Data Fetching Methods in Next.js: A Comprehensive Guide
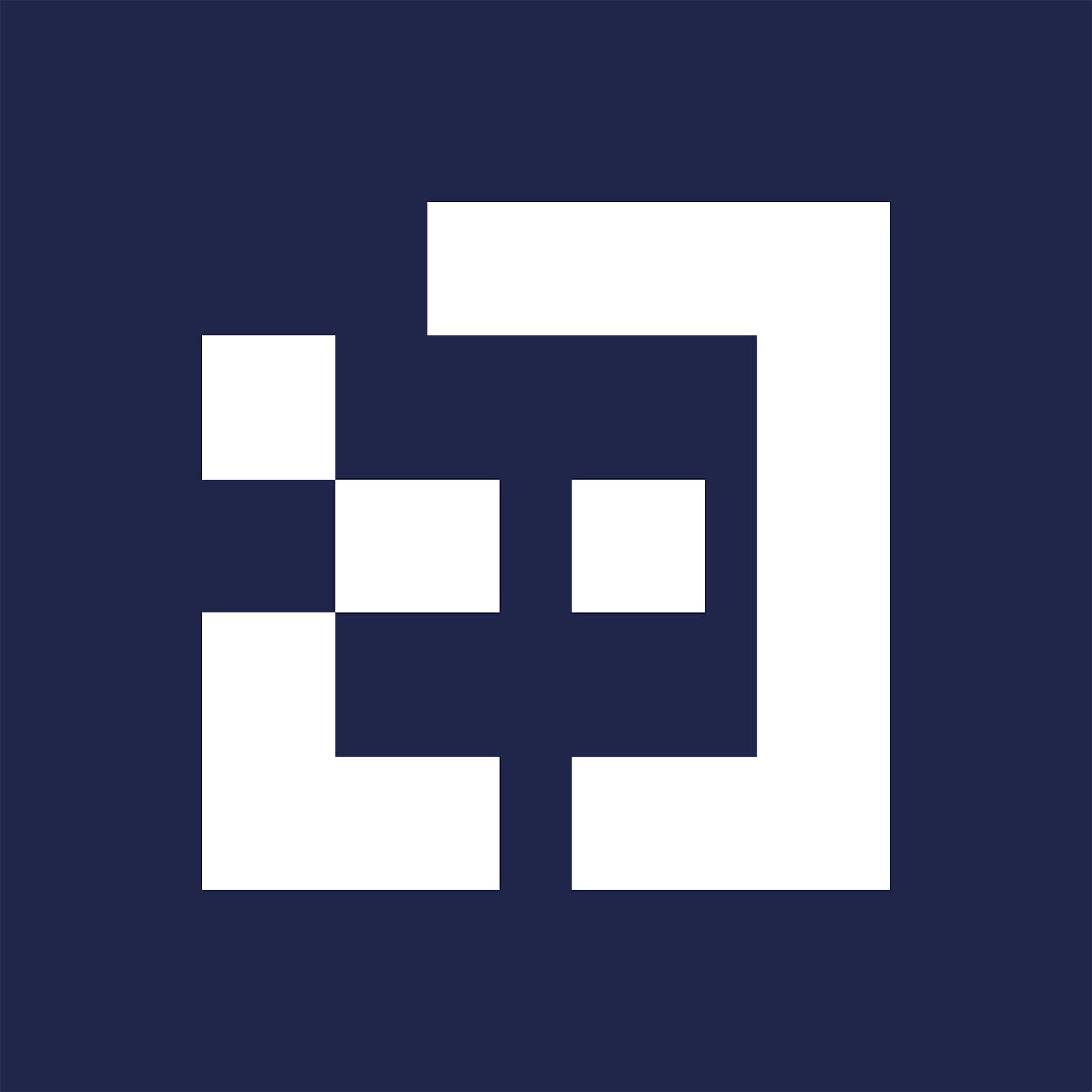
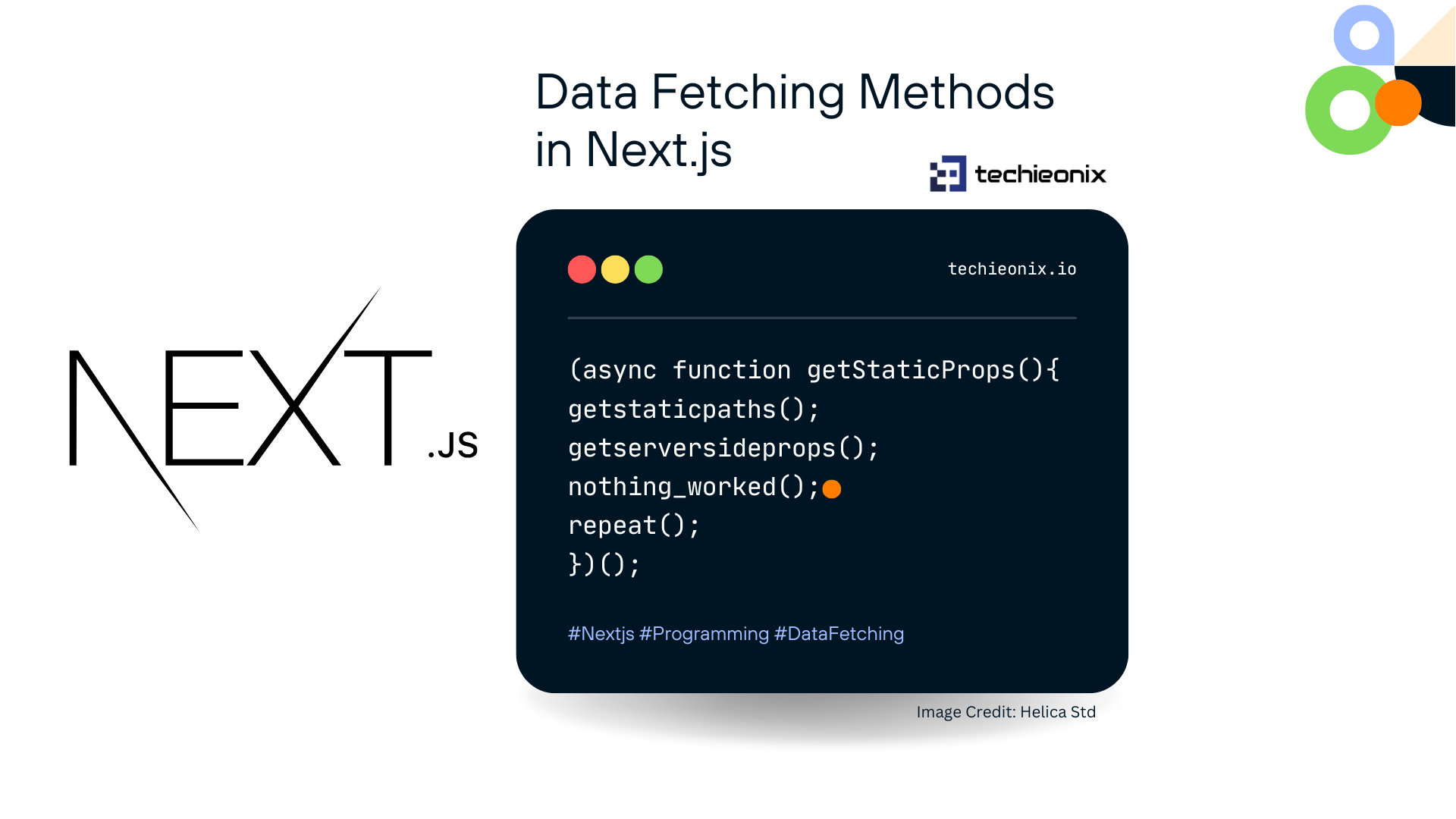
Next.js is a powerful React framework that simplifies the process of building server-rendered and statically generated web applications. One of its core strengths lies in its data fetching methods, which allow developers to optimize performance and enhance user experience. This article delves into three essential data-fetching methods in Next.js:
getStaticProps
getStaticPaths
getServerSideProps
We will explore each method in detail, complete with examples and real-world scenarios to help you grasp their practical applications.
getStaticProps
What is getStaticProps
?
getStaticProps
is a Next.js function that allows you to fetch data at build time. It enables you to pre-render pages with dynamic data, resulting in faster load times and improved SEO since the content is served as static HTML.
