The Mystery of if __name__ == '__main__': in Python – Explained with a Post Office Analogy

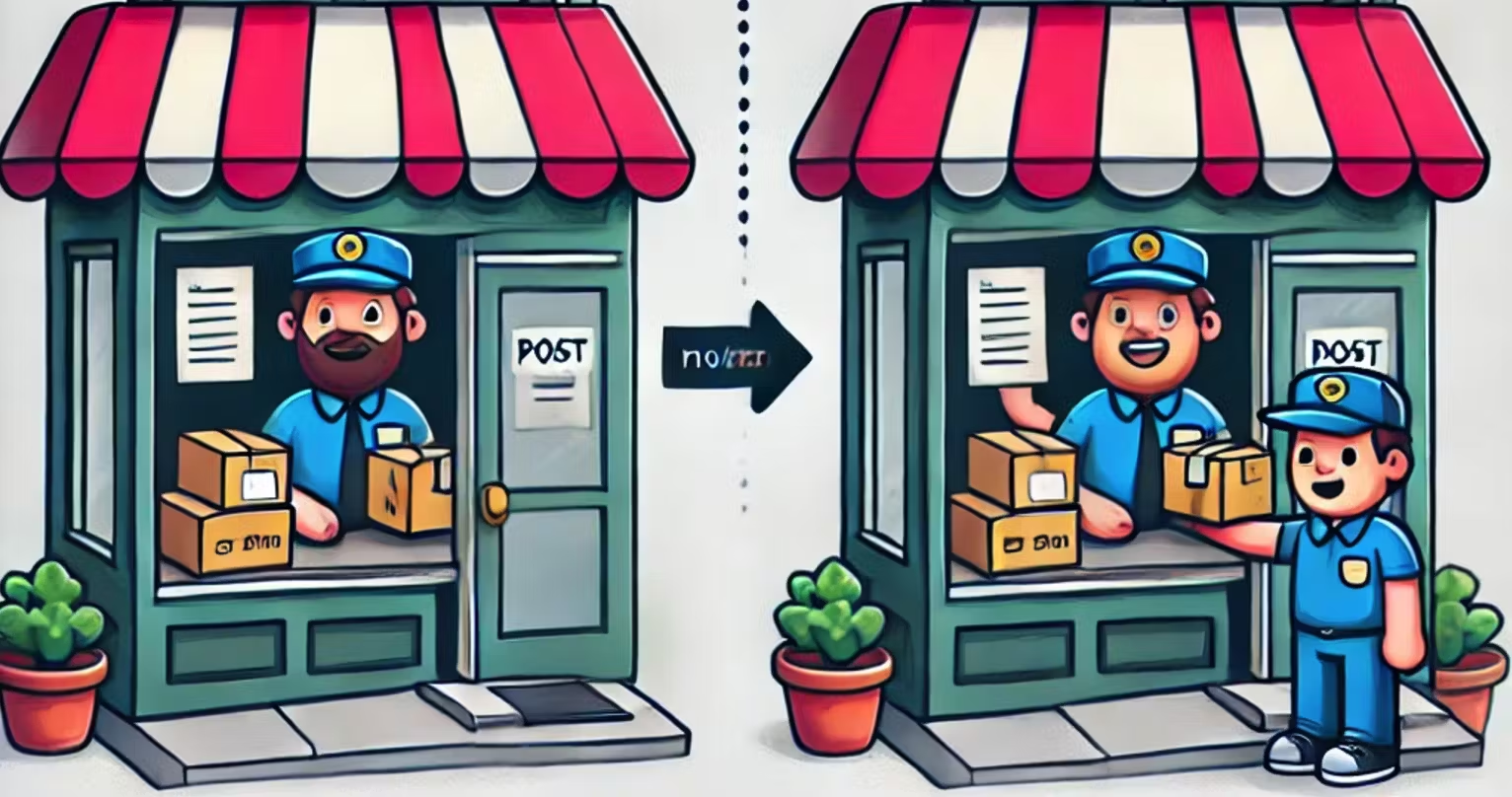
When you're learning Python, stumbling upon the line if __name__ == '__main__':
might feel like deciphering a secret code. But trust me, it’s simpler than it seems! This line serves as a gatekeeper, determining whether your Python script should execute everything or just selected parts based on how it’s being used. Let’s break it down using an easy analogy and some enhancements to make it crystal clear.
The Post Office Analogy
Imagine your script is like a post office. This post office has two main responsibilities:
Serving Customers Directly: A customer walks in and hands over a letter. The post office handles everything—accepting, processing, and delivering the letter.
Assisting Another Office: Sometimes, another post office might ask for help delivering a batch of letters. In this case, you only deliver the letters and skip the tasks meant for direct customers.
In Python terms:
When your script is run directly, it acts like the post office serving customers, doing everything from start to finish.
When your script is imported as a module, it acts like a helper post office—only performing the tasks requested by the other script.
What Does if __name__ == '__main__':
Do?
In every Python script, there’s a special variable called __name__
. Here’s how it behaves:
When the script is run directly,
__name__
is set to'__main__'
.When the script is imported,
__name__
is set to the name of the file (module).
The statement if __name__ == '__main__':
checks this variable. If it equals '__main__'
, the script knows it’s being run directly and executes the code inside that block. Otherwise, it skips it.
How It Works
File: post_office.py
pythonCopy codedef deliver_letter(address):
print(f"Delivering letter to {address}.")
if __name__ == '__main__':
print("Post Office is open for business!")
deliver_letter("123 Python Street")
Case 1: Running Directly
bashCopy code$ python post_office.py
Output:
kotlinCopy codePost Office is open for business!
Delivering letter to 123 Python Street
Here, the script recognizes it’s running directly and executes both the print
statement and the deliver_letter
function.
Case 2: Importing
pythonCopy codeimport post_office
post_office.deliver_letter("456 Code Avenue")
Output:
cssCopy codeDelivering letter to 456 Code Avenue
The script skips the if __name__ == '__main__':
block because it’s being used as a helper module.
Why Is This Useful?
Dual Functionality: Scripts can act as both standalone programs and reusable modules.
Testing Without Interference: You can add test code or examples inside the
if __name__ == '__main__':
block, ensuring they only run during direct execution.
Post Office Example
Direct Execution: A customer enters the post office, and all tasks are performed.
Imported Module: The post office receives a request from another office to handle a specific delivery, skipping tasks meant for direct customers.
Another Analogy: The Swiss Army Knife
Think of your script as a Swiss Army knife.
If someone runs the script directly, it opens all tools (blade, screwdriver, scissors) and performs every task.
If someone imports the script, they might only use the screwdriver or blade, leaving the other tools folded away.
References
For further reading, check out these links:
TL;DR
if __name__ == '__main__':
ensures your script knows when to run everything (direct execution) and when to remain silent (imported as a module). It’s like a gatekeeper that decides whether to handle everything itself or just assist another script.
Call to Action
Now that you understand this, try adding if __name__ == '__main__':
to one of your scripts and observe its behavior when run directly vs. imported. If you have questions or examples to share, feel free to drop them in the comments!
Let’s make Python scripting fun and efficient together! 😊
Subscribe to my newsletter
Read articles from Akshay Siwal directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
