Securing APIs with OAuth2 and OpenID Connect for Modern Web Applications
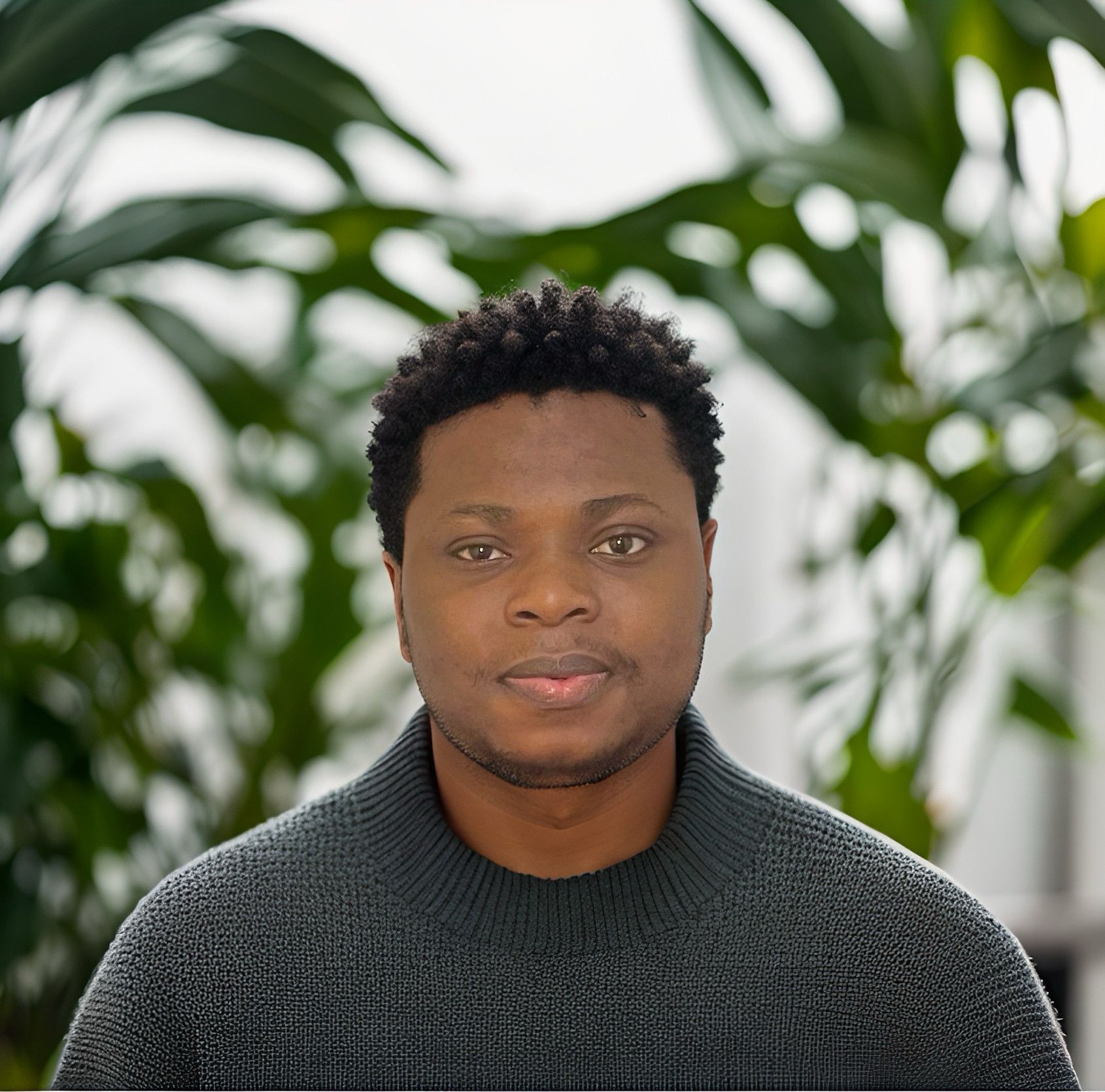
Table of contents
- What Are OAuth2 and OpenID Connect?
- Key Concepts of OAuth2 and OpenID Connect
- Example Use Case: Securing a Node.js API with OAuth2 and OpenID Connect
- Step 1: Set Up an OAuth2 Provider
- Step 2: Set Up the Node.js API
- Step 3: Implement Token-Based Authentication in a Client
- Best Practices for Securing APIs with OAuth2 and OIDC
- Wrapping Up
In today’s interconnected world, securing RESTful APIs is critical. As APIs often handle sensitive data, ensuring that only authorized users and applications can access them is paramount. OAuth2 and OpenID Connect (OIDC) have emerged as robust frameworks to secure APIs, enabling secure authentication and authorization while supporting modern use cases like single sign-on (SSO) and third-party integrations.
