Bubble Sort
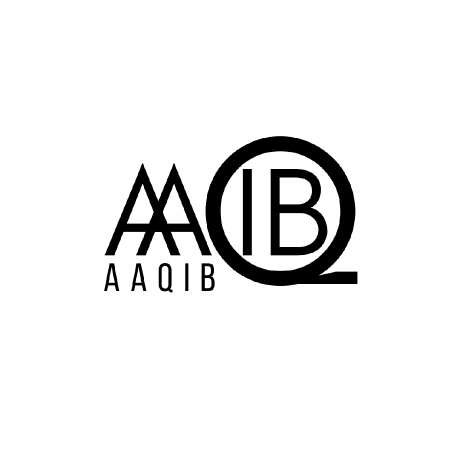
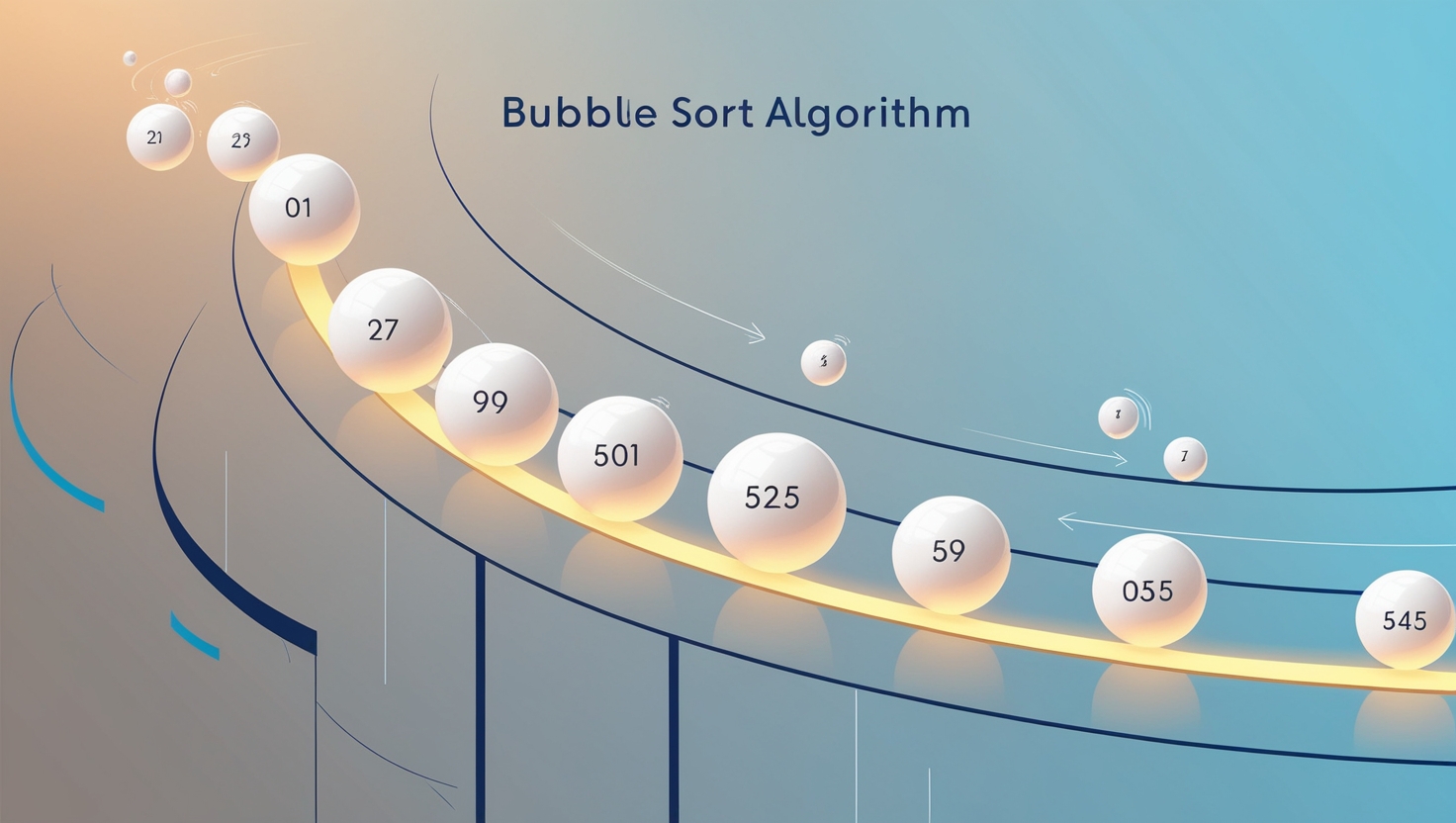
Understanding Bubble Sort: The Simplest Sorting Algorithm
Bubble Sort is one of the most basic and widely taught sorting algorithms. Although not the most efficient, its simplicity makes it a great starting point for anyone new to algorithms. Let’s dive into the concept, implementation, and analysis of Bubble Sort.
