How to Run a .NET Project Manually and in Docker

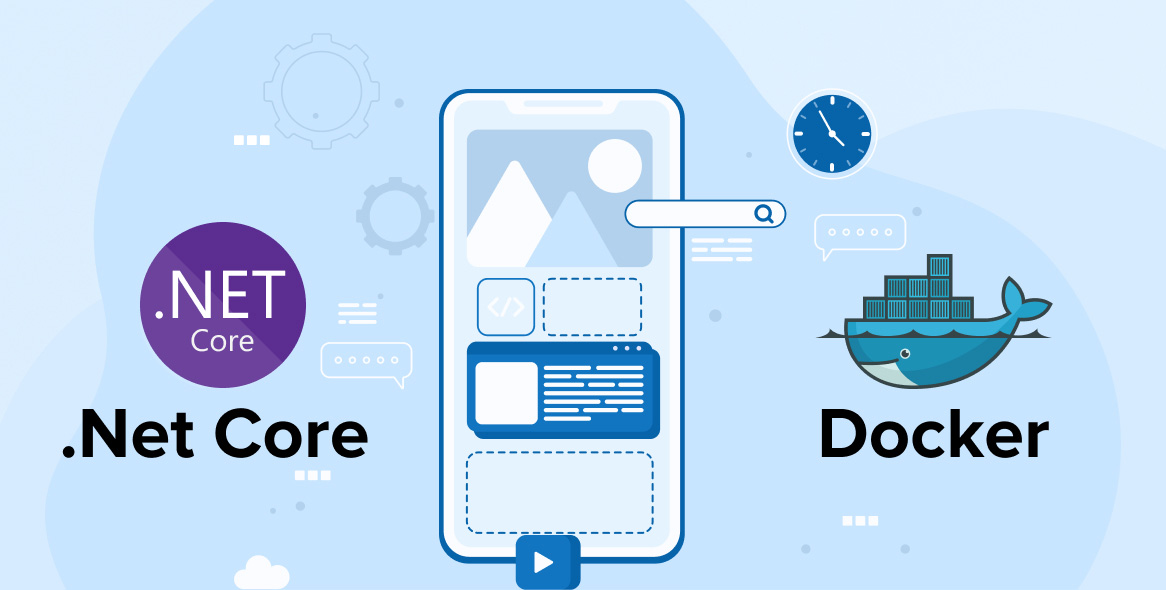
In this guide, we’ll walk through two methods to run a .NET 6.0 application:
Running the application manually on your local machine.
Using Docker to containerize and run the application efficiently.
By the end, you’ll have a clear understanding of how to set up and run the application in both environments.
Part 1: Running the Application Manually
Running a .NET application manually involves installing necessary tools, building the project, and running it locally. Let’s break this down step-by-step.
Step 1: Install Prerequisites
Ensure you have the following installed:
.NET SDK 6.0 to build and run the application.
A terminal or command prompt (e.g., PowerShell, Bash).
Step 2: Navigate to the Project Directory
Assume the project structure is as follows:
QRCode_CoreMvc/
├── QRCode_UI/
│ ├── QRCode_UI.csproj
├── QRCode_CoreMvc.sln
├── Dockerfile
Navigate to the project directory:
cd QRCode_CoreMvc
Step 3: Restore Dependencies
Run the following command to restore the project dependencies:
dotnet restore
Explanation:
This command:
Downloads the required NuGet packages.
Resolves dependencies defined in the
.csproj
file.
Step 4: Build the Project
Compile the project using:
dotnet build
Explanation:
This step:
Compiles the source code.
Generates binaries for your application.
Step 5: Publish the Application
Prepare the application for deployment:
dotnet publish -c Release -o ./publish
Explanation:
This command:
Builds the application (if not already built).
Packages all necessary files into the
./publish
directory.
Step 6: Run the Application
Navigate to the publish
directory and start the application:cd publish
dotnet QRCode_UI.dll
Explanation:
This starts the .NET runtime to execute the application.
Step 7: Access the Application
Open a browser and visit the following URLs to access the application:
http://localhost:5000
(HTTP)http://localhost:5001
(HTTPS)
You’ve successfully run the application manually!
Part 2: Running the Application in Docker
Now let’s containerize the application using Docker. This approach ensures portability and simplifies deployment across different environments.
Dockerfile Overview
The Dockerfile
defines how the application is built and run in a container. Here’s the Dockerfile
we’ll use:
# Stage 1: Build the application
FROM mcr.microsoft.com/dotnet/sdk:6.0 AS build-env
WORKDIR /app
# Restore dependencies
COPY *.sln ./
COPY QRCode_UI/*.csproj ./QRCode_UI/
RUN dotnet restore
# Build and publish the application
COPY . ./
WORKDIR /app/QRCode_UI
RUN dotnet publish -c Release -o /out
# Stage 2: Run the application
FROM mcr.microsoft.com/dotnet/aspnet:6.0
WORKDIR /app
# Copy the published output from the build stage
COPY --from=build-env /out .
# Expose the ports used by the application
EXPOSE 5000
EXPOSE 5001
# Configure the application to listen on all network interfaces
ENV ASPNETCORE_URLS="http://0.0.0.0:5000;http://0.0.0.0:5001"
# Run the application
ENTRYPOINT ["dotnet", "QRCode_UI.dll"]
This Dockerfile has two stages:
Build Stage: Compiles and publishes the application.
Runtime Stage: Runs the published application in a lightweight container.
Step 1: Build the Docker Image
Run the following command in the directory containing the Dockerfile
:
docker build -t my-dotnet-app .
Explanation:
docker build
: Builds a Docker image.-t my-dotnet-app
: Tags the image asmy-dotnet-app
..
: Specifies the current directory as the build context.
Step 2: Run the Docker Container
Start a container from the built image:
docker run -d -p 5000:5000 -p 5001:5001 --name my-dotnet-container my-dotnet-app
Explanation:
docker run
: Creates and starts a container.-d
: Runs the container in detached mode (in the background).-p 5000:5000 -p 5001:5001
: Maps container ports to your machine.--name my-dotnet-container
: Names the containermy-dotnet-container
.my-dotnet-app
: Specifies the image to use.
Step 3: Access the Application
Open a browser and navigate to:
http://localhost:5000
https://localhost:5001
The application is now running inside a Docker container!
Step 4: Manage the Container
To view the running containers:
docker ps
To stop the container:
docker stop my-dotnet-container
To remove the container:
docker rm my-dotnet-container
To delete the image:
docker rmi my-dotnet-app
Conclusion
We’ve demonstrated how to run a .NET application both manually and using Docker. The manual approach is straightforward for development and testing, while Docker simplifies deployment and ensures portability.
Key Benefits of Docker:
Consistency across environments.
Easy scaling and deployment.
With this knowledge, you can confidently run .NET applications in any environment. Happy coding!
Additional Resources:
Docker Documentation
This blog provides a complete and well-structured guide for both manual and Docker-based application deployment.
Subscribe to my newsletter
Read articles from Muhammad Hassan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Muhammad Hassan
Muhammad Hassan
Hey there! I'm currently working as an Associate DevOps Engineer, and I'm diving into popular DevOps tools like Azure Devops,Linux, Docker, Kubernetes,Terraform and Ansible. I'm also on the learning track with AWS certifications to amp up my cloud game. If you're into tech collaborations and exploring new horizons, let's connect!