Writing a Twitter (X) API Script to Post Tweets with Python

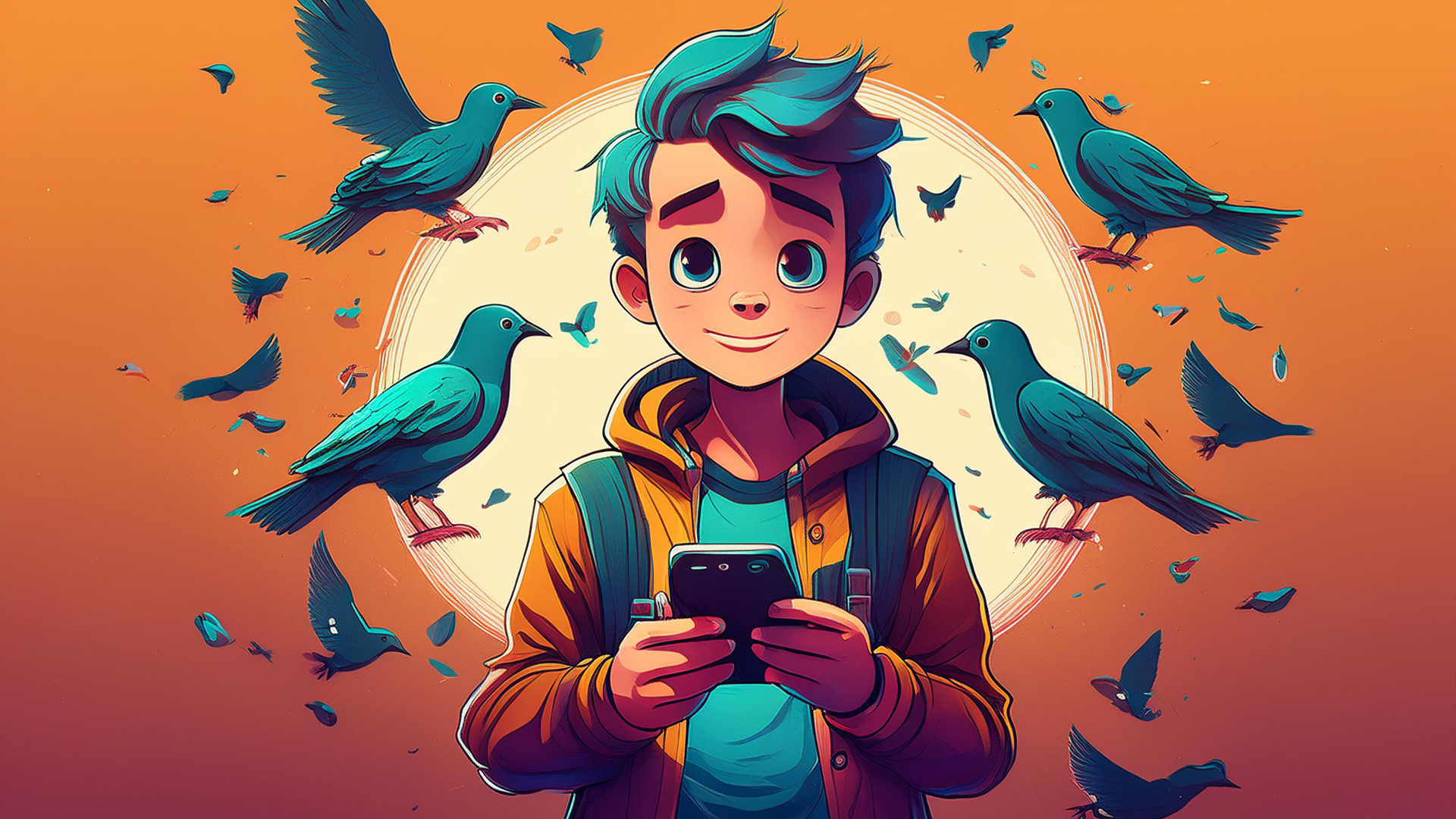
Social media automation can save time and improve engagement, whether you're managing a personal brand, running marketing campaigns, or sharing product updates.
Using Python, we can leverage Twitter's API to post tweets programmatically. I’ll break down a script that reads product information from an Excel file and posts customized tweets for each entry.
If you want to skip the article and just get the source, it is here on GitHub.
Tools and Libraries
Before we dive into the code, you’ll need to install the following libraries:
tweepy: A Python wrapper for the Twitter API.
pandas: A library for handling Excel files and data manipulation.
Install these libraries using pip:
pip install tweepy pandas
Prerequisites
To use this script, you’ll need:
A Twitter Developer Account: Sign up at developer.twitter.com to create a project and app.
API credentials:
API Key
API Secret Key
Access Token
Access Token Secret
An Excel file (x_post_list.xlsx) containing the following columns:
ProductName: The name of the product.
Price: The product's price.
URL: The link to the product.
Breaking Down the Script
1. Importing Libraries
import pandas as pd
import tweepy
pandas: Used to read and manipulate the Excel file containing the tweet data.
tweepy: Provides a simple interface for interacting with Twitter’s API.
