Mastering the Node.js Module System: A Comprehensive Guide for Developers
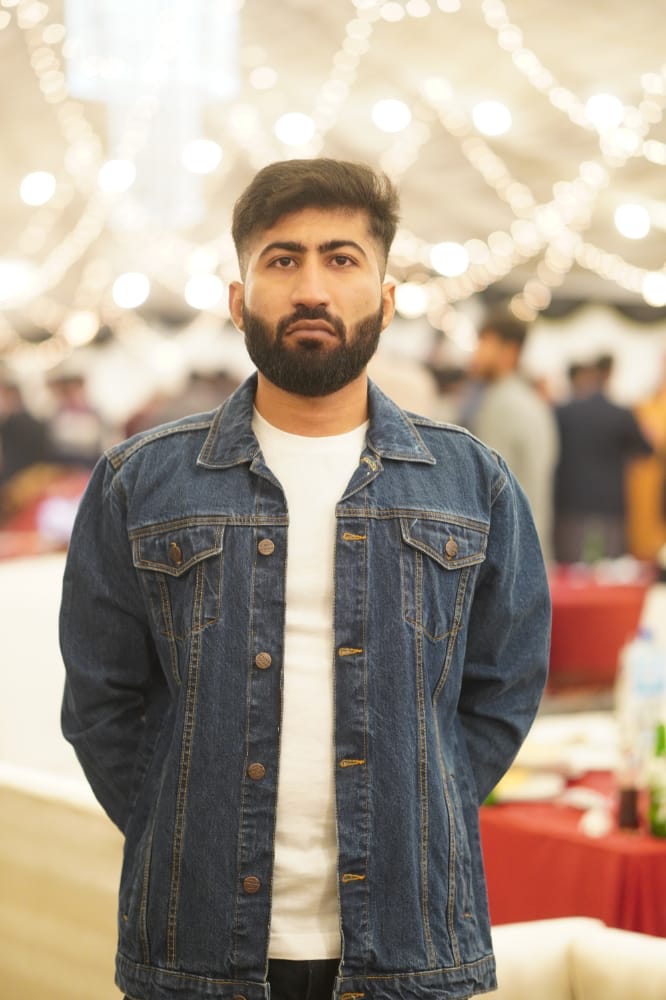
A module is a reusable piece of code encapsulated in its own file or directory. Node.js uses the CommonJS module system by default and also supports ECMAScript Modules (ESM).
1. Node.js Module System
Node.js has a built-in module system that allows you to organize your code into reusable blocks called modules. These modules can be files, directories, or packages. The system helps manage dependencies, isolate functionalities, and improve code reusability.
2. Types of Modules
A. Core Modules
These are built into Node.js.
Always available and require no installation.
Examples:
fs
,path
,http
,crypto
, etc.
Example:
const http = require('http');
const server = http.createServer((req, res) => {
res.end('Hello, Node.js!');
});
server.listen(3000, () => console.log('Server running on port 3000'));
B. Local Modules
Custom modules created by you.
These are your own files or directories.
Example:
// utils.js
module.exports.greet = (name) => `Hello, ${name}!`;
// app.js
const utils = require('./utils');
console.log(utils.greet('World'));
C. Third-Party Modules
Installed via
npm
oryarn
.Examples:
express
,lodash
,mongoose
.
Install and use:
npm install lodash
const _ = require('lodash');
console.log(_.shuffle([1, 2, 3, 4, 5]));
3. CommonJS (Default Module System)
Files are modules by default in Node.js.
Use
require
to import andmodule.exports
orexports
to export.
Exporting:
// math.js
module.exports = {
add: (a, b) => a + b,
subtract: (a, b) => a - b,
};
Importing:
const math = require('./math');
console.log(math.add(3, 7)); // 10
4. ECMAScript Modules (ESM)
Introduced in Node.js v12, stable in v14+.
Use
import
andexport
.Requires
"type": "module"
inpackage.json
or.mjs
extension.
Exporting:
// math.mjs
export const add = (a, b) => a + b;
export const subtract = (a, b) => a - b;
Importing:
import { add, subtract } from './math.mjs';
console.log(add(3, 7)); // 10
5. Module Loading and Resolution
A. How Node.js Finds Modules
Core Modules: Directly loaded if their names match (e.g.,
fs
).File or Directory Modules:
Looks for the file:
./module.js
If not found, checks for a directory with
index.js
orindex.mjs
.
