🚀 Transpiling and Polyfilling: Making Your JavaScript Universally Understood

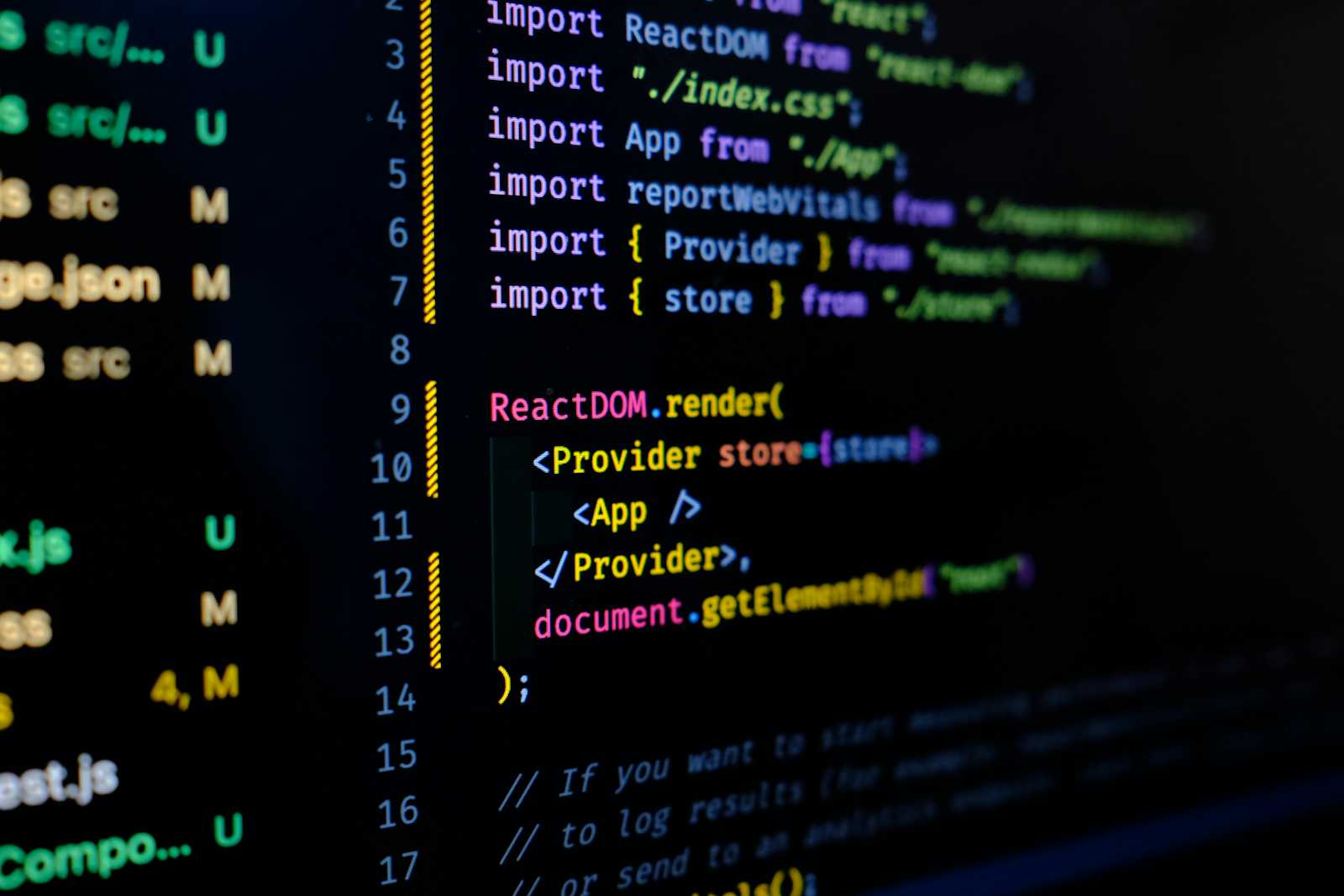
🚀 Transpiling and Polyfilling
Hello, fellow code wranglers and JavaScript adventurers! 👋 Today, we’re diving into two superheroes of modern web development: Transpiling and Polyfilling. These champions ensure your cutting-edge JavaScript works seamlessly even on those old, crusty browsers (looking at you, IE11 🫠).
Let’s make your code universally understood while keeping this read fun. Ready? Grab your favorite beverage, and let's dive in. ☕
🌍 Why Transpiling and Polyfilling Matter
You love using the latest JavaScript features, right? Fancy stuff like let
, const
, async/await
, and even Array.includes()
make your life easier. But some older browsers take one look at your code and panic.
Enter Transpiling and Polyfilling:
Transpiling: Converts your modern ES6+ code into older ES5 code. Think of it as rewriting your hip lingo so even your grandparents (aka old browsers) can understand.
