The Start: 5 Days, 10+ Math Problems

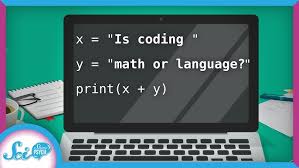
Over the last 5 days, I’ve focused on solving math-based coding problems. These problems not only improved my logical thinking but also helped me strengthen the basics of problem-solving. Here are a few things I’ve learned:
Breaking complex problems into smaller steps.
Understanding edge cases and writing efficient solutions.
Staying consistent and enjoying the process of problem-solving.
- Subtract the Product and Sum of Digits of a Number
def subtractProductAndSum(n: int) -> int:
# Your code goes here
sum=0
product=1
while n!=0:
a=n%10
sum +=a
product *=a
n=n//10
return product-sum
v=subtractProductAndSum(245)
print(v)
Here I used modulo for single digits, then I found the sum and product, and finally, I subtracted them.
Three Divisor
class Solution(object): def isThree(self, n): """ :type n: int :rtype: bool """ count=0 i=1 for i in range (1,n,i*i): if n%i==0: count +=2 if i*i==n: count -=1 if count ==3: return True else: return False s=Solution() r=s.isThree(4) print(r)
Here, I first found how many divisors a number has, then checked if that number has exactly 3 divisors.
By solving this problem, I learned a new way to find divisors. In the basic method, we loop up to the given number and check which numbers divide it, its takes O(n) time. Here, I used a different approach that identifies a line of symmetry and finds another divisor by dividing the given number by one of its divisors. This method has a time complexity of O(sqrt(n)).
And I solved another problem too; please check my GitHub repository using the link below.
https://github.com/r-rajesh4/DSA-Coding/tree/main/Math%20Coding
Subscribe to my newsletter
Read articles from Rajesh Sahu directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
