Using OpenAPI/Swagger Documentation for Node.js APIs
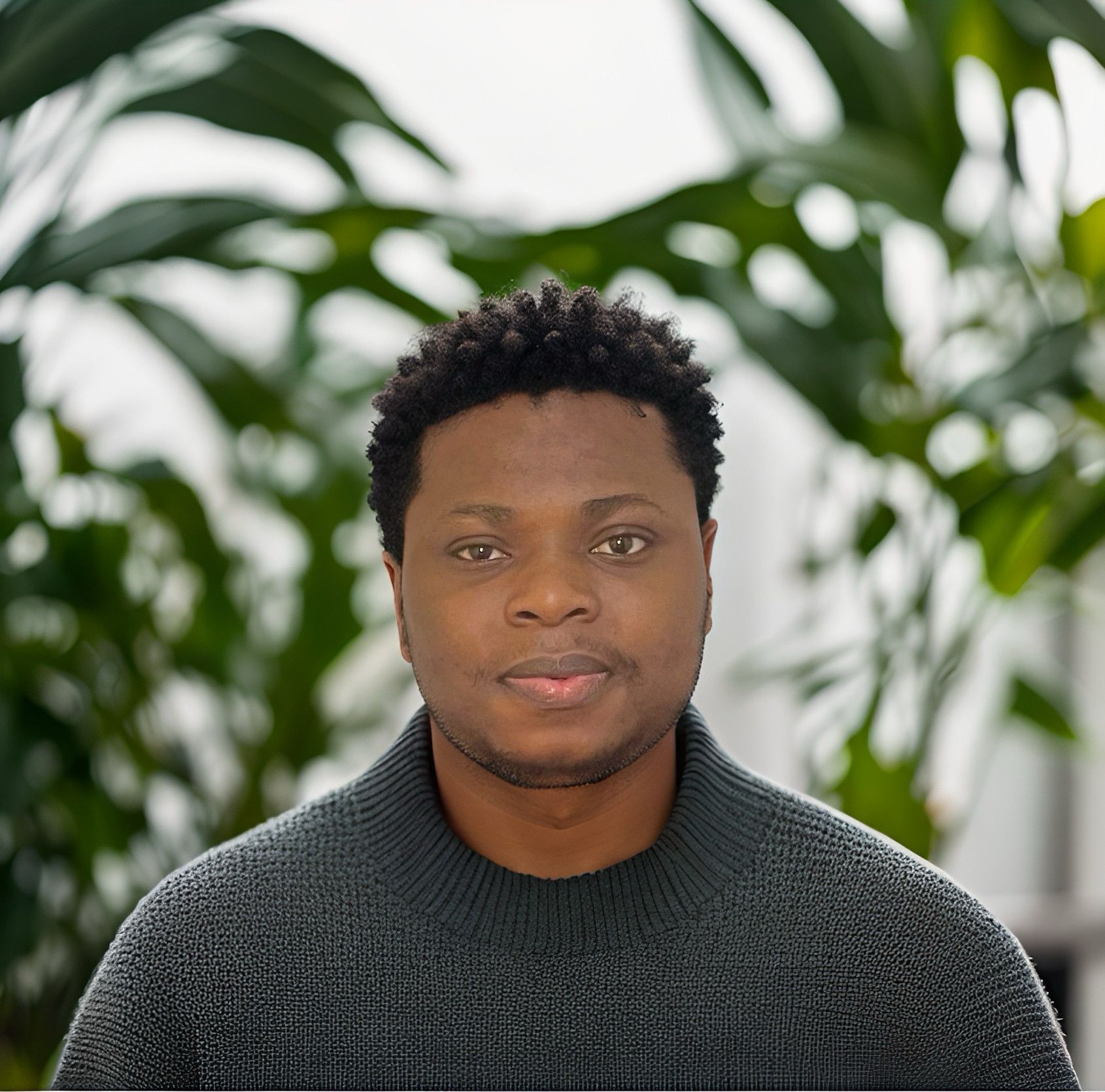
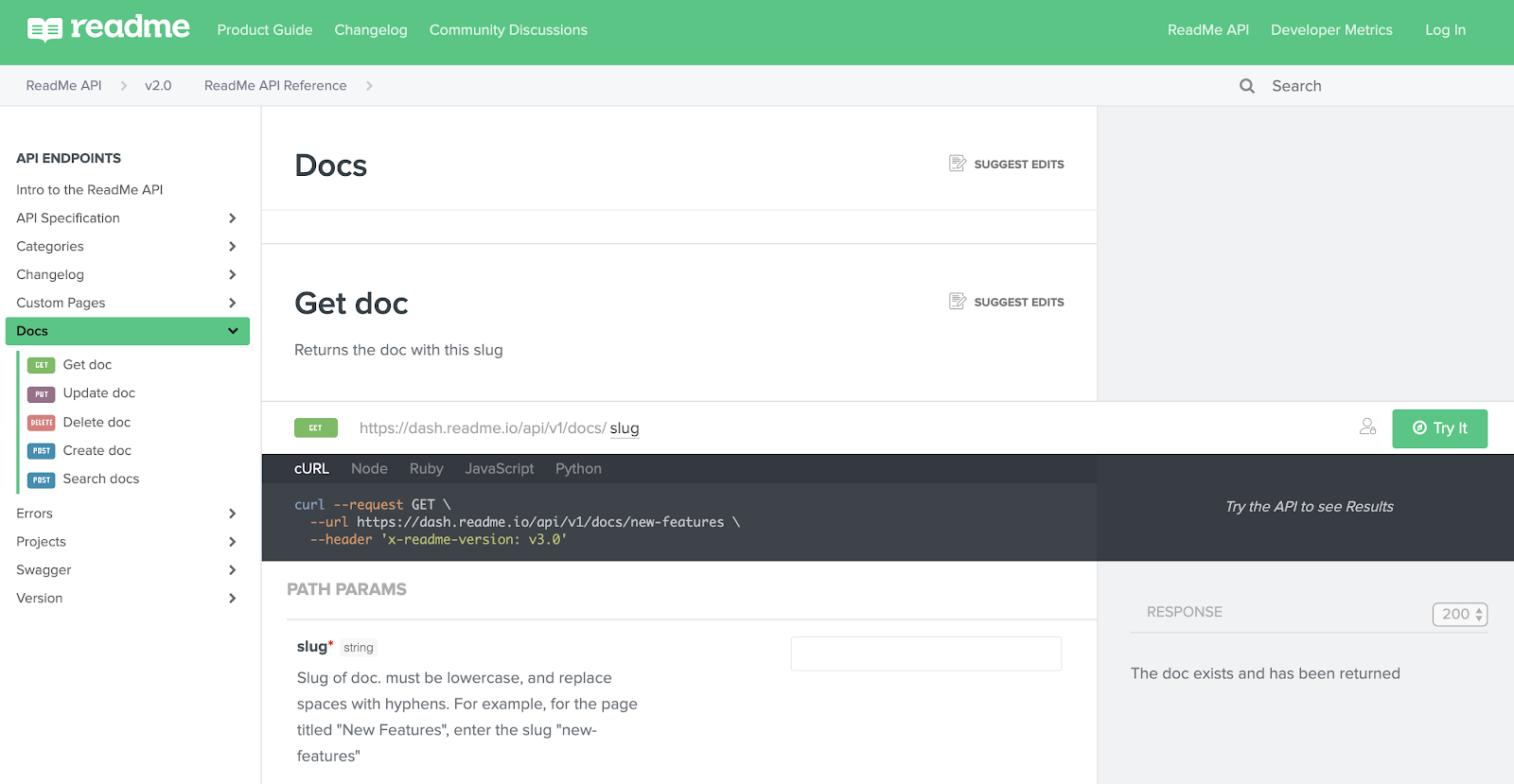
API documentation is a critical part of any modern backend application, and for developers building APIs with Node.js, ensuring that your API is well-documented can vastly improve collaboration with your team and consumption by clients. Clear, up-to-date documentation helps prevent misunderstandings, reduces integration errors, and ultimately leads to better user and developer experiences.
In this article, we’ll explore how to generate dynamic API documentation for Node.js applications using OpenAPI (formerly known as Swagger), focusing on tools like swagger-jsdoc
and swagger-ui-express
. We’ll also discuss how to version and test your API documentation to keep it accurate as your project evolves.
What is OpenAPI (Swagger)?
OpenAPI is a specification for describing and documenting RESTful APIs. It provides a standard format for defining your API endpoints, input parameters, responses, and authentication mechanisms. Swagger is a set of tools that helps you generate, document, and test OpenAPI specifications.
The combination of OpenAPI and Swagger offers:
Auto-generated documentation: Automatically generates detailed API documentation.
Interactive UI: Provides an interactive, user-friendly interface for developers to test API endpoints.
Standardized format: Ensures your API documentation adheres to an industry-standard format, making it easier for others to understand and integrate.
