Fixing Common SSL/TLS Errors in Node.js Applications
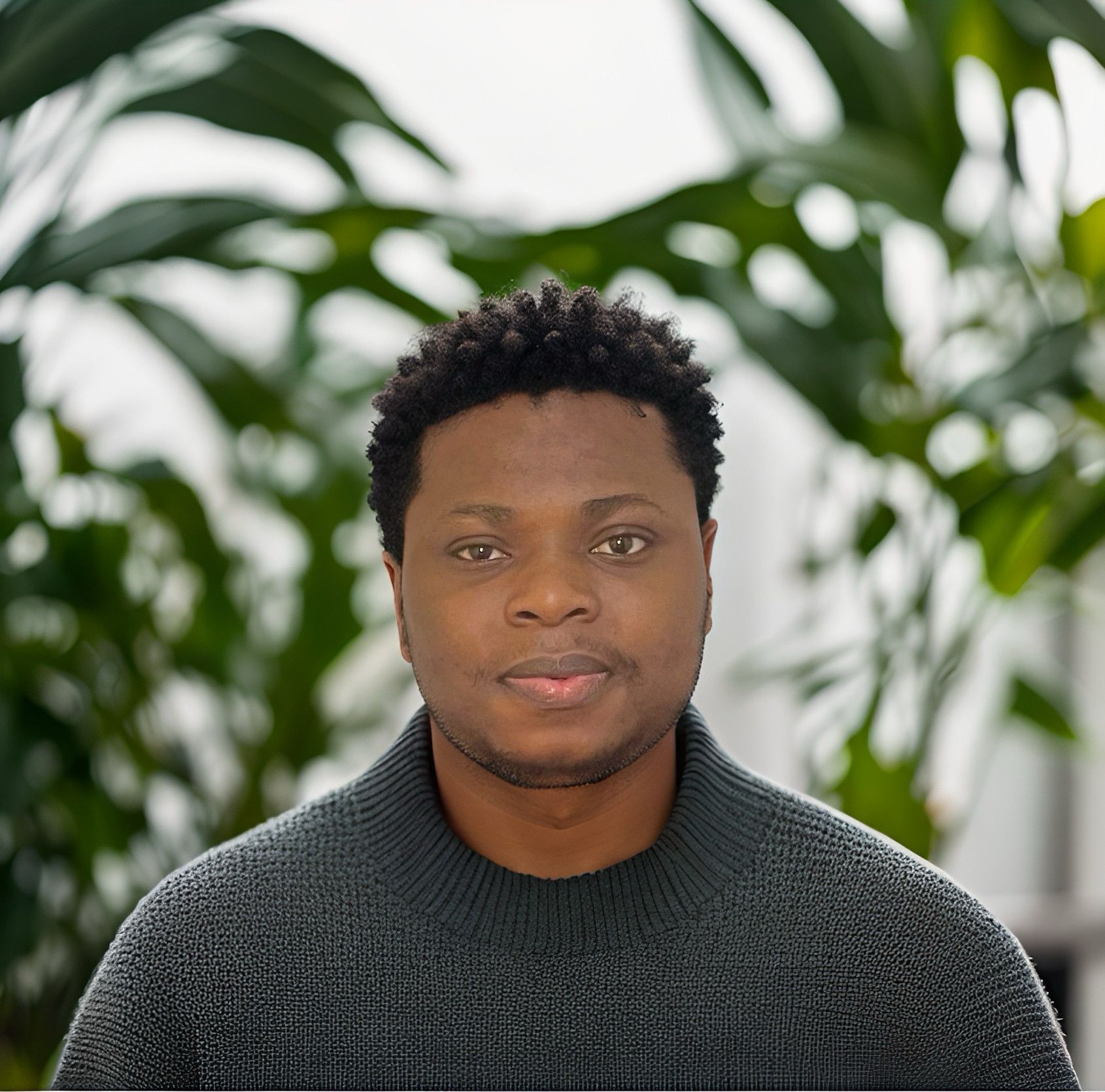
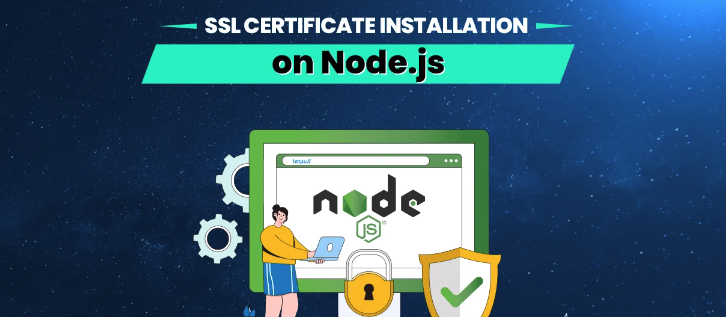
As web security becomes more critical, SSL/TLS (Secure Sockets Layer / Transport Layer Security) is a foundational protocol for ensuring encrypted communication between servers and clients. When building secure Node.js applications, you may encounter SSL/TLS issues, such as self-signed certificate errors, expired certificates, or misconfigured HTTPS setups. These errors can lead to serious security vulnerabilities or connection failures, making it crucial to handle them effectively.
