The Fun World of JavaScript

Table of contents
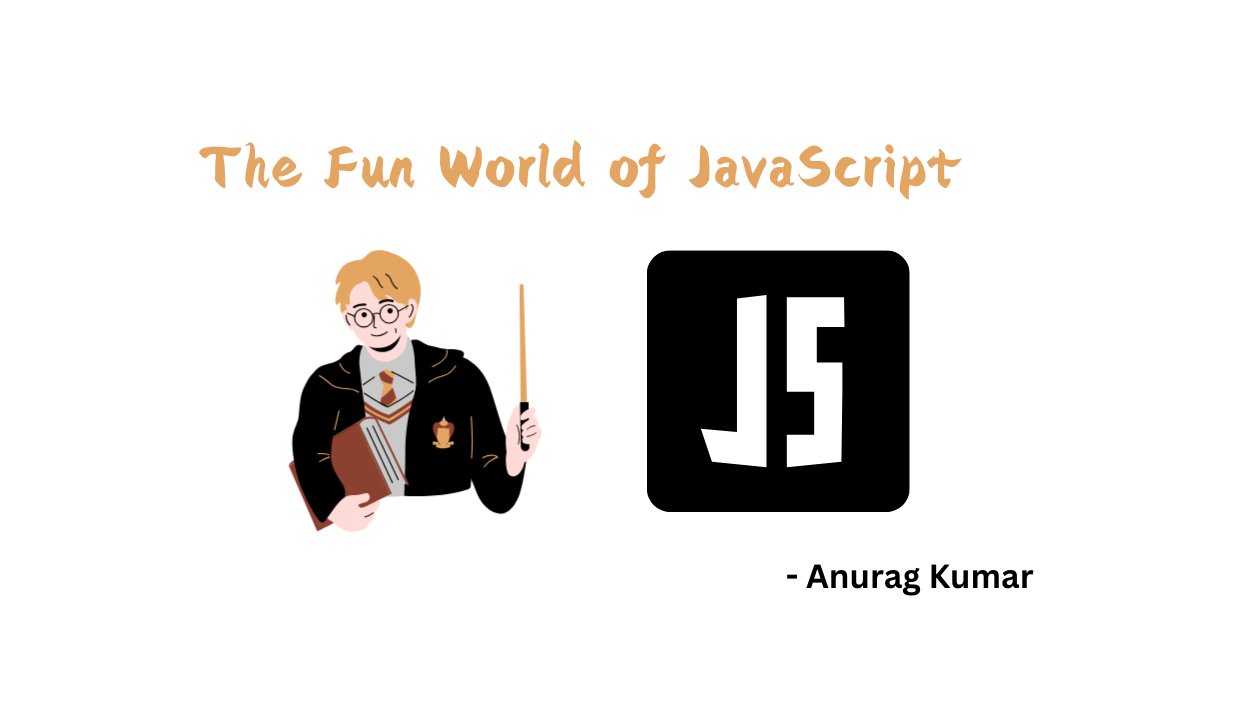
JavaScript is the language that makes websites interactive, but it’s also full of surprises! It can be confusing, funny, and even a little bit strange at times.
In this blog, we’ll understand some of the fun and unexpected things JavaScript does, which have been asked in interviews.
Not a Typo!
Let’s explore some operators that might look like typos in other languages but hold special meaning in JavaScript.
??
Operator
To fellow developers who don’t have much experience in JS, it might look like ‘??‘ is some kind of typo, but it’s not.
Haa ji, you have read it correct.
It’s the operator in JS, which returns the right-hand side (RHS) operand when the left-hand side (LHS) operand is
null
orundefined
.
null ?? 'I win'; // 'I win'
undefined ?? 'Me too'; // 'Me too'
false ?? 'Hi' // false
0 ?? 'Hiii..' // 0
'Hlo' ?? 'Damn it' // 'Hlo'
?.
Operator
What about it?
It’s also an operator in JavaScript 😅.
It is the optional chaining operator, which allows you to safely access deeply nested properties of an object without having to explicitly check if each property in the chain exists.
When you use ?.
, JavaScript will stop evaluation and return undefined
if any part of the chain is null
or undefined
— instead of throwing an error.
// object?.property
const user = { name: "Alice" };
console.log(user?.name); // "Alice"
console.log(user?.age); // undefined (no error)
This will return the property
value if object
is not null
or undefined
; otherwise, it returns undefined
.
- It’s helpful when you are dealing with data from sources where some properties might be
null
orundefined
(like API responses).
It helps avoid errors like TypeError: Cannot read property 'x' of undefined
.
Is 0.2 + 0.4 == 0.4
?
0.2 + 0.4 == 0.4 // false
JavaScript uses the IEEE 754 standard for representing floating-point numbers. This standard cannot precisely represent some decimal numbers, leading to small rounding errors.
When you add 0.2
and 0.4
, the result is not exactly 0.6
, but rather a number very close to it, specifically 0.6000000000000001
.