When to Use getStaticProps
?
Use getStaticProps
when:
The data required for the page is available at build time.
The data doesn't change frequently, or you can tolerate slight data staleness.
You aim to achieve better performance and SEO through pre-rendering.
How Does It Work?
Next.js invokes
getStaticProps
during the build process.The function fetches necessary data and passes it as props to the page component.
The page is pre-rendered into static HTML using this data.
The generated HTML is served to users on each request without re-running
getStaticProps
.
Basic Example
// pages/index.js
export default function Home({ posts }) {
return (
<div>
<h1>Latest Posts</h1>
<ul>
{posts.map((post) => (
<li key={post.id}>{post.title}</li>
))}
</ul>
</div>
);
}
export async function getStaticProps() {
// Fetch data from an external API
const res = await fetch('https://api.example.com/posts');
const posts = await res.json();
return {
props: {
posts,
},
};
}
In this example:
getStaticProps
fetches posts from an external API.The fetched posts are passed to the Home component as props.
The Home component renders a list of posts.
Real-World Scenario: Static Blog Generation
Scenario: You're developing a blog where new articles are added infrequently. You want each blog post to load quickly and be optimized for search engines.
Implementation:
1. Fetch Posts at Build Time
Use getStaticProps
to fetch all blog posts during the build process.
// pages/blog/index.js
export default function Blog({ posts }) {
return (
<div>
<h1>Blog</h1>
<ul>
{posts.map((post) => (
<li key={post.id}>
<a href={`/blog/${post.slug}`}>{post.title}</a>
</li>
))}
</ul>
</div>
);
}
export async function getStaticProps() {
const res = await fetch('https://api.example.com/posts');
const posts = await res.json();
return {
props: {
posts,
},
};
}
2. Generate Individual Post Pages
For each blog post, create a static page using getStaticProps
and getStaticPaths
(covered in the next section).
getStaticPaths
What is getStaticPaths
?
getStaticPaths
is a Next.js function used alongside getStaticProps
to enable static generation for dynamic routes. It specifies which dynamic pages should be pre-rendered at build time.
When to Use getStaticPaths
?
Use getStaticPaths
when:
You're working with dynamic routes (e.g.,
[id].js
).You want to pre-render pages based on dynamic data.
You're using
getStaticProps
on a page that includes dynamic parameters.
How Does It Work?
Next.js runs
getStaticPaths
during the build process.The function returns an array of path parameters to pre-render.
For each path, Next.js invokes
getStaticProps
to fetch data and generate the page.The pages are statically generated and served upon request.
Basic Example
// pages/posts/[id].js
export default function Post({ post }) {
return (
<div>
<h1>{post.title}</h1>
<p>{post.content}</p>
</div>
);
}
export async function getStaticPaths() {
// Fetch list of posts to determine paths
const res = await fetch('https://api.example.com/posts');
const posts = await res.json();
// Generate paths for each post
const paths = posts.map((post) => ({
params: { id: post.id.toString() },
}));
// Fallback false means other routes should 404
return { paths, fallback: false };
}
export async function getStaticProps({ params }) {
// Fetch individual post data
const res = await fetch(`https://api.example.com/posts/${params.id}`);
const post = await res.json();
return {
props: {
post,
},
};
}
Real-World Scenario: Dynamic Product Pages
Scenario: You're building an e-commerce site and want each product page to be pre-rendered for optimal performance and SEO.
Implementation:
1. Create a Dynamic Route
Define a dynamic route file pages/products/[id].js
.
2. Fetch All Product IDs
Use getStaticPaths
to fetch all product IDs that need to be pre-rendered.
