Compilation in Java: JIT vs AOT
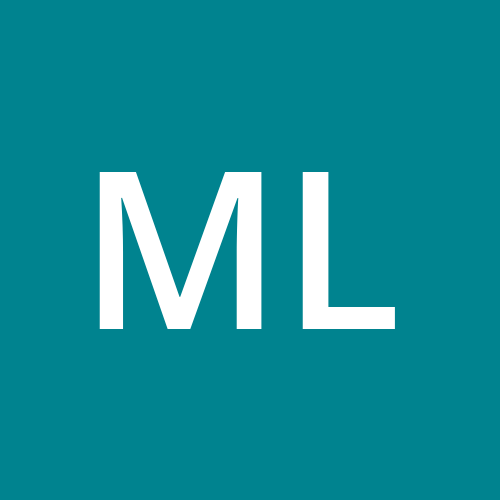
Table of contents
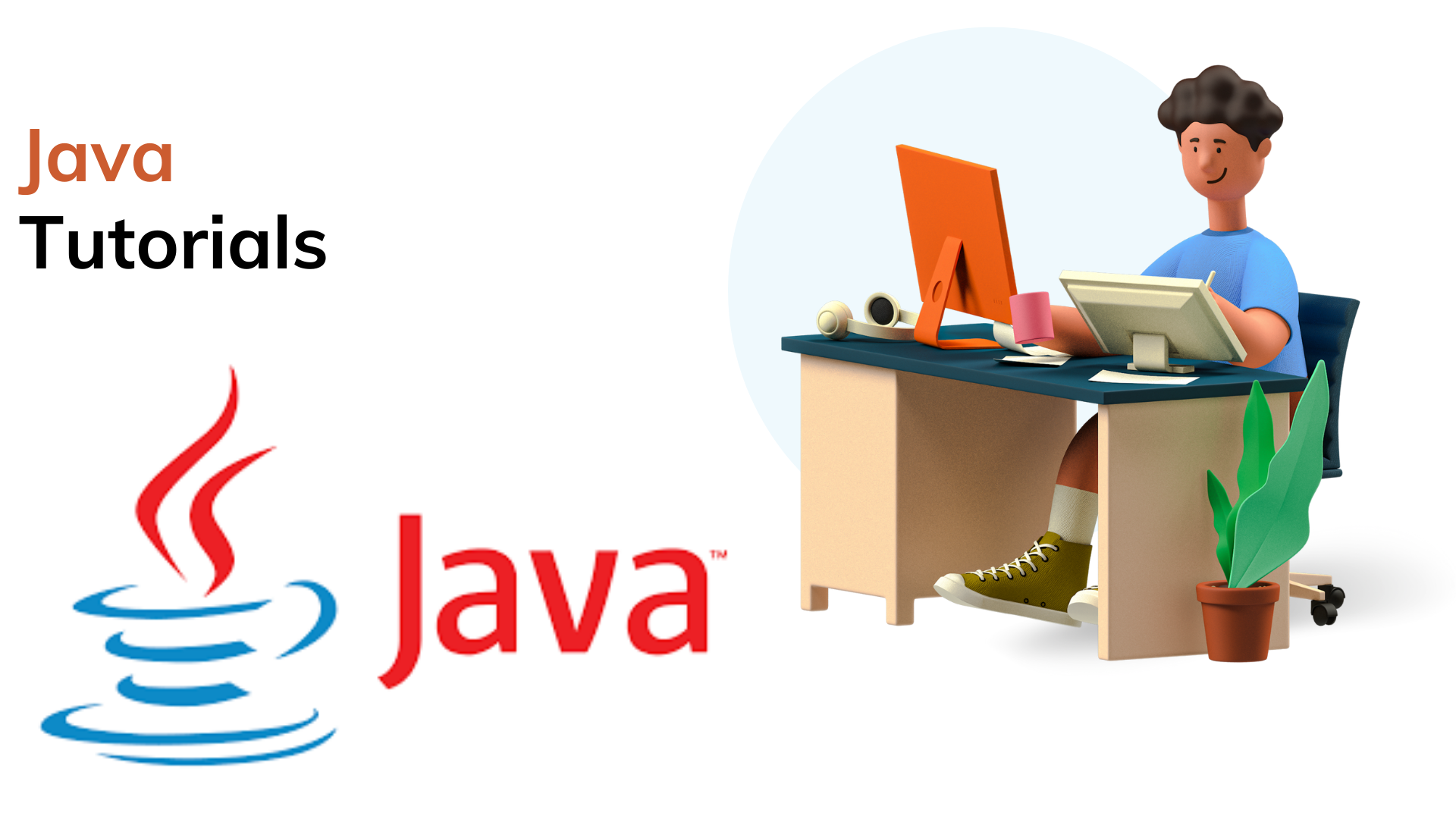
Java provides a unique balance of portability and performance through its use of bytecode and the Java Virtual Machine (JVM). When it comes to compiling this bytecode, two primary approaches optimize Java's performance: Just-In-Time (JIT) compilation and Ahead-Of-Time (AOT) compilation. This article explores these two compilation strategies, examining how each works, their advantages, trade-offs, and use cases.
1. Introduction to Java Compilation
Java programs are typically written in .java
files, which are compiled into bytecode by the Java compiler (javac
). This bytecode is platform-independent and runs on the JVM, making Java highly portable. However, bytecode itself needs to be further optimized to achieve native-level performance, which is where JIT and AOT come into play. These strategies differ in when and how they optimize the bytecode for execution.
