Chapter 8: CSS: Understanding Positioning, Flexbox, and Practical Implementation
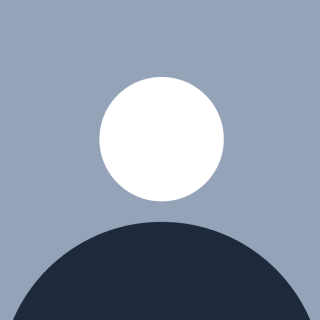
Table of contents
- 1. Introduction
- 2. Positioning in CSS
- 1. Static Positioning
- 2. Relative Positioning
- 3. Absolute Positioning
- 4. Fixed Positioning
- 5. Sticky Positioning
- Key Differences in Positioning:
- Differences Between Positioning Types
- 3. Flexbox Basics
- 4. Advanced Flexbox Concepts
- Conclusion
- 5. Practical Projects
- Project 1: Sticky Navbar with Flexbox
- Project 2: Responsive Card Layout
- Project 3: Tooltips with Absolute Positioning
- Conclusion
- 6.Flexbox Froggy – Solved All Levels
- 7. Conclusion
- Related Posts in My Series:
- Other Series:
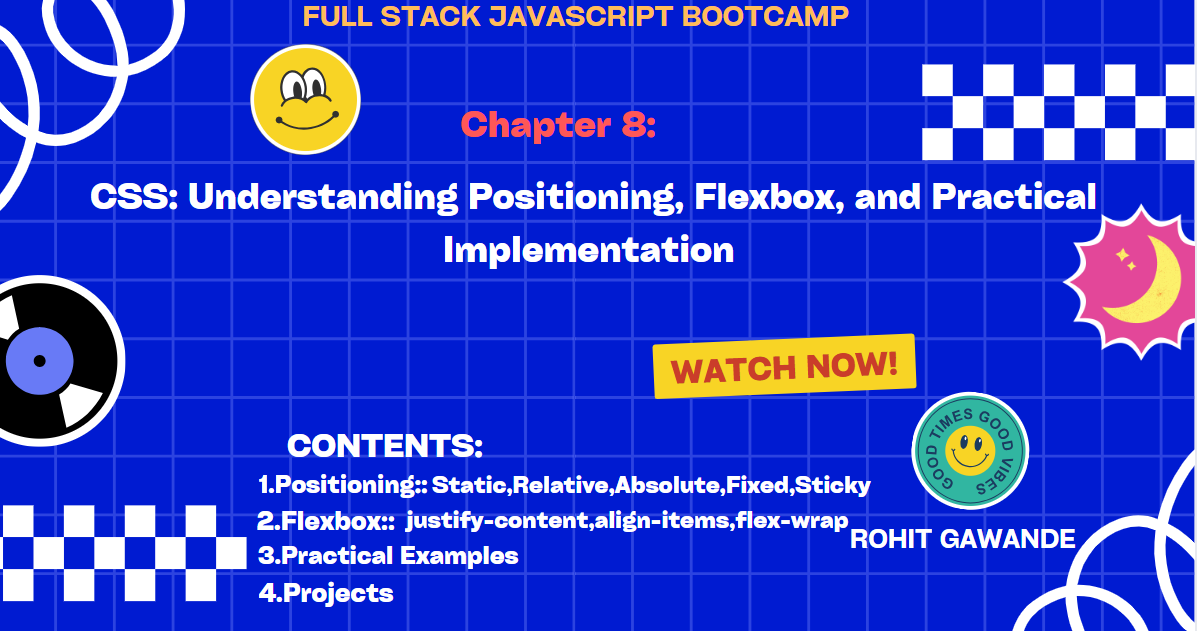
1. Introduction
CSS (Cascading Style Sheets) allows us to design and format HTML documents effectively. Concepts like positioning and flexbox are fundamental for controlling layout and alignment. This guide covers:
CSS positioning: static, relative, absolute, fixed, and sticky.
Flexbox for layout management, including alignment and spacing.
Practical examples and projects.
By the end of this guide, you’ll understand the mechanics of positioning and Flexbox and be equipped to apply them in real-world projects.
2. Positioning in CSS
Positioning in CSS is a core concept that defines how elements are placed on a webpage. There are five main types of positioning: static, relative, absolute, fixed, and sticky. Each serves a unique purpose, and their behaviors vary depending on their context.
1. Static Positioning
Static positioning is the default behavior for all HTML elements. Elements follow the normal document flow without any manual adjustments to their position.
Theory:
Elements are stacked in the order they appear in the HTML.
No extra positioning properties like
top
,right
,bottom
, orleft
are applied.
Code Example:
Output:
- The boxes are stacked vertically with margins, following the natural flow of the document.
2. Relative Positioning
An element with position