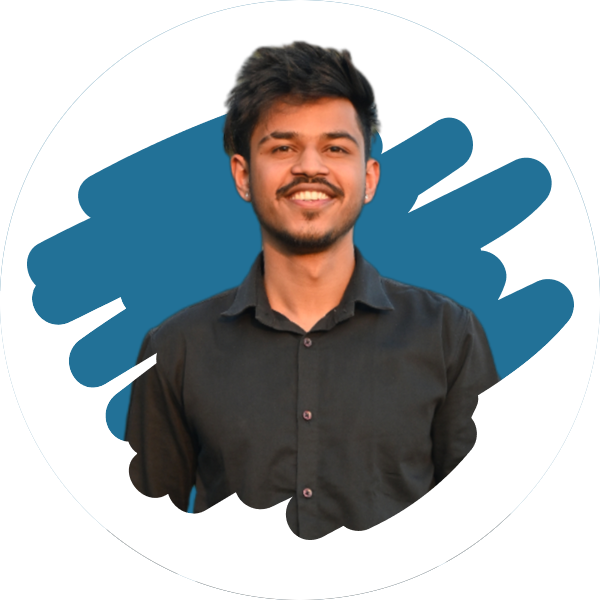
Pravesh Sudha
Pravesh Sudha
Bridging critical thinking and innovation, from philosophy to DevOps.