OOP : Journey from Procedural Beginnings
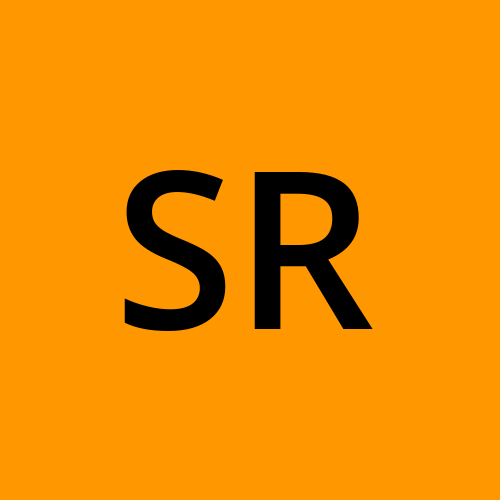
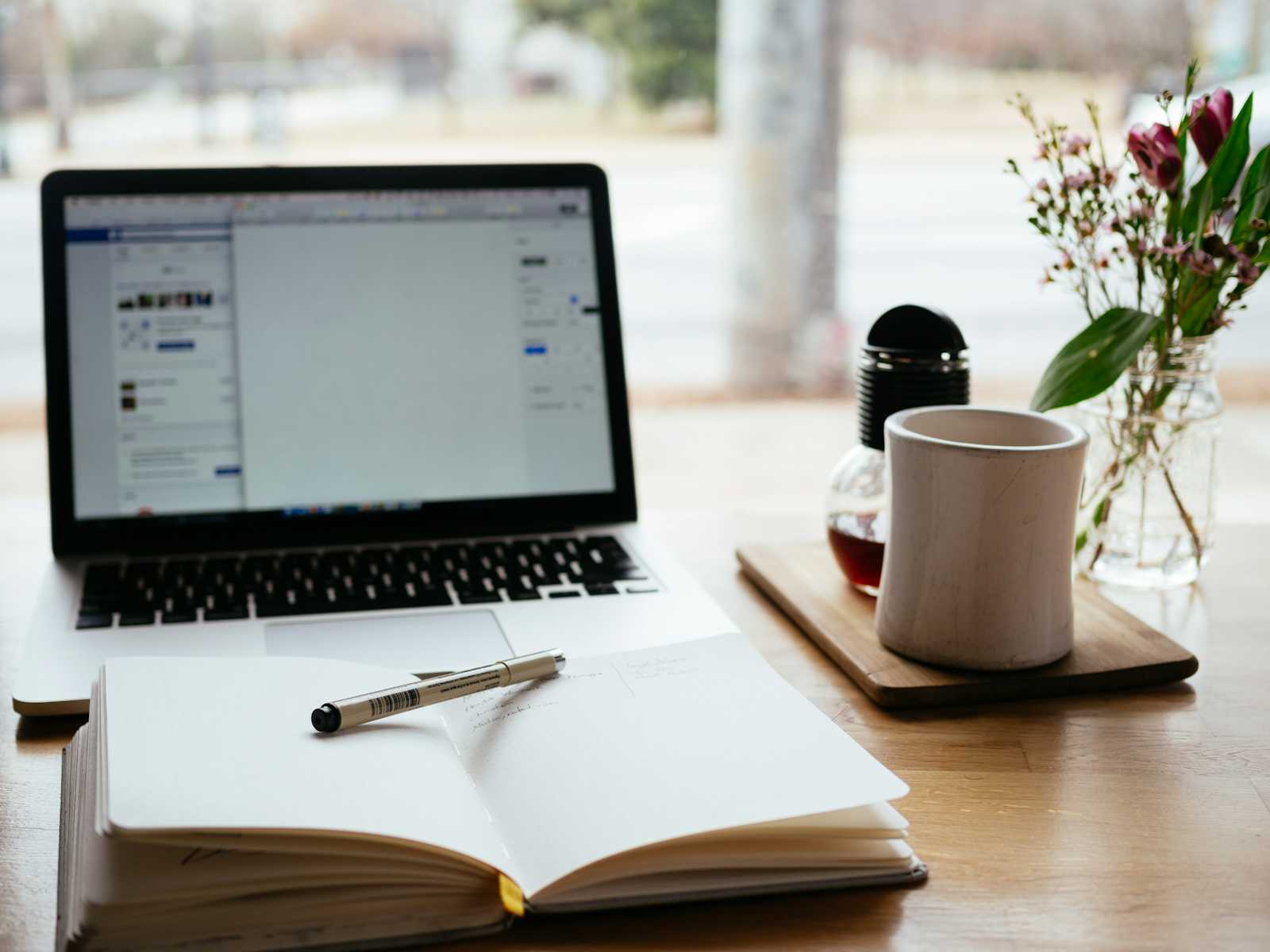
Let’s start with when humans started programming…
Ohh no! That would be too long ago. But you can read about how we began programming here. I’ll focus on OOP for now!