Third-Party Modules: Checks
node_modules
directory.
B. Resolving Paths
Relative Paths:
./math.js
Absolute Paths:
/Users/project/src/utils.js
Node Modules:
require('express')
looks innode_modules
.
6. Module Caching
Modules are cached after the first
require
call.To avoid reloading, Node.js returns the cached version.
Example:
require('./module'); // Loaded and cached
require('./module'); // Cached version used
To reload, use:
delete require.cache[require.resolve('./module')];
require('./module'); // Reloads the module
7. JSON and Other Files
You can require
non-JS files, such as JSON:
const data = require('./data.json');
console.log(data.name);
8. package.json
and Exports
The package.json
file is critical for module configuration.
A. The "main"
Field
Defines the entry point for the module (default: index.js
).
{
"main": "src/index.js"
}
B. The "exports"
Field
Allows selective exposure of files.
{
"exports": {
".": "./lib/main.js",
"./utils": "./lib/utils.js"
}
}
9. Dynamic Imports
Import modules dynamically using import()
(ESM):
import('./math.mjs').then((math) => {
console.log(math.add(2, 3));
});
10. Advanced Concepts
A. Module Wrapping
Every module is wrapped in a function like this:
(function(exports, require, module, __filename, __dirname) {
// Your module code here
});
B. Built-in Variables
__dirname
: Directory of the current module.__filename
: Full path of the current module.
Example:
console.log(__dirname); // Outputs current directory
console.log(__filename); // Outputs current file path
11. Creating Node.js Packages
Initialize a package:
npm init
Create the package:
// index.js module.exports = { greet: (name) => `Hello, ${name}!` };
Publish to npm:
npm publish
12. Debugging and Best Practices
A. Debugging Modules
Use
console.log
for debugging.Use Node.js debugging tools (
node inspect
or IDE debuggers).
B. Best Practices
Use descriptive names for modules.
Organize code into directories (e.g.,
controllers
,services
).Use ES Modules for modern projects.
Keep
node_modules
out of version control (.gitignore
).
Subscribe to my newsletter
Read articles from Asawer directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
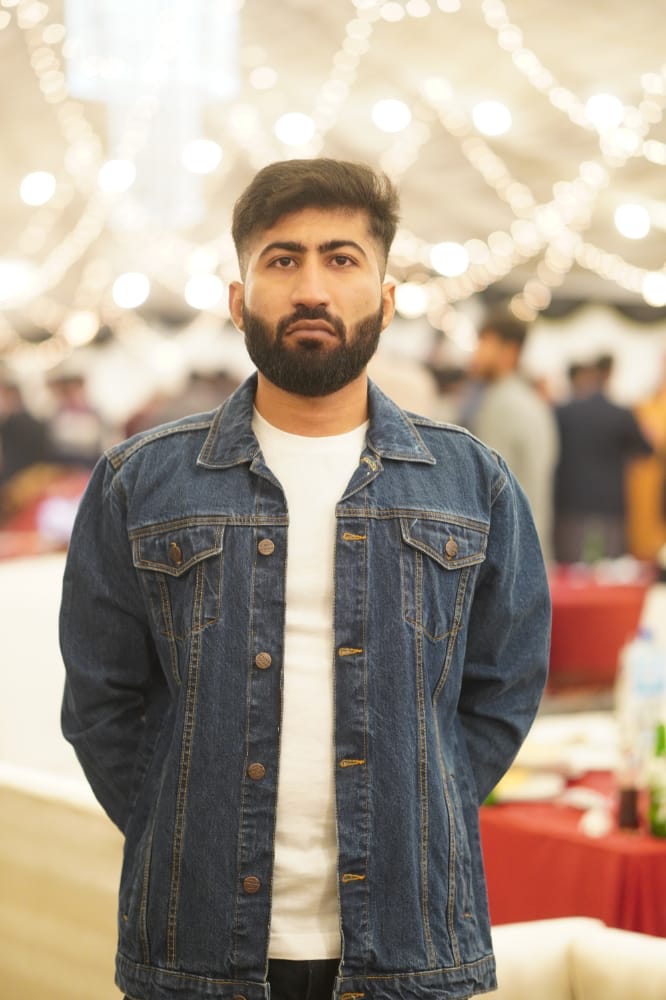