Optimizing Database Performance in Backend Systems with Indexing, Partitioning, and Caching
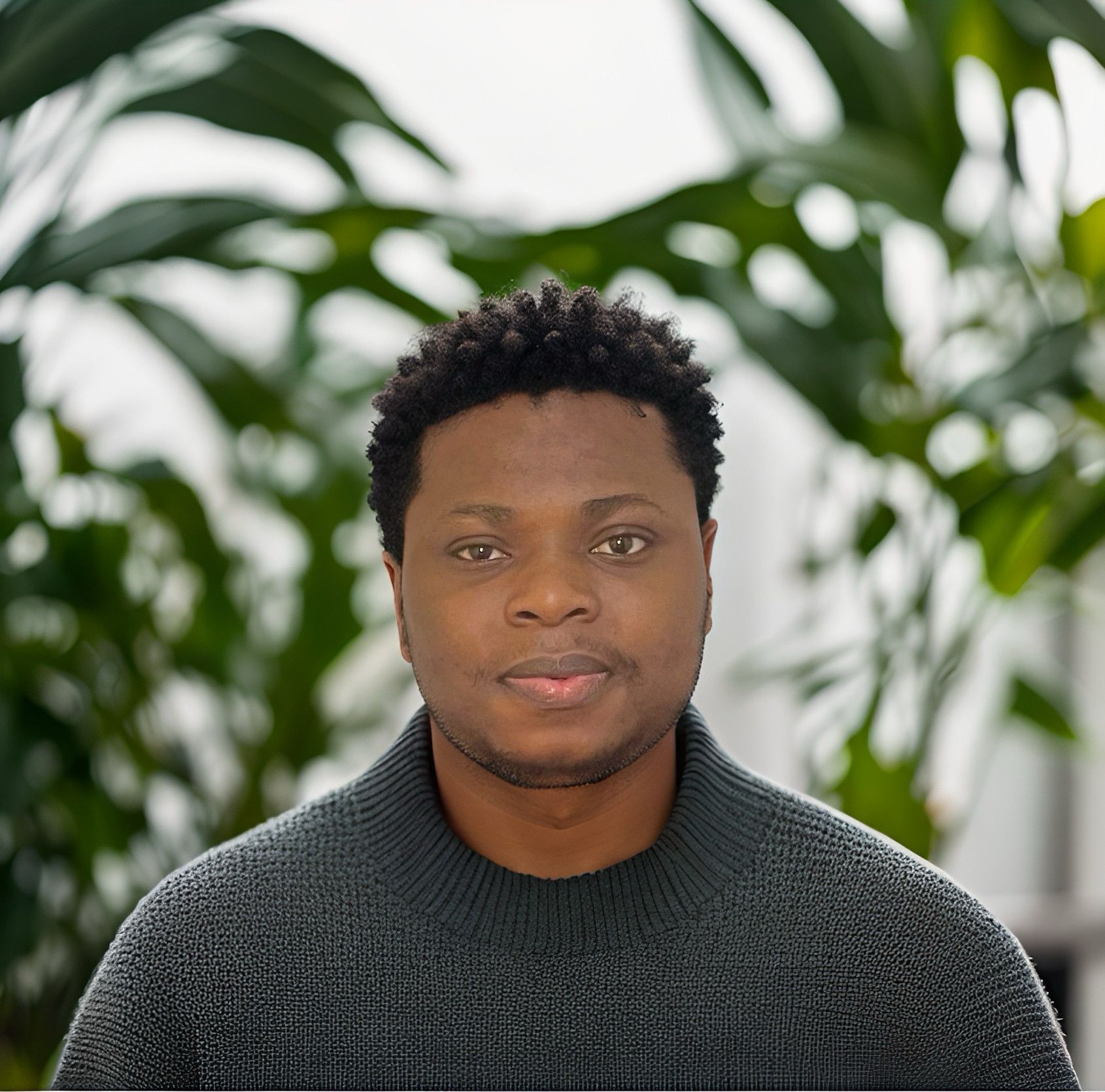
Table of contents
- Identifying Database Performance Bottlenecks
- 1. Indexing: Speed Up Query Execution
- 2. Partitioning: Divide and Conquer
- 3. Caching: Reduce Database Load
- Combining Techniques for Maximum Performance
- Tools and Technologies
- Example Case: Optimizing a User Search System
- Best Practices for Database Optimization
- Conclusion
Databases are the backbone of most backend systems, and their performance directly impacts application speed and scalability. Poor database performance can lead to slow queries, high latency, and an overall degraded user experience. By leveraging techniques like indexing, partitioning, and caching, you can significantly optimize database performance.
This article dives into these techniques, helping you identify performance bottlenecks, implement database-specific optimizations, and integrate caching mechanisms using tools like Redis or Memcached.
Identifying Database Performance Bottlenecks
Before optimizing, you must understand where the bottlenecks are. Common signs of database performance issues include:
Slow Queries: Queries that take too long to execute.
High Latency: Delayed responses from the database server.
Increased Resource Usage: High CPU, memory, or disk I/O utilization.
