Blockchain Data Structures in Python: Creation and Management Tips
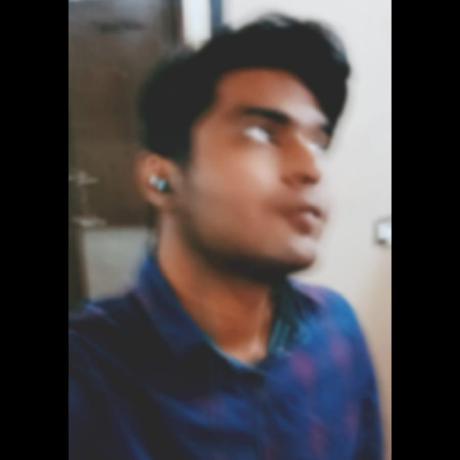
With the rise in blockchain technology, different fields of studies are experimenting vigorously to see how blockchain fits into their own environment. The wait is over!!
This python library allows creating secure blockchains with system level speed with configurable proof-of-work factors. The modstore
library comes in handy while creating and testing blockchain right on your fingertips. It could be for your semester projects or your life’s work.
The modstore
library offers creating a blockchain data structure from scratch that can store any kind of data into its data blocks. Leveraging a mix of Python and Rust, it is available for most operating systems.
Let’s not delay one second more and start by installing modstore.
pip install modstore
Open up your terminal or CMD/Powershell (on Windows) and copy paste the above snippet into it and hit Enter. This will install the modstore
library into your python environment.
Now let us begin by importing the BlockChain
class.
from modstore.rust import BlockChain
Once we have imported the BlockChain
class, we can then create a blockchain object.
blockchain = BlockChain(
difficulty= # your difficulty needs (int),
timezone = # TimeZone
)
In the above definition, BlockChain
class takes two parameters:
difficulty
- The difficulty specifies how many leading zeros are required for a block's hash to be considered valid. A higher difficulty makes it more computationally expensive to find a valid hash, thereby regulating the time between block generations and maintaining the security of the blockchain. During the mining process (`mine` method (Internally)), the block repeatedly computes its hash until the first difficulty
characters of the hash match the required pattern (i.e., a string of 0
s). This process involves trial and error, and the number of iterations needed depends on the difficulty level.
The more the difficulty factor of a blockchain, the more computationally hard it will be tamper with the blockchain. You should also consider your current hardware before setting it too high. I would suggest checking the time it takes to add one block with your desired difficulty before moving on with your project.
timezone
- This parameter sets the timezone of the timestamp to be used in each block. This parameter takes literals ['UTC', 'IST'] or "HH:MM:SS"
to be added to UTC to get the desired timezone.
Now that we have initiated our blockchain, we can add blocks into it. Note that when we initiated the blockchain, a starting block called the Genesis Block has been created and all newer blocks will connect in a chain from this block onwards.
# To add a block use the addBlock Method
blockchain.addBlock(
uniqueIdentifier="block-1",
# a unique identifier that will identify
# this block and this block only.
data="Some data",
# The data can be any string
# or any bytes
# or any object from python.
)
The above code will create a block and mine it (update nonce and calculate hash until there are defined number of leading 0s in the hash) and finally add it into the blockchain.
To see the number of blocks in the blockchain,
number_of_blocks = blockchain.length
To check if the blockchain is valid and there is no tamper,
validity: bool = blockchain.valid
To get the identifiers of all the blocks as a list,
list_of_identifiers = blockchain.identifiers
To get a block from the the blockchain,
# The following code returns a
# <class 'modstore.rust.blockchain.Block'> type object
from modstore.rust import Block
block: Block = blockchain.search(identifier='block-1')
The above variable named block
is a Block
type variable. The Block
class is an internal class and should not be initialised. However, when we search a block in the blockchain, we would get a Block
type value. We can get a lot of information from it.
## the block varibale ##
# block identifier
print(block.identifier)
# block index (position)
print(block.index)
# block timestamp
print(block.timestamp)
# block previous block's hash
print(block.previous_hash)
# block hash
print(block.hash)
# IMPORTANT
# Getting the data from the block
data = block.data()
# If the original data was <class 'str'> or <class 'bytes'>,
# the above line will work and no change is needed.
# However, if you added some other type of data (can be any object),
# like list, dict, tuple, functions or classes or anything that can be
# called an object in python.
# then you need to modify the above line a bit.
data = block.data(return_original=True)
With this, the tutorial ends. You can experiment with it as you like, for experimental purposes you can checkout the modstore._binaries
module which provides direct access to the rust binaries using python.
To print a blockchain, simply use print(blockchain)
. Happy Learning!! :)
Subscribe to my newsletter
Read articles from Soumyo Deep Gupta directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
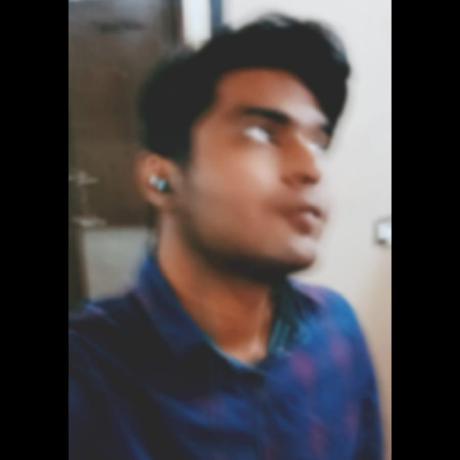