Distributed Tracing in Microservices Using OpenTelemetry with Node.js
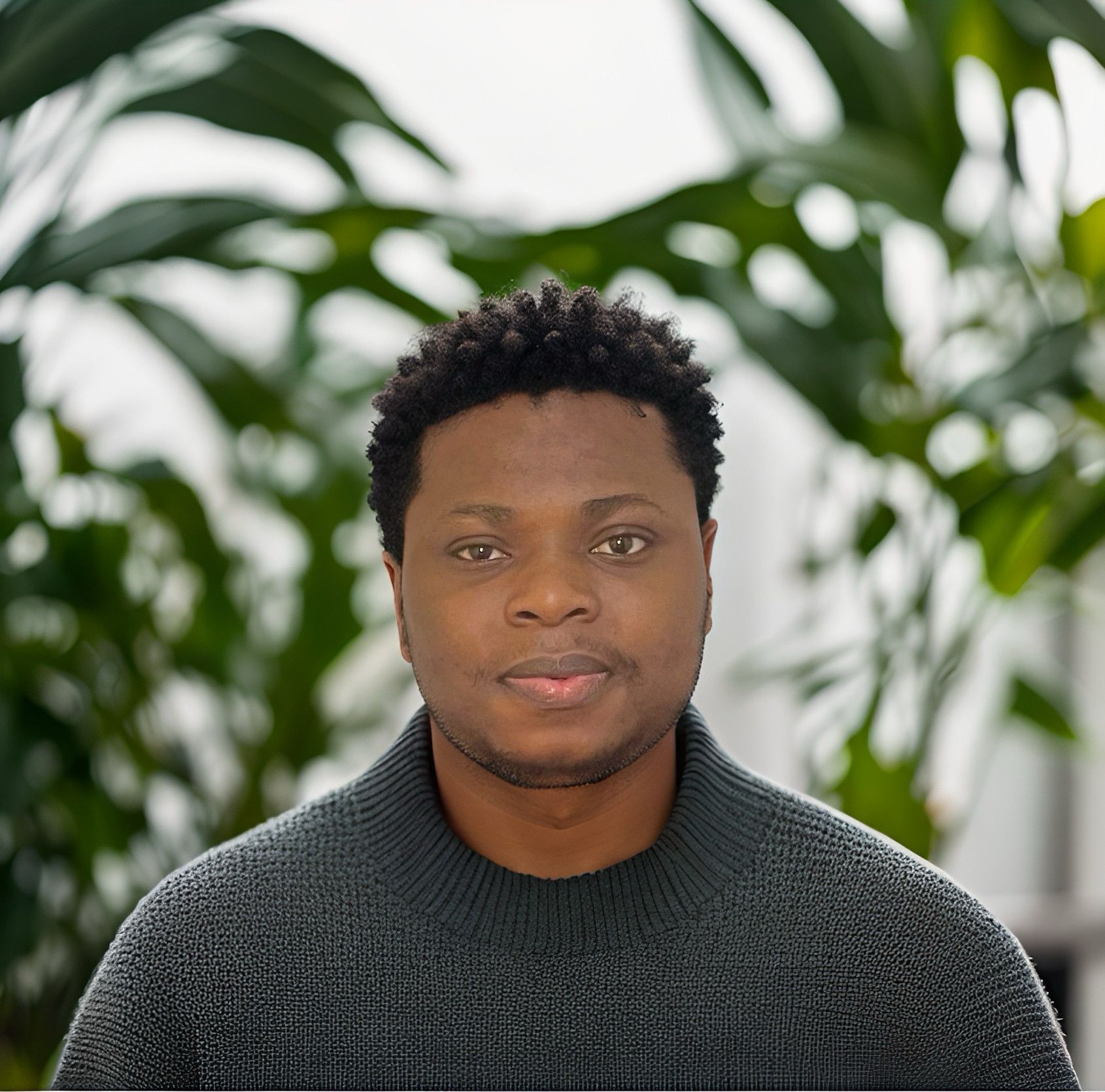
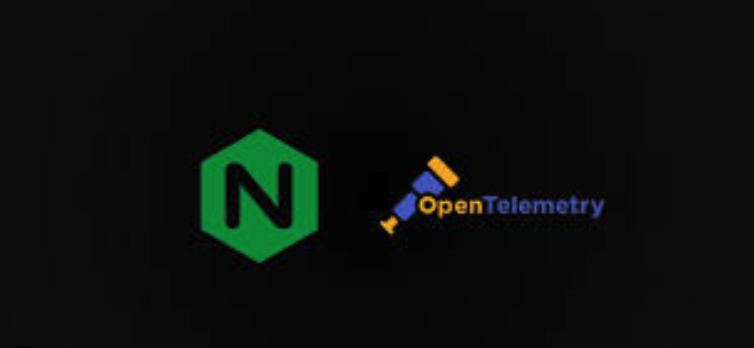
In a microservices architecture, requests often traverse multiple services before reaching a response. Understanding how data flows through these services is essential for diagnosing performance bottlenecks and ensuring reliable operations. This is where distributed tracing comes in, and OpenTelemetry is one of the most powerful tools to achieve this.