In this article, we'll walk through some of the most common SSL/TLS issues in Node.js applications, how to debug them, and the best practices for correctly configuring HTTPS and managing SSL certificates.
Common SSL/TLS Errors in Node.js
Before diving into the solutions, let’s first take a look at some of the most frequent SSL/TLS errors developers encounter when building Node.js applications:
Self-Signed Certificate Errors:
- When your Node.js application uses a self-signed certificate, it’s not trusted by default because it hasn’t been verified by a trusted Certificate Authority (CA). Browsers and clients will flag this as an insecure connection.
Expired Certificate Errors:
- SSL certificates have an expiration date. If your certificate expires, users will receive warnings about an insecure connection. Expired certificates need to be renewed or replaced before your application can be accessed securely.
Certificate Chain Issues:
- Sometimes, intermediate certificates are missing in the certificate chain, causing Node.js or browsers to not trust the server’s certificate.
Invalid Certificate Hostname:
- If the domain name in the SSL certificate doesn’t match the domain name of the server, Node.js will throw an error.
Incorrect HTTPS Configuration:
- If HTTPS is misconfigured (e.g., not using the proper certificates or setting insecure cipher suites), your application can be vulnerable to attacks or won’t serve over HTTPS at all.
Debugging SSL/TLS Errors in Node.js
Step 1: Understanding How Node.js Handles SSL/TLS
In Node.js, HTTPS is typically configured using the https
module. When setting up an HTTPS server, you need to provide SSL certificates—a public certificate (.crt
file) and a private key (.key
file). Here’s a basic example of how to configure HTTPS in a Node.js server:
const https = require('https');
const fs = require('fs');
const options = {
key: fs.readFileSync('path/to/private.key'),
cert: fs.readFileSync('path/to/certificate.crt'),
ca: fs.readFileSync('path/to/chainfile.pem') // For intermediate certificates
};
https.createServer(options, (req, res) => {
res.writeHead(200);
res.end('Hello, secure world!');
}).listen(443, () => {
console.log('HTTPS server running on https://localhost');
});
Step 2: Using OpenSSL to Debug Certificates
One of the most useful tools for diagnosing SSL/TLS errors is OpenSSL. OpenSSL allows you to inspect your certificates, check their validity, and troubleshoot common errors.
Check Certificate Expiry:
To check if your certificate has expired, run the following command in your terminal:
openssl x509 -enddate -noout -in path/to/certificate.crt
This will print the expiration date of your certificate.
Verify the Certificate Chain:
If there are certificate chain issues (missing intermediate certificates), use this command:
openssl s_client -connect yourdomain.com:443
This will provide details about the certificate chain, and you’ll be able to see if the server is sending all the necessary certificates.
Solutions to Common SSL/TLS Errors
1. Fixing Self-Signed Certificate Errors
Self-signed certificates are not trusted by default because they haven't been signed by a trusted Certificate Authority (CA). To fix this error:
For Development/Testing: You can ignore SSL validation (not recommended for production environments). In Node.js, you can use the
rejectUnauthorized: false
option in yourhttps
request configuration. However, this is not secure and should only be used for local development.Example:
const https = require('https'); const options = { hostname: 'localhost', port: 443, path: '/', method: 'GET', rejectUnauthorized: false // Disable SSL validation }; https.request(options, (res) => { console.log('Response status code:', res.statusCode); }).end();
For Production: Ensure that your certificate is signed by a trusted CA. You can use services like Let's Encrypt to obtain free, valid SSL certificates. Alternatively, you can buy SSL certificates from providers like DigiCert, GoDaddy, or Comodo.
2. Resolving Expired Certificate Errors
When your SSL certificate expires, you need to renew it. Follow these steps:
Renew the Certificate: Contact your Certificate Authority (CA) or use tools like Let’s Encrypt to automatically renew certificates.
Update Your Certificate Files: Replace the expired certificate files in your Node.js application with the new ones.
3. Fixing Certificate Chain Issues
If the certificate chain is incomplete (e.g., missing intermediate certificates), you may encounter errors like "unable to verify the first certificate."
Include Intermediate Certificates: Make sure that your certificate chain is complete by including intermediate certificates in the
ca
option when configuring the HTTPS server in Node.js.Example:
const options = { key: fs.readFileSync('path/to/private.key'), cert: fs.readFileSync('path/to/certificate.crt'), ca: fs.readFileSync('path/to/intermediate-cert.pem') // Add intermediate certs };
Verify the Certificate Chain: Use
openssl s_client
to check that the server is sending the full certificate chain, including intermediate certificates.
4. Handling Invalid Hostname Errors
An "invalid certificate" error often occurs when the hostname in the certificate doesn't match the requested domain. To fix this:
Ensure Matching Domain: Double-check that the domain on the certificate matches the domain your Node.js server is serving. For example, if your server is hosted at
www.example.com
, your certificate should be issued towww.example.com
and not justexample.com
.Wildcard Certificates: If you are using subdomains, a wildcard certificate (e.g.,
*.
example.com
) can be used.
