Using wkhtmltopdf on Amazon Linux 2023 (ARM64) in EC2: A Lightweight Alternative to Puppeteer for HTML to PDF Conversion

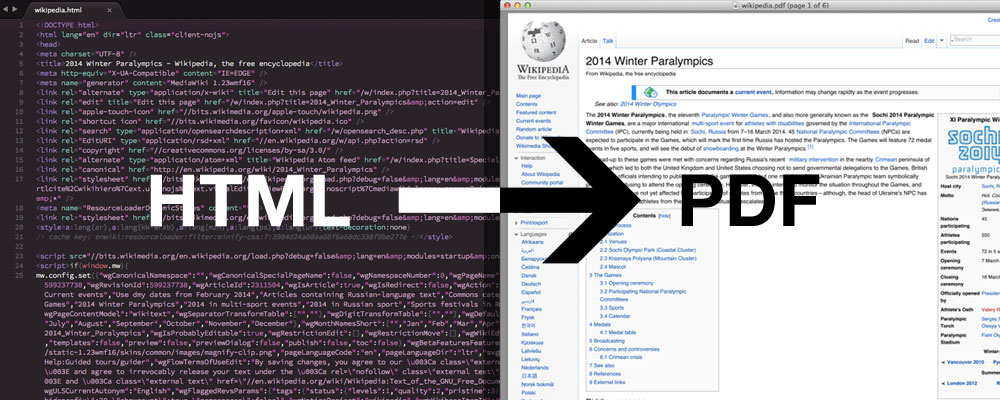
Background
We had a requirement to include a Terms and Conditions block in an existing PDF. The terms and conditions were represented as rows of an HTML table. For this, we were converting HTML to PDF using the puppeteer-html-pdf
Node.js package.
On our development server (running on an EC2 instance with Ubuntu and an x86_64 CPU architecture), this approach worked perfectly. Here’s the code snippet we used:
const PuppeteerHTMLPDF = require("puppeteer-html-pdf");
const options = { format: "A4" };
const file = {
content: `<div style="padding: 20px"><h2 style="text-align: center;">Terms And Conditions</h2>${customTnc}</div>`,
};
const htmlPDF = new PuppeteerHTMLPDF();
htmlPDF.setOptions(options);
const customTncBuffer = await htmlPDF.create(file.content);
The Problem
When we tried the same solution on our staging server, it failed. Our staging server was managed by a third-party firm and ran on Amazon Linux 2023 with an ARM64 (aarch64) architecture.
The issue arose because Puppeteer depends on a Chromium binary, which is not natively supported on ARM64. Despite trying solutions suggested in blogs and tools like ChatGPT, we couldn’t resolve the problem.
Limitations of Other Alternatives
We explored several other Node.js packages, such as jsPDF
, Playwright
, and html-pdf
. Unfortunately, none of these either met our requirements or worked on the staging server.
The Solution: wkhtmltopdf
Finally, we discovered the command-line tool wkhtmltopdf
. After some troubleshooting, we managed to install it on Amazon Linux 2023 (ARM64). Here's how you can do it easily:
Installing wkhtmltopdf on Amazon Linux 2023 (ARM64)
Run the following commands:
sudo yum install --assumeyes zlib fontconfig freetype libX11 libXext libXrender xorg-x11-fonts-75dpi xorg-x11-fonts-Type1
sudo yum localinstall -y https://repo.almalinux.org/almalinux/8/AppStream/aarch64/os/Packages/libpng15-1.5.30-7.el8.aarch64.rpm
sudo yum localinstall -y https://yum.oracle.com/repo/OracleLinux/OL8/appstream/aarch64/getPackage/compat-openssl10-1.0.2o-3.el8.aarch64.rpm
sudo yum localinstall -y https://github.com/wkhtmltopdf/packaging/releases/download/0.12.6-1/wkhtmltox-0.12.6-1.amazonlinux2.aarch64.rpm
Implementing wkhtmltopdf in Node.js
After installing wkhtmltopdf
, we switched to the corresponding Node.js package. This approach worked perfectly. Here’s the updated implementation:
const wkhtmltopdf = require("wkhtmltopdf");
const fs = require("fs");
const os = require("os");
const path = require("path");
const tncHtmlContent = `<div style="padding: 20px"><h2 style="text-align: center;">Terms And Conditions</h2>${customTnc}</div>`;
// Define a temporary file path
const tempFilePath = path.join(os.tmpdir(), `tempTnc_${Date.now()}.pdf`);
// Generate PDF for Terms and Conditions
await new Promise((resolve, reject) => {
wkhtmltopdf(tncHtmlContent, { output: tempFilePath }, (err) => {
if (err) {
reject(new Error(`Error generating T&C PDF: ${err.message}`));
} else {
resolve();
}
});
});
// Read the generated PDF
const customTncBuffer = fs.readFileSync(tempFilePath);
// Clean up temporary file
fs.unlinkSync(tempFilePath);
Key Takeaways
Puppeteer Limitations: Puppeteer relies on Chromium, which isn’t supported on ARM64 in Amazon Linux 2023.
Advantages of wkhtmltopdf: It is a lightweight command-line tool that doesn’t require Chromium and works seamlessly with ARM64.
Installation Challenges: Installing
wkhtmltopdf
on Amazon Linux 2023 with ARM64 requires additional dependencies, but the commands above simplify the process.
This solution allowed us to seamlessly generate PDFs with the desired Terms and Conditions block, even on our ARM64 staging server.
Subscribe to my newsletter
Read articles from Bhargab Hazarika directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
