Unlocking the Power of React Hooks: A Friendly Guide
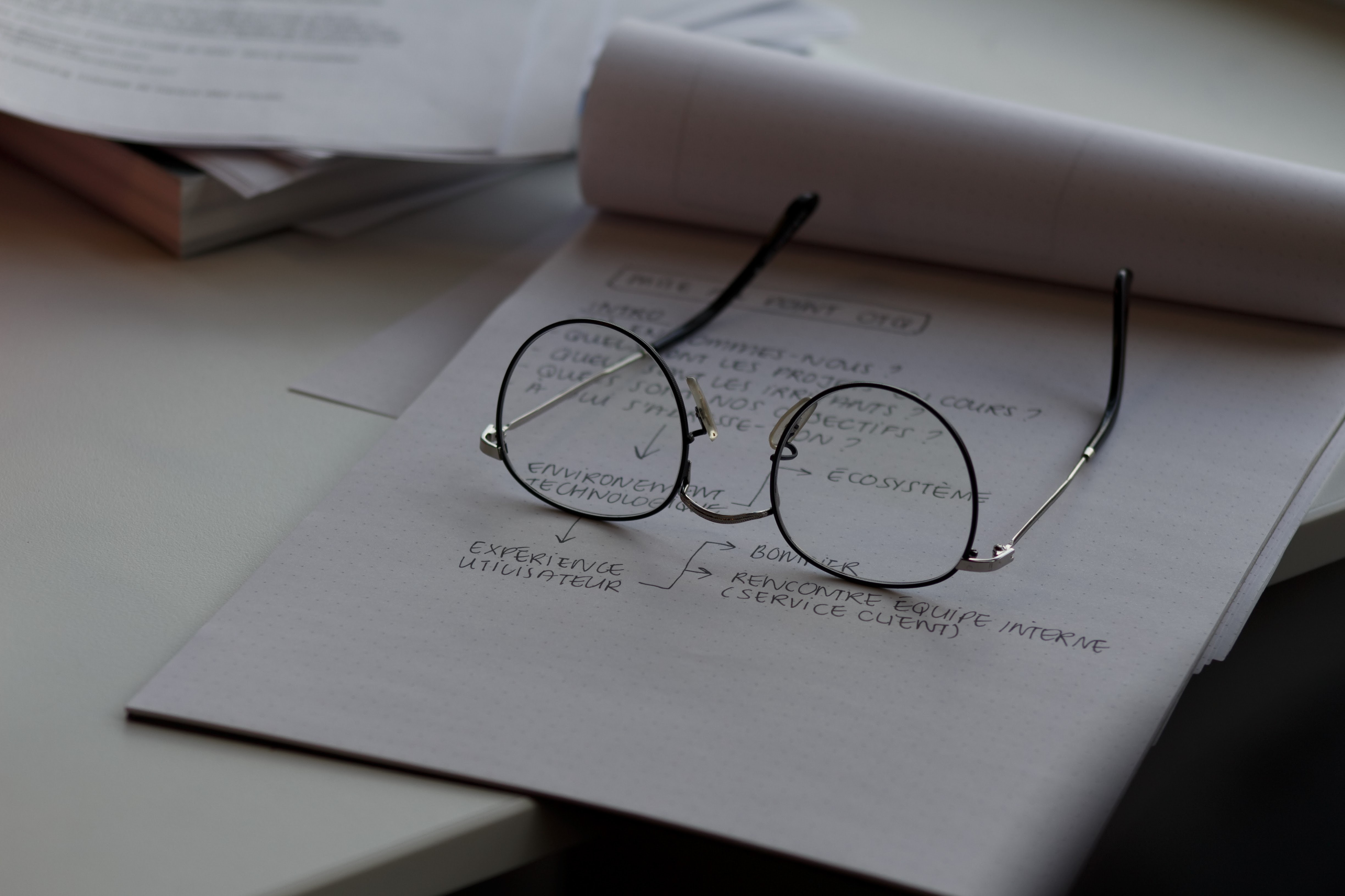
Hey there, fellow developers! If you’ve been diving into React lately, you’ve likely stumbled upon the magical term “Hooks.” They’ve really changed the game for how we build components and manage state, making our coding journey a lot smoother (and more enjoyable!). Let’s chat about what Hooks are and why they’re worth your time.