5. Correct HTTPS Configuration
Ensure that your Node.js server is properly configured to handle SSL connections:
Use Strong Cipher Suites: Configure your server to only accept strong encryption ciphers to avoid vulnerabilities.
Example using
tls
options in Node.js:const options = { key: fs.readFileSync('path/to/private.key'), cert: fs.readFileSync('path/to/certificate.crt'), ciphers: 'ECDHE-ECDSA-AES128-GCM-SHA256', // Use strong ciphers honorCipherOrder: true };
Set Up Automatic Certificate Renewal: If you’re using Let’s Encrypt, you can set up automatic certificate renewal with tools like Certbot.
Conclusion
SSL/TLS issues are common in Node.js applications, but with the right tools and practices, they can be quickly identified and resolved. OpenSSL is an invaluable tool for debugging certificates, and proper configuration of your SSL/TLS setup is crucial for maintaining secure communication between clients and servers.
By addressing common SSL/TLS errors such as self-signed certificate issues, expired certificates, and certificate chain problems, you can ensure that your Node.js application is running securely in production environments. Always prioritize using trusted certificates, handle HTTPS properly, and make sure to keep your certificates up to date to avoid potential security risks.
Subscribe to my newsletter
Read articles from Nicholas Diamond directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
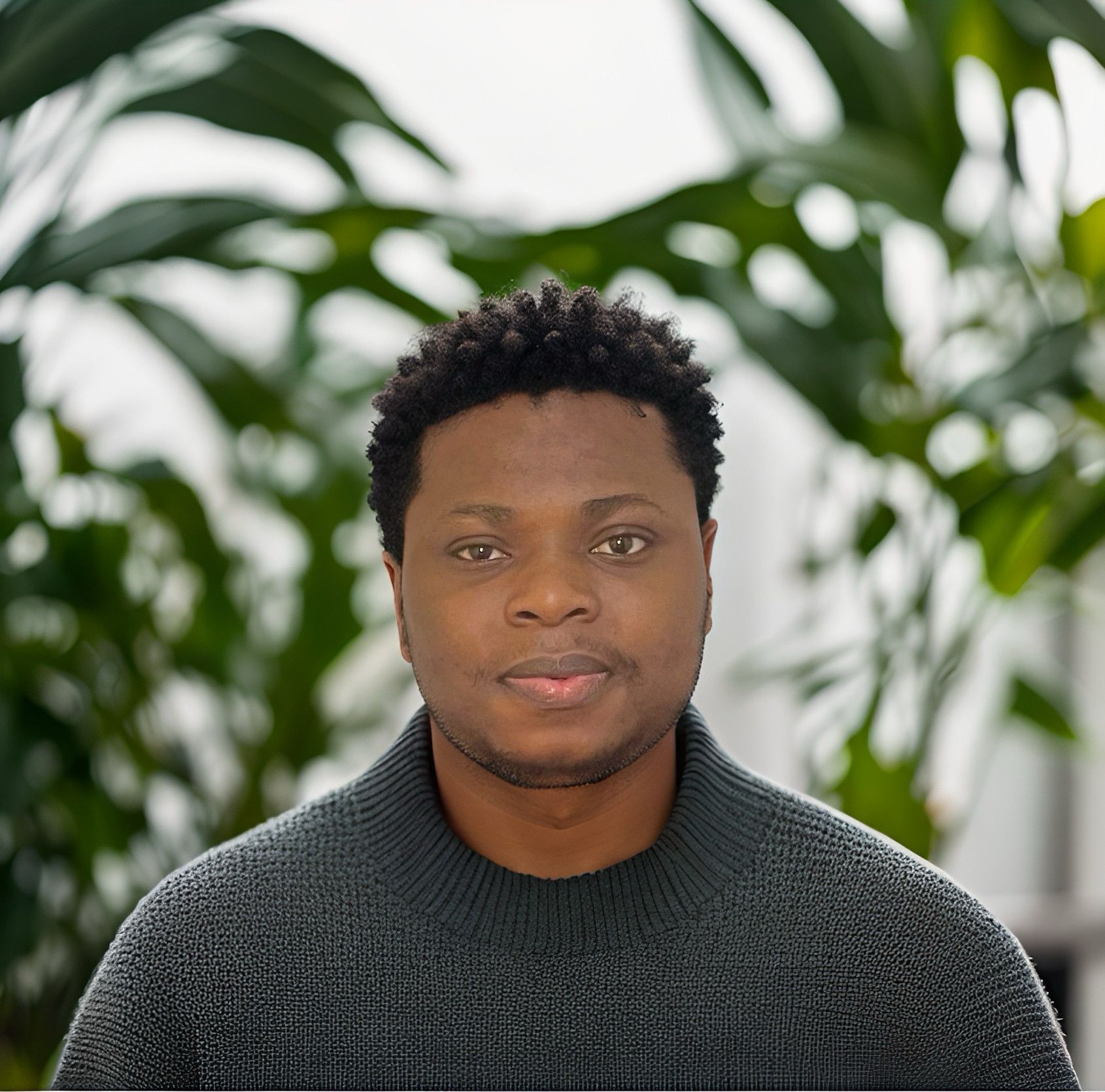