Learn about npm: Step-by-Step Guide to Node Package Manager
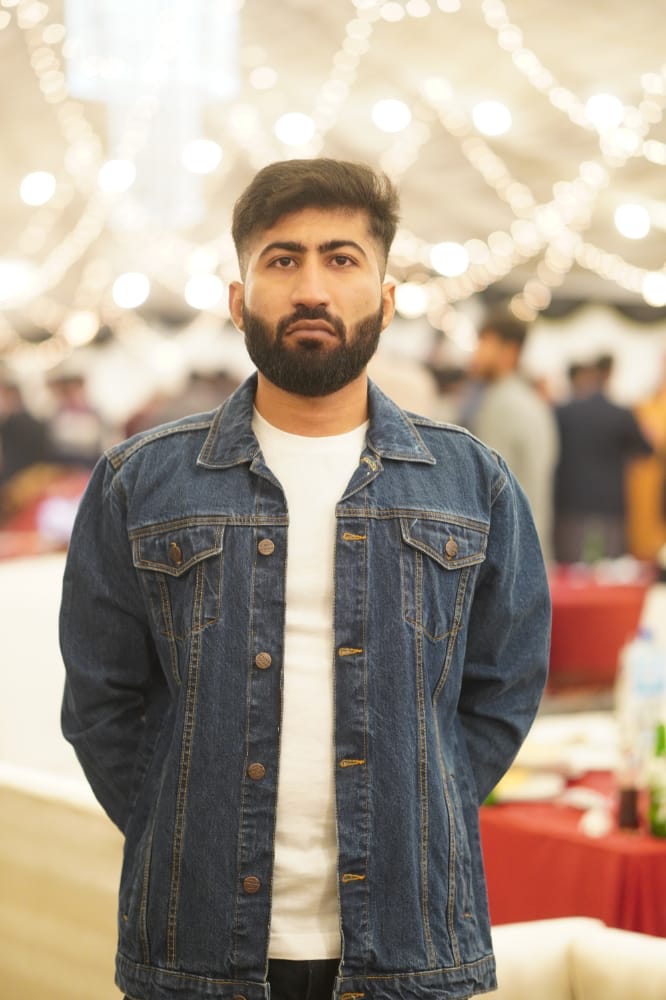
1. What is npm?
npm stands for Node Package Manager. It's the default package manager for Node.js.
It helps developers install, share, and manage dependencies in their Node.js projects.
It provides access to the npm registry, a vast collection of reusable packages.
2. Setting Up npm
A. Installing Node.js
npm comes bundled with Node.js.
Download and install Node.js from the official site.
B. Verifying Installation
Check the installed versions:
node -v # Check Node.js version
npm -v # Check npm version
3. Initializing a Project
A. Creating a package.json
File
The package.json
file is the heart of your Node.js project, storing metadata about the project and its dependencies.
Run:
npm init
You’ll be prompted for details like the project name, version, description, etc. Add -y
to skip the prompts and create a default package.json
:
npm init -y
Example package.json
:
{
"name": "my-project",
"version": "1.0.0",
"description": "A sample project",
"main": "index.js",
"scripts": {
"start": "node index.js"
},
"dependencies": {},
"devDependencies": {}
}
4. Installing Packages
A. Installing a Single Package
To install a package (e.g., lodash
):
npm install lodash
This adds lodash
to your dependencies
in package.json
and stores it in the node_modules
folder.
B. Installing Development Dependencies
For packages needed only during development (e.g., testing libraries):
npm install jest --save-dev
C. Global Installation
For tools used across projects (e.g., nodemon
):
npm install -g nodemon
D. Installing Specific Versions
npm install express@4.17.1
5. Dependency Management
A. Semantic Versioning (SemVer)
npm uses SemVer for versioning:
^1.2.3
: Allows minor and patch updates (e.g.,1.3.0
,1.2.4
).~1.2.3
: Allows only patch updates (e.g.,1.2.4
).1.2.3
: Installs exactly this version.
B. package-lock.json
This file locks the exact versions of dependencies installed, ensuring consistency across environments.
