An In-Depth Guide to Time Series Data and Forecasting

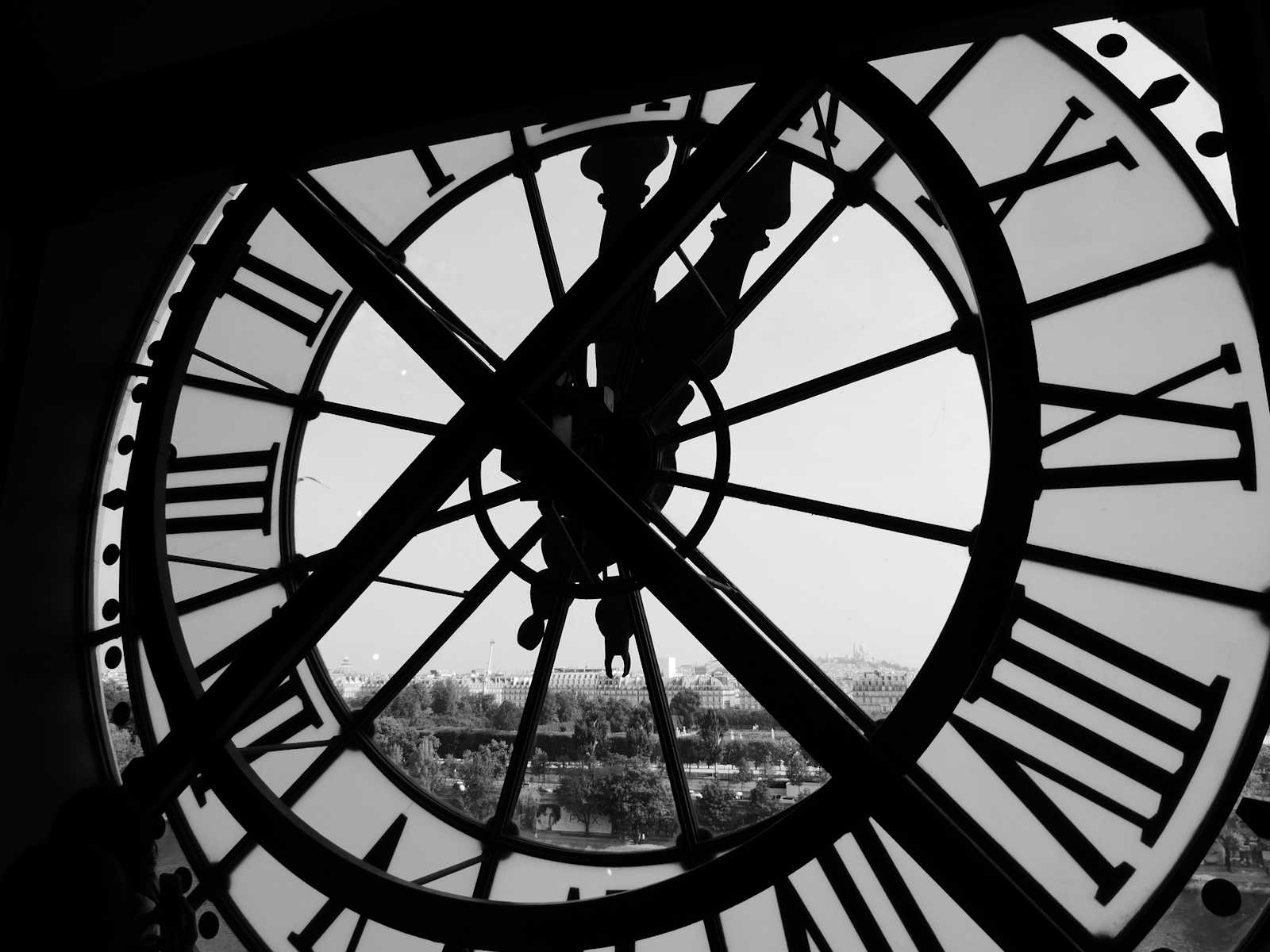
Welcome to this comprehensive guide on Time Series Data and Forecasting. This article aims to provide an in-depth understanding of time series data, its applications, methods for handling null values, and essential terms associated with time series forecasting. We'll also include code examples to help you implement these concepts practically.
Table of Contents
What is Time Series Data?
Time series data is a sequence of data points collected or recorded at successive points in time, usually at uniform intervals. Each data point is time-stamped, and the order is crucial because it reflects how the data evolves over time.
Key Characteristics:
Temporal Ordering: The sequence of data points is in chronological order.
Regular Intervals: Data is collected at consistent intervals (e.g., daily, monthly).
Dependence: Current observations may depend on past observations.
Applications of Time Series Data
Time series data is prevalent across various domains due to its ability to model and predict future values based on historical patterns.
Fields of Application:
Finance: Stock prices, interest rates, and market indices.
Economics: GDP growth rates, unemployment rates, and inflation.
Meteorology: Temperature readings, rainfall amounts, and climate models.
Healthcare: Patient vital signs monitoring over time.
Engineering: Sensor data from machinery for predictive maintenance.
Retail: Sales forecasting, inventory management.
Handling Null Values in Time Series Data
Null values (missing data) can significantly affect the analysis and forecasting of time series data. It's essential to handle them appropriately to maintain the integrity of the dataset.
1. Deletion Methods
a. Listwise Deletion
Description: Remove any time periods with null values.
Use Case: When the dataset is large, and missing values are minimal.
Drawback: Potential loss of valuable information.
# Python code example
df_clean = df.dropna()
b. Pairwise Deletion
Description: Use all available data without discarding entire records.
