1.2 Objects , Classes , Data Abstraction , Encapsulation & Inheritance
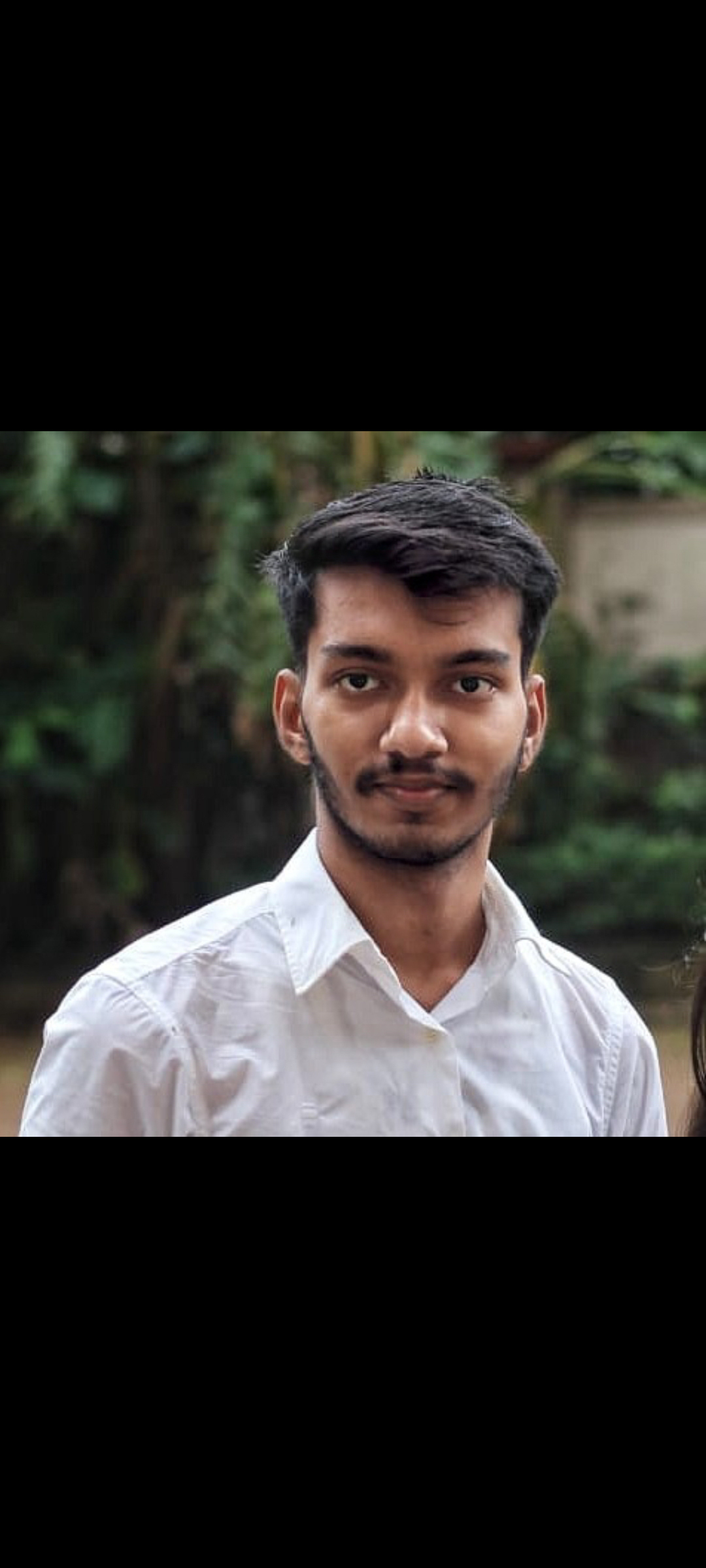
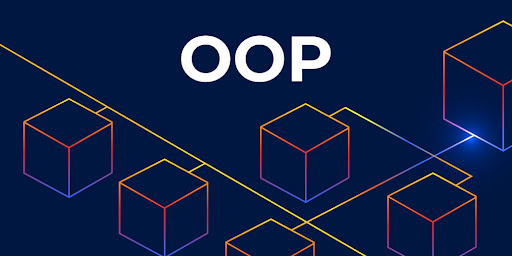
Object-Oriented Programming
Object-oriented programming (OOP) is a way to organize and conceptualize a program as a set of interacting objects.
The programmer defines the types of objects that will exist.
The programmer creates object instances as they are needed.
The programmer specifies how these various object will communicate and interact with each other.
What is an Object?
