Python Project: Building a Store Calculator

Table of contents
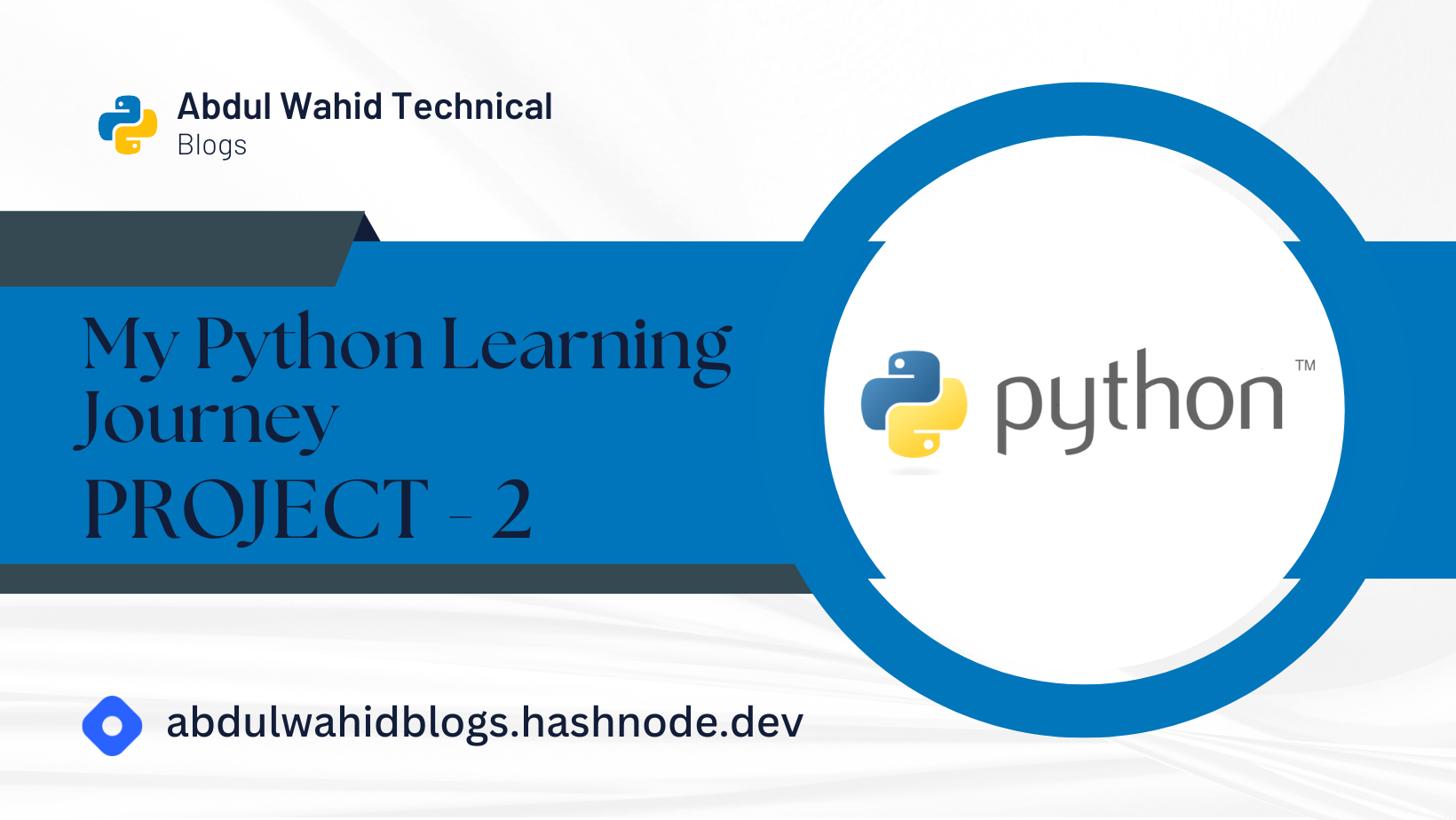
Introduction:
I created a Store Calculator using Python. It is a simple program that calculates the total amount of items, allows the user to quit by pressing "q," and generates a receipt with all item prices. In this article, I’ll share how I built this calculator, the challenges I faced, and their solutions.
These are the steps I took to grasp the concepts:
The first step is to understand the below objective:
Accept item prices as input.
Allow quitting by pressing "q."
Generate a receipt with items and the total price.
Writing the Code:
Used a while loop to get inputs until the user quits.
Stored prices and calculated the total.
Displayed a receipt at the end.
Error Handling:
Checked for invalid inputs (e.g., letters other than "q" or empty values).
Prevented negative prices from being entered.
Below is the full code snippet :
These are the problems that I encountered:
Validating User Input to Avoid Crashes.
Negative Price Handling Error.
Misplacement of
break
Statement.
This is how I solved those problems:
1. Validating User Input to Avoid Crashes:
Problem**:** The program would crash if the user entered something other than a number or "q."
userinput = input("Enter the item price or press q to quit: ")
# If the input is not a valid number or "q", the program would crash.
Solution: Added try-except
block to catch invalid inputs and prompt the user correctly.
try:
price = float(userinput) # Try to convert the input to a number
except ValueError:
print("Invalid input. Please enter a valid number or 'q' to quit.")
2. Negative Price Handling Error:
Problem: The program accepted negative prices, which doesn’t make sense for the store calculator.
price = float(userinput)
# No validation for negative price.
Solution: Added a check to ensure only positive numbers are accepted.
if price < 0:
print("Invalid input. Please enter a positive number.")
3. Misplacement of break
Statement:
Problem**:** The break
statement was placed inside the loop after every valid input, causing the program to exit prematurely.
if userinput.lower() == "q":
print("\nReceipt Generated:")
break # Incorrect placement of break
Solution: Moved the break
statement to only activate when the user presses "q" and the receipt is ready.
if userinput.lower() == "q":
print("\nReceipt Generated:")
for i, price in enumerate(prices, start=1):
print(f"Item {i}: {price}")
print(f"\nTotal: {sum:.2f}")
break # Correct placement to exit after generating receipt
Output when running in the terminal:
Enter the item price or press 'q' to quit: 25
The total price so far: 25.0.
Enter the item price or press 'q' to quit:
Invalid input. Please enter a valid number or 'q' to quit.
Enter the item price or press 'q' to quit: -95
Invalid input. Please enter a positive number or 'q' to quit.
Enter the item price or press 'q' to quit: 95
The total price so far: 120.0.
Enter the item price or press 'q' to quit: 40
The total price so far: 160.0.
Enter the item price or press 'q' to quit: P
Invalid input. Please enter a valid number or 'q' to quit.
Enter the item price or press 'q' to quit: q
Receipt Generated:
Item 1: 25.0
Item 2: 95.0
Item 3: 40.0
The total price is 160.0. Thanks for shopping.
Resources:
My GitHub repository with the project code: GitHub Link
Subscribe to my newsletter
Read articles from Sheikh Abdul Wahid directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