2. Understanding JIT (Just-In-Time) Compilation
Just-In-Time (JIT) compilation is the process where bytecode is compiled into native machine code at runtime, just before it’s executed. The JIT compiler resides within the JVM and compiles frequently used portions of the code, improving execution speed over time as the application runs.
How JIT Works:
Interpretation: The JVM starts by interpreting bytecode, line by line.
Profiling: As the JVM interprets code, it profiles the application to identify "hot spots"—sections of code that are frequently executed.
Compilation: When hot spots are identified, the JIT compiler compiles these sections into native machine code, which can be executed faster.
Optimization: JIT performs various optimizations, like inlining, dead-code elimination, and loop unrolling, to enhance performance.
3. Advantages and Limitations of JIT Compilation
Advantages:
Dynamic Optimization: JIT continuously profiles the application and re-optimizes code based on runtime behavior, providing adaptive performance enhancements.
Lower Memory Usage at Startup: Since code is only compiled when needed, JIT compilation can lead to faster startup times and reduced initial memory footprint.
Platform Independence: Because JIT compilation happens at runtime on the JVM, Java applications remain platform-independent.
Limitations:
Warm-Up Time: JIT compilation can introduce a "warm-up" period where the application performs slower initially until hot spots are identified and compiled.
Higher Memory Consumption Over Time: As more code is compiled at runtime, memory usage may increase, especially in long-running applications.
Potential Latency: JIT compilation may add latency during runtime as it pauses the execution to compile hot spots.
4. Understanding AOT (Ahead-Of-Time) Compilation
Ahead-Of-Time (AOT) compilation involves compiling bytecode into native machine code before the application is run. Java introduced AOT compilation support starting in Java 9, allowing bytecode to be compiled at build time, creating a pre-optimized, platform-specific binary.
How AOT Works:
Compile at Build Time: Bytecode is compiled into native code ahead of time, eliminating the need for runtime compilation.
Single Native Image: AOT generates a native executable image, which includes the Java application and necessary dependencies, and can be directly executed on the target system.
Pre-Built Optimizations: Unlike JIT, AOT does not have runtime profiling, so optimizations are fixed at build time.
5. Advantages and Limitations of AOT Compilation
Advantages:
Fast Startup Time: AOT-compiled applications start almost immediately since the bytecode has already been compiled into native code.
Lower Runtime Overhead: With no need for runtime profiling or compilation, AOT applications run without the potential latency of JIT compilation.
Reduced Memory Usage Over Time: Since all code is compiled before execution, AOT may result in lower memory consumption, especially for long-running applications.
Limitations:
Platform Dependency: AOT compilation produces a platform-specific binary, reducing Java's portability.
Lack of Runtime Optimizations: AOT lacks the adaptive optimizations provided by JIT since the code is fixed at build time.
Larger File Size: Native executables generated through AOT tend to be larger in size due to precompiled code and dependencies.
6. JIT vs. AOT: Key Differences
Feature | JIT Compilation | AOT Compilation |
Compilation Time | Runtime | Build time |
Performance Adaptation | Dynamic runtime optimization | Fixed optimizations at build time |
Startup Time | Slower initial startup | Fast startup |
Memory Usage | Higher in long runs | Lower over time |
Platform Independence | Platform-independent | Platform-dependent |
File Size | Smaller application footprint | Larger due to precompiled code |
7. When to Use JIT or AOT
Use JIT Compilation When:
You need Java’s platform independence for cross-platform applications.
The application is long-running and benefits from dynamic optimizations over time.
Startup latency is acceptable, and the focus is on peak performance after initial startup.
Use AOT Compilation When:
Fast startup time is critical, such as in serverless applications or microservices.
The application is short-lived, such as in command-line tools, where peak performance is needed immediately.
You can target a specific platform and do not require platform independence.
8. Conclusion
Both JIT and AOT compilation strategies provide unique benefits to Java developers. While JIT continues to be the default for Java applications due to its adaptive optimizations and platform independence, AOT offers significant advantages in startup speed and reduced runtime overhead, especially for targeted platforms.
Java developers now have the flexibility to choose the compilation approach that best suits their application's requirements. With the rise of cloud-native applications and microservices, understanding the strengths of JIT and AOT is essential to building efficient, responsive, and high-performing Java applications.
More such articles:
https://www.youtube.com/@maheshwarligade
Subscribe to my newsletter
Read articles from Maheshwar Ligade directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
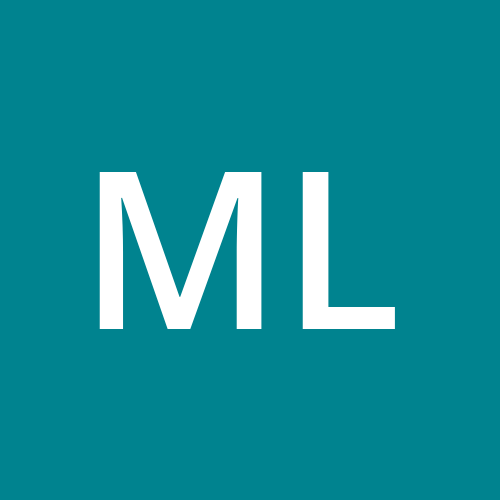
Maheshwar Ligade
Maheshwar Ligade
Learner, Love to make things simple, Full Stack Developer, StackOverflower, Passionate about using machine learning, deep learning and AI