In this guide, we’ll walk through setting up OpenAPI/Swagger documentation for a Node.js API, using the swagger-jsdoc
package to generate an OpenAPI specification and swagger-ui-express
to render it in a user-friendly interface.
Prerequisites
Before we dive into the setup, ensure you have the following:
Node.js installed on your machine.
A Node.js application with Express set up.
Basic understanding of RESTful APIs and JavaScript/Node.js.
If you don’t have a Node.js app set up yet, you can create one using express-generator
or manually by installing express
.
npm init -y
npm install express
Step 1: Install the Required Packages
To begin, install swagger-jsdoc
and swagger-ui-express
:
npm install swagger-jsdoc swagger-ui-express
swagger-jsdoc: This package helps you generate Swagger-compatible API documentation from JSDoc comments in your code.
swagger-ui-express: This package allows you to serve the Swagger UI in your application, so users can interact with the API directly.
Step 2: Set Up swagger-jsdoc
Now, let’s configure swagger-jsdoc
to generate the OpenAPI specification for your Node.js API.
- Create a
swagger.js
file in your project to configureswagger-jsdoc
andswagger-ui-express
:
// swagger.js
const swaggerJsDoc = require('swagger-jsdoc');
const swaggerUi = require('swagger-ui-express');
const options = {
definition: {
openapi: '3.0.0',
info: {
title: 'My API',
version: '1.0.0',
description: 'This is an API for demonstrating Swagger with Node.js and Express.',
},
},
apis: ['./routes/*.js'], // Specify the path where your route files are located
};
const swaggerSpec = swaggerJsDoc(options);
module.exports = { swaggerUi, swaggerSpec };
In this configuration:
The
openapi
version is set to3.0.0
, which is the current version of the OpenAPI specification.The
apis
field points to the directory containing your route files (in this case,./routes/*.js
).
Step 3: Documenting Your API Endpoints
In your route files (for example, routes/user.js
), use JSDoc comments to document each endpoint. Here’s an example:
// routes/user.js
const express = require('express');
const router = express.Router();
/**
* @swagger
* /users:
* get:
* description: Get all users
* responses:
* 200:
* description: A list of users
* content:
* application/json:
* schema:
* type: array
* items:
* type: object
* properties:
* id:
* type: integer
* name:
* type: string
*/
router.get('/users', (req, res) => {
res.status(200).json([
{ id: 1, name: 'John Doe' },
{ id: 2, name: 'Jane Smith' }
]);
});
module.exports = router;
Here, we’ve added a Swagger-specific JSDoc comment (@swagger
) above the /users
endpoint:
description: Describes what the endpoint does.
responses: Details the possible responses, including the status code and response body schema.
You can continue documenting all your routes similarly by specifying HTTP methods (GET
, POST
, etc.), parameters, request bodies, and responses.
Step 4: Serve the Swagger UI
Next, let’s set up Swagger UI so we can view and interact with the API documentation.
Modify your app.js
file to integrate Swagger UI:
// app.js
const express = require('express');
const app = express();
const { swaggerUi, swaggerSpec } = require('./swagger');
const userRoutes = require('./routes/user');
// Serve API documentation
app.use('/api-docs', swaggerUi.serve, swaggerUi.setup(swaggerSpec));
// Use your routes
app.use('/users', userRoutes);
app.listen(3000, () => {
console.log('Server is running on http://localhost:3000');
console.log('API docs available at http://localhost:3000/api-docs');
});
Here, we:
Serve the Swagger UI at the
/api-docs
endpoint.The
swaggerUi.setup(swaggerSpec)
call uses the OpenAPI spec generated byswagger-jsdoc
to populate the Swagger UI with documentation.
Now, you can navigate to http://localhost:3000/api-docs
in your browser to view the interactive API documentation.
Step 5: Best Practices for API Documentation
1. Versioning:
API versioning is important for ensuring backward compatibility. You can document versioning in your OpenAPI definition like this:
openapi: 3.0.0
info:
title: My API
version: 1.0.0
As your API evolves, make sure to update the version in both the info
section of the spec and in the route URLs.
2. Authentication:
If your API uses authentication, ensure that it is documented clearly in the OpenAPI spec. Here’s an example for Bearer token authentication:
components:
securitySchemes:
BearerAuth:
type: http
scheme: bearer
bearerFormat: JWT
security:
- BearerAuth: []
This will ensure that your users know how to authenticate their requests.
3. Testing:
Use the Swagger UI to manually test your API by clicking through the endpoints. This makes it easier to verify that the API documentation matches the actual implementation.
