A Beginner's Guide to Docker: From Writing a Dockerfile to Pushing Your Image to Docker Hub

Table of contents
- 1. Install Docker
- 2. Create a Dockerfile
- Explanation of the Dockerfile Instructions:
- 3. Build the Docker Image
- 4. Verify the Image Build
- 5. Run the Docker Container
- 6. Tag the Image for Docker Hub
- 7. Login to Docker Hub
- 8. Push the Image to Docker Hub
- 9. Verify the Image on Docker Hub
- 10. Pull the Image from Docker Hub (Optional)
- Summary of Commands
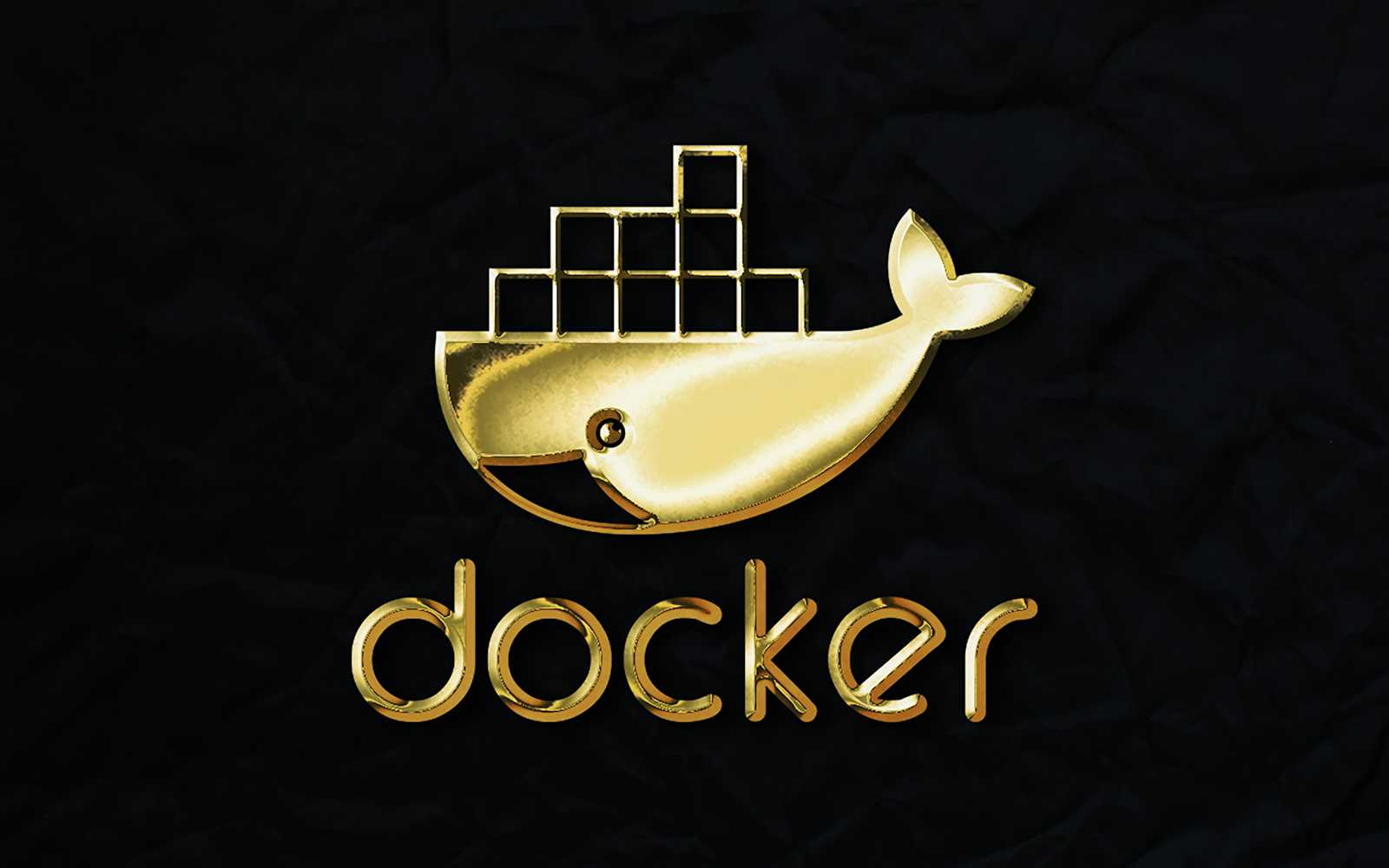
Getting started with Docker involves a series of steps, from writing a Dockerfile to building and pushing Docker images to Docker Hub. Below is a step-by-step guide on how to achieve this, including Docker commands and snippets:
1. Install Docker
First, ensure Docker is installed on your machine. You can install Docker by following the instructions for your operating system from the official Docker website.