What Are Hooks, Anyway?
At their essence, React Hooks are special functions that allow you to tap into React’s features, like state and lifecycle methods, without having to write a class. Think of them as nifty little tools that help you write cleaner, more maintainable code. Plus, they keep your component logic organized and reusable, which is a win-win!
Why Use Hooks?
You might be asking, “Why should I care about Hooks?” Here are a few friendly reasons:
Simplicity: Hooks enable you to use state and other React features in functional components. If you’re not a fan of class components, this is great news!
Reusability: You can create custom Hooks to extract and reuse logic, helping you avoid repetitive code.
Better Organization: Grouping related logic in a single function means you can keep your code tidy instead of scattering it across various lifecycle methods.
Getting Started with the Basics
Let’s explore some of the most commonly used Hooks: useState
and useEffect
.
useState
Imagine you’re building a simple counter app. Here’s how to manage state using useState
:
import React, { useState } from 'react';
const Counter = () => {
const [count, setCount] = useState(0);
return (
<div>
<h1>{count}</h1>
<button onClick={() => setCount(count + 1)}>Increment</button>
</div>
);
};
In this snippet, useState
initializes the count to 0, and setCount
updates it every time the button is clicked. Easy peasy, right?
useEffect
Now, let’s spice things up with useEffect
. This Hook lets you handle side effects in your components, like fetching data or subscribing to events.
Here’s an example that fetches data from an API when the component mounts:
import React, { useState, useEffect } from 'react';
const DataFetcher = () => {
const [data, setData] = useState([]);
useEffect(() => {
const fetchData = async () => {
const response = await fetch('<https://api.example.com/data>');
const result = await response.json();
setData(result);
};
fetchData();
}, []); // Runs once when the component mounts
return (
<ul>
{data.map(item => (
<li key={item.id}>{item.name}</li>
))}
</ul>
);
};
In this case, useEffect
does the heavy lifting by fetching data when the component first renders. Since we passed an empty array as the second argument, it runs only once. Neat, huh?
Custom Hooks: Your New Best Friend
Once you’re comfortable with useState
and useEffect
, you might want to create your own custom Hooks. This is where the real fun begins!
Here’s a simple custom Hook for managing form inputs:
const useFormInput = initialValue => {
const [value, setValue] = useState(initialValue);
const handleChange = e => {
setValue(e.target.value);
};
return { value, onChange: handleChange };
};
// Usage in a component
const MyForm = () => {
const name = useFormInput('');
return (
<input type="text" {...name} placeholder="Enter your name" />
);
};
This custom Hook makes managing form inputs a breeze, eliminating repetitive code across components.
Wrapping Up
React Hooks are a fantastic way to write more intuitive and maintainable components. They help you keep your code organized so you can focus on what really matters—building amazing user experiences!
Next time you’re working on a React project, give Hooks a try. They just might become your new favorite tool!
I’ll be back soon with a deeper dive into useCallback
and useMemo
—two more powerful hooks that can help you optimize performance in your React apps. Stay tuned!
I’d love to hear about your experiences with Hooks in the comments below. Happy coding!
