Event-Driven Architecture in Node.js Using RabbitMQ and Kafka
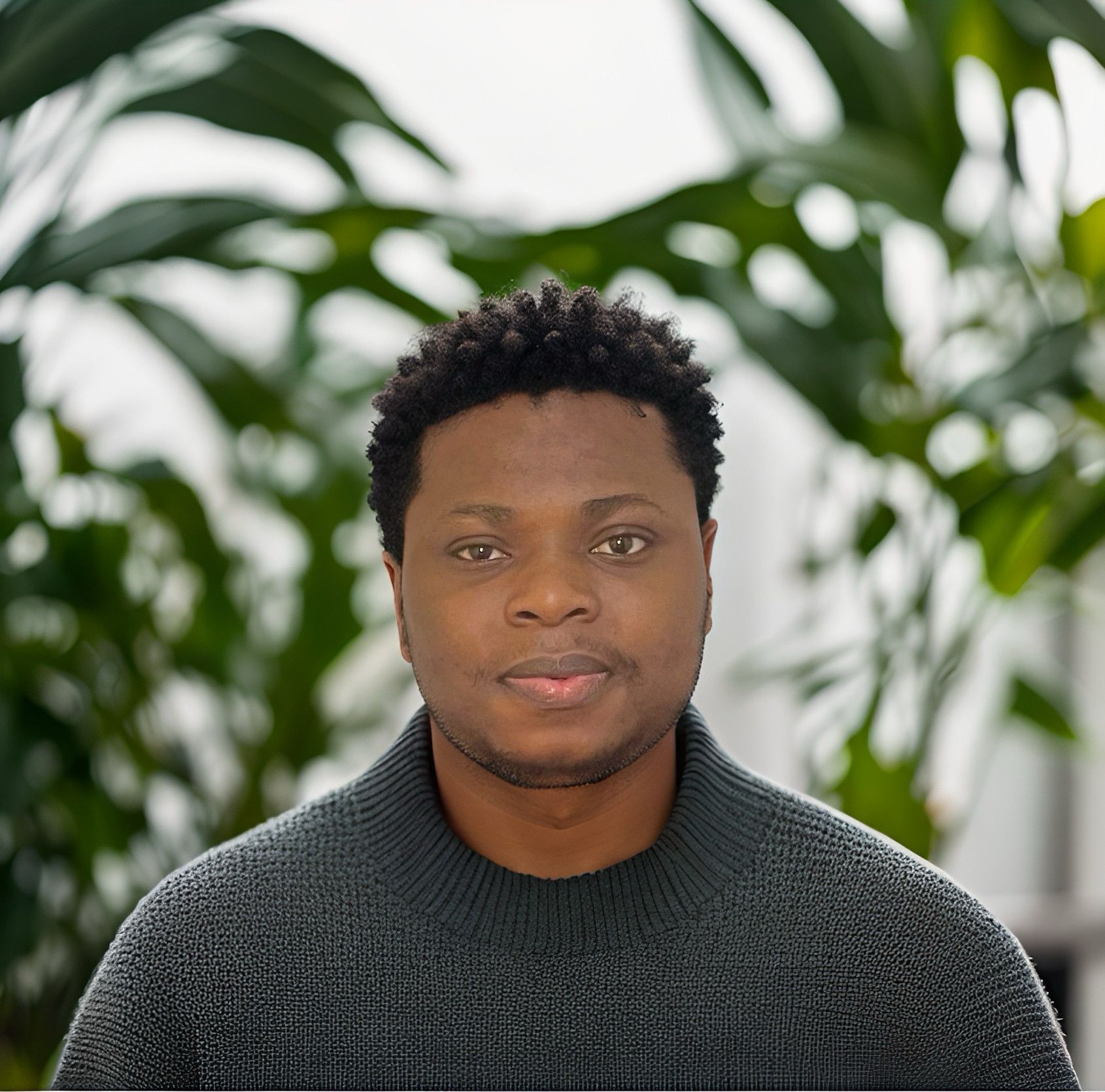
Modern applications often require real-time updates, asynchronous processing, and the ability to scale seamlessly. Event-driven architecture (EDA) offers an efficient way to design systems that are resilient, scalable, and responsive by using message brokers like RabbitMQ and Apache Kafka.
This article explores how to implement an event-driven architecture in Node.js, covering key concepts such as event producers, consumers, and strategies for fault tolerance in distributed systems. We’ll compare RabbitMQ and Kafka and provide practical examples to help you get started.
What Is Event-Driven Architecture?
Event-driven architecture revolves around the idea of events: a significant change in the system state (e.g., user registration, order placement). These events are produced and consumed asynchronously, enabling decoupled communication between services.
Producers: Emit events when something noteworthy happens.
Consumers: React to events and perform tasks based on the received data.
