Hardening Node.js APIs Against Injection Attacks and Data Breaches
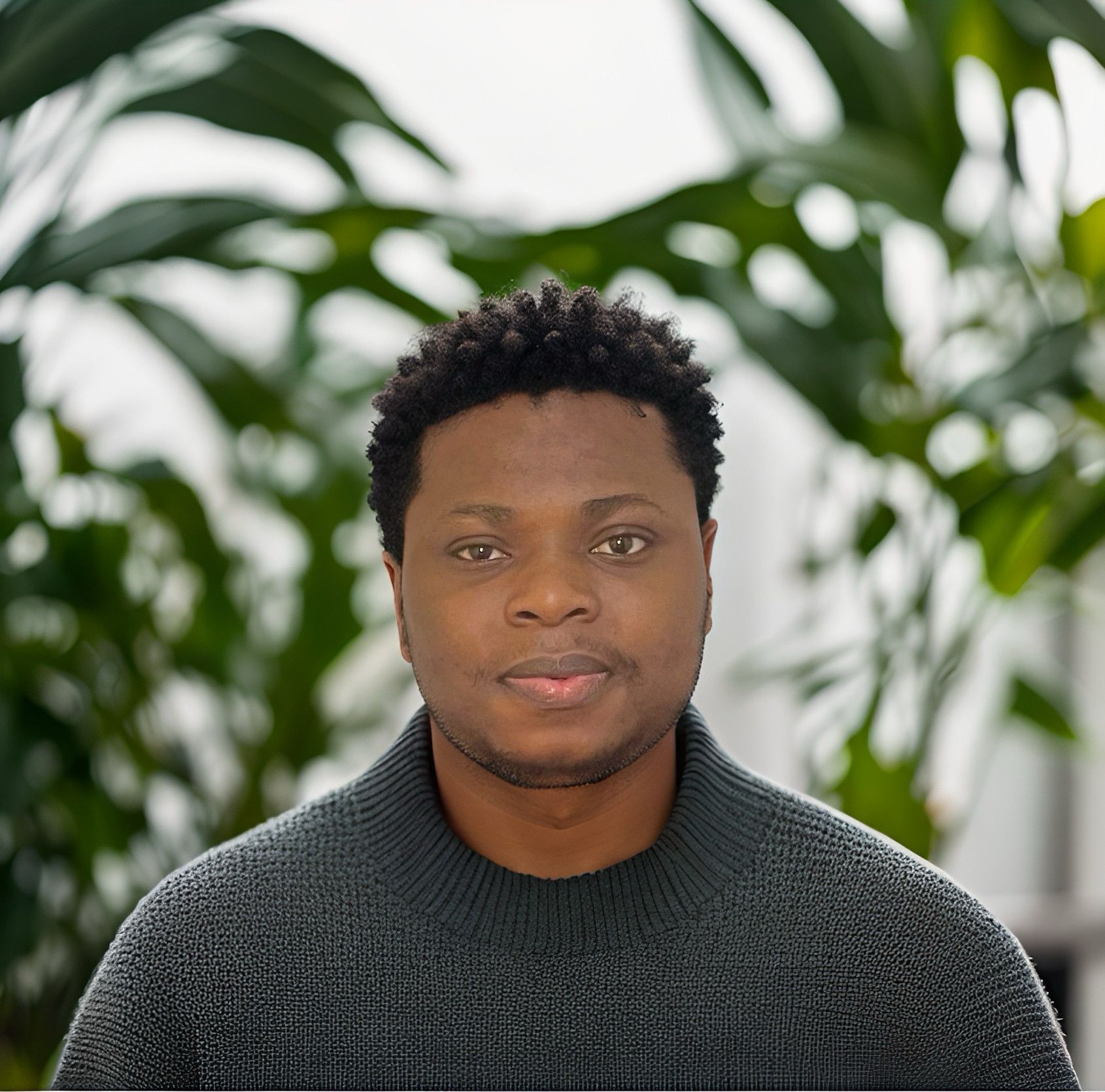
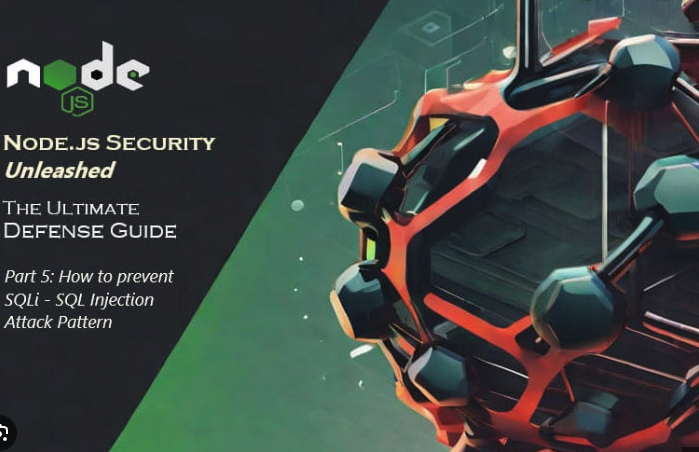
API security is a critical aspect of building modern applications, especially as the threat landscape continues to evolve. Among the most common attack vectors targeting APIs are injection attacks, such as SQL injection and NoSQL injection. These attacks can lead to significant data breaches, potentially exposing sensitive information and damaging your reputation.