In this article, we’ll explore how to implement distributed tracing in Node.js microservices using OpenTelemetry, enabling you to track requests across services and identify performance bottlenecks.
What is Distributed Tracing?
Distributed tracing tracks the lifecycle of a request as it propagates through different components of a distributed system.
Key benefits include:
Diagnosing Latency: Identify which services or components introduce delays.
Error Detection: Pinpoint failures in complex request chains.
Performance Monitoring: Gain insights into overall system performance.
Why OpenTelemetry?
OpenTelemetry is an open-source observability framework that provides APIs, libraries, and agents to capture metrics, logs, and traces.
Benefits of using OpenTelemetry:
Vendor-Agnostic: Works with various backends like Jaeger, Zipkin, or Prometheus.
Standardized: Provides a consistent API and data model.
Extensible: Easily integrates with other observability tools.
Setting Up OpenTelemetry in Node.js
Step 1: Install Required Dependencies
To get started with OpenTelemetry:
npm install @opentelemetry/api @opentelemetry/sdk-node @opentelemetry/auto-instrumentations-node @opentelemetry/exporter-trace-otlp-http
This includes:
@opentelemetry/api
: The core API for tracing.@opentelemetry/sdk-node
: Node.js SDK for OpenTelemetry.@opentelemetry/auto-instrumentations-node
: Automatically instruments popular Node.js modules (e.g., HTTP, Express).@opentelemetry/exporter-trace-otlp-http
: Exporter for sending traces to a backend like Jaeger or Zipkin.
Step 2: Configure OpenTelemetry SDK
Create a file tracing.js
to initialize OpenTelemetry:
const { NodeSDK } = require('@opentelemetry/sdk-node');
const { getNodeAutoInstrumentations } = require('@opentelemetry/auto-instrumentations-node');
const { OTLPTraceExporter } = require('@opentelemetry/exporter-trace-otlp-http');
const traceExporter = new OTLPTraceExporter({
url: 'http://localhost:4318/v1/traces', // Update for your backend
});
const sdk = new NodeSDK({
traceExporter,
instrumentations: [getNodeAutoInstrumentations()],
});
sdk.start().then(() => {
console.log('OpenTelemetry tracing initialized');
}).catch((err) => {
console.error('Error initializing OpenTelemetry tracing', err);
});
Step 3: Integrate Tracing into Your Application
Import tracing.js
at the entry point of your application (e.g., index.js
):
require('./tracing'); // Must be imported before other modules
const express = require('express');
const app = express();
app.use(express.json());
app.get('/service-a', (req, res) => {
setTimeout(() => {
res.send('Response from Service A');
}, 200);
});
app.listen(3000, () => console.log('Service A running on port 3000'));
Step 4: Add Context Propagation Between Services
For microservices, ensure trace context is propagated across HTTP requests. Install and configure @opentelemetry/propagator-b3
or similar propagators.
Example: Propagating Context in HTTP Requests
Install a context propagator:
npm install @opentelemetry/propagator-b3
Update tracing.js
:
const { B3Propagator } = require('@opentelemetry/propagator-b3');
sdk.configure({
textMapPropagator: new B3Propagator(),
});
In a second service (service-b
):
require('./tracing'); // Import OpenTelemetry tracing
const express = require('express');
const axios = require('axios');
const app = express();
app.use(express.json());
app.get('/service-b', async (req, res) => {
try {
const response = await axios.get('http://localhost:3000/service-a');
res.send(`Service B received: ${response.data}`);
} catch (error) {
res.status(500).send('Error calling Service A');
}
});
app.listen(4000, () => console.log('Service B running on port 4000'));
Step 5: Export Traces to a Backend
OpenTelemetry supports multiple trace backends like Jaeger and Zipkin. Let’s use Jaeger as an example.
Install and Run Jaeger
Download and run Jaeger using Docker:
docker run -d --name jaeger \
-e COLLECTOR_ZIPKIN_HTTP_PORT=9411 \
-p 5775:5775/udp \
-p 6831:6831/udp \
-p 6832:6832/udp \
-p 5778:5778 \
-p 16686:16686 \
-p 14268:14268 \
-p 14250:14250 \
-p 9411:9411 \
jaegertracing/all-in-one:latest
Access the Jaeger UI at http://localhost:16686
.
Step 6: Visualizing Traces
When you call the endpoints (/service-b
), traces appear in Jaeger showing the flow of requests between services.
Key details include:
Service Names: Each traced service is identified.
Spans: Breakdown of individual operations within a request.
Timings: Identify latency in specific services or operations.