2. Setting Up Twitter API Credentials
TWITTER_API_KEY = 'API_KEY'
TWITTER_API_SECRET = 'API_SECRET'
TWITTER_ACCESS_TOKEN = 'ACCESS_TOKEN'
TWITTER_ACCESS_SECRET = 'ACCESS_SECRET'
Replace these placeholder values with your actual Twitter API credentials. These credentials authenticate your app with Twitter.
3. Authenticating with Twitter's API
client = tweepy.Client(
consumer_key=TWITTER_API_KEY,
consumer_secret=TWITTER_API_SECRET,
access_token=TWITTER_ACCESS_TOKEN,
access_token_secret=TWITTER_ACCESS_SECRET
)
- tweepy.Client: Used to authenticate with the v2 Twitter API. This object allows you to perform actions like posting tweets.
4. Reading the Excel File
df = pd.read_excel('x_post_list.xlsx')
- pd.read_excel: Reads the Excel file into a Data Frame. Each row in the Data Frame represents a tweet.
5. Constructing and Posting Tweets
The script iterates through each row in the data frame to create and post a tweet.
a. Iterating Through Rows
for index, row in df.iterrows():
product_name = str(row['ProductName'])
price = str(row['Price'])
url = str(row['URL'])
df.iterrows: Loops through each row in the data frame.
Extracts product details (ProductName, Price, and URL) for the tweet.
b. Constructing the Tweet Text
tweet_template = "Check out {ProductName} that is currently ${Price} Click here for more details {URL}"
tweet_text = tweet_template.format(ProductName=product_name, Price=price, URL=url)
tweet_template: Defines the format of the tweet.
format(): Dynamically replaces placeholders with product details.
c. Handling Twitter’s Character Limit
if len(tweet_text) > 280:
extra_chars = len(tweet_text) - len(product_name)
allowed_length = 280 - extra_chars - 3 # Subtract 3 for '...'
allowed_length = max(allowed_length, 0)
product_name_short = product_name[:allowed_length] + '...'
tweet_text = tweet_template.format(ProductName=product_name_short, Price=price, URL=url)
Checks if the tweet exceeds Twitter’s 280-character limit.
If the tweet is too long:
Shortens ProductName to fit within the limit.
Adds ... to indicate truncation.
d. Posting the Tweet
try:
tweet_response = client.create_tweet(text=tweet_text)
print(f"Tweet posted successfully. Tweet ID: {tweet_response.data['id']}")
except tweepy.TweepyException as e:
print(f"An error occurred with Tweepy: {e}")
client.create_tweet: Posts the tweet using the v2 Twitter API.
If successful, prints the Tweet ID.
Catches and handles any exceptions raised by Tweepy (e.g., invalid credentials or API limits).
How the Script Works
Authenticate with Twitter: Ensures you have the necessary permissions to post tweets.
Read Data from Excel: Extracts product details like name, price, and URL.
Construct and Validate Tweets:
Ensures tweets adhere to Twitter’s 280-character limit.
Dynamically formats tweets with product details.
Post Tweets: Publishes each tweet to your Twitter account.
Example Workflow
Given the following Excel file (x_post_list.xlsx):
ProductName | Price | URL |
Gaming Laptop | 999 | https://example.com/laptop |
Wireless Headphones | 199 | https://example.com/headphones |
The script will post tweets like:
Tweet 1:
"Check out Gaming Laptop that is currently $999 Click here for more details https://example.com/laptop"
Tweet 2:
"Check out Wireless Headphones that is currently $199 Click here for more details https://example.com/headphones"
Error Handling
The script includes basic error handling:
API Errors: Catches exceptions from Tweepy and prints the error message.
Tweet Length: Ensures tweets stay within the character limit by truncating ProductName.
Next Steps and Customization Ideas
Schedule Tweets: Use the time or schedule library to automate posting at specific times.
Dynamic Hashtags: Add trending or category-specific hashtags to each tweet.
Image Attachments: Modify the script to include product images using Twitter’s media upload API.
This script is a powerful starting point for automating your Twitter activity. Whether you’re promoting products, sharing content, or simply learning how to use APIs, this script highlights the value of Python in social media automation.
Subscribe to my newsletter
Read articles from Steve Hatmaker Jr. directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Steve Hatmaker Jr.
Steve Hatmaker Jr.
I’m Steve Hatmaker, Jr., a programmer, coder, and problem solver who thrives on turning complex challenges into elegant solutions. My passion for technology fuels my journey as I navigate the ever-evolving world of programming. With a deep understanding of coding principles and a knack for innovative problem-solving, I’m dedicated to pushing the boundaries of what’s possible in the digital realm. From crafting sleek, functional software to developing intricate algorithms, my focus is on creating technology that not only meets but exceeds expectations. Each project is a new puzzle, and I approach it with the same curiosity and enthusiasm as a musician finding the perfect note or an artist discovering a new medium. While my website primarily highlights my work in programming and coding, my broader creative endeavors enrich my approach, offering a unique perspective on problem-solving. When I’m not immersed in code, I find inspiration in music and art, channels that influence my technical work in subtle yet profound ways. I believe that the creativity and discipline required in these fields complement and enhance my programming skills, allowing me to deliver solutions that are both innovative and practical.