In this article, we’ll guide you through the best practices to secure your Node.js APIs against injection attacks and data breaches. We’ll cover input validation, prepared statements, Content Security Policy (CSP), and rate limiting—essential techniques for preventing common vulnerabilities.
Understanding Injection Attacks
Injection attacks occur when an attacker can manipulate an application's input to execute malicious code or queries. Common injection attacks include:
SQL Injection (SQLi): Attackers inject SQL queries into API inputs that interact with relational databases, potentially allowing them to access, modify, or delete data.
NoSQL Injection: Similar to SQL injection but targeting NoSQL databases (e.g., MongoDB).
Command Injection: Injecting system commands through an API that interacts with the operating system.
These attacks exploit poor validation of user input, allowing malicious payloads to be executed on the backend.
Securing Node.js APIs: Best Practices
1. Input Validation
The first line of defense against injection attacks is validating input. By ensuring that all user inputs are properly sanitized and validated, you can prevent malicious data from reaching your database or system.
Key Steps for Effective Input Validation:
Whitelist Approach: Only accept known good values. For example, if a field expects a number, make sure only numeric input is allowed.
Regex Validation: Use regular expressions to enforce rules on input data, such as validating email formats, phone numbers, or credit card numbers.
Sanitization: Sanitize input to escape any characters that could be interpreted as executable code (e.g.,
<script>
tags,--
for SQL).
Example in Node.js:
const express = require('express');
const app = express();
app.use(express.json());
const validateUsername = (username) => {
// Only allow alphanumeric characters and underscores
const regex = /^[a-zA-Z0-9_]+$/;
return regex.test(username);
};
app.post('/user', (req, res) => {
const { username } = req.body;
if (!validateUsername(username)) {
return res.status(400).send('Invalid username');
}
// Proceed with the logic (e.g., save to the database)
res.send('User created');
});
app.listen(3000, () => console.log('API is running'));
2. Prepared Statements (for SQL and NoSQL Databases)
Prepared statements are a method to execute database queries in a safe manner by separating the SQL query structure from the input data. This prevents attackers from injecting arbitrary SQL or NoSQL commands.
SQL Prepared Statements (with MySQL/PostgreSQL):
const mysql = require('mysql');
const connection = mysql.createConnection({ /* connection details */ });
const username = req.body.username;
const query = 'SELECT * FROM users WHERE username = ?';
connection.query(query, [username], (error, results) => {
if (error) throw error;
res.send(results);
});
In this example, the ?
placeholder ensures that the input is treated as a value, not part of the SQL query.
NoSQL Prepared Statements (with MongoDB):
const mongoose = require('mongoose');
const User = mongoose.model('User', { username: String });
app.get('/user', async (req, res) => {
const username = req.query.username;
const user = await User.findOne({ username: username }); // Safe query
if (!user) {
return res.status(404).send('User not found');
}
res.send(user);
});
By using the appropriate query functions (e.g., findOne()
in MongoDB), you ensure that the input is treated safely.
3. Content Security Policy (CSP)
A Content Security Policy (CSP) helps prevent injection attacks, particularly Cross-Site Scripting (XSS). CSP allows you to specify which content sources are trusted, thus blocking malicious scripts from executing.
Setting up CSP in Express:
const helmet = require('helmet');
const app = express();
// Enable CSP to restrict content sources
app.use(helmet.contentSecurityPolicy({
directives: {
defaultSrc: ["'self'"],
scriptSrc: ["'self'", 'trusted-cdn.com'],
styleSrc: ["'self'", 'trusted-styles.com'],
},
}));
This configuration ensures that scripts and styles are only loaded from trusted sources, reducing the risk of malicious injections.
4. Rate Limiting
Rate limiting is an essential strategy for protecting APIs from abuse, including denial-of-service (DoS) attacks, brute force attacks, and excessive requests from a single user. By limiting the number of requests a user can make in a given timeframe, you can mitigate the impact of an injection attack.
Example using express-rate-limit
:
const rateLimit = require('express-rate-limit');
// Set up rate limiting to allow 100 requests per 15 minutes
const limiter = rateLimit({
windowMs: 15 * 60 * 1000,
max: 100,
message: 'Too many requests from this IP, please try again later.',
});
app.use(limiter); // Apply to all routes
This basic rate-limiting setup helps ensure that an attacker can't overload your API with requests, reducing the window for performing an injection attack.
5. Error Handling
Providing detailed error messages to users or attackers can expose sensitive information about your system, making it easier to exploit vulnerabilities. Ensure that your error messages are generic, especially in production environments.
app.use((err, req, res, next) => {
console.error(err.stack); // Log error for internal purposes
res.status(500).send('Something went wrong!');
});
This avoids exposing sensitive stack traces to the user and helps prevent attackers from gaining insight into your backend structure.
