Docker Containerization for Django Applications - A Comprehensive Guide
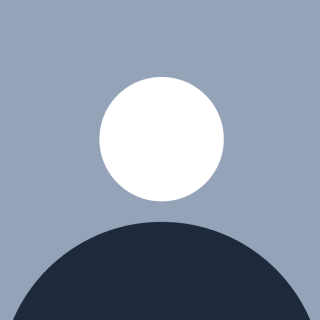
Introduction
Containerization has become an essential part of modern application deployment. This guide focuses on containerizing Django applications using Docker, exploring both the theoretical concepts and practical implementation.
Prerequisites for DevOps Engineers
Basic understanding of Python and Django application structure
Docker installed on your system
Basic knowledge of containerization concepts
Understanding Django Application Structure
Project Components
Django Project Files
settings.py
: Contains project configurationsurls.py
: Handles URL routingwsgi.py
: Web Server Gateway Interface configuration
Django App Files
Basic Django Project Setup
# Create new project
django-admin startproject projectname
# Create new app
python manage.py startapp appname
Docker Containerization Process
1. Dockerfile Structure
# Base image
FROM ubuntu
# Set working directory
WORKDIR /app
# Copy requirements first (for better caching)
COPY requirements.txt .
# Install Python and dependencies
RUN apt-get update && apt-get install -y python3 python3-pip
RUN pip3 install -r requirements.txt
# Copy project files
COPY . .
# Entry point and command
ENTRYPOINT ["python3"]
CMD ["manage.py", "runserver", "0.0.0.0:8000"]
2. Key Docker Commands
# Build image
docker build -t django-app .
# Run container
docker run -p 8000:8000 django-app
Important Concepts
Entry Point vs CMD
ENTRYPOINT: Non-overrideable starting command (e.g.,
python3
)CMD: Configurable parameters (e.g., port numbers, server options)
Port Mapping
Use
-p
flag to map container ports to host portsExample:
-p 8000:8000
maps container port 8000 to host port 8000
Security Considerations
Configure security group rules for EC2 instances
Open necessary ports in inbound traffic rules
Consider using specific IP ranges instead of 0.0.0.0/0
Best Practices
Dependencies Management
Copy requirements.txt separately
Install dependencies before copying application code
Use virtual environments when appropriate
Docker Image Optimization
Use appropriate base images
Minimize layer size
Consider multi-stage builds for production
Development vs Production
Use different Dockerfile configurations
Consider using Docker Compose for development
Implement proper environment variable management
Common Issues and Solutions
Port Conflicts
Use different port mappings if 8000 is occupied
Configure Django to listen on different ports
Permission Issues
Properly configure user permissions in container
Handle static files permissions
Container Access
Configure ALLOWED_HOSTS in Django settings
Set proper network configurations
Conclusion
Containerizing Django applications with Docker provides consistency across different environments and simplifies deployment. Understanding both Django application structure and Docker concepts is crucial for successful containerization.
Next Steps
Learn about Docker networking
Explore multi-stage builds
Study Docker Compose for multi-container applications
Implement CI/CD pipelines for containerized applications
Subscribe to my newsletter
Read articles from Amulya directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by