Week 2 updates

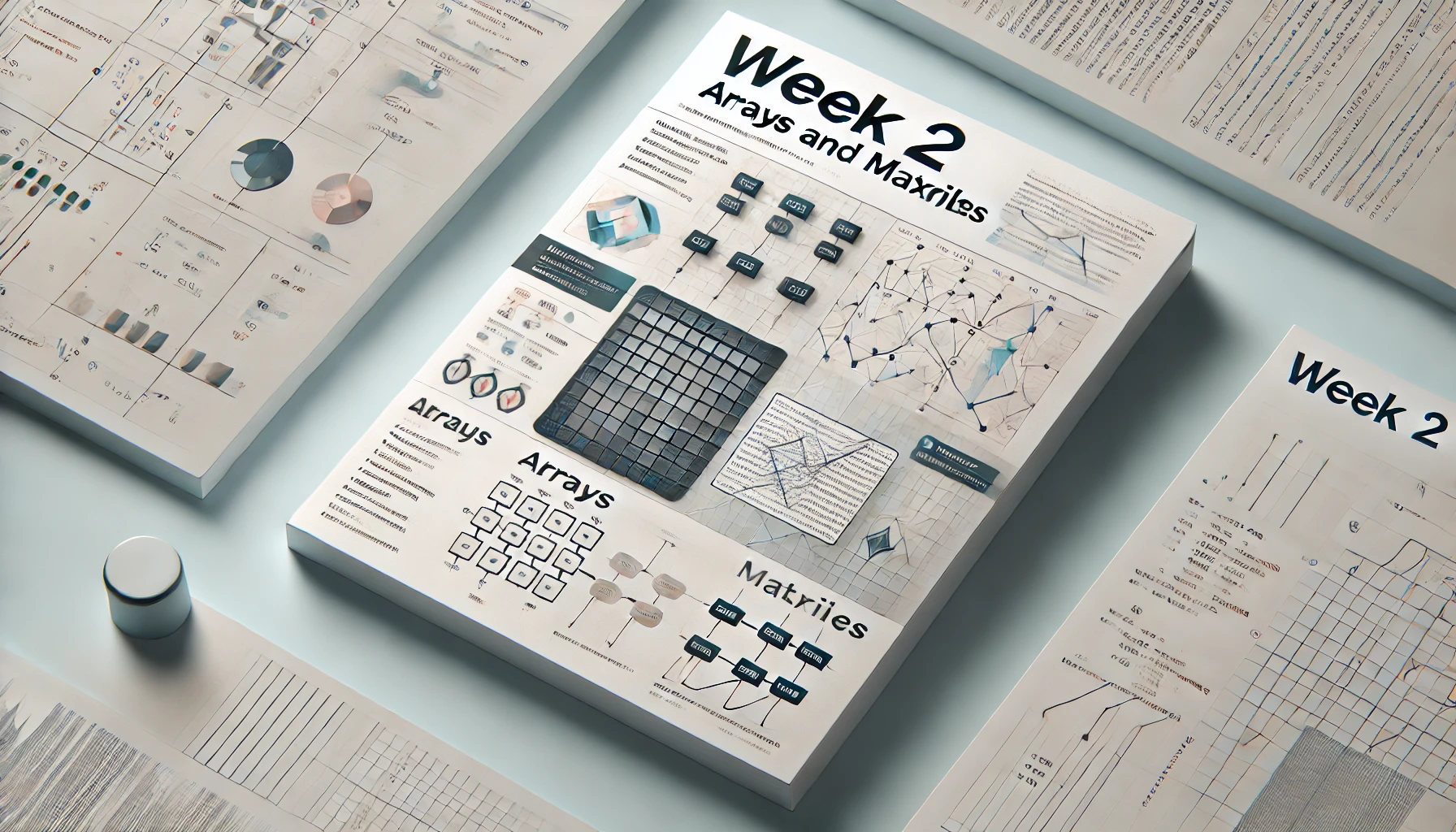
"Life is full of twists and turns; it's how we navigate them that defines our journey."
After a successful Week 1, I was all geared up to replicate the same momentum in Week 2. Much like a car that takes a little extra time to start in winter, beginning something new in life requires warming up and persistence.
My plan was ambitious—covering arrays, matrices, and recursion. But as we know, life rarely unfolds exactly as planned. Unexpected projects landed on my desk, demanding significant attention and time. While this could have easily been an excuse to let my commitment slide, I chose to adapt instead.
I focused on what I could do within the time I had, diving deep into arrays and matrices. Though I couldn't complete recursion this week, I’m proud of the effort I put in despite the constraints.
This week was a reminder that progress isn’t always linear, and that’s okay. I know many of you might face similar roadblocks, and it's important to remember that growth isn't always about perfection.
Sometimes, simply showing up and doing your best is an achievement in itself.
Tackling the Remaining Crucial Aspects of Arrays
Having completed a few key problems as stated in the last article, it was time to tackle the remaining crucial aspects of arrays that would further solidify my foundation and help me dive into more complex challenges.
Starting with one of the most asked questions, trapping rain water, I quickly realized that solving this was like trying to find the right umbrella for a downpour — not as easy as it looks! This problem introduced me to the idea of using two auxiliary arrays to store the left maximum and right maximum values for each element in the array. For the first and last blocks, no water is stored, so we focus on the blocks in between. After implementing the auxiliary arrays, the solution became clear: we calculate the minimum of the left and right maximums, and then subtract the element value to determine the amount of water stored.
The next question I encountered was subarray with a given sum. This problem led me to recall the sliding window technique, which was the perfect fit for solving it efficiently. By applying this logic, the entire problem became much simpler, and I was able to solve it with ease.
