Prevent XSS Attacks in Laravel: Best Practices & Examples

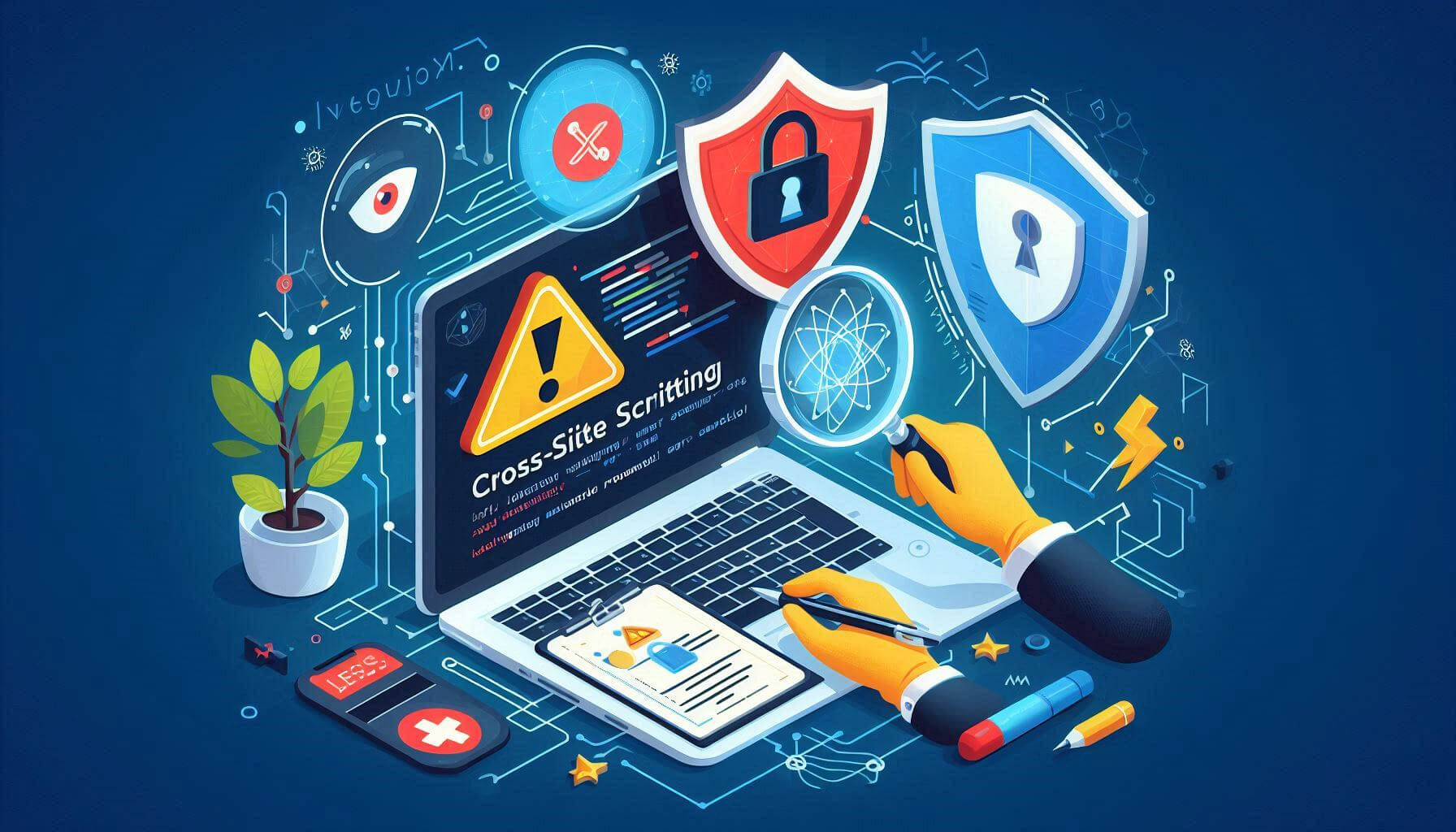
Cross-Site Scripting (XSS) is one of the most prevalent vulnerabilities affecting web applications. Attackers inject malicious scripts into your website, compromising the user experience and potentially stealing sensitive data. As Laravel developers, it's critical to implement robust measures against XSS to secure your applications and build trust with your users.
In this guide, we’ll explore what XSS is, why it’s dangerous, and how to prevent it in Laravel with practical coding examples.
To make things even easier, we recommend using our Free Website Security Checker Tool to identify vulnerabilities in your application. Below, you'll also find a sample vulnerability report generated by the tool to give you actionable insights.
What is Cross-Site Scripting (XSS)?
XSS allows attackers to inject malicious code, typically JavaScript, into web pages viewed by other users. These attacks can lead to:
Data theft
Session hijacking
Phishing attacks
Malware distribution
Laravel provides some default security measures, but manual intervention is often necessary to fully safeguard your app.
How to Prevent XSS in Laravel
1. Use Blade’s Escaping Syntax
Laravel’s Blade template engine automatically escapes variables to prevent XSS. Use {{ $variable }}
to safely output data.
Example:
php
// Safe Output
{{ $userInput }}
// Unsafe Output - Avoid using this!
{!! $userInput !!}
2. Validate and Sanitize User Inputs
Use Laravel’s validation rules to sanitize inputs before processing them.
Example:
php
$request->validate([
'username' => 'required|string|max:255',
'email' => 'required|email',
]);
3. Utilize Content Security Policy (CSP)
Implement a CSP header to restrict the types of content that can be executed in your application.
Example:
Add the following in your app/Http/Middleware/CustomHeaders.php
file:
php
$response->headers->set
('Content-Security-Policy', "default-src 'self';
script-src 'self'; style-src 'self';");
Using the Free Website Security Checker Tool
Take Action Against Vulnerabilities
Run your website through our Free Website Security Checker Tool. The tool provides a comprehensive vulnerability assessment, helping you identify potential threats, including XSS.Generate Vulnerability Assessment Reports
Get actionable insights by downloading a detailed vulnerability report. This report highlights specific areas that need fixing, to ensure your website’s security.
Common Mistakes to Avoid
Directly using
eval()
orinnerHTML
: These functions can execute malicious scripts.Trusting user-generated content: Always sanitize before storing or displaying it.
Ignoring API responses: Sanitize all external API data before processing.
Conclusion
Cross-Site Scripting is a serious threat to Laravel applications, but with the right measures, it’s entirely preventable. Using Blade’s escaping syntax, validating inputs, and leveraging CSP headers are just some of the strategies to secure your web app.
Don’t forget to use our tool to test website security free for real-time vulnerability checks and proactive security measures.
Stay secure and code responsibly!
Additional Tips for Hashnode Readers
Share your experiences with XSS attacks and how you mitigated them in the comments below.
Follow us for more Laravel security tips and tools!
Subscribe to my newsletter
Read articles from Pentest_Testing_Corp directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Pentest_Testing_Corp
Pentest_Testing_Corp
Pentest Testing Corp. offers advanced penetration testing to identify vulnerabilities and secure businesses in the USA and UK, helping safeguard data and strengthen defenses against evolving cyber threats. Visit us at free.pentesttesting.com now to get a Free Website Security Check.