An object in Java is a fundamental unit of object-oriented programming that represents a real-world entity. It's like a blueprint of an object, defining its properties (attributes) and behaviors (methods).
Object-oriented programming is a methodology that gives programmers tools to make this modeling process easier.
Software objects, like real-world objects, have attributes and behaviors.
Your best bet is to think in terms as close as possible to the real world; trying to be tricky or cool with your system is almost always the wrong thing to do (remember, you can’t beat mother nature!)
Key characteristics of an object:
State: This refers to the data or attributes that define the object's characteristics. For example, a
Car
object might have attributes likecolor
,model
, andyear
.Behavior: This refers to the actions or functions that the object can perform. For example, a
Car
object might have methods likestart()
,stop()
, andaccelerate()
Creating Objects: To create an object in Java, you need a class. A class is a blueprint or template for creating objects. Once you have a class, you can create objects of that class using the new
keyword.
class Car {
String color;
String model;
int year;
public void start() {
System.out.println("Car started");
}
public void stop() {
System.out.println("Car stopped");
}
}
public class Main {
public static void main(String[] args) {
Car myCar = new Car();
myCar.color = "red";
myCar.model = "Camry";
myCar.year = 2023;
myCar.start();
myCar.stop();
}
}
In this example:
We define a
Car
class with attributescolor
,model
, andyear
, and methodsstart()
andstop()
.In the
main
method, we create an object of theCar
class namedmyCar
.We assign values to the attributes of
myCar
.We call the
start()
andstop()
methods ofmyCar
.
By understanding objects and classes, you can create complex and modular programs in Java.
In traditional programming languages (Fortran, Cobol, C, etc) data structures and procedures are defined separately.
In object-oriented languages, they are defined together.
An object is a collection of attributes and the behaviors that operate on them.
Variables in an object are called attributes.
Procedures associated with an object are called methods.
Classes
The definitions of the attributes and methods of an object are organized into a class. Thus, a class is the generic definition for a set of similar objects (i.e. Person as a generic definition for Jane, Mitch and Sue).
A class can be thought of as a template used to create a set of objects.
A class is a static definition; a piece of code written in a programming language.
One or more objects described by the class are instantiated at runtime.
The objects are called instances of the class.
Each instance will have its own distinct set of attributes.
Every instance of the same class will have the same set of attributes:
every object has the same attributes but, each instance will have its own distinct values for those attributes.
Example:
public class Car {
// Attributes (properties)
private String color;
private String model;
private int year;
// Constructor
public Car(String color, String model, int year) {
this.color = color;
this.model = model;
this.year = year;
}
// Methods (behaviors)
public void start() {
System.out.println("Car started.");
}
public void stop() {
System.out.println("Car stopped.");
}
// Getter and Setter methods (optional)
public String getColor() {
return color;
}
public void setColor(String color) {
this.color = color;
}
// ... other getter and setter methods for model and year
}
public class Main {
public static void main(String[] args) {
// Create a car object
Car myCar = new Car("Red", "Camry", 2023);
// Access and modify properties
myCar.setColor("Blue");
// Call methods
myCar.start();
myCar.stop();
}
}
Encapsulation
When classes are defined, programmers can specify that certain methods or state variables remain hidden inside the class.
These variables and methods are accessible from within the class, but not accessible outside it.
The combination of collecting all the attributes of an object into a single class definition, combined with the ability to hide some definitions and type information within the class, is known as encapsulation.
Encapsulation ensures that the internal workings of a class are hidden, promoting modular code.
// Java Program to demonstrate
// Java Encapsulation
// Person Class
class Person {
// Encapsulating the name and age
// only approachable and used using
// methods defined
private String name;
private int age;
public String getName() { return name; }
public void setName(String name) { this.name = name; }
public int getAge() { return age; }
public void setAge(int age) { this.age = age; }
}
// Driver Class
public class Main {
// main function
public static void main(String[] args)
{
// person object created
Person person = new Person();
person.setName("John");
person.setAge(30);
// Using methods to get the values from the
// variables
System.out.println("Name: " + person.getName());
System.out.println("Age: " + person.getAge());
}
}
Instance Methods and Instance Variables
The methods and variables described in this module so far are know as instance methods and instance variables.
The variables that the object contains are called instance variables. The methods (that is, subroutines) that the object contains are called instance methods.
These state variables are associated with the one instance of a class; the values of the state variables may vary from instance to instance.
Instance variables and instance methods can be public or private.
It is necessary to instantiate (create an instance of) a class to use it’s instance variables and instance methods.