Decode This
Here’s a little JavaScript snippet—let’s see if you can guess the output:
console.log(('b' + 'a' + + 'a' + 'a').toLowerCase());
Think you have the answer? Try solving it on your own first! But don’t worry if you’re stuck, we’re about to break it down.
Let’s see how it works :
'b' + 'a'
This simply concatenates the two strings. So,
'b' + 'a'
results in the string "ba".+ 'a'
Now, things get interesting. The
+
before'a'
is trying to convert'a'
into a number. But since'a'
isn't a number, JavaScript can’t convert it and instead results in NaN (Not-a-Number).'ba' + NaN
Now you’re concatenating the string "ba" with NaN. So,
"ba" + NaN
results in the string "baNaN".'baNaN' + 'a'
- Next, you add
'a'
to the string"baNaN"
. This gives you "baNaNa".
- Next, you add
.toLowerCase()
Finally, the
.toLowerCase()
method is applied to the string"baNaNa"
, turning it all to lowercase, resulting in "banana".
Understanding Type Coercion in JavaScript
Type coercion—the process of automatically converting one data type to another.
The ==
Operator:
Where we compare the two values after performing the type conversion.
Let’s look at some examples:
'0' == false
// true
(Here, JavaScript converts both'0'
andfalse
into 0 before comparing them, and 0 is equal to 0.)'0' == true
// false
(JavaScript converts'0'
to0
andtrue
to1
. Since0
is not equal to1
, the result isfalse
.)'1' == false
// false
(JavaScript tries to convert both values, butfalse
becomes0
and'1'
becomes the number1
, so they’re not equal.)'1' == true
// true
(Here,'1'
is converted into the number1
, andtrue
becomes1
. So, they are equal!)
null
and undefined
: A Special Case
When comparing null
and undefined
using ==
, they are considered equal—without any further conversion! But when you use the ===
operator, they are not equal, because ===
checks both value and type.
null == undefined
// truenull === undefined
// false
So, if you’re ever in doubt about how these behave, remember: ==
gives them a pass, but ===
is a stickler!
Zero is equal to Empty Array ?
In JavaScript, 0 == []
evaluates to true
.
This happens because of type coercion during the equality check:
The
==
operator attempts to convert both operands to a common type.An empty array
[]
is converted to an empty string""
.The empty string is then converted to
0
when compared with a number.Thus,
0 == 0
results intrue
.
Array is equal not array:
[] == ![]; // -> true
Right-hand side (![]
):
The empty array
[]
is truthy.Applying
!
to a truthy value ([]
) turns it intofalse
, which is then coerced to0
when compared to a number.
Left-hand side ([]
):
The empty array
[]
is truthy.But when an array is compared to a primitive (like
false
or a number), JavaScript coerces the array to a primitive value.An empty array
[]
is coerced to""
(an empty string) when converted to a primitive.Then, when the empty string
""
is compared tofalse
using==
, JavaScript coercesfalse
to0
, and the empty string""
is also coerced to0
.
Using ===
for Strict Comparison
If you want to avoid the confusion that comes with type coercion, use the strict equality operator (===
). It checks both the value and type.
For example:
'0' === false
// false'1' === true
// false
In both cases, ===
returns false
because the values are of different types.
The Mystery of NaN
You’ve probably seen NaN
(Not-a-Number) when something goes wrong in a calculation, but did you know that NaN
is technically a number? It’s true!
typeof NaN
// "number"
So, even though NaN
means "not a number," JavaScript still considers it to be of the type number.
Mixing Strings and Numbers
JavaScript has a way of handling numbers and strings together, and it can be a bit tricky. Let’s see how it behaves:
'5' + 3
// '53'When you use the
+
operator with a string and a number, JavaScript concatenates them as strings. So,'5' + 3
turns into the string '53'.'5' - 3
// 2But when you use the
-
operator, JavaScript converts the string'5'
into a number and performs a normal subtraction. So'5' - 3
results in 2.
These are some of the topics, code snippets, and concepts I discussed here. JavaScript has a lot more. Its quirks and surprises make it fun, but sometimes tricky to learn.
As you continue exploring, remember that every oddity in JavaScript has a reason rooted in its design philosophy—embrace the weirdness, and it will become your strength!
If you've made it to the end, thank you so much for reading!
Feel free to drop any questions or thoughts in the comments below—I’d love to hear about your experience with JavaScript! 😊👨💻👩💻
Check out my portfolio website to connect with me or just say hi!!
Subscribe to my newsletter
Read articles from Anurag Kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Anurag Kumar
Anurag Kumar
Hi, I’m Anurag Kumar, a Software Engineer who loves exploring the latest technologies and sharing insights to help others grow. With a focus on web development and DevOps, I enjoy writing about practical tips, emerging trends, and tools that make developers' lives easier. My goal is to keep my blogs simple, engaging, and useful for everyone—whether you're just starting out or looking to level up your skills. Follow me for friendly and accessible content that bridges the gap between learning and doing!