Polyfilling: Adds the missing features (like
Promise
orincludes
) that old browsers didn’t get at birth.
Without these two, your sleek modern app might look like a 404 disaster in legacy browsers.
🛠️ Transpiling: Teaching Old Browsers New Syntax
Imagine talking to your grandparents in TikTok slang. They’ll nod politely but have no clue what you’re saying. Transpiling translates your fancy modern syntax into something old browsers can actually understand.
What is Transpiling?
Transpiling converts ES6+ (modern JavaScript) to ES5 (older JavaScript), making your code compatible with older browsers.
Example: Transpiling in Action
Let’s write some sleek ES6 code:
// ES6+
const greet = () => console.log("Hello, world!");
🚨 Old browsers: “Syntax error!” 😵
With transpiling, we convert it to ES5:
// ES5
var greet = function() {
console.log("Hello, world!");
};
👴 Old browsers: “Ah, yes. I understand this perfectly.”
Tools for Transpiling
The most popular tool for transpiling is Babel. Think of it as Google Translate but for JavaScript versions.
💡 How to Use Babel
Install Babel and presets:
npm install --save-dev @babel/core @babel/cli @babel/preset-env
Create a Babel config file (
babel.config.json
):{ "presets": ["@babel/preset-env"] }
Transpile your code:
npx babel src --out-dir dist
But wait! Using Parcel?
Good news: Parcel comes with Babel pre-configured out of the box! 🎉 That means you don’t need to install or configure Babel separately. Just write your ES6+ code, and Parcel handles the rest.
🔗 Learn more about Babel here.
🔗 Explore Parcel here.
🧩 Polyfilling: Giving Old Browsers New Gadgets
Now imagine your grandparents still rock flip phones. You get them a smartphone so they can FaceTime you. That’s polyfilling! It gives old browsers new abilities they didn’t originally support.
What is Polyfilling?
Polyfilling adds code to provide missing features, like Promise
, async/await
, or Array.includes()
, in browsers that don’t support them.
Example: Polyfilling in Action
Here’s some modern ES6+ code:
let numbers = [1, 2, 3];
console.log(numbers.includes(2)); // true
🚨 Old browsers: “What’s an includes
?!”
With a polyfill, we can add the missing includes
feature:
if (!Array.prototype.includes) {
Array.prototype.includes = function(value) {
return this.indexOf(value) !== -1;
};
}
👴 Old browsers: “Ah, I can do this now!”
Tools for Polyfilling
Two tools you’ll use a lot:
Core-js – For polyfilling ES6+ features.
Regenerator-runtime – For polyfilling
async/await
and generator functions.
💡 How to Use Them Together
Install Core-js and Regenerator-runtime:
npm install core-js regenerator-runtime
Import them in your code:
import 'core-js/stable'; import 'regenerator-runtime/runtime';
Why both?
Core-js adds missing ES6+ methods like
Array.includes
.Regenerator-runtime adds support for
async/await
and generators.
🔗 Core-js GitHub
🔗 Regenerator-runtime GitHub
🎉 Transpiling + Polyfilling = Compatibility Superpowers
With both tools in your arsenal:
Transpiling ensures your code syntax is old-browser-friendly.
Polyfilling ensures all modern features work, even if the browser never had them natively.
Let’s make your web app accessible to everyone, from the coolest new browsers to the ancient ones.
🤓 Live Examples
Transpiling Example
<script src="https://cdnjs.cloudflare.com/ajax/libs/babel-standalone/7.20.5/babel.min.js"></script>
<script type="text/babel">
const greet = () => console.log("Hello, ES6!");
greet();
</script>
Polyfilling Example
<script>
// Polyfill for Array.includes
if (!Array.prototype.includes) {
Array.prototype.includes = function(value) {
return this.indexOf(value) !== -1;
};
}
console.log([1, 2, 3].includes(2)); // Works everywhere!
</script>
🤔 Final Thoughts
Transpiling and polyfilling might sound intimidating at first, but trust me, they’re your best friends for making your web app a universal hit. They ensure that whether someone’s using the latest Chrome or an ancient version of IE, your app will still work like a charm.
So, next time you’re coding, don’t forget to give old browsers a helping hand. Your users (and their browsers) will thank you. 🙌
Happy coding! 🎉
Resources to Explore
Got questions or funny browser horror stories? Drop them in the comments below!
Subscribe to my newsletter
Read articles from Oohnohassani directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Oohnohassani
Oohnohassani
Hi 👋 I'm a developer with experience in building websites with beautiful designs and user friendly interfaces. I'm experienced in front-end development using languages such as Html5, Css3, TailwindCss, Javascript, Typescript, react.js & redux and NextJs 🚀