Best Practices for Distributed Tracing
Instrument All Services: Ensure consistent tracing across all microservices.
Use Meaningful Span Names: Name spans based on operation types (e.g.,
HTTP GET /users
).Optimize Exporter Configuration: Adjust sampling rates to balance performance and trace detail.
Integrate Logging and Metrics: Combine tracing with logs and metrics for holistic observability.
Secure Trace Data: Mask sensitive data in spans and restrict access to trace backends.
Wrapping Up
Distributed tracing with OpenTelemetry is a game-changer for debugging and optimizing Node.js microservices. By implementing end-to-end tracing, you can gain deep insights into request flows, diagnose bottlenecks, and ensure reliable performance in production.
Start small with essential traces and gradually expand to cover all critical paths in your application. With OpenTelemetry, your microservices architecture will be more observable and resilient.
Happy tracing!
Subscribe to my newsletter
Read articles from Nicholas Diamond directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
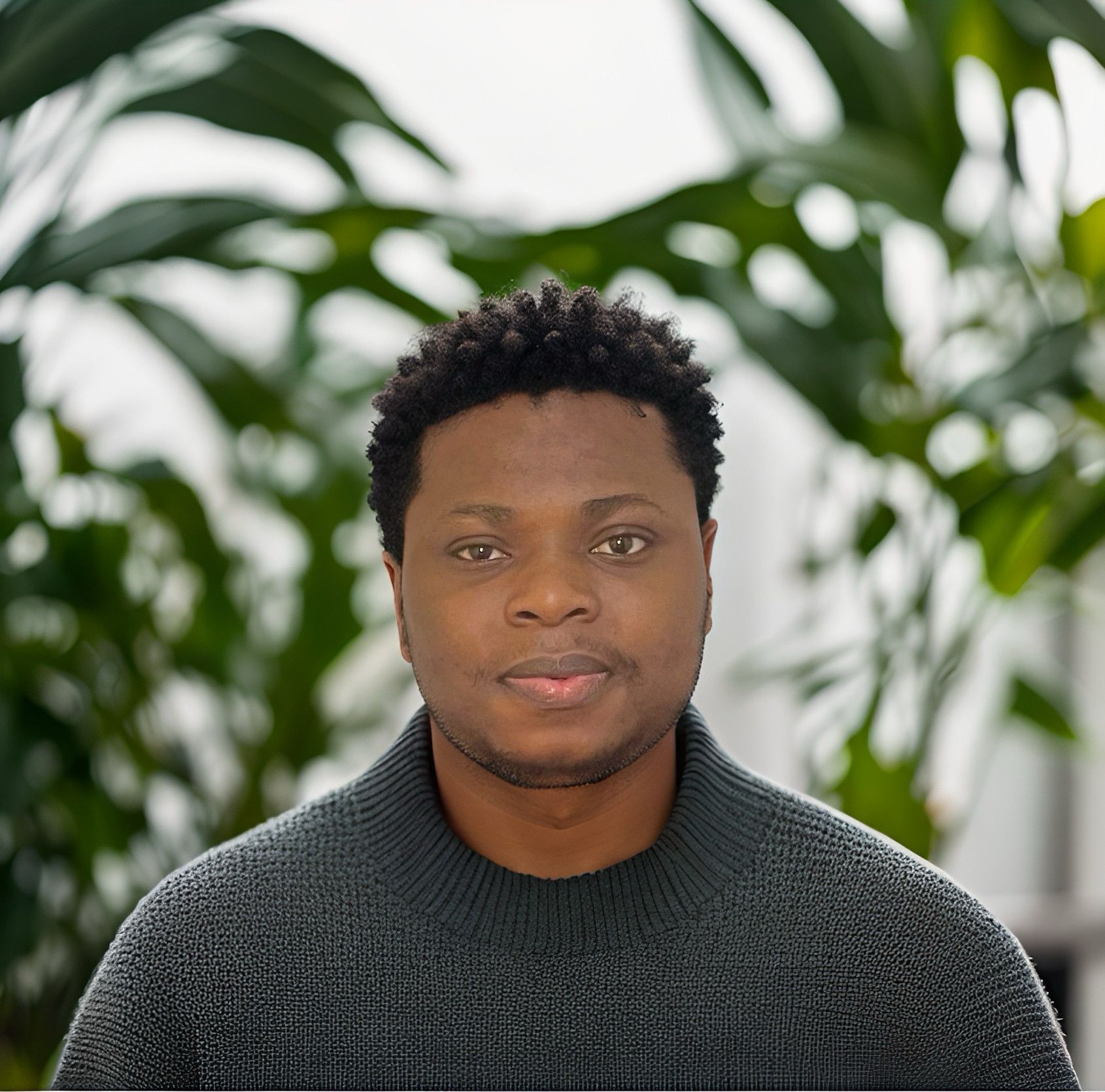