[React.js] Uncaught TypeError: Cannot read properties of undefined (reading 'map')
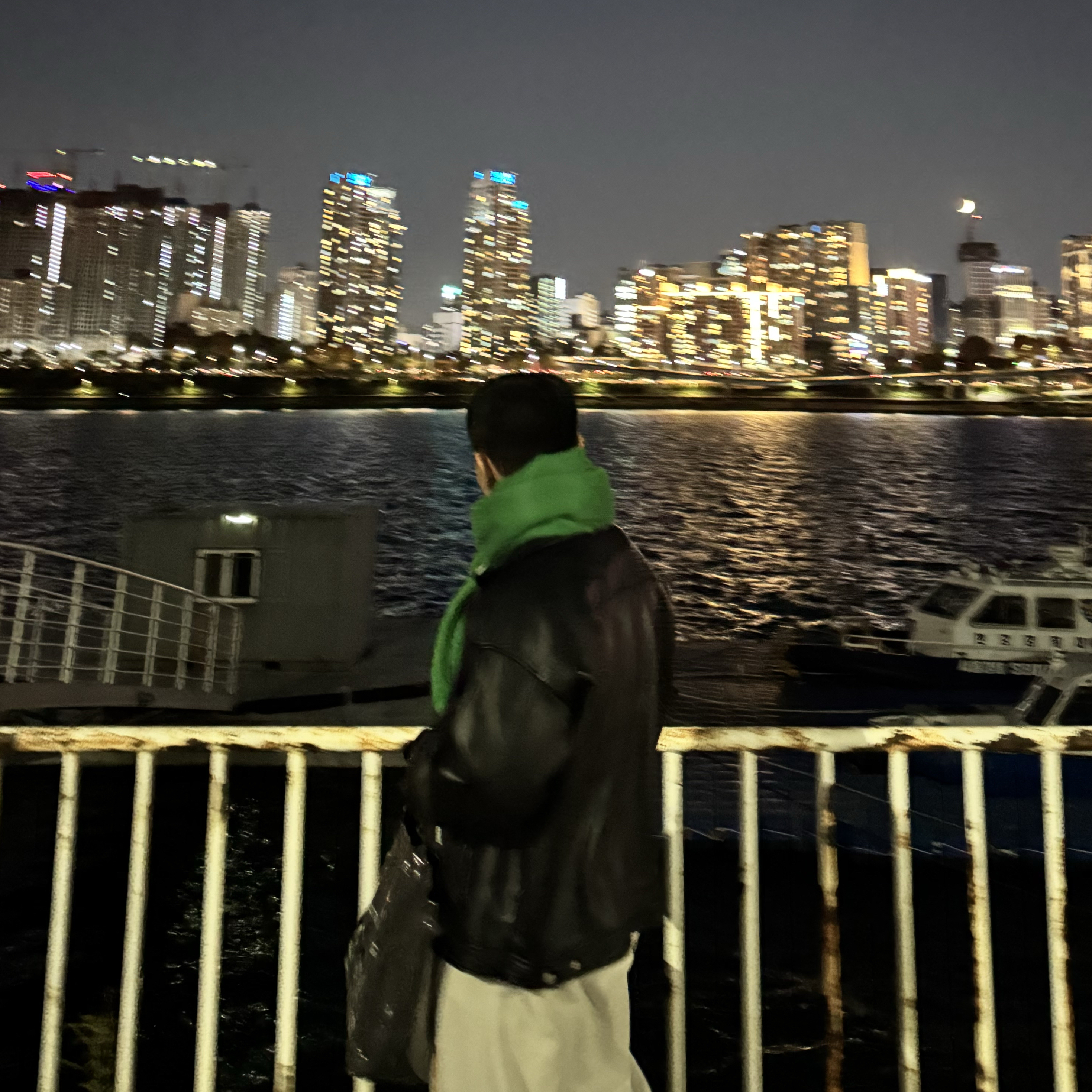
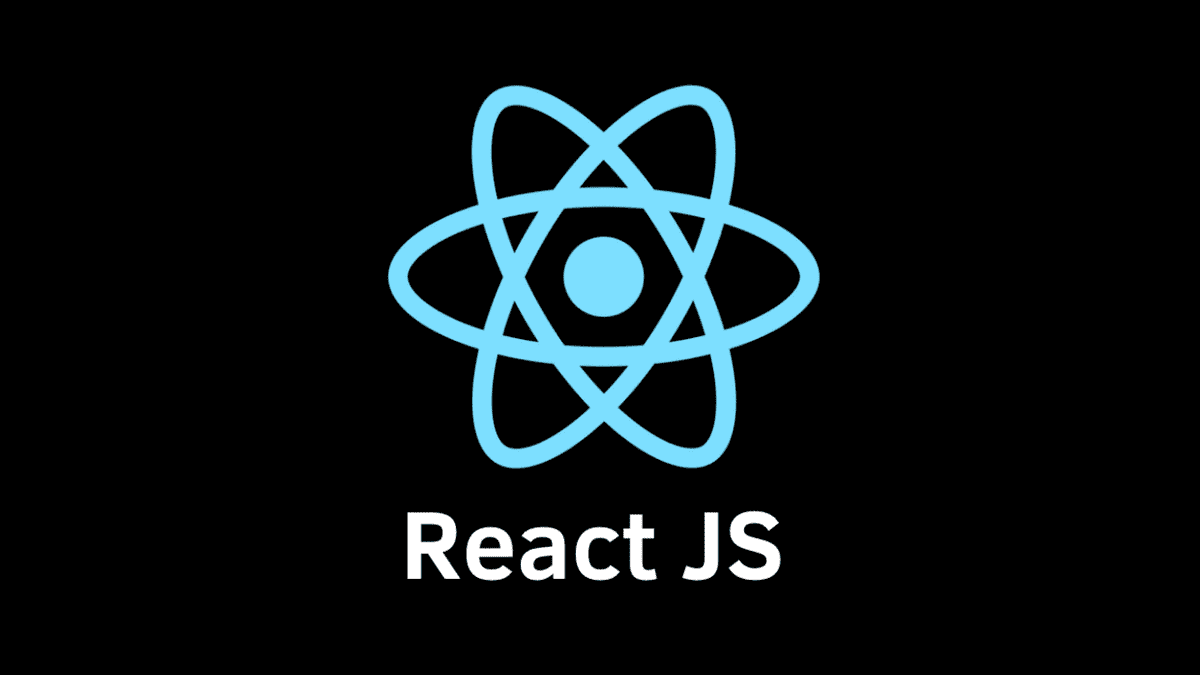
About Error
When I execute this react code,
<div id={id} className="row__posters">
{movies.length > 0 ? (
movies.map((movie) => {
return (
<SwiperSlide>
<img
key={movie.id}
style={{ padding: "25px 0" }}
className={`row__poster ${
isLargeRow && "row__posterLarge"
}`}
src={`https://image.tmdb.org/t/p/original/${
isLargeRow ? movie.poster_path : movie.backdrop_path
}`}
alt={movie.name || movie.title}
onClick={() => handleClick(movie)}
/>
</SwiperSlide>
);
})
) : (
<p>No movies available.</p>
)}
</div>
It results in TypeError.
Why?
It is because react.js is going to map through the movies
when movies
do not have any elements. In other words, movies
is currently undefined.
Solution
Initializing
movies
is undefined because the array might not be initialized. You can initialize it by using useState in react.
const [movies, setMovies] = useState([]); // initialize movies to []
Optional chaining (
?.
)if movies is nullish (meaning
null
orundefined
), the expression short circuits and evaluates toundefined
instead of throwing an error.movies?.map((movie) => { // your code }
Logical AND (&&)
Logical AND (
&&
) works similar to optional chaining (?.
