Linear Search Explained: The Simple Approach to Finding Data

Table of contents
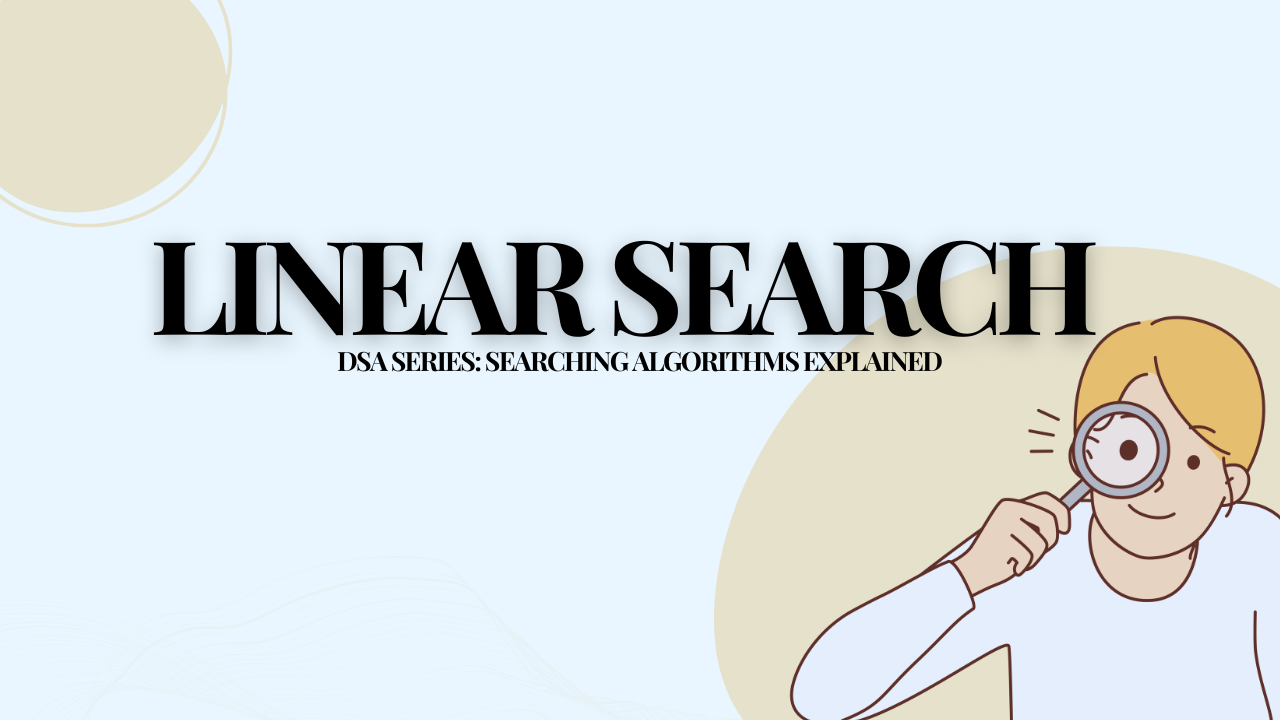
In our exploration of data structures and algorithms, we've seen the importance of sorting algorithms like Bubble Sort, Quick Sort, and Merge Sort, which help us arrange data in a particular order to make it easier to find, analyze, and manipulate. Now, let's turn our attention to search algorithms, which allow us to efficiently locate specific elements within a dataset.
Just as sorting arranges data in a meaningful order, search algorithms are crucial for quickly finding elements, whether it's locating a specific value in a list or finding the shortest path between points in a graph. One of the simplest and most intuitive search algorithms is Linear Search.
Linear Search
Linear Search is one of the simplest search algorithms, where we search for a target element by checking each element in a dataset sequentially. Starting from the first element, it compares each element to the target until it either finds the target or reaches the end of the list. If the target is found, the algorithm returns the index of that element; otherwise, it returns a signal (often -1) indicating that the target is not present.
Linear search process
Start at the beginning: Begin from the first element of the dataset (array or list).
Check each element: Compare the target element with the current element in the dataset.
Move to the next: If the current element isn’t the target, move to the next element and repeat the comparison.
End the search: Continue this process until the target is found or the dataset is fully traversed.
Example
Suppose we have an array of unsorted integers [4, 7, 9, 2, 5]
and we want to find the element 2
. Here's how Linear Search works:
Start with the first element 4
. It’s not equal to 2
, so move to the next element.
Check the second element 7
, it’s not 2
. Move to the next element.
Check the next element 9
,it's not 2
. Move to the next element.
Check 2
, it’s a match! The target is found at index 3.
Linear Search Pseudocode
LinearSearch(array, target):
for each element in array:
if element == target:
return index of element
return -1 // Target not found
Linear Search Python Program
def linear_search(arr, target):
# Iterate through the array
for i in range(len(arr)):
if arr[i] == target:
return i # Target found, return index
return -1 # Target not found
# Sample usage
arr = [4, 7, 9, 2, 5]
target = 2
result = linear_search(arr, target)
if result != -1:
print(f"Element found at index {result}")
else:
print("Element not found")
Time Complexity
Best Case: O(1) – This occurs if the target element is at the first position in the dataset.
Worst Case: O(n) – This occurs if the target element is at the last position or not in the dataset at all, requiring us to check every element.
Average Case: O(n) – In general, since we have no way of knowing where the target is, we might need to search through roughly half the elements on average.
Space Complexity
- O(1): Linear Search requires only a constant amount of extra space, regardless of the size of the input dataset, since it only uses a few variables for tracking the current element and index.
Advantages
Simplicity: It’s easy to implement and doesn’t require complex data structures.
Unsorted Data: Works with both sorted and unsorted data, making it versatile.
Disadvantages
- Inefficiency for large datasets: Since it checks each element one by one, it can be slow for large datasets compared to more advanced algorithms like Binary Search.
Subscribe to my newsletter
Read articles from Keerthi Ravilla Subramanyam directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Keerthi Ravilla Subramanyam
Keerthi Ravilla Subramanyam
Hi, I'm Keerthi Ravilla Subramanyam, a passionate tech enthusiast with a Master's in Computer Science. I love diving deep into topics like Data Structures, Algorithms, and Machine Learning. With a background in cloud engineering and experience working with AWS and Python, I enjoy solving complex problems and sharing what I learn along the way. On this blog, you’ll find articles focused on breaking down DSA concepts, exploring AI, and practical coding tips for aspiring developers. I’m also on a journey to apply my skills in real-world projects like predictive maintenance and data analysis. Follow along for insightful discussions, tutorials, and code snippets to sharpen your technical skills.