This article explores how to implement OAuth2 and OpenID Connect in your APIs using Node.js, covering token-based authentication, managing access tokens securely, and best practices for robust API security.
What Are OAuth2 and OpenID Connect?
OAuth2: A protocol for authorization, OAuth2 allows users to grant third-party applications access to their resources without sharing credentials. It uses tokens for secure communication between the client, API, and authorization server.
OpenID Connect (OIDC): Built on top of OAuth2, OIDC adds an identity layer to handle user authentication. It provides a standard way to verify user identity and access user profile information.
Key Concepts of OAuth2 and OpenID Connect
Access Tokens:
Short-lived tokens used to access protected resources (APIs).
Example: JWT (JSON Web Token).
Refresh Tokens:
- Long-lived tokens used to obtain new access tokens without requiring user re-authentication.
Authorization Flows:
Authorization Code Flow: Best for server-side applications.
Implicit Flow: Suitable for public clients like SPAs (deprecated for security reasons).
Client Credentials Flow: Used for machine-to-machine communication.
Password Flow: Avoided due to security concerns but involves direct credential exchange.
Scopes:
- Define the permissions or resources the client can access.
Example Use Case: Securing a Node.js API with OAuth2 and OpenID Connect
Prerequisites:
A Node.js environment.
An OAuth2 provider (e.g., Auth0, Okta, Keycloak, or AWS Cognito).
Basic knowledge of Express.js.
Step 1: Set Up an OAuth2 Provider
For this example, we’ll use Auth0 as the OAuth2 and OIDC provider.
Create an API:
Go to the Auth0 dashboard and create a new API.
Set an identifier (e.g.,
https://myapi.example.com
).Define scopes (e.g.,
read:data
,write:data
).
Register a Client Application:
Create a client application in Auth0.
Configure the redirect URIs (e.g.,
http://localhost:3000/callback
).
Step 2: Set Up the Node.js API
Install the required libraries:
npm install express express-jwt jwks-rsa dotenv
API Code:
Load Environment Variables: Create a
.env
file to store sensitive information securely:AUTH0_DOMAIN=your-auth0-domain AUTH0_AUDIENCE=your-auth0-api-identifier
Create an Express.js API:
const express = require('express'); const { expressjwt: jwt } = require('express-jwt'); const jwksRsa = require('jwks-rsa'); require('dotenv').config(); const app = express(); const port = 3000; // Middleware to validate access tokens const checkJwt = jwt({ secret: jwksRsa.expressJwtSecret({ cache: true, rateLimit: true, jwksRequestsPerMinute: 5, jwksUri: `https://${process.env.AUTH0_DOMAIN}/.well-known/jwks.json` }), audience: process.env.AUTH0_AUDIENCE, issuer: `https://${process.env.AUTH0_DOMAIN}/`, algorithms: ['RS256'] }); // Protected route app.get('/api/protected', checkJwt, (req, res) => { res.send({ message: 'You have accessed a protected API route!' }); }); // Public route app.get('/api/public', (req, res) => { res.send({ message: 'This is a public API route.' }); }); app.listen(port, () => { console.log(`API is running on http://localhost:${port}`); });
Test the API:
Access the public route:
http://localhost:3000/api/public
.Try the protected route with a valid access token.
Step 3: Implement Token-Based Authentication in a Client
Node.js Client Example:
Install Axios:
npm install axios
Client Code:
const axios = require('axios'); const qs = require('qs'); const AUTH0_DOMAIN = 'your-auth0-domain'; const CLIENT_ID = 'your-client-id'; const CLIENT_SECRET = 'your-client-secret'; const AUDIENCE = 'https://myapi.example.com'; async function getAccessToken() { const tokenUrl = `https://${AUTH0_DOMAIN}/oauth/token`; const data = qs.stringify({ grant_type: 'client_credentials', client_id: CLIENT_ID, client_secret: CLIENT_SECRET, audience: AUDIENCE }); const config = { headers: { 'Content-Type': 'application/x-www-form-urlencoded' } }; const response = await axios.post(tokenUrl, data, config); return response.data.access_token; } async function callProtectedApi() { const token = await getAccessToken(); const response = await axios.get('http://localhost:3000/api/protected', { headers: { Authorization: `Bearer ${token}` } }); console.log(response.data); } callProtectedApi().catch(console.error);
Best Practices for Securing APIs with OAuth2 and OIDC
Use HTTPS: Always use HTTPS for API endpoints to encrypt communication.
Validate Tokens: Verify access tokens against the authorization server to prevent tampering.
Use Short-Lived Tokens: Minimize the lifetime of access tokens and use refresh tokens for obtaining new ones.
Define Fine-Grained Scopes: Grant only the permissions a client application needs.