Message Broker: Manages event delivery between producers and consumers.
This model is highly beneficial for building scalable systems, as it allows services to operate independently while maintaining responsiveness.
RabbitMQ vs. Apache Kafka: When to Use Each
Feature | RabbitMQ | Apache Kafka |
Type | Traditional Message Queue | Distributed Streaming Platform |
Use Case | Task queues, request-response | Event streams, log aggregation |
Message Retention | Deletes after consumption | Persistent storage (configurable) |
Ordering | Queue-level ordering | Partition-level ordering |
Scalability | Scales vertically with clusters | Scales horizontally with partitions |
Use RabbitMQ for traditional messaging patterns like work queues and short-lived messages.
Use Kafka for real-time data pipelines and long-term event storage.
Setting Up Event-Driven Architecture with RabbitMQ
1. Install RabbitMQ
You can install RabbitMQ using Docker:
docker run -d --name rabbitmq -p 5672:5672 -p 15672:15672 rabbitmq:management
Access the management interface at http://localhost:15672
(default credentials: guest/guest
).
2. Create a Producer
Install dependencies:
npm install amqplib
Code for the producer:
const amqp = require('amqplib');
async function sendMessage() {
const connection = await amqp.connect('amqp://localhost');
const channel = await connection.createChannel();
const queue = 'task_queue';
await channel.assertQueue(queue, { durable: true });
const message = { task: 'processOrder', orderId: 12345 };
channel.sendToQueue(queue, Buffer.from(JSON.stringify(message)), { persistent: true });
console.log(`Sent: ${JSON.stringify(message)}`);
await channel.close();
await connection.close();
}
sendMessage().catch(console.error);
3. Create a Consumer
const amqp = require('amqplib');
async function receiveMessages() {
const connection = await amqp.connect('amqp://localhost');
const channel = await connection.createChannel();
const queue = 'task_queue';
await channel.assertQueue(queue, { durable: true });
console.log(`Waiting for messages in ${queue}`);
channel.consume(queue, (msg) => {
const message = JSON.parse(msg.content.toString());
console.log(`Received: ${JSON.stringify(message)}`);
channel.ack(msg);
});
}
receiveMessages().catch(console.error);
Setting Up Event-Driven Architecture with Kafka
1. Install Kafka
You can install Kafka using Docker Compose:
version: '3.8'
services:
zookeeper:
image: confluentinc/cp-zookeeper
environment:
ZOOKEEPER_CLIENT_PORT: 2181
ports:
- "2181:2181"
kafka:
image: confluentinc/cp-kafka
depends_on:
- zookeeper
environment:
KAFKA_ZOOKEEPER_CONNECT: zookeeper:2181
KAFKA_ADVERTISED_LISTENERS: PLAINTEXT://localhost:9092
KAFKA_OFFSETS_TOPIC_REPLICATION_FACTOR: 1
ports:
- "9092:9092"
Run Kafka with:
docker-compose up -d
2. Create a Producer
Install Kafka client library:
npm install kafkajs
Code for the producer:
const { Kafka } = require('kafkajs');
async function sendMessage() {
const kafka = new Kafka({ brokers: ['localhost:9092'] });
const producer = kafka.producer();
await producer.connect();
const topic = 'order_events';
const message = { task: 'processOrder', orderId: 12345 };
await producer.send({
topic,
messages: [{ value: JSON.stringify(message) }],
});
console.log(`Sent: ${JSON.stringify(message)}`);
await producer.disconnect();
}
sendMessage().catch(console.error);
3. Create a Consumer
const { Kafka } = require('kafkajs');
async function consumeMessages() {
const kafka = new Kafka({ brokers: ['localhost:9092'] });
const consumer = kafka.consumer({ groupId: 'order_group' });
await consumer.connect();
await consumer.subscribe({ topic: 'order_events', fromBeginning: true });
console.log('Waiting for messages...');
await consumer.run({
eachMessage: async ({ topic, partition, message }) => {
const msg = JSON.parse(message.value.toString());
console.log(`Received: ${JSON.stringify(msg)}`);
},
});
}
consumeMessages().catch(console.error);
Fault Tolerance Strategies
RabbitMQ:
Message Durability: Set
durable: true
for queues andpersistent: true
for messages.Acknowledgments: Use
channel.ack(msg)
to confirm processing.
Kafka:
Replication: Configure partitions with replication for failover.
Consumer Groups: Ensure high availability by distributing messages across consumers.
Observability in Event-Driven Systems
Logging: Centralize logs using tools like ELK Stack (Elasticsearch, Logstash, Kibana).
Metrics: Use Prometheus and Grafana to monitor queue lengths, consumer lag, and throughput.
Tracing: Implement distributed tracing with OpenTelemetry to visualize event flow across services.
When to Choose RabbitMQ or Kafka
Scenario | Recommended Tool |
Task queues and transient messages | RabbitMQ |
Real-time streaming or log data | Kafka |
High durability and replayability | Kafka |
Simpler setups and use cases | RabbitMQ |
Wrapping Up
Event-driven architecture is essential for building responsive, scalable, and decoupled systems. By leveraging RabbitMQ for task queues or Kafka for event streaming, you can create robust backend systems with Node.js.
Start small by implementing producers and consumers, then scale with fault-tolerant strategies and observability tools. Whether you choose RabbitMQ or Kafka depends on your specific use case, but both are invaluable tools in a modern backend developer’s toolkit.
Focus on designing for scalability, resilience, and transparency to future-proof your event-driven system.
Subscribe to my newsletter
Read articles from Nicholas Diamond directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
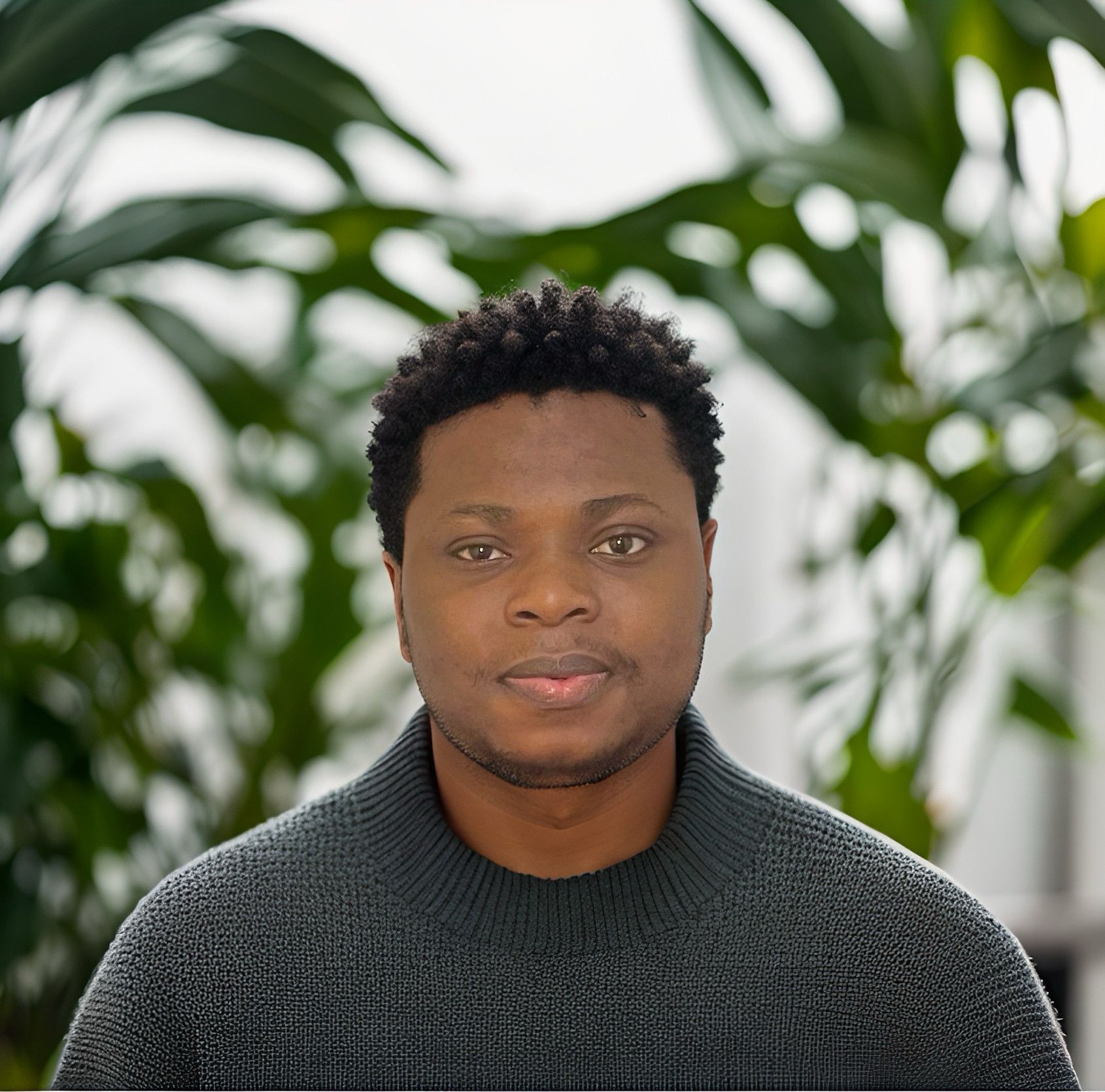