Example: Instance Method
public void disp( )
{
int a= 10;
System.out.println(a);
}
Example: Instance Variables
In the following Java program, a class Studentsrecords is declared and instance variables are created using different access modifiers.
public class Studentsrecords
{
/* declaration of instance variables. */
public String name; //public instance
String division; //default instance
private int age; //private instance
/* Constructor that initialize an instance variable. */
public Studentsrecords(String sname)
{
name = sname;
}
/* Method to intialize an instance variable. */
public void setDiv(String sdiv)
{
division = sdiv;
}
/* Method to intialize an instance variable. */
public void setAge(int sage)
{
age = sage;
}
/* Method to display the values of instance variables. */
public void printstud()
{
System.out.println("Student Name: " + name );
System.out.println("Student Division: " + division);
System.out.println("Student Age: " + age);
}
public static void main(String args[])
{
Studentsrecords s = new Studentsrecords("Monica");
s.setAge(14);
s.setDiv("B");
s.printstud();
}
}
Difference between Local, Instance and Static variables in Java
Sr. No. | Local variables | Instance variables | Static variables |
1. | Variables declared within a method are local variables. | An instance variable is declared inside a class but outside of any method or block. | Static variables are declared inside a class but outside of a method starting with a keyword static. |
2. | The scope of the local variable is limited to the method it is declared inside. | An instance variable is accessible throughout the class. | The static variable is accessible throughout the class. |
3. | A local variable starts its lifetime when the method is invoked. | The object associated with the instance variable decides its lifetime. | The static variable has the same lifetime as the program. |
4. | Local variable is accessible to all the objects of the class. | Instance variable has different copies for different objects. | Static variables only have one single copy of the entire class. |
5. | Used to store values that are required for a particular method. | Used to store values that are needed to be accessed by different methods of the class. | Used for storing constants. |
Inheritance
Inheritance in Java is a mechanism in which one object acquires all the properties and behaviors of a parent object. It is an important part of OOPs (Object Oriented programming system).
Inheritance in Java enables a class to inherit properties and actions from another class, called a superclass or parent class. A class derived from a superclass is called a subclass or child group. Through inheritance, a subclass can access members of its superclass (fields and methods), enforce reuse rules, and encourage hierarchy.
Class: A class is a group of objects which have common properties. It is a template or blueprint from which objects are created.
Sub Class/Child Class: Subclass is a class which inherits the other class. It is also called a derived class, extended class, or child class.Subclasses are used to define special cases, extensions, or other variations from the originally defined class.
Super Class/Parent Class: Super class is the class from where a subclass inherits the features. It is also called a base class or a parent class.
Re usability: As the name specifies, re usability is a mechanism which facilitates you to reuse the fields and methods of the existing class when you create a new class. You can use the same fields and methods already defined in the previous class.
The syntax of Java Inheritance:
class Subclass-name extends Superclass-name
{
//methods and fields
}
The extends keyword indicates that we are making a new class that derives from an existing class. The meaning of "extends" is to increase the functionality.
Message Passing in Java
In object-oriented programming, communication between objects is a vital aspect of building complex systems. One of the key mechanisms for achieving this communication is message passing. In Java, message passing allows objects to interact with each other by invoking methods and passing data between them. In this article, we will explore the concept of message passing in Java and demonstrate its usage through example programs.
Message components include:
The name of the object to receive the message.
The name of the method to perform.
Any parameters needed for the method.
Polymorphism
Polymorphism in Java is a concept by which we can perform a single action in different ways. Polymorphism is derived from 2 Greek words: poly and morphs. The word "poly" means many and "morphs" means forms. So polymorphism means many forms.
True Polymorphism in Java: Runtime Polymorphism
True polymorphism, often referred to as runtime polymorphism or dynamic polymorphism, is a powerful feature in Java that allows objects of different classes to be treated as objects of a common superclass. This enables the same method to be invoked on different objects, resulting in different behaviors based on the object's actual type.
How it Works:
Inheritance: A subclass inherits the properties and methods of its superclass.
Method Overriding: The subclass can override a method inherited from the superclass, providing a specific implementation.
Dynamic Binding: At runtime, the Java Virtual Machine (JVM) determines the appropriate method to call based on the actual type of the object, not its declared type.
Example:
class Animal {
public void makeSound() {
System.out.println("Generic animal sound");
}
}
class Dog extends Animal {
@Override
public void makeSound() {
System.out.println("Woof!");
}
}
class Cat extends Animal {
@Override
public void makeSound() {
System.out.println("Meow!");
}
}
public class Main {
public static void main(String[] args) {
Animal animal1 = new Dog();
Animal animal2 = new Cat();
animal1.makeSound(); // Output: Woof!
animal2.makeSound(); // Output: Meow!