// pages/products/[id].js
export async function getStaticPaths() {
const res = await fetch('https://api.example.com/products');
const products = await res.json();
const paths = products.map((product) => ({
params: { id: product.id.toString() },
}));
return { paths, fallback: false };
}
3. Fetch Individual Product Data
Use getStaticProps
to fetch data for each product based on the dynamic id.
export async function getStaticProps({ params }) {
const res = await fetch(`https://api.example.com/products/${params.id}`);
const product = await res.json();
return {
props: {
product,
},
};
}
4. Render the Product Page
export default function Product({ product }) {
return (
<div>
<h1>{product.name}</h1>
<p>{product.description}</p>
<p>Price: ${product.price}</p>
</div>
);
}
getServerSideProps
What is getServerSideProps
?
getServerSideProps
is a Next.js function that enables you to fetch data on each request. It facilitates server-side rendering (SSR), ensuring that pages are rendered with the most up-to-date data.
When to Use getServerSideProps
?
Use getServerSideProps
when:
The data required for the page changes frequently and must be current.
The page content depends on request-specific data (e.g., user authentication).
You need to render dynamic content that is SEO-friendly.
How Does It Work?
On every request, Next.js invokes
getServerSideProps
on the server.The function fetches data and passes it as props to the page component.
The page is server-rendered and the HTML is sent to the client.
Basic Example
// pages/profile.js
export default function Profile({ user }) {
return (
<div>
<h1>Your Profile</h1>
<p>Name: {user.name}</p>
</div>
);
}
export async function getServerSideProps(context) {
const { req } = context;
const sessionToken = req.cookies.sessionToken;
if (!sessionToken) {
return {
redirect: {
destination: '/login',
permanent: false,
},
};
}
// Fetch user data based on session token
const res = await fetch('https://api.example.com/user', {
headers: {
Authorization: `Bearer ${sessionToken}`,
},
});
if (res.status !== 200) {
return { notFound: true };
}
const user = await res.json();
return {
props: {
user,
},
};
}
Real-World Scenario: Personalized User Dashboard
Scenario: You're building a dashboard that displays personalized data for each authenticated user. The data must be fresh on each request.
Implementation:
1. Use getServerSideProps
to Fetch User-Specific Data
// pages/dashboard.js
export default function Dashboard({ userData }) {
return (
<div>
<h1>Welcome, {userData.name}!</h1>
<p>Your last login was on {userData.lastLoginDate}</p>
<!-- Additional personalized content -->
</div>
);
}
export async function getServerSideProps({ req }) {
const sessionToken = req.cookies.sessionToken;
if (!sessionToken) {
return {
redirect: {
destination: '/login',
permanent: false,
},
};
}
const res = await fetch('https://api.example.com/user/dashboard', {
headers: {
Authorization: `Bearer ${sessionToken}`,
},
});
if (res.status !== 200) {
return { notFound: true };
}
const userData = await res.json();
return {
props: {
userData,
},
};
}
2. Handle Authentication
Since getServerSideProps
runs on every request, you can:
Check if the user is authenticated by inspecting cookies or headers.
Redirect unauthenticated users to the login page.
Fetch user-specific data securely on the server side.
3. Ensure Data Freshness
Because data is fetched on each request, users always see the most recent information, enhancing the user experience.
Transform Your Vision into Reality with Techieonix
Ready to elevate your systems with the latest frameworks and technologies? Techieonix is here to turn your vision into reality. Our team of seasoned experts excels in developing high-performance, scalable solutions customized to your unique requirements. Contact Techieonix today to revolutionize your development process and take a decisive step toward innovation and success.
Conclusion
Understanding the data-fetching methods in Next.js is crucial for building optimized and scalable web applications. Here's a quick recap of when to use each method:
getStaticProps
: Ideal for pages where data can be fetched at build time and doesn't change frequently.getStaticPaths
: Use with dynamic routes to pre-render pages based on dynamic data.getServerSideProps
: Best for pages that require up-to-date data on every request or depend on request-specific information.
By leveraging these methods appropriately, you can enhance your application's performance, improve SEO, and provide a better user experience.
Next Steps:
Experiment with each data-fetching method in a sample Next.js project.
Consider caching strategies to optimize data fetching.
Explore incremental static regeneration (ISR) for revalidating static pages.
Happy coding!