Procedural Programming
Okay, so the first high-level language is C and it utilizes a procedural paradigm.
Now procedure usually means a usual way of doing things
and here it means we give proper instructions through the program to work on its data.
To print student details in a procedural way using C, say we have a function printStudent that takes a student's data as a struct and prints the details of the student
#include <stdio.h>
struct Student {
char name[50];
int age;
float gpa;
};
void printStudentDetails(struct Student student) {
printf("Name: %s\n", student.name);
printf("Age: %d\n", student.age);
printf("GPA: %.2f\n", student.gpa);
}
int main() {
struct Student student1 = {"John Doe", 20, 3.5};
printStudentDetails(student1);
return 0;
}
This code defines a Student
structure and a function printStudentDetails
to print the details of a student. The main
function creates a Student
instance and calls the function to display the student's information.
Now, if you notice, the printStudent function is being applied on Student student1.
Moving from Procedural Programming to OOP
But, if you think of how we usually function in the real world…
take the following sentences:
Ada is writing code.
I am writing a blog.
You are reading the blog.
So, now if we focus on the sentence structure, we notice that the action is perfomed by the doer (in an active voice which we usually do and comes naturally to us)
While in the procedural world of programming, the action was performed on the doer.
This is the main difference between OOP and Procedural Programming. We have OOP as close to the world as possible to facilitate ease for creating new programs.
Also, everything in OOP is an idea at its base from there it can be further classified to various classifications we’ll see later.
An idea in OOP has its own attributes and behaviours just as in the real world everything has its attributes and behaviours.
Example :
1. a cat
attributes: has 4 legs, has fur, has a mouth, likes meat.
behaviours: walking, meowing, sleeping, eating
Let’s take something inanimate:
2. a water bottle
attributes: made of metal, has a cap, may be insulated
behaviour: holds water
OOP - its Principle and Pillars
The principle - the basic law of functioning, of OOP is Abstarction.
Abstraction means to hide the details of the implementation of the idea.
Now, to support Abstraction we have 3 pillars in OOP:
Encapsulation
Inheritance
Polymorphism.
I’ll talk about each of these in detail in the next blog..
Till then keep learning!
Subscribe to my newsletter
Read articles from Srushti directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
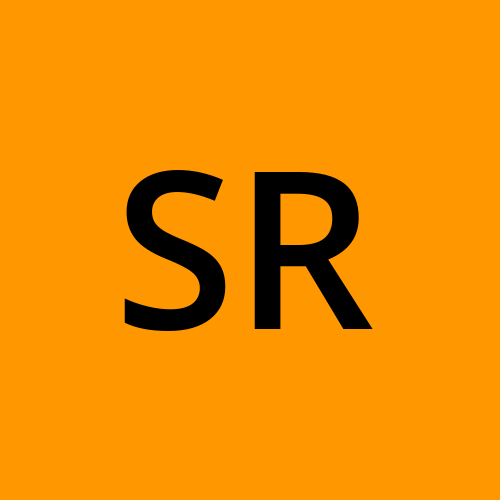