Mastering Stripe Subscriptions in .NET Core: A Step-by-Step Guide to Recurring Payments
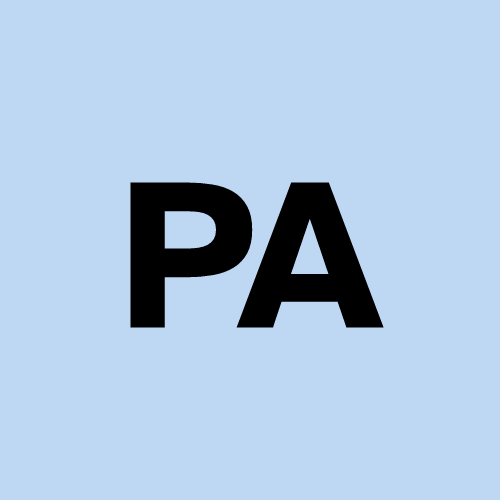
Table of contents
- Step 1: Set Up Your Stripe Account and Obtain API Keys
- Step 2: Install the Stripe.NET SDK
- Step 3: Configure Stripe in Your Application
- Step 4: Create Products and Pricing Plans in Stripe
- Step 5: Implement Subscription Logic
- Step 6: Handle Webhooks for Events
- Step 7: Test Your Implementation
- Step 8: Deploy and Monitor
- Best Practices
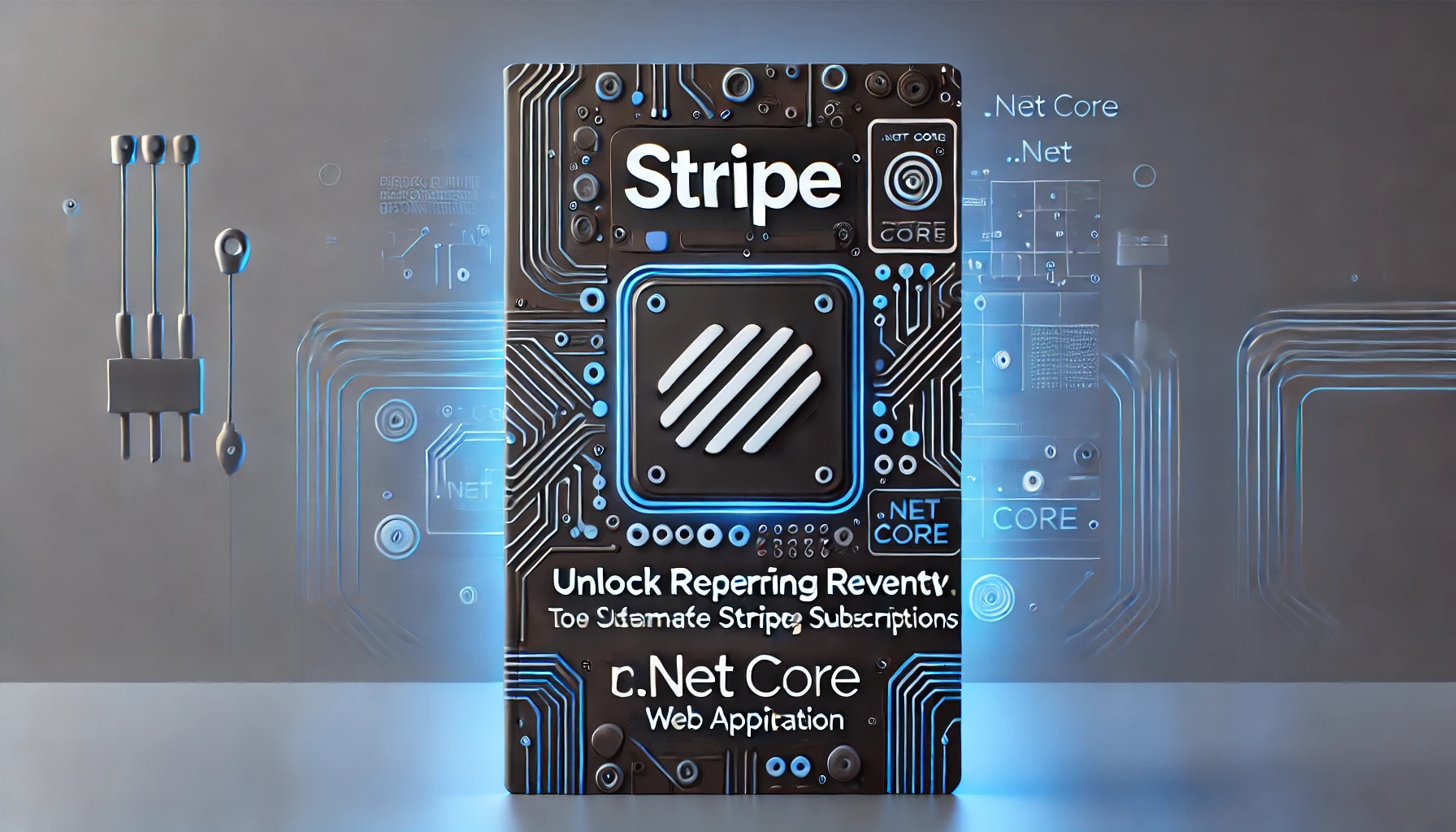
ntegrating recurring payment solutions into your application is crucial for any modern SaaS platform. In this guide, we’ll walk you through setting up Stripe subscriptions within your .NET Core project, enabling seamless billing and payments for your customers. Whether you're new to Stripe or .NET Core, this tutorial will provide you with all the tools you need to get started with recurring payments.