♻️Repost if you find this valuable
🔔Follow Techieonix for more such content
🌐https://twitter.com/techieonix
Subscribe to my newsletter
Read articles from Techieonix directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
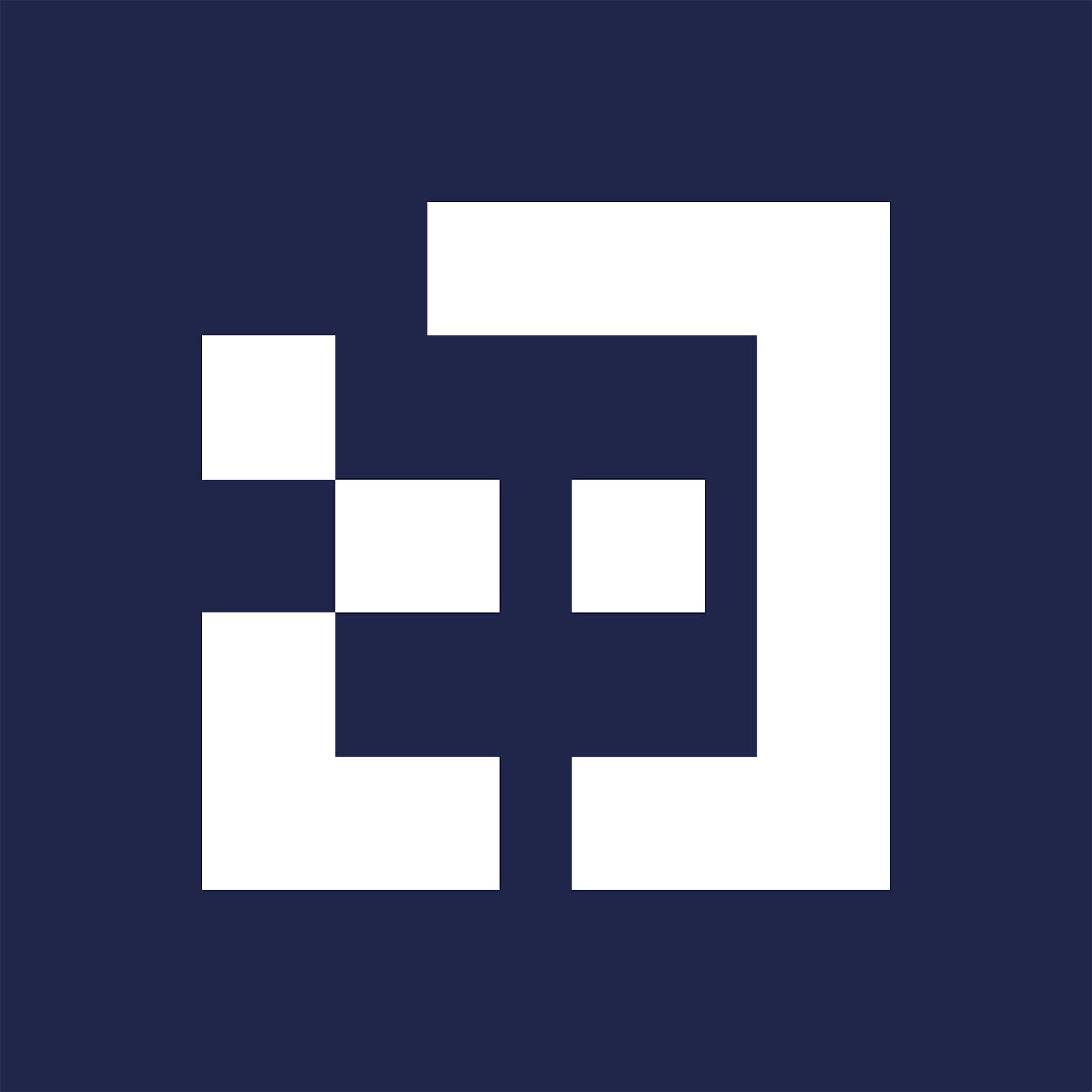
Techieonix
Techieonix
We are a team of developers who are committed to providing innovative and cutting-edge technology solutions to make work and life easier. We take pleasure in sharing our knowledge and expertise in the field of technology.