Node.js Path Module: A Comprehensive Guide for Beginners
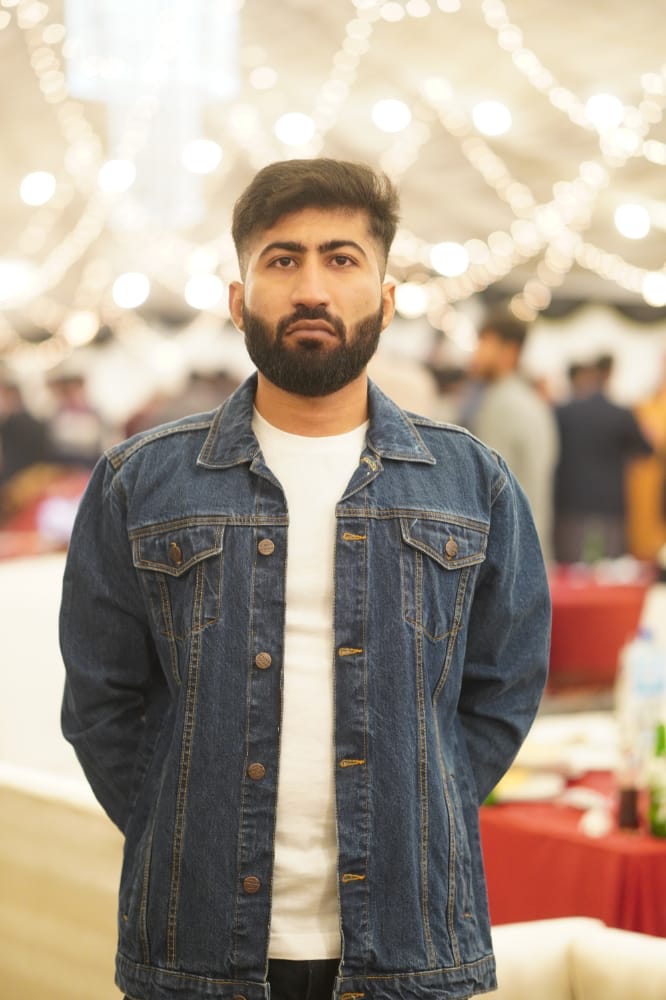
1. What is the path
Module?
The path
module is a built-in Node.js module used to work with file and directory paths. It provides utilities to:
Construct paths.
Normalize paths.
Resolve absolute paths.
Extract specific parts of paths (like directories, file extensions, etc.).
Importing the path
Module
const path = require('path');
2. Key Features of the path
Module
The path
module works consistently across different operating systems by abstracting platform-specific details like:
Windows:
C:\folder\file.txt
Linux/macOS:
/folder/file.txt
3. Understanding Path Formats
A. Path Types
Absolute Path: Starts from the root directory.
Windows:
C:\Users\example\file.txt
Linux/macOS:
/home/example/file.txt
Relative Path: Relative to the current working directory.
- Example:
./folder/file.txt
- Example:
B. Separators
- Use
path.sep
to get the platform-specific separator.
console.log(path.sep); // '\' on Windows, '/' on Linux/macOS
4. Commonly Used Methods in path
A. path.basename()
Returns the last part of a path (the file name).
const filePath = '/users/docs/file.txt';
console.log(path.basename(filePath)); // Output: file.txt
console.log(path.basename(filePath, '.txt')); // Output: file
B. path.dirname()
Returns the directory portion of a path.
const filePath = '/users/docs/file.txt';
console.log(path.dirname(filePath)); // Output: /users/docs
C. path.extname()
Returns the extension of a file.
const filePath = '/users/docs/file.txt';
console.log(path.extname(filePath)); // Output: .txt
D. path.join()
Joins multiple path segments into a single path, handling separators automatically.
const fullPath = path.join('users', 'docs', 'file.txt');
console.log(fullPath); // Output: users/docs/file.txt
E. path.resolve()
Resolves a sequence of paths into an absolute path.
const absolutePath = path.resolve('users', 'docs', 'file.txt');
console.log(absolutePath);
// Output depends on the current working directory (e.g., /home/example/users/docs/file.txt)
F. path.normalize()
Normalizes a path by resolving ..
and .
segments.
const messyPath = '/users/docs/../file.txt';
console.log(path.normalize(messyPath)); // Output: /users/file.txt
G. path.relative()
Calculates the relative path from one location to another.