Rotate Keys Regularly: Use a JWKS endpoint and rotate keys periodically to strengthen security.
Monitor Token Usage: Log and analyze token usage to detect unauthorized access.
Secure Secrets: Never hardcode client credentials; use environment variables or secrets management tools.
Wrapping Up
Securing RESTful APIs is essential for protecting sensitive data and ensuring reliable user interactions. By implementing OAuth2 and OpenID Connect, you can secure your APIs while enabling modern authentication and authorization use cases like SSO and third-party integrations.
Using tools like Auth0 and libraries like express-jwt
, you can seamlessly integrate OAuth2 into your Node.js backend, ensuring that only authorized users and applications access your resources. With proper best practices, you can maintain the security, scalability, and reliability of your APIs in today’s rapidly evolving web environment.
Subscribe to my newsletter
Read articles from Nicholas Diamond directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
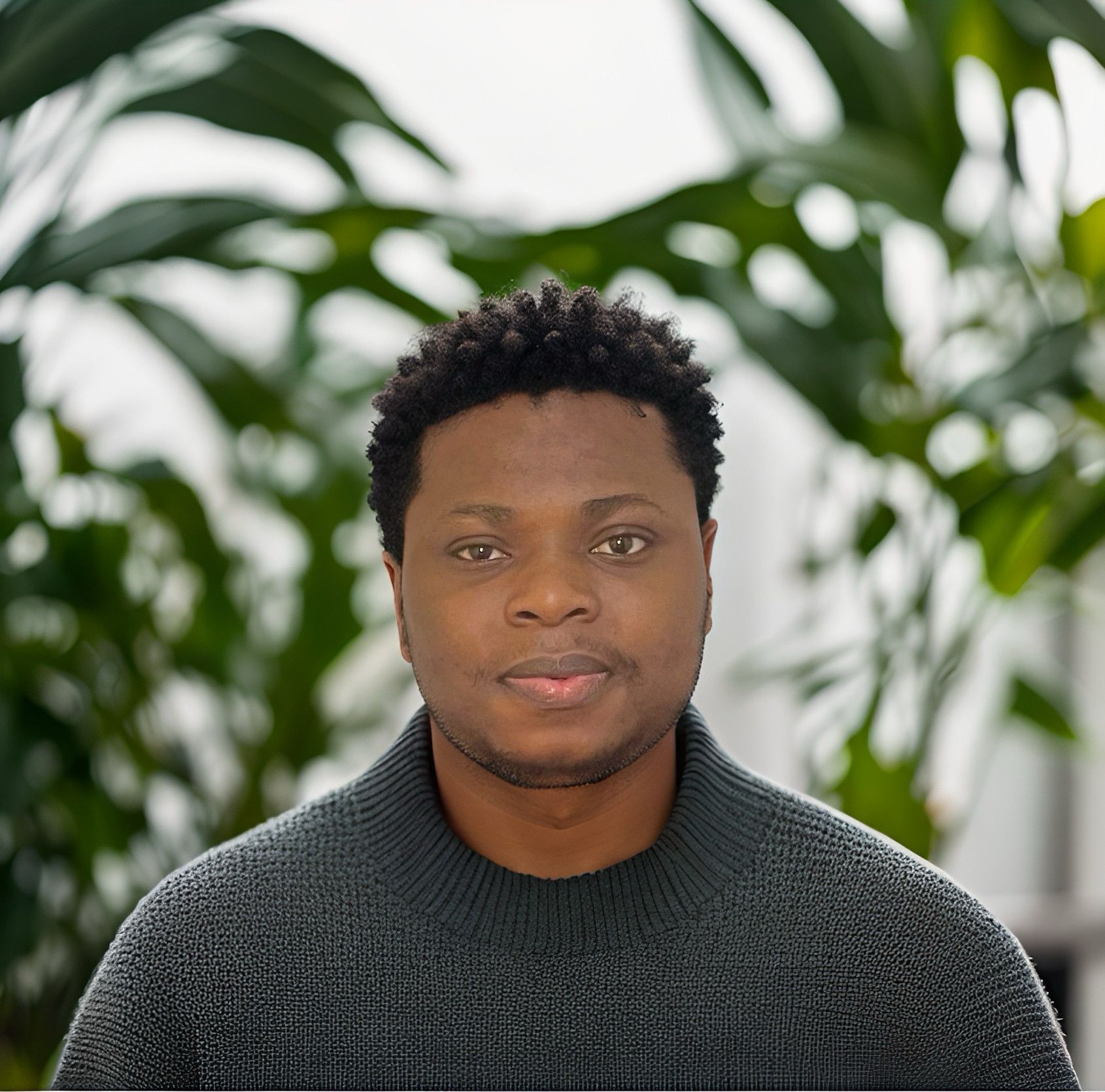