Implementing Stripe subscriptions in a .NET Core web application involves setting up your application to interact with the Stripe API for creating and managing subscriptions. Below is a step-by-step guide to get you started, along with advice, official documentation links, and best practices.
Step 1: Set Up Your Stripe Account and Obtain API Keys
Sign up at Stripe: Create an account if you don't have one.
Get API Keys:
Go to the Stripe Dashboard.
Navigate to the API Keys section under Developers.
Copy the Publishable Key and Secret Key.
Use the test keys for development and switch to live keys in production.
Click here for official Stripe API Keys Documentation
Step 2: Install the Stripe.NET SDK
- Add the Stripe.NET NuGet package to your .NET Core project:
using Stripe;
- Import the namespace in your project:
dotnet add package Stripe.net
Always keep he SDK updated to ensure compatibility with the latest Stripe features.
Step 3: Configure Stripe in Your Application
- Add your Stripe API keys to your configuration file (e.g.,
appsettings.json
):
{
"Stripe": {
"SecretKey": "sk_test_...",
"PublishableKey": "pk_test_..."
}
}
- Load these keys in the
Startup.cs
or a configuration service:
public void ConfigureServices(IServiceCollection services)
{
services.Configure<StripeSettings>(Configuration.GetSection("Stripe"));
}
- Create a settings class:
public class StripeSettings
{
public string SecretKey { get; set; }
public string PublishableKey { get; set; }
}
Never hardcode keys directly in your code; use environment variables or a secrets manager in production.
Step 4: Create Products and Pricing Plans in Stripe
Go to your Stripe Dashboard.
Under Products, create a product and set up pricing plans (e.g., monthly, annual).
Note the
price_id
for the plans you create
click here for Products and Prices Documentation.
Step 5: Implement Subscription Logic
Create a Customer: Use the Stripe API to create a customer:
Attach a Payment Method: Collect a payment method (e.g., credit card) using Stripe Elements on the frontend. Send the
payment_method_id
to your backend.Create a Subscription: Once the customer and payment method are set up,
Use Stripe webhooks to handle subscription lifecycle events like payment success, failure, or cancellations.
Step 6: Handle Webhooks for Events
Set up a Webhook Endpoint:
- In the Stripe Dashboard, configure a webhook endpoint to handle events (e.g., subscription created, invoice paid).
Verify Webhook Signatures:
var json = await new StreamReader(HttpContext.Request.Body).ReadToEndAsync();
var stripeEvent = EventUtility.ConstructEvent(
json,
Request.Headers["Stripe-Signature"],
"your_webhook_secret"
);
- Process Events:
if (stripeEvent.Type == Events.InvoicePaid)
{
// Handle successful payment
}
else if (stripeEvent.Type == Events.CustomerSubscriptionDeleted)
{
// Handle subscription cancellation
}
Always validate webhook signatures to ensure security.
Implement idempotency to avoid processing the same event multiple times.
Step 7: Test Your Implementation
Use Stripe's test mode to simulate different scenarios (e.g., successful payments, failed payments).
Test using the test card numbers provided by Stripe.
Step 8: Deploy and Monitor
Switch to live API keys in production.
Monitor logs and events in the Stripe Dashboard.
Best Practices
Secure Your API Keys: Use environment variables or secret management tools.
Handle Errors Gracefully: Log errors and provide user-friendly messages.
Use Webhooks Extensively: To stay updated on subscription events and automate workflows.
Ensure Compliance: Follow Stripe’s compliance guidelines for handling payment data.
By integrating Stripe subscriptions into your .NET Core application, you can unlock recurring revenue streams while providing a smooth customer experience. With Stripe's robust API, you can build flexible, scalable subscription systems tailored to your business needs.
Whether you're a developer implementing this for your organization or a client looking to monetize services, this guide provides the foundation to get started.
👉 Resources:
By leveraging Stripe’s robust subscription tools and integrating them into your .NET Core web application, you’re not just adding a payment gateway—you’re building a foundation for consistent, scalable revenue growth.
Subscribe to my newsletter
Read articles from Pragyanshu Aryan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
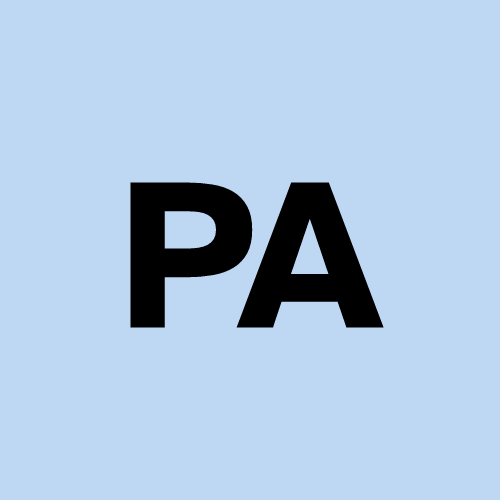