const from = '/users/docs';
const to = '/users/photos';
console.log(path.relative(from, to)); // Output: ../photos
H. path.parse()
and path.format()
path.parse()
: Breaks a path into its components.
const filePath = '/users/docs/file.txt';
console.log(path.parse(filePath));
/* Output:
{
root: '/',
dir: '/users/docs',
base: 'file.txt',
ext: '.txt',
name: 'file'
}
*/
path.format()
: Combines components into a path.
const pathObj = {
root: '/',
dir: '/users/docs',
base: 'file.txt',
};
console.log(path.format(pathObj)); // Output: /users/docs/file.txt
I. path.isAbsolute()
Checks if a path is absolute.
console.log(path.isAbsolute('/users/docs')); // Output: true
console.log(path.isAbsolute('./docs')); // Output: false
5. Practical Examples
A. Creating a File Path
const fileName = 'report.pdf';
const directory = '/users/docs';
const filePath = path.join(directory, fileName);
console.log(filePath); // Output: /users/docs/report.pdf
B. Resolving a Config File Path
const configPath = path.resolve(__dirname, 'config', 'settings.json');
console.log(configPath);
// Output: Absolute path to settings.json based on the current file location
6. Cross-Platform Compatibility
Paths differ between Windows and Linux/macOS. Use path
utilities to avoid hardcoding:
const crossPlatformPath = path.join('folder', 'subfolder', 'file.txt');
console.log(crossPlatformPath);
// Output: folder\subfolder\file.txt (Windows) or folder/subfolder/file.txt (Linux/macOS)
7. Advanced Usage
A. Working with Relative and Absolute Paths
const relativePath = './docs/file.txt';
console.log(path.resolve(relativePath));
// Converts to absolute path based on current working directory
B. Path Aliases in Projects
In large projects, define aliases using a tool like Webpack or TypeScript to simplify path management.
8. Debugging Tips
Log Paths at Each Step: Use
console.log()
to check intermediate paths.Use
path.normalize()
: Resolve unexpected path issues due to..
or.
.
9. Best Practices
Always Use Path Utilities: Avoid manually concatenating paths using
/
or\
.Normalize Before Use: When accepting external input, normalize the path.
Keep Relative Paths Modular: Avoid hardcoding paths; rely on
__dirname
.
Subscribe to my newsletter
Read articles from Asawer directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
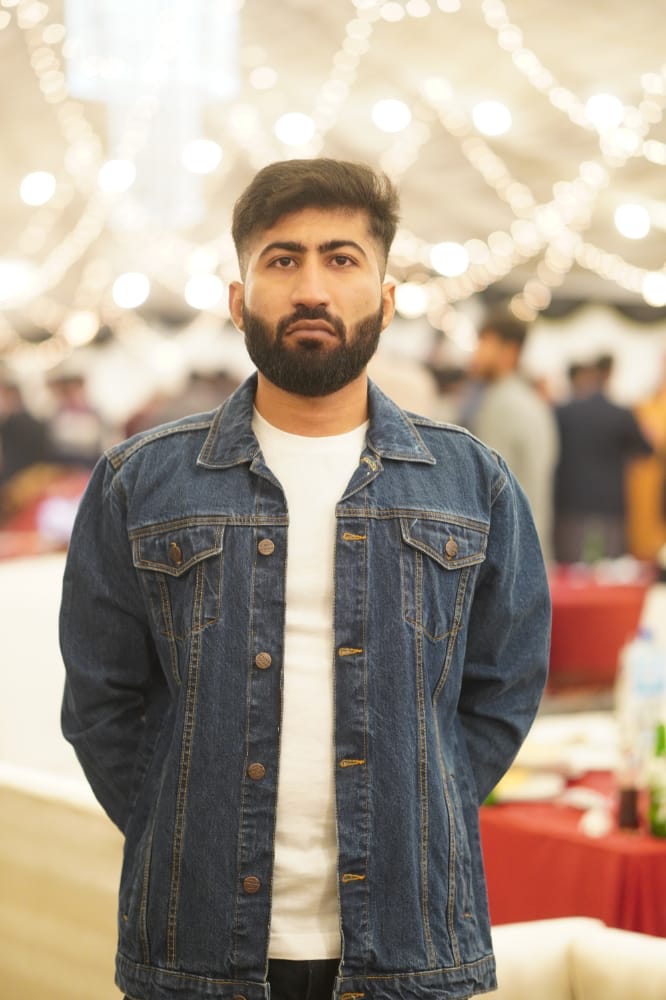