6. Useful npm Commands
A. Viewing Installed Packages
npm list # Lists installed dependencies
npm list -g # Lists globally installed packages
B. Updating Packages
npm update # Updates all dependencies
npm update lodash # Updates a specific package
C. Removing Packages
npm uninstall lodash
D. Cleaning Up
Remove unused dependencies:
npm prune
7. Scripts in npm
The scripts
section in package.json
lets you define custom commands:
"scripts": {
"start": "node index.js",
"test": "jest"
}
Run a script:
npm run start
npm run test
8. Creating Your Own npm Package
A. Step 1: Prepare the Project
Initialize the project:
npm init
Write your code:
// index.js module.exports = { greet: (name) => `Hello, ${name}!` };
B. Step 2: Add Metadata
- Ensure
package.json
has a proper name, version, and description.
C. Step 3: Publish the Package
Log in to npm:
npm login
Publish your package:
npm publish
D. Install Your Published Package
npm install your-package-name
9. Managing npm Configurations
A. View and Edit npm Config
npm config list
npm config set init-author-name "Your Name"
npm config delete init-author-name
B. Use .npmrc
Add custom configurations:
registry=https://registry.npmjs.org/
10. Security Best Practices
A. Check for Vulnerabilities
npm audit
npm audit fix
B. Avoid Installing Untrusted Packages
Review package popularity and code before installing.
Avoid globally installing unknown packages.
11. Troubleshooting npm
A. Clearing npm Cache
npm cache clean --force
B. Fixing Permissions Issues
Use npx
for isolated environments:
npx create-react-app my-app
12. Advanced npm Concepts
A. Workspaces
Workspaces allow managing multiple packages in a single project.
Create a
package.json
with:{ "workspaces": ["packages/*"] }
Add sub-packages under
packages/
.
B. Package Bundlers
Use tools like webpack
or esbuild
to bundle your npm packages for production.
Subscribe to my newsletter
Read articles from Asawer directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
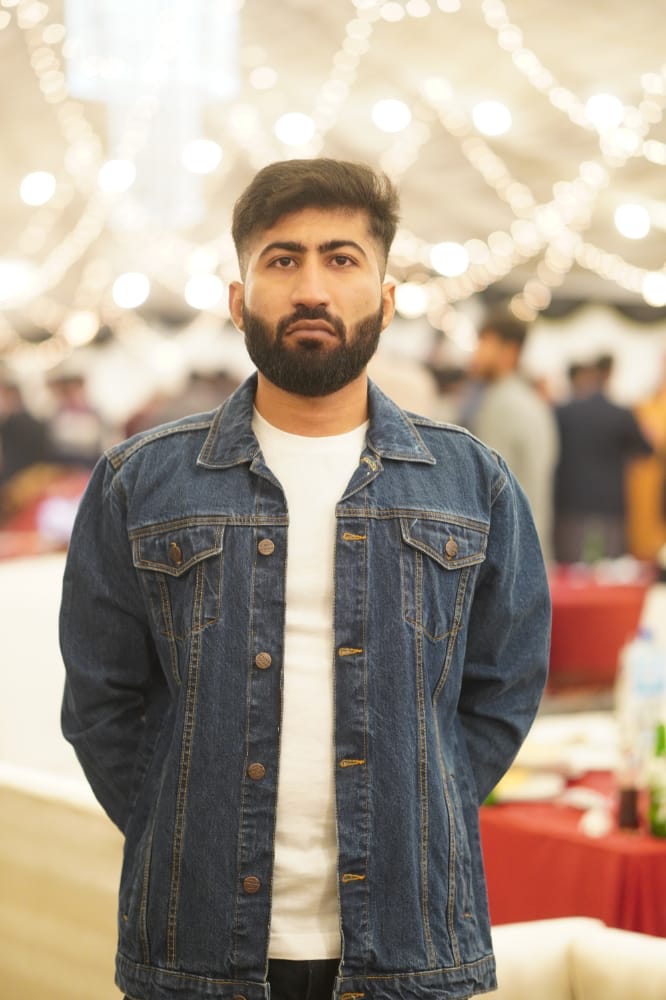