Understanding MVC: A Simple Introduction to Software Architecture
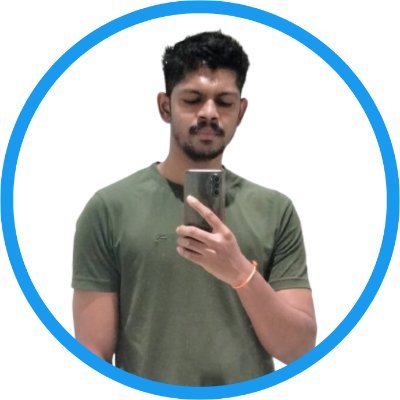
When building applications, maintaining a clean, modular, and scalable architecture is key to long-term success. The Model-View-Controller (MVC) pattern is one of the most widely used architectural patterns, providing a clear separation of concerns and simplifying development. Whether you’re developing a web app, a mobile app, or even a desktop application, understanding MVC can help you structure your code more effectively.
What is the MVC Pattern?
The Model-View-Controller (MVC) pattern divides your application into three interconnected components:
Model:
Manages the data and business logic of the application.
Represents the state of the application.
Interacts with databases, APIs, or any external services.
View:
The presentation layer responsible for what the user sees.
Displays data from the model to the user.
Captures user interactions and sends them to the controller.
Controller:
Acts as the intermediary between the model and the view.
Processes user input, manipulates the model, and updates the view.
Defines the application’s flow and behavior.
How Does MVC Work?
Here’s an example of how these components interact:
User Input: A user clicks a button or submits a form.
Controller: The controller processes the user input and updates the model.
Model: The model retrieves or updates the data (e.g., fetches records from a database).
View: The view displays the updated data back to the user.
This separation of responsibilities ensures that changes in one layer don’t directly affect the others, making your application easier to maintain and scale.
Why Use MVC?
Separation of Concerns:
Each component has a distinct responsibility.
Changes in one layer (e.g., swapping a database in the model) don’t directly affect the others.
Scalability:
- MVC structures your codebase into modular pieces, making it easier to grow and maintain.
Reusability:
Views and controllers can be reused across different parts of the application.
Models can be shared among different views.
Testability:
- MVC makes it easier to write unit tests for individual components.
Breaking Down the Components
1. Model
The model is the heart of your application’s logic. It:
Handles the state of the application.
Interacts with the database or APIs.
Contains the core business rules.
Example in a Node.js app using Prisma ORM:
import { PrismaClient } from '@prisma/client';
const prisma = new PrismaClient();
export const getUserById = async (id: number) => {
return await prisma.user.findUnique({ where: { id } });
};
2. View
The view is what the user interacts with. It:
Displays data from the model.
Captures user interactions and sends them to the controller.
Example in a React component:
const UserProfile = ({ user }: { user: { id: number; name: string } }) => {
return (
<div>
<h1>{user.name}</h1>
<p>ID: {user.id}</p>
</div>
);
};
3. Controller
The controller ties everything together. It:
Receives user input.
Interacts with the model to fetch or update data.
Passes the data to the view for rendering.
Example in an Express.js app:
import { Request, Response } from 'express';
import { getUserById } from './model';
export const getUserController = async (req: Request, res: Response) => {
const userId = parseInt(req.params.id);
const user = await getUserById(userId);
if (!user) {
return res.status(404).send('User not found');
}
res.json(user);
};
MVC in Action
Here’s how the pieces come together in a real-world scenario:
A user navigates to
/user/1
.The controller (
getUserController
) processes the request, calls the model (getUserById
), and retrieves user data from the database.The view (
UserProfile
) renders the user data and sends it back to the client.
When Should You Use MVC?
MVC works well for:
Applications with a clear separation of data, UI, and logic.
Multi-page or multi-screen applications where different views share the same data model.
Projects that require scalability and maintainability.
Conclusion
The Model-View-Controller (MVC) pattern remains a cornerstone of software development. By dividing your application into three distinct layers, MVC ensures that your codebase is clean, modular, and easy to manage. Whether you’re building a simple web app or a complex enterprise solution, understanding and implementing MVC can significantly improve your development process.
Now that you know the basics of MVC, why not try it in your next project? Start small, and see how this timeless pattern can make your life as a developer easier!
Subscribe to my newsletter
Read articles from Sanjit Gawade directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
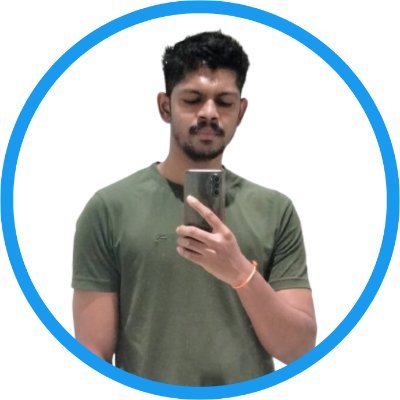
Sanjit Gawade
Sanjit Gawade
ME IT'22 GEC GOA