The next problem I tackled was about finding the equilibrium point. (I’m still trying to find the perfect equilibrium in my life as well! 😅) This problem required initializing two variables: left sum and right sum. The left sum started at 0, and the right sum was initialized to the total sum of the array. As we traversed the array, we subtract the current element from the right sum and check if both sums are equal. If they are, we return the element at that point. Else, we increment the left sum by the current element and repeat the same.
Another problem that introduced me to a new concept was prefix sum. In this problem, we first need to preprocess a prefix sum array, where each element at index i
holds the sum of all elements from the start of the array up to index i
. Once we have this, if we’re given two indices, say i
and j
, to find the sum of elements between them, we simply subtract the value at prefix[i-1]
from prefix[j]
. This gives the sum of elements from index i
to j
, allowing for an efficient solution to range sum queries.
Into the Matrix World: Exploring the Power of Multi-Dimensional Arrays
Matrices in Python are essentially 2-dimensional arrays, which added a new layer of excitement for me. While I was already comfortable with 1D arrays, visualizing the rows and columns in a matrix stepped up the complexity — not more complex than my sleep cycle though! 😅 But that challenge made the learning process more engaging. Tackling problems that required traversing, manipulating, and calculating with matrices kept the process both fun and rewarding!
The first twist that matrices threw at me was when I tried to create and manipulate one. I thought I had everything figured out, so I used
mat = [[0] * cols] * rows,
mat[0][0]=1
print(mat)
But, something unexpected happened! When I printed the output of mat
, it didn’t look anything like what I expected. It was a good reminder that the way Python handles lists can sometimes be a bit tricky — definitely not as straightforward as it seemed!
[[1,0,0],[1,0,0],[1,0,0]]
The correct way to create a 2D matrix in Python is:
pythonCopy codemat = [[0] for j in range(cols)] for i in range(rows)]
This ensures that each row is a separate list, avoiding the issues that arise from shallow copying.
After solving a few easy problems like matrix in snake pattern, I explored various matrix traversals.
In the boundary traversal of a matrix, after covering the first row, the last column, and the last row in anticlockwise order, the final step is to traverse the first column from bottom to top. This allows you to complete the outer boundary traversal, ensuring all outer elements are covered without repeating any of the elements already traversed
This was a great foundation for spiral traversal. In spiral traversal, we keep track of four variables: top, bottom, left, and right. The loop runs until top <= bottom
and left <= right
. Here's the traversal process:
Traverse the top row from left to right.
Traverse the right column from top to bottom.
Traverse the bottom row from right to left.
Traverse the left column from bottom to top.
After each step, we update the boundaries by incrementing or decrementing the variables accordingly. This method is particularly useful when dealing with circular or layered matrix problems. It’s a great way to efficiently access the entire matrix in a spiral pattern!
I came across a problem of finding an element in a row-wise and column-wise sorted matrix. The approach was to start from the rightmost top element. If the element to be found is smaller than the current element, move left. If it's larger, move down. This technique allows for efficient search, as it takes advantage of the sorted properties of the matrix. By narrowing down the possible locations in this manner, we optimize the solution significantly, reducing the time complexity compared to brute force methods.
Transposing a matrix is a crucial operation, and in Python, it can be done in-place using the property that in a transpose, rows become columns and columns become rows. This allows for efficient manipulation of matrix data. You can achieve this by swapping the elements at mat[i][j]
and mat[j][i]
for all i < j
, which effectively switches the rows and columns without needing extra space for another matrix.
One of the most challenging problems I faced was to rotate a matrix anticlockwise by 90 degrees. However, it wasn't as difficult as I initially thought. The approach relied on the fact that we can transpose the matrix first. After transposing, we simply need to reverse the rows of the matrix, which gives us the desired result. This method effectively handles the rotation without needing to manipulate the matrix extensively, making it a clean and efficient solution.
Learning to work with matrices is incredibly useful as it’s like learning to play a musical instrument—at first, it seems complex, but once you get the hang of it, everything falls into place. Matrices are key to solving problems in fields like computer graphics, machine learning, data science and dynamic programming. And let’s be real: mastering them gives you that "I can do anything" feeling.
If you're wondering what I've been working on lately, here’s a project that’s been taking up much of my time and, in turn, reduced my focus on DSA: an AI-powered data retrieval and extraction tool. This tool allows users to input a CSV or Google Sheet, then provide a prompt for data scraping. Using SerpAPI, it fetches the required data, which is then processed with Groq AI to extract only the most relevant and meaningful information. The user can then choose to view this data directly or have it updated in their Google Sheet. This project combines data retrieval, AI, and automation to streamline processes, making it a valuable tool for anyone needing quick, accurate insights from web data. (Project link-https://github.com/AbhiD1678/Ai-Agent-Project)
Conclusion
As we wrap up this update, I want to remind you that progress is a journey, not a race. Whether you’re diving deep into DSA, working on a project, or balancing both, remember that every step counts. I'm excited to continue sharing my learning experiences with you, and I hope you find them just as valuable as I do. Keep challenging yourself, keep growing, and most importantly, keep moving forward. Thanks for being a part of this journey with me—stay tuned for more updates!
If you are curious about what problems have I solved then here’s a list for you:
Trapping Rain Water
Subarray with Given Sum
Equilibrium Point
Prefix Sum
Matrix Traversal (Boundary, Spiral)
Finding Element in Row Wise and Column Wise Sorted Matrix
Matrix Transposition
Rotating a Matrix by 90 Degrees (Anticlockwise)
Subscribe to my newsletter
Read articles from Abhishek Dubey directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