Use Case: When performing correlation or covariance analyses.
Drawback: Can lead to inconsistent sample sizes.
2. Imputation Techniques
a. Mean/Median Imputation
Description: Replace null values with the mean or median of the series.
Use Case: When data is missing at random.
Drawback: Can underestimate variability.
# Mean Imputation
df['value'] = df['value'].fillna(df['value'].mean())
b. Forward Fill (Last Observation Carried Forward)
Description: Replace null values with the last observed value.
Use Case: Suitable for data that changes slowly over time.
Drawback: Can propagate outdated information.
# Forward Fill
df['value'] = df['value'].fillna(method='ffill')
c. Backward Fill (Next Observation Carried Backward)
Description: Replace null values with the next observed value.
Use Case: When future values are known.
Drawback: Often impractical, as future data may not be available.
# Backward Fill
df['value'] = df['value'].fillna(method='bfill')
3. Interpolation Methods
a. Linear Interpolation
Description: Estimate missing values by connecting surrounding data points with a straight line.
Use Case: When data changes at a constant rate.
# Linear Interpolation
df['value'] = df['value'].interpolate(method='linear')
b. Polynomial Interpolation
Description: Fits a polynomial curve to the data.
Use Case: When data follows a non-linear trend.
# Polynomial Interpolation
df['value'] = df['value'].interpolate(method='polynomial', order=2)
c. Time Series Specific Methods
Description: Use models like ARIMA to predict missing values.
Use Case: When data has seasonal or trend components.
Basic Terms in Time Series Forecasting
Understanding the fundamental terms is crucial for effective time series analysis and forecasting.
Trend
Definition: The long-term movement in a time series without seasonal or cyclical variations.
Example: An upward trend in housing prices over several years.
Seasonality
Definition: Regular, repeating patterns or cycles in a time series tied to calendar periods.
Example: Increased retail sales during the holiday season.
Cyclicality
Definition: Fluctuations in time series data that are not of a fixed period.
Example: Economic recessions occurring at irregular intervals.
Stationarity
Definition: A time series is stationary if its statistical properties (mean, variance) are constant over time.
Importance: Many forecasting models assume stationarity.
Autocorrelation
Definition: The correlation of a time series with its own past values.
Use: Helps identify patterns and select appropriate models.
Lag
Definition: The time difference between observations in a time series.
Application: Used in models to predict current values based on past values.
Forecasting Models
ARIMA: AutoRegressive Integrated Moving Average.
SARIMA: Seasonal ARIMA.
Exponential Smoothing: A technique that applies decreasing weights over time.
LSTM Networks: Long Short-Term Memory networks, a type of neural network suitable for time series data.
Practical Implementation with Python
Let's apply these concepts using Python libraries such as pandas
, numpy
, matplotlib
, and statsmodels
.
Data Preparation
import numpy as np
import pandas as pd
import matplotlib.pyplot as plt
from statsmodels.tsa.seasonal import seasonal_decompose
# Generate synthetic time series data
np.random.seed(42)
dates = pd.date_range(start='2020-01-01', periods=100, freq='D')
data = np.random.normal(loc=50, scale=5, size=(100,))
# Introduce a trend
data += np.arange(100) * 0.1
# Introduce seasonality
data += 10 * np.sin(np.linspace(0, 20, 100))
# Introduce missing values
data[20] = np.nan
data[45:50] = np.nan
# Create DataFrame
df = pd.DataFrame({'Date': dates, 'Value': data}).set_index('Date')
Handling Null Values
Checking for Null Values
print(df.isnull().sum())
Imputing Missing Values with Linear Interpolation
df['Value'] = df['Value'].interpolate(method='linear')
Visualization Before and After Imputation
# Before Imputation
plt.figure(figsize=(12, 6))
plt.plot(df.index, data, label='Original Data with Nulls')
plt.title('Time Series with Missing Values')
plt.legend()
plt.show()
# After Imputation
plt.figure(figsize=(12, 6))
plt.plot(df['Value'], label='Data after Imputation', color='orange')
plt.title('Time Series after Handling Null Values')
plt.legend()
plt.show()
Time Series Decomposition
# Decompose the time series
decomposition = seasonal_decompose(df['Value'], model='additive', period=30)
# Plot the decomposition
fig = decomposition.plot()
fig.set_size_inches(14, 9)
plt.show()
Forecasting with ARIMA
Stationarity Check
from statsmodels.tsa.stattools import adfuller
# Perform Augmented Dickey-Fuller test
result = adfuller(df['Value'])
print('ADF Statistic:', result[0])
print('p-value:', result[1])
Differencing to Achieve Stationarity
df_diff = df['Value'].diff().dropna()
Fit ARIMA Model
from statsmodels.tsa.arima.model import ARIMA
# Define the model
model = ARIMA(df['Value'], order=(1, 1, 1)) # (p, d, q)
# Fit the model
model_fit = model.fit()
# Summary of the model
print(model_fit.summary())
Forecasting Future Values
# Forecast the next 10 days
forecast = model_fit.forecast(steps=10)
print(forecast)
# Plot the forecast
plt.figure(figsize=(12, 6))
plt.plot(df['Value'], label='Historical Data')
plt.plot(forecast.index, forecast, label='Forecast', color='red')
plt.title('Time Series Forecast')
plt.legend()
plt.show()
Conclusion
Time series data is a powerful tool for analyzing how variables change over time. Handling null values appropriately is critical for accurate analysis and forecasting. By understanding the basic terms and applying suitable models, you can uncover patterns and make informed predictions.
Subscribe to my newsletter
Read articles from Sai Prasanna Maharana directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