). Before mapping, we can check if movies isundefined
by using&&
operator.
movies && movies.map((movie) => {
// your code
}
Subscribe to my newsletter
Read articles from Lim Woojae directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
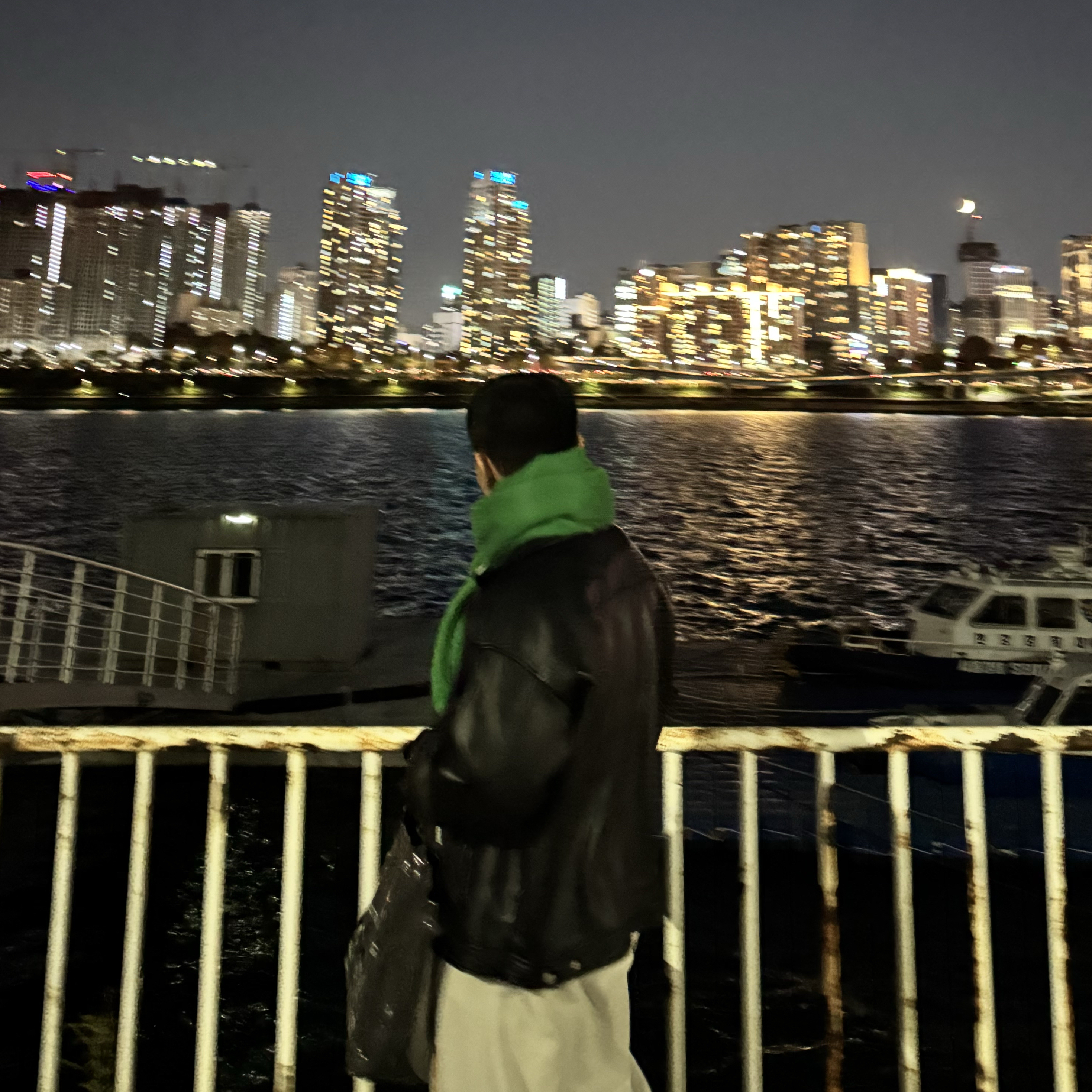
Lim Woojae
Lim Woojae
Computer Science Enthusiast with a Drive for Excellence | Data Science | Web Development | Passionate About Tech & Innovation