Connection Timeouts: Occasional or frequent dropped connections.
Tools to Identify Bottlenecks:
Database-Specific Tools:
MySQL: Use
EXPLAIN
orSHOW STATUS
.PostgreSQL: Use
EXPLAIN (ANALYZE)
orpg_stat_activity
.
Monitoring Tools:
- Tools like New Relic, Datadog, or Percona Monitoring and Management provide real-time insights.
Query Profilers:
- Profile slow queries using database logs or built-in tools.
1. Indexing: Speed Up Query Execution
Indexes are like a table of contents for your database, helping locate rows faster. Without proper indexing, the database must scan entire tables, which is time-consuming for large datasets.
Types of Indexes:
Single-Column Index: Speeds up queries filtering or sorting by a single column.
Composite Index: Combines multiple columns into a single index for multi-column searches.
Full-Text Index: Optimized for text search operations.
Best Practices:
Index Frequently Queried Columns: Use indexes on columns in
WHERE
,JOIN
, andORDER BY
clauses.CREATE INDEX idx_users_email ON users (email);
Use Covering Indexes: Ensure the index contains all columns required by the query to avoid reading the actual table.
Avoid Over-Indexing: Too many indexes slow down
INSERT
andUPDATE
operations. Regularly analyze and remove unused indexes.Monitor Index Usage: Tools like MySQL’s
information_schema
or PostgreSQL’spg_stat_user_indexes
can identify unused or ineffective indexes.
2. Partitioning: Divide and Conquer
Partitioning splits a large table into smaller, more manageable pieces, allowing the database to perform operations on smaller datasets.
Types of Partitioning:
Horizontal Partitioning (Sharding): Distributes rows into multiple tables or databases based on a partition key. For example, partitioning users by region.
CREATE TABLE users_usa PARTITION OF users FOR VALUES IN ('USA');
Vertical Partitioning: Splits columns into separate tables. For instance, separate frequently accessed columns from rarely accessed ones.
Range Partitioning: Divides data based on ranges of values (e.g., dates or numeric ranges).
CREATE TABLE sales_q1 PARTITION OF sales FOR VALUES FROM (1) TO (4);
Benefits of Partitioning:
Improved Query Performance: Queries scan only relevant partitions.
Easier Maintenance: Simplifies operations like archiving and purging old data.
Load Distribution: Distributes data across multiple disks or servers.
Tools and Support:
SQL Databases: PostgreSQL, MySQL, and SQL Server offer native partitioning features.
NoSQL Databases: MongoDB and Cassandra inherently support sharding.
3. Caching: Reduce Database Load
Caching stores frequently accessed data in memory, reducing the need for repeated database queries.
Tools for Caching:
Redis: A fast, in-memory key-value store with support for data structures.
Memcached: Lightweight, high-performance key-value caching system.
Caching Strategies:
Query Result Caching: Store the results of expensive queries in a cache.
const redis = require("redis"); const client = redis.createClient(); async function getCachedData(queryKey, queryFunction) { const cachedData = await client.get(queryKey); if (cachedData) return JSON.parse(cachedData); const result = await queryFunction(); await client.set(queryKey, JSON.stringify(result), { EX: 3600 }); return result; }
Object Caching: Cache frequently accessed objects like user profiles or session data.
Page Caching: Store pre-rendered pages or partial content for static or semi-dynamic responses.
Write-Through Caching: Update the cache as soon as data is written to the database, ensuring freshness.
Read-Through Caching: Query the cache first and only access the database on a cache miss.
Avoiding Common Caching Pitfalls:
Stale Data: Use cache expiration (
TTL
) to keep data fresh.Cache Invalidation: Implement strategies to update or remove outdated cache entries when data changes.
Combining Techniques for Maximum Performance
To achieve the best results, combine indexing, partitioning, and caching.
Example Workflow:
Analyze Bottlenecks: Use query profiling tools to identify slow queries.
Apply Indexing: Add indexes to improve query execution time.
Partition Data: Partition large tables for better query focus and scalability.
Implement Caching: Cache the results of frequent queries or static data to reduce database load.
Tools and Technologies
SQL Databases:
- MySQL, PostgreSQL, Microsoft SQL Server.
NoSQL Databases:
- MongoDB (supports sharding), Cassandra (native partitioning).
Caching Systems:
- Redis, Memcached.
Monitoring:
- pg_stat_activity, MySQL Query Profiler, Datadog, Prometheus.
Example Case: Optimizing a User Search System
Suppose you have a user database with millions of records and frequent searches by email or name.
Indexing:
Add indexes on
email
andname
fields:CREATE INDEX idx_email ON users (email); CREATE INDEX idx_name ON users (name);
Partitioning:
Partition the
users
table by region:CREATE TABLE users_north PARTITION OF users FOR VALUES IN ('North');
Caching:
Cache search results in Redis to handle frequent requests efficiently:
const redis = require("redis");
const client = redis.createClient();
async function searchUser(email) {
const cachedUser = await client.get(email);
if (cachedUser) return JSON.parse(cachedUser);
const user = await db.query("SELECT * FROM users WHERE email = ?", [email]);
await client.set(email, JSON.stringify(user), { EX: 3600 });
return user;
}
Best Practices for Database Optimization
Monitor Regularly: Continuously analyze performance to detect new bottlenecks.
