Fixing CORS Issues in Node.js APIs
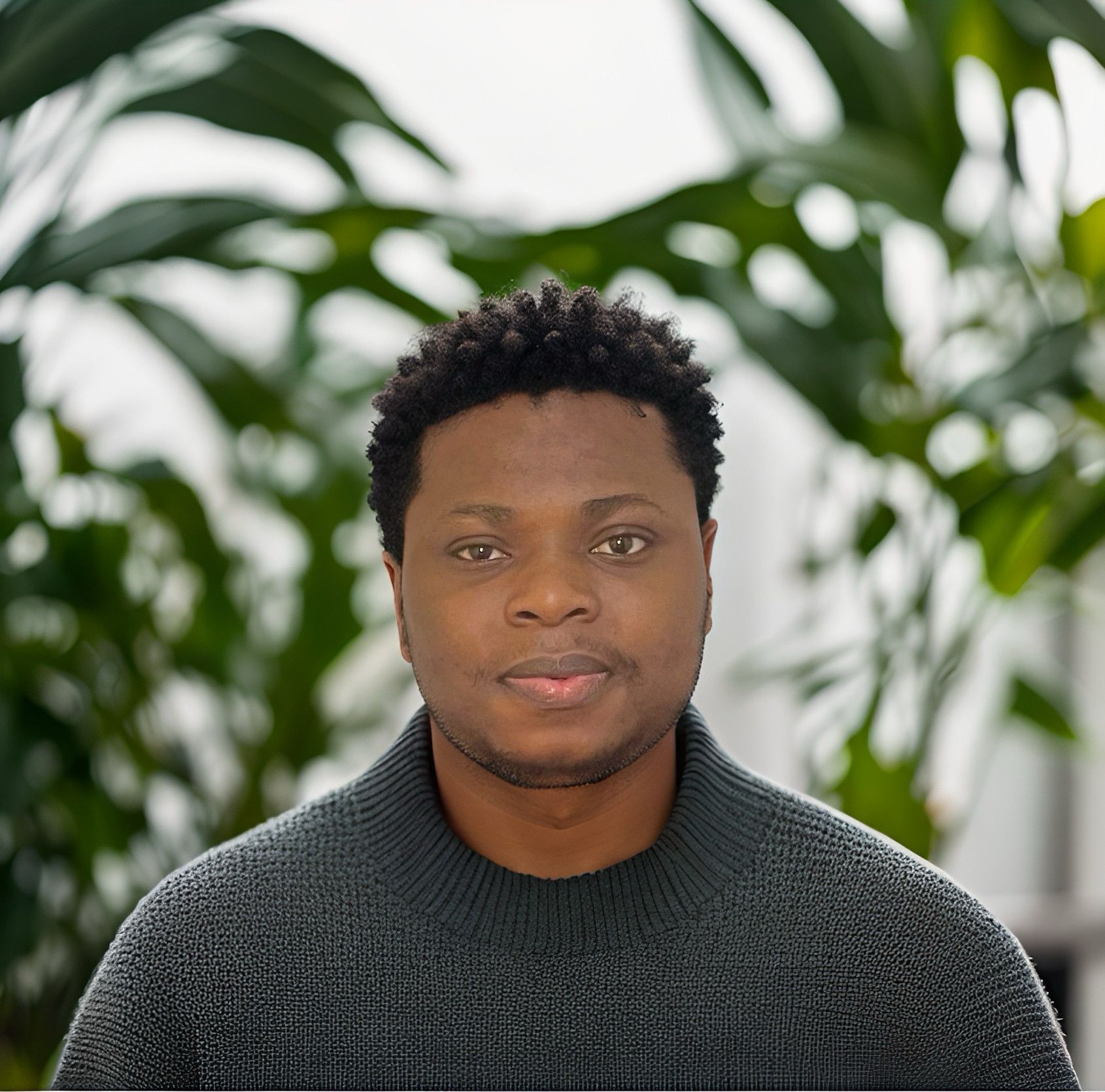
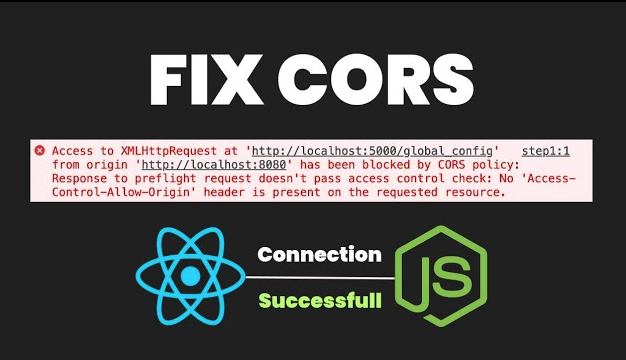
When building APIs with Node.js, one of the most common issues you might encounter is Cross-Origin Resource Sharing (CORS) errors. These errors typically occur when your backend (Node.js API) is on a different domain or port from your frontend, and your frontend tries to make requests to the backend. CORS is a security feature implemented by web browsers to prevent potentially harmful requests from untrusted sources, but it can also create headaches for developers trying to build APIs that are accessible from multiple domains.