Additional Security Measures
Escape User Input: When dealing with dynamic queries or inserting user input directly into HTML, ensure that you escape user input to prevent XSS and other injection attacks.
Use HTTPS: Ensure that all API traffic is encrypted with HTTPS to prevent data interception and man-in-the-middle attacks.
Secure Your Database: Enforce least privilege access controls and regularly update database software to patch security vulnerabilities.
Conclusion
Protecting your Node.js APIs from injection attacks and data breaches is essential for building secure, scalable applications. By following the best practices outlined in this article—such as input validation, using prepared statements, setting up a strong Content Security Policy (CSP), and implementing rate limiting—you can significantly reduce the risk of injection attacks.
Security is an ongoing process, so it’s crucial to keep up with the latest threats and continuously audit your systems for potential vulnerabilities. With a well-secured API, your Node.js application will be more resilient against attackers and provide a safer experience for your users.
Subscribe to my newsletter
Read articles from Nicholas Diamond directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
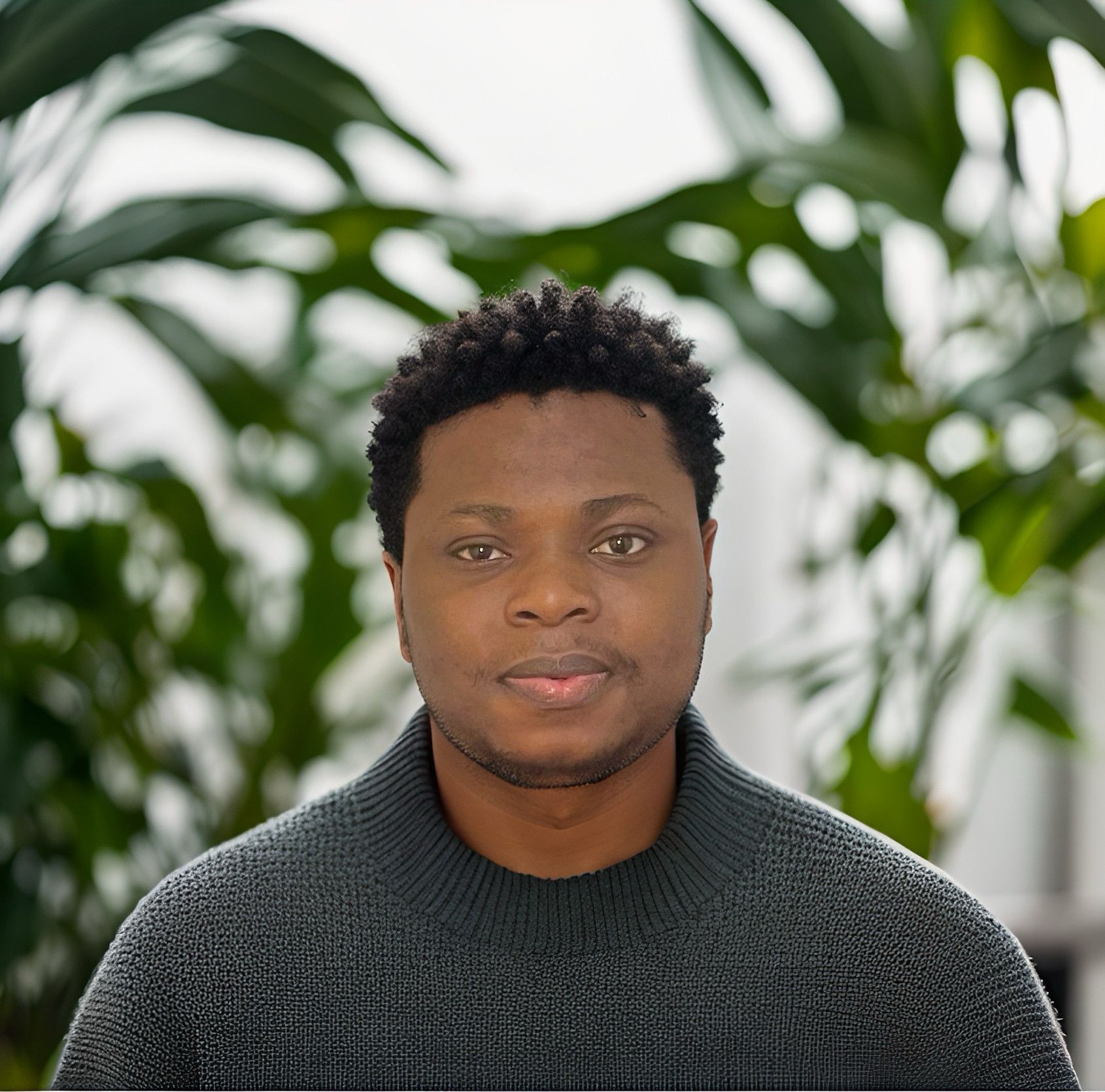