Optimize Queries: Write efficient SQL queries, avoiding unnecessary joins or subqueries.
Choose the Right Tools: Use database-specific features like PostgreSQL’s
VACUUM
or MySQL’sANALYZE TABLE
.Scale Proactively: Partition or shard data before it becomes unmanageable.
Leverage Caching Wisely: Balance between cache freshness and performance.
Conclusion
Optimizing database performance in backend systems requires a combination of techniques tailored to your specific workload. Indexing accelerates query execution, partitioning scales data management, and caching reduces load on your database.
By implementing these strategies, you can build backend systems that handle high traffic, scale efficiently, and deliver excellent user experiences. Start by identifying bottlenecks, applying targeted optimizations, and monitoring results to ensure sustained performance improvements.
Subscribe to my newsletter
Read articles from Nicholas Diamond directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
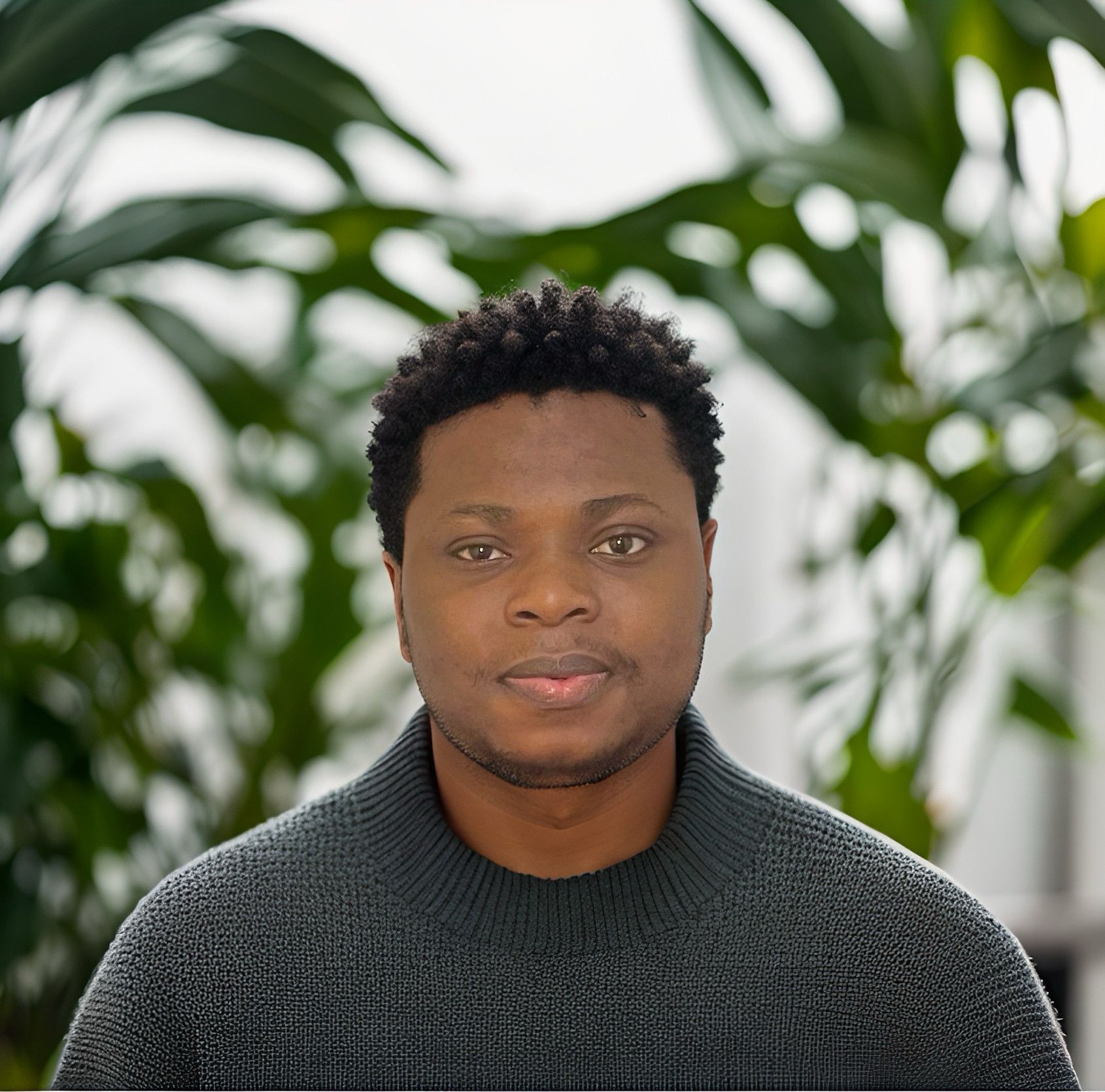