In this article, we will explore how to fix CORS issues in Node.js APIs, configure CORS headers using the cors
middleware in Express, and troubleshoot common CORS-related problems.
What is CORS and Why Does It Matter?
Cross-Origin Resource Sharing (CORS) is a security mechanism that restricts web pages from making requests to a domain other than the one from which the page was served. For example, if your Node.js API is hosted on https://api.example.com
, but your frontend application is served from https://frontend.example.com
, browsers will block requests from the frontend to the API unless you explicitly allow it via CORS headers.
CORS errors usually manifest as something like:
Access to XMLHttpRequest at 'https://api.example.com/data' from origin 'https://frontend.example.com' has been blocked by CORS policy: Response to preflight request doesn't pass access control check.
This happens because, by default, browsers block cross-origin requests for security reasons.
Configuring CORS in Node.js with Express
To fix CORS issues in your Node.js APIs, you can use the cors
middleware, which makes it easy to configure CORS headers and control which domains can access your resources. Here's how to set it up:
Step 1: Install the CORS Middleware
First, install the cors
package via npm:
npm install cors
Step 2: Set Up CORS in Your Express Application
In your Express application, import and use the cors
middleware. Here's a simple example:
const express = require('express');
const cors = require('cors');
const app = express();
// Enable CORS for all routes
app.use(cors());
// A sample API route
app.get('/data', (req, res) => {
res.json({ message: 'This is some data from the server.' });
});
app.listen(3000, () => {
console.log('Server is running on http://localhost:3000');
});
By calling app.use(cors())
, you're allowing all incoming requests from any domain. However, this is not always secure, especially in production environments.
Customizing CORS for Specific Origins
In most cases, you will want to control which domains are allowed to make requests to your API. This is where you can customize the CORS configuration. You can specify which origins, methods, and headers are allowed.
Allow Specific Origins
You can allow only specific domains to access your API by passing an options object to the cors()
middleware. For example, if you want to allow only https://frontend.example.com
to access your API, you can configure CORS like this:
const corsOptions = {
origin: 'https://frontend.example.com', // Allow only this domain
methods: 'GET,POST', // Allow only specific HTTP methods
allowedHeaders: 'Content-Type, Authorization', // Allow specific headers
};
app.use(cors(corsOptions));
Allow Multiple Origins
If you need to allow multiple origins, you can use a function to dynamically check the origin and decide whether to allow it:
const corsOptions = {
origin: (origin, callback) => {
if (['https://frontend.example.com', 'https://anotherdomain.com'].includes(origin)) {
callback(null, true); // Allow the request
} else {
callback(new Error('Not allowed by CORS policy'));
}
},
};
app.use(cors(corsOptions));
Handling CORS Preflight Requests
A preflight request is an HTTP OPTIONS request sent by the browser to check if the server allows a particular CORS request. Preflight requests are sent automatically for HTTP methods such as PUT
, DELETE
, or when custom headers are used in a request.
To handle preflight requests, you can configure the CORS middleware to allow the necessary methods and headers:
const corsOptions = {
origin: 'https://frontend.example.com',
methods: 'GET,POST,PUT,DELETE', // Allow specific methods
allowedHeaders: 'Content-Type, Authorization', // Allow custom headers
preflightContinue: false, // Don't pass the preflight request to the next middleware
optionsSuccessStatus: 204, // Respond with a success status for preflight requests
};
app.use(cors(corsOptions));
This configuration ensures that the server responds to preflight requests correctly, and clients won't encounter CORS issues when making requests using methods like PUT
or DELETE
.
Allowing Credentials
If you need to include cookies or authorization headers in your cross-origin requests (e.g., for user authentication), you need to allow credentials in your CORS configuration.
Here’s how to configure CORS to allow credentials:
const corsOptions = {
origin: 'https://frontend.example.com',
credentials: true, // Allow cookies and credentials
};
app.use(cors(corsOptions));
Make sure your client-side requests are also set to include credentials, for example:
fetch('https://api.example.com/data', {
method: 'GET',
credentials: 'include', // Include cookies with the request
})
.then(response => response.json())
.then(data => console.log(data));
Debugging CORS Issues
Even after configuring CORS correctly, you might still encounter issues. Here are some common troubleshooting steps:
Check Browser Console Logs: The browser will typically display detailed CORS error messages in the console, which can help you understand what went wrong.