What is Bubble Sort?
Bubble Sort is a comparison-based sorting algorithm. It repeatedly steps through the list, compares adjacent elements, and swaps them if they are in the wrong order. This process continues until the list is sorted.
The name "Bubble Sort" comes from the way larger elements "bubble up" to the end of the list through successive swaps.
How Does Bubble Sort Work?
Start at the beginning of the array.
Compare the first two elements:
- If the first element is greater than the second, swap them.
Move to the next pair and repeat step 2.
Continue this process until the largest element is "bubbled" to its correct position at the end of the array.
Repeat the process for the remaining unsorted portion of the array.
Stop when no swaps are needed, indicating that the array is sorted.
Pass 1:
Compare 5 and 3 → Swap → [3, 5, 8, 6, 2]
Compare 5 and 8 → No Swap → [3, 5, 8, 6, 2]
Compare 8 and 6 → Swap → [3, 5, 6, 8, 2]
Compare 8 and 2 → Swap → [3, 5, 6, 2, 8]
Largest element (8) is now at the end.
Pass 2:
Compare 3 and 5 → No Swap → [3, 5, 6, 2, 8]
Compare 5 and 6 → No Swap → [3, 5, 6, 2, 8]
Compare 6 and 2 → Swap → [3, 5, 2, 6, 8]
Second largest element (6) is now in its correct position.
Pass 3:
Compare 3 and 5 → No Swap → [3, 5, 2, 6, 8]
Compare 5 and 2 → Swap → [3, 2, 5, 6, 8]
Pass 4:
- Compare 3 and 2 → Swap → [2, 3, 5, 6, 8]
Now the array is sorted.
Complexity Analysis
Time Complexity:
Best Case (Already Sorted): O(n)
Average Case: O(n²)
Worst Case (Reversed Array): O(n²)
Space Complexity:
- O(1): Bubble Sort is an in-place sorting algorithm, requiring no additional space.
When to Use Bubble Sort?
While Bubble Sort isn’t the most efficient, it’s ideal for:
Teaching basic sorting concepts.
Small datasets where performance isn’t critical.
Situations where simplicity is preferred over efficiency.
Subscribe to my newsletter
Read articles from Aaqib Bashir Mir directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
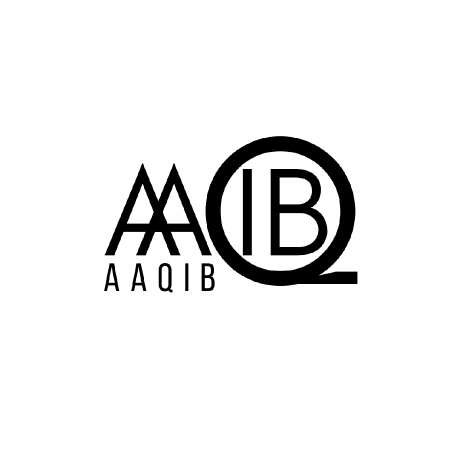