}
}
Parametric Polymorphism (Generics)
Parametric polymorphism, often referred to as generics, is a programming technique that allows you to write code that can work with different data types without compromising type safety. This is achieved by using type parameters, which are placeholders for actual types that will be specified when the code is used.
This is the use of the same method name within a class, but with a different signature (different parameters).
public class Box<T> {
private T content;
public Box(T content) {
this.content = content;
}
public T getContent() {
return content;
}
}
Thank You :)
Subscribe to my newsletter
Read articles from Arnab Bhowmik directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
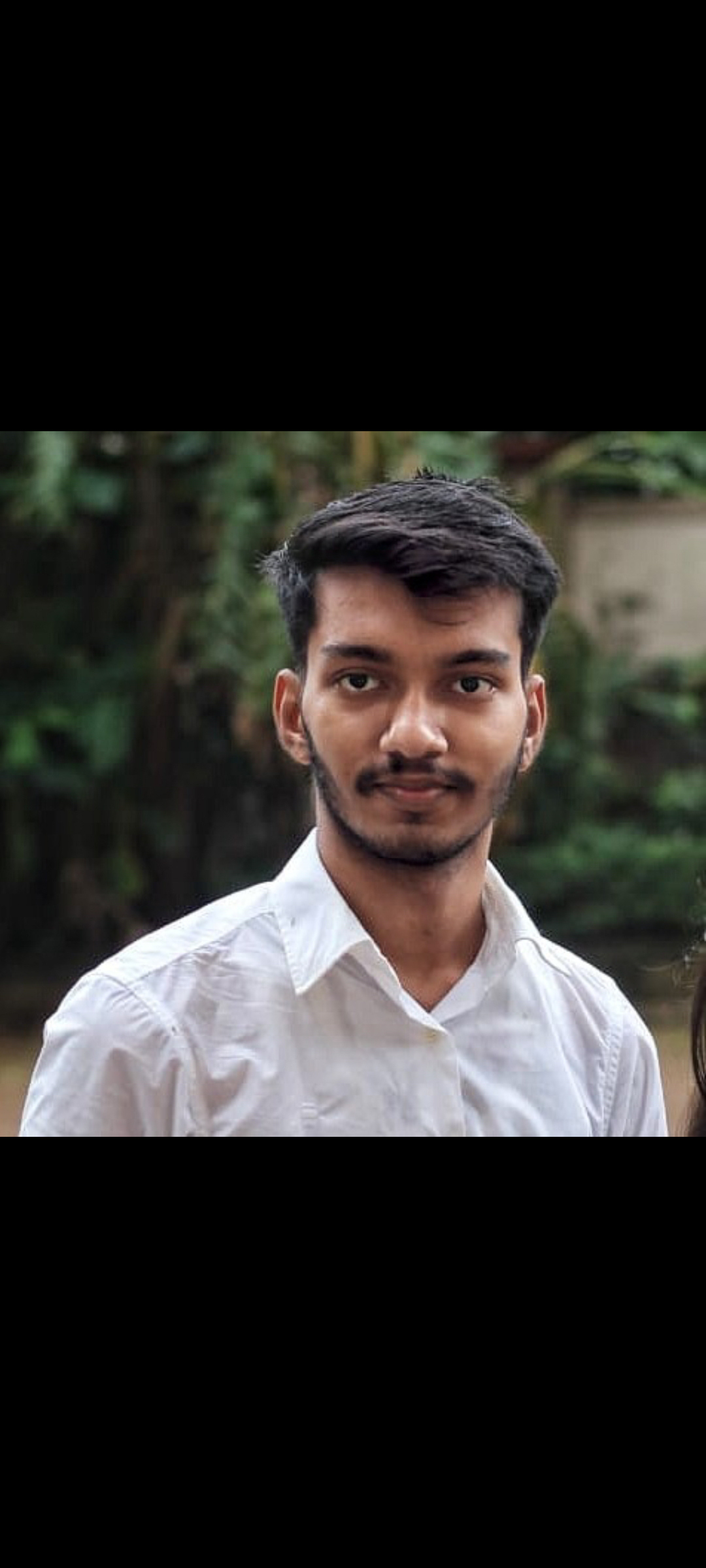
Arnab Bhowmik
Arnab Bhowmik
Noob programmer & problem solver.