Ensure the Correct Headers: Check the response headers to ensure they include the appropriate CORS headers (e.g.,
Access-Control-Allow-Origin
,Access-Control-Allow-Methods
,Access-Control-Allow-Headers
).Verify the Preflight Request: If the CORS error occurs only with specific methods (like
PUT
orDELETE
), check the preflight OPTIONS request to ensure it’s being handled correctly.Check for Proxy Issues: If you're using a proxy server (e.g., in a development environment with
http-proxy-middleware
orwebpack-dev-server
), ensure that the proxy is correctly passing through the necessary CORS headers.
Conclusion
CORS issues are a common challenge when building APIs that need to be accessed from web browsers across different domains. By using the cors
middleware in Express, you can easily configure your Node.js API to handle cross-origin requests securely. With the right configuration, you can control which domains, methods, and headers are allowed, and ensure that your API is compatible with modern web applications.
Remember to:
Restrict access to trusted origins.
Handle preflight requests for non-simple HTTP methods.
Allow credentials when needed for secure user authentication.
By following these best practices, you can avoid common CORS issues and build secure, reliable Node.js APIs.
Happy coding!
Subscribe to my newsletter
Read articles from Nicholas Diamond directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
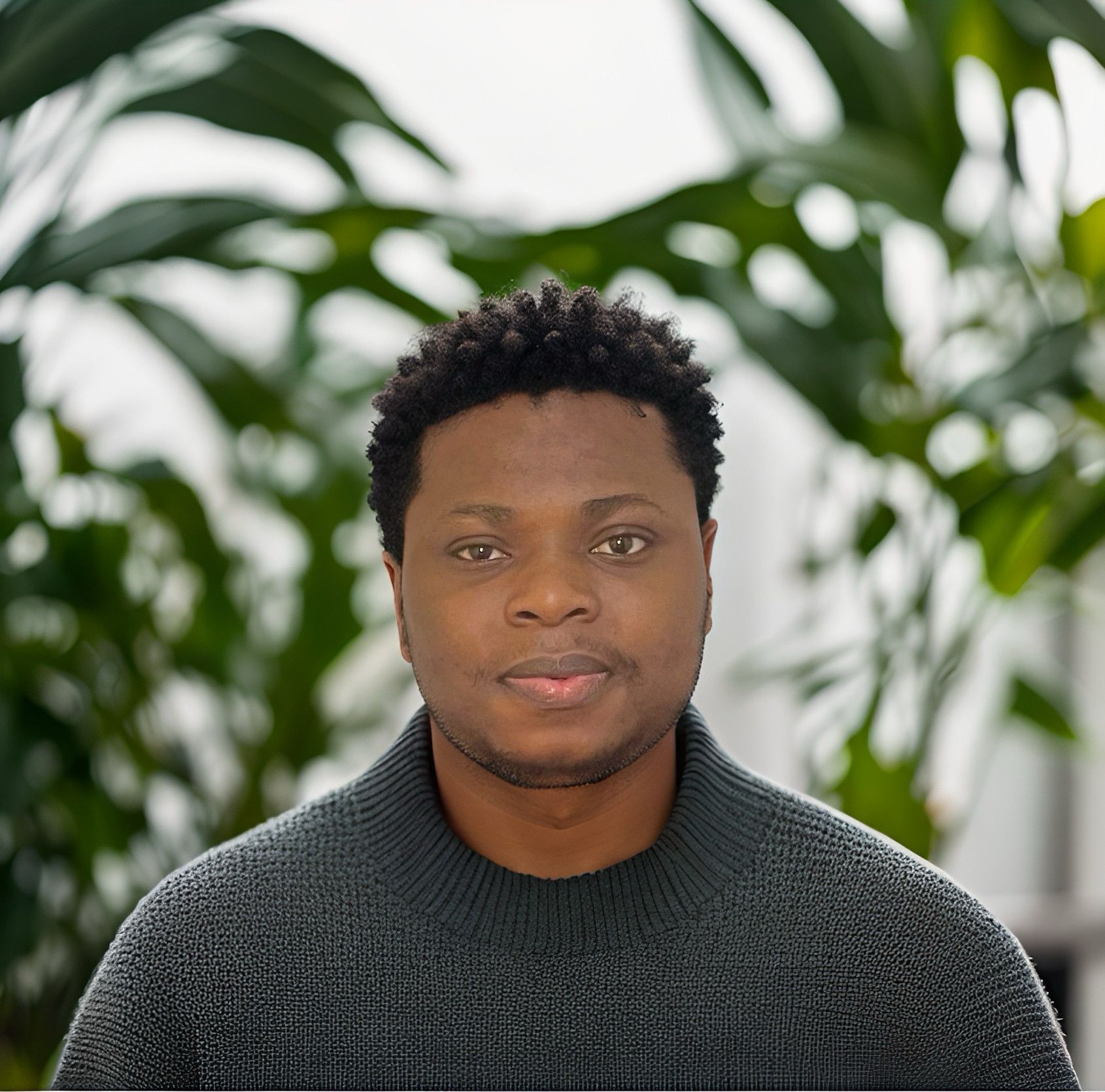