Conclusion
Generating and maintaining API documentation for your Node.js application with OpenAPI and Swagger is a powerful practice for ensuring transparency and improving collaboration. With tools like swagger-jsdoc
and swagger-ui-express
, you can automate the process of generating interactive API documentation that can be easily consumed by both developers and clients.
By following the steps outlined in this article, you can set up robust, user-friendly API documentation that scales with your application and remains consistent as your API evolves. Don’t forget to version your API documentation and test it regularly to ensure it remains accurate.
Happy coding, and happy documenting!
Subscribe to my newsletter
Read articles from Nicholas Diamond directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
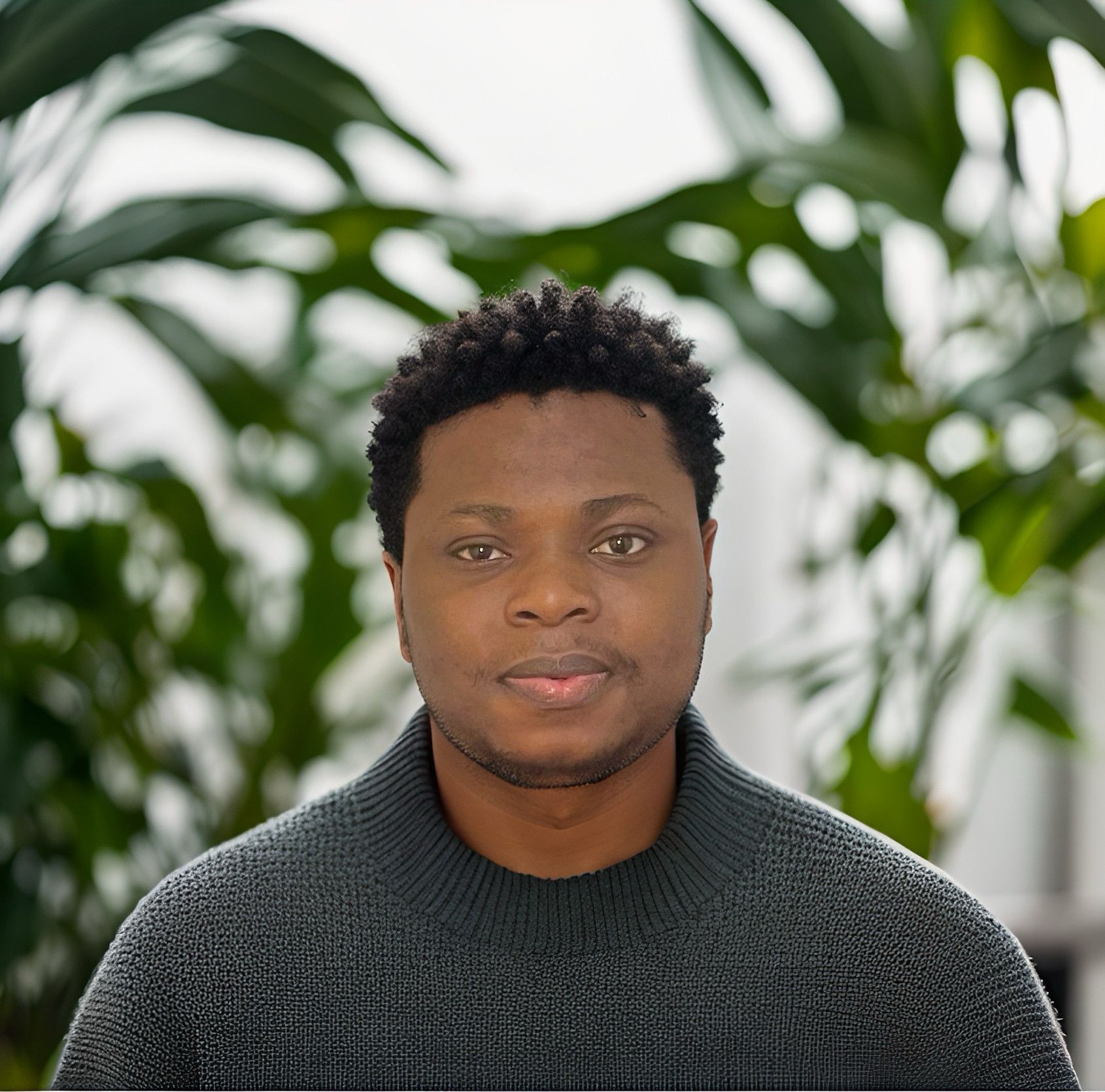