You can verify your installation by running:
docker --version
2. Create a Dockerfile
A Dockerfile
is a script containing a series of instructions to create a Docker image. It defines the base image, application dependencies, configuration, and commands to run.
Dockerfile Example:
Here’s an example of a simple Dockerfile
for a Node.js application:
# Use an official Node.js runtime as a parent image
FROM node:16-alpine
# Set the working directory in the container
WORKDIR /app
# Copy package.json and package-lock.json (if exists)
COPY package*.json ./
# Install the application dependencies
RUN npm install
# Copy the rest of the application code
COPY . .
# Expose port the app runs on
EXPOSE 3000
# Command to run the app
CMD ["node", "index.js"]
Explanation of the Dockerfile Instructions:
FROM node:16-alpine
: This sets the base image. In this case, it's a minimal Node.js image with Alpine Linux.WORKDIR /app
: This sets/app
as the working directory in the container.COPY package*.json ./
: This copies thepackage.json
andpackage-lock.json
files into the working directory.RUN npm install
: This installs the app’s dependencies defined inpackage.json
.COPY . .
: This copies the rest of the application code into the container.EXPOSE 3000
: This exposes port 3000, which is the port the app will run on.CMD ["node", "index.js"]
: This sets the command that runs when the container starts.
3. Build the Docker Image
Once you have your Dockerfile ready, navigate to the directory containing your Dockerfile
and build the Docker image using the docker build
command.
docker build -t my-node-app .
-t my-node-app
: This tags the image with the namemy-node-app
. You can replace it with any name you like..
: The dot represents the current directory, which contains the Dockerfile and application code.
4. Verify the Image Build
After the build completes, you can verify that your image was created successfully by listing all Docker images:
docker images
You should see something like this:
REPOSITORY TAG IMAGE ID CREATED SIZE
my-node-app latest a1b2c3d4e5f6 1 minute ago 200MB
5. Run the Docker Container
To test your Docker image locally, you can run it using the docker run
command:
docker run -p 3000:3000 my-node-app
This command runs the image and maps port 3000 of the container to port 3000 of your host machine, so you can access the app at http://localhost:3000
.
6. Tag the Image for Docker Hub
Before pushing the image to Docker Hub, you need to tag it with your Docker Hub username and repository name.
docker tag my-node-app your-dockerhub-username/my-node-app:v1
your-dockerhub-username
: Replace this with your Docker Hub username.v1
: The version or tag you want to give your image (optional).
7. Login to Docker Hub
To push an image to Docker Hub, you need to log in first. If you don’t have an account on Docker Hub, you can create one at hub.docker.com.
docker login
You’ll be prompted to enter your Docker Hub username and password.
8. Push the Image to Docker Hub
After logging in, you can push the tagged image to Docker Hub using the docker push
command:
docker push your-dockerhub-username/my-node-app:v1
This will upload your image to Docker Hub under the your-dockerhub-username/my-node-app
repository with the tag v1
.
9. Verify the Image on Docker Hub
Once the push is complete, you can visit your Docker Hub repository to verify that the image is available. You should see it listed with the correct tag.
10. Pull the Image from Docker Hub (Optional)
If you want to test or share your image, you can pull it from Docker Hub:
docker pull your-dockerhub-username/my-node-app:v1
This will download the image to your local machine.
Summary of Commands
Here's a summary of the key Docker commands used in the process:
Build the Docker image:
docker build -t my-node-app .
Run the Docker container:
docker run -p 3000:3000 my-node-app
Tag the image for Docker Hub:
docker tag my-node-app your-dockerhub-username/my-node-app:v1
Login to Docker Hub:
docker login
Push the image to Docker Hub:
docker push your-dockerhub-username/my-node-app:v1
Pull the image from Docker Hub (optional):
docker pull your-dockerhub-username/my-node-app:v1
This is a basic introduction to Docker, from writing a Dockerfile
to pushing the image to Docker Hub. You can expand this process to include more advanced features like multi-stage builds, Docker Compose for multi-container applications, and more.
Thank you.
- Prashant
Subscribe to my newsletter
Read articles from Prashant Sharma directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Prashant Sharma
Prashant Sharma
Passionate about Automating tasks, From code to deployment, I believe in making things smoother, faster, and more reliable. On a mission to eliminate manual work and bring the power of automation to every part of the tech stack. Let’s automate the world, one script at a time! 🔧🌐