Subscribe to my newsletter
Read articles from Nidhin M directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
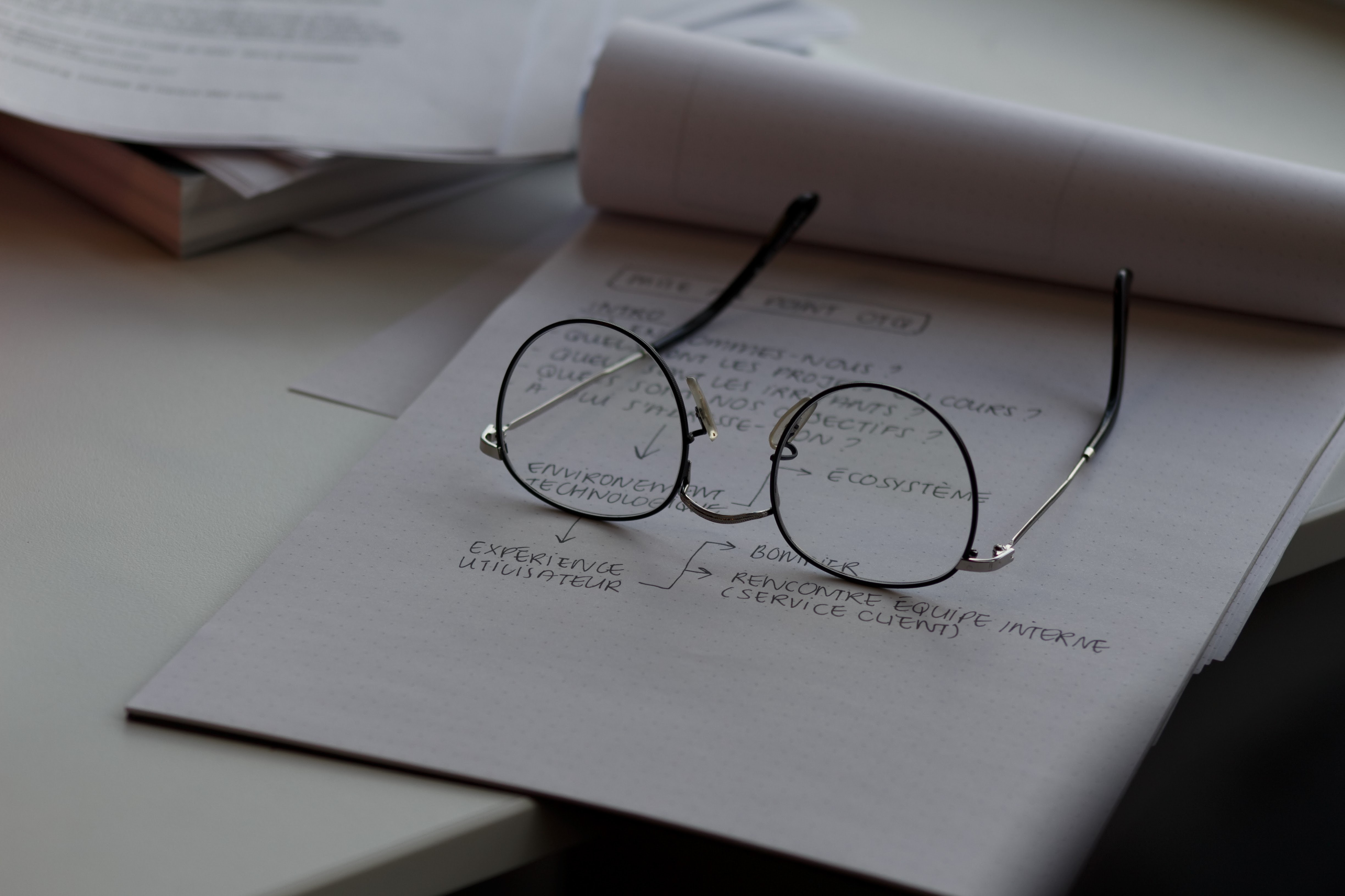
Nidhin M
Nidhin M
Hey there! I'm a passionate software developer who thrives in the TypeScript world and its companions. I enjoy creating tutorials, sharing tech tips, and finding ways to boost performance. Let's explore coding and tech together! 🚀