A Beginner’s Guide to Version Control with Git

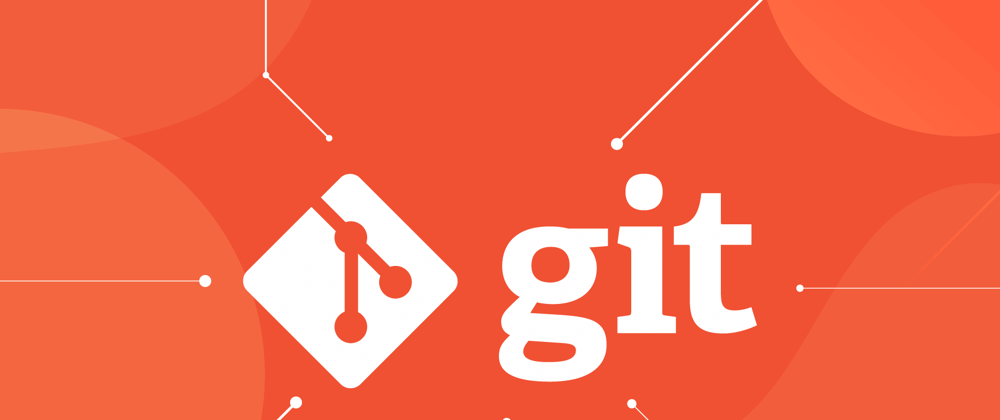
Introduction
If you’re new to programming or software development, you’ve probably heard of Git and version control. These tools are essential for managing code, collaborating with others, and ensuring that changes are tracked in an organized way. In this article, we’ll walk through the basics of Git, why it’s important, and how to get started with it.
What is Version Control?
Version control is a system that records changes to a file or set of files over time, allowing you to track progress, collaborate with others, and even revert to previous versions if needed. This is especially useful in software development, where multiple people may be working on the same project simultaneously.
Without version control, managing changes can quickly become chaotic. A good version control system helps you:
Track changes: See who made what changes and when.
Revert to previous versions: If something breaks, you can easily roll back to a stable state.
Collaborate efficiently: Multiple people can work on the same project without overwriting each other’s work.
What is Git?
Git is a popular distributed version control system that allows multiple developers to work on the same project efficiently. Unlike centralized version control systems, Git is distributed, meaning each developer has a full copy of the project’s history on their machine.
Key Features of Git:
Distributed: Every developer has their own local copy of the repository.
Branching and Merging: Easily create and merge branches, which is great for trying out new features without affecting the main project.
Commit History: Every change you make is recorded in a history log, making it easy to track changes over time.
Getting Started with Git
Let’s dive into how to set up and use Git. First, you’ll need to install Git on your machine.
Step 1: Install Git
Git can be installed on different operating systems, including Windows, macOS, and Linux.
Windows: Download Git from the official website git-scm.com and follow the installation instructions.
macOS: You can install Git using Homebrew by running the following command:
brew install git
- Linux: On most Linux distributions, Git is available through the default package manager. For example:
sudo apt-get install git
Step 2: Configure Git
After installing Git, you’ll want to configure it with your name and email, which will be associated with your commits.
git config --global user.name "Your Name"
git config --global user.email "you@example.com"
Step 3: Create a Git Repository
A Git repository is where your project files and their version history are stored. To create a new Git repository, navigate to your project folder and initialize Git.
cd your-project-folder
git init
This creates a hidden .git
folder that tracks all the changes to your project.
Step 4: Add Files to the Repository
Once Git is initialized, you can start adding files to the repository. Let’s say you have a file called index.html
in your project. You can add it to Git with the following command:
git add index.html
This stages the file, telling Git that you want to include it in the next commit.
Step 5: Commit Changes
After staging your files, the next step is to commit the changes. A commit is like a snapshot of your project at a specific point in time. To commit your changes, use:
git commit -m "Initial commit"
The -m
option allows you to include a message describing the changes. Writing meaningful commit messages is important, especially when working on larger projects.
Step 6: Check Status
To see the current state of your project and which files have been changed, you can use the following command:
git status
This will tell you which files have been modified, which are staged for commit, and which are untracked.
Step 7: Viewing Commit History
You can view the history of your project with:
git log
This will display a list of commits along with the associated messages, commit hashes, and authors.
Branching in Git
One of Git’s most powerful features is branching. Branches allow you to work on different parts of a project simultaneously without affecting the main codebase.
Create a New Branch
To create a new branch, use the following command:
git checkout -b new-feature
This creates a new branch called new-feature
and switches to it. You can now work on this branch without affecting the main branch.
Merging Branches
Once you’re done working on your branch, you’ll want to merge it back into the main branch. First, switch back to the main branch:
git checkout main
Then merge your changes:
git merge new-feature
Working with Remote Repositories
Git also allows you to collaborate with others by using remote repositories. A popular service for hosting Git repositories is GitHub.
Cloning a Repository
To clone an existing repository from GitHub, use the following command:
git clone https://github.com/username/repository.git
Pushing Changes
Once you’ve made changes to your local repository, you’ll want to push them to the remote repository so others can see them:
git push origin main
Conclusion
Version control with Git is an essential skill for developers, whether you’re working alone or as part of a team. By understanding the basics of Git—how to initialize repositories, commit changes, work with branches, and collaborate using remote repositories—you’ll be well-equipped to manage your projects effectively.
Happy coding!
Subscribe to my newsletter
Read articles from Likhith SP directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Likhith SP
Likhith SP
My mission is to help beginners in technology by creating easy-to-follow guides that break down complicated operations, installations, and emerging technologies into bite-sized steps.