Comprehensive Guide to Implementing Content Security Policies in Ruby on Rails 8

Table of contents
- Why CSP Matters in Web Security
- Understanding Rails 8’s CSP Enhancements
- Setting Up CSP in Rails 8
- Inline Scripts and Nonce Generation
- Advanced CSP Directives in Rails 8
- Common Challenges and How to Overcome Them
- Best Practices for Implementing CSP in Rails 8
- Integrating CSP with CI/CD Workflows
- Case Study: Securing an E-Commerce Platform
- Future of CSP in Rails
- Conclusion
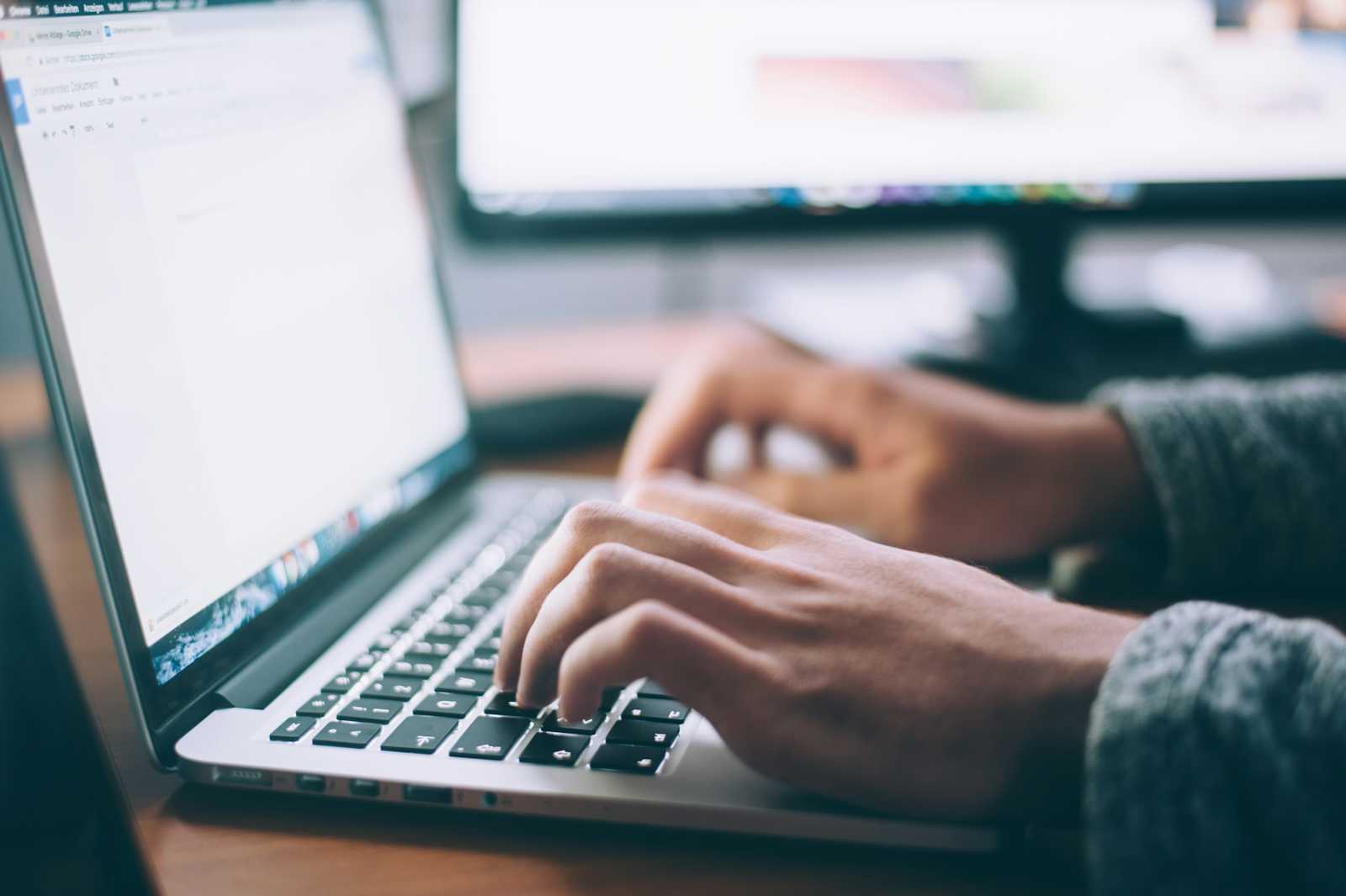
Content Security Policy (CSP) is a critical security feature for modern web applications. It mitigates vulnerabilities such as Cross-Site Scripting (XSS) and data injection attacks by controlling which resources a web page can load or execute.
With cyber threats evolving, CSP ensures a proactive defense mechanism for securing client-side resources.
Ruby on Rails 8, released in November 2024, enhances CSP implementation with native tools and modern features.
This guide provides an in-depth look at leveraging CSP in Rails 8, including best practices, code examples, and insights into the framework’s updates.
Why CSP Matters in Web Security
CSP acts as a web application’s policy layer, governing the content a browser can load.
Its core benefits include:
Preventing Cross-Site Scripting (XSS): Blocking execution of unauthorized scripts.
Mitigating Data Injection Attacks: Restricting access to unapproved resources.
Protecting User Data: Ensuring sensitive data is only transmitted to trusted sources.
Real-world examples illustrate the importance of CSP:
In 2023, a major e-commerce platform mitigated a large-scale XSS vulnerability using a well-configured CSP.
Studies show applications with active CSP policies are 85% less likely to face client-side attacks.
Rails 8 simplifies the adoption of CSP for developers, enabling seamless integration with minimal overhead.
Understanding Rails 8’s CSP Enhancements
Ruby on Rails 8 has introduced several improvements for CSP:
Native CSP Configuration Support: CSP policies are managed directly within Rails without requiring third-party gems.
Improved Reporting Mechanism: Supports
report-to
andreport-uri
directives, integrating with modern browser capabilities.Strict Defaults: Rails 8 adopts a secure-by-default approach, minimizing the risk of misconfigurations.
JavaScript Compatibility: Simplified support for modern JavaScript frameworks like React and Vue.js under CSP constraints.
Setting Up CSP in Rails 8
Rails 8 makes it straightforward to define a CSP for your application.
Begin by creating an initializer file:
# config/initializers/content_security_policy.rb
Rails.application.config.content_security_policy do |policy|
policy.default_src :self, :https
policy.font_src :self, :https, 'https://fonts.gstatic.com'
policy.img_src :self, :https, :data
policy.object_src :none
policy.script_src :self, :https
policy.style_src :self, :https
policy.connect_src :self, :https
policy.report_uri '/csp_reports' if Rails.env.production?
end
This configuration:
Restricts content to trusted sources (
default_src :self, :https
).Allows fonts from Google’s CDN (
font_src :https, 'https://fonts.gstatic.com'
).Disables inline scripts by default, unless explicitly permitted.
Inline Scripts and Nonce Generation
Handling inline scripts remains a challenge in CSP configurations. Rails 8 introduces nonce support to tackle this issue:
<%= content_security_policy_nonce_tag %>
<script nonce="<%= content_security_policy_nonce %>">
console.log('Secured with CSP');
</script>
Using nonces ensures only scripts with the correct identifier are executed, providing an additional layer of security.
Advanced CSP Directives in Rails 8
Rails 8 fully supports modern directives, allowing developers to take advantage of advanced browser features:
Trusted Types: Prevents untrusted script creation:
policy.trusted_types :default
Upgrade Insecure Requests: Automatically upgrades HTTP requests to HTTPS:
policy.upgrade_insecure_requests
Sandboxing: Isolates potentially risky content:
policy.sandbox :allow_forms, :allow_scripts
These directives ensure compatibility with cutting-edge security practices.
Common Challenges and How to Overcome Them
1. Framework Integration (React, Vue.js, Angular):
Modern JavaScript frameworks often conflict with CSP’s strict rules. Rails 8 addresses this by supporting nonce-based whitelisting for dynamic components.
Solution: Add a nonce to dynamically injected elements:
const nonce = document.querySelector('meta[name=csp-nonce]').content;
const script = document.createElement('script');
script.nonce = nonce;
2. Handling Third-Party Resources:
External libraries, like analytics or ads, require careful whitelisting to prevent policy violations.
Solution:
Audit external resources to ensure they align with your security standards.
Use the
connect_src
directive for API endpoints.
3. Policy Violations and Debugging:
CSP violations can disrupt functionality during initial setup.
Solution: Enable a report-uri
endpoint to capture detailed logs:
policy.report_uri '/csp_reports'
Configure a controller to handle these reports:
rubyCopy codeclass CspReportsController < ApplicationController
skip_before_action :verify_authenticity_token
def create
Rails.logger.info "CSP Violation: #{request.body.read}"
head :ok
end
end
Best Practices for Implementing CSP in Rails 8
Start in Report-Only Mode: Before enforcing CSP, use
Rails.application.config.content_security_policy_report_only
to test policies.Iterate Gradually: Begin with a restrictive policy, then whitelist necessary resources.
Leverage Nonce Values: Replace inline scripts with nonced scripts for maximum security.
Monitor Violations Regularly: Analyze reports to refine your policies.
Integrating CSP with CI/CD Workflows
Incorporating CSP validation into your CI/CD pipeline ensures continuous compliance with security best practices.
Steps:
Use tools like CSP Evaluator for static policy analysis.
Automate violation testing with headless browsers (e.g., Puppeteer).
Perform periodic reviews of third-party dependencies to eliminate unnecessary whitelisting.
Case Study: Securing an E-Commerce Platform
A leading e-commerce site adopted CSP using Rails 8. Key steps included:
Whitelisting trusted content sources for payment gateways and CDNs.
Transitioning inline scripts to nonce-based mechanisms.
Enabling
report-to
for real-time monitoring.
Results:
A 70% reduction in reported XSS vulnerabilities.
Improved user trust due to visible commitment to security.
Future of CSP in Rails
As CSP evolves, Rails will likely introduce:
Enhanced support for
trusted-types
.Seamless integration with WebAssembly security features.
Expanded directives for handling AI-based resources.
Conclusion
Ruby on Rails 8 revolutionizes Content Security Policy implementation by providing native tools, improved directives, and modern security practices.
By adopting CSP, developers not only protect their applications from prevalent threats but also build trust with users.
Implementing CSP in Rails 8 may seem complex initially, but with careful planning, monitoring, and iterative improvement, it becomes an invaluable part of a secure web application.
Subscribe to my newsletter
Read articles from Chetan Mittal directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Chetan Mittal
Chetan Mittal
I stumbled upon Ruby on Rails beta version in 2005 and has been using it since then. I have also trained multiple Rails developers all over the globe. Currently, providing consulting and advising companies on how to upgrade, secure, optimize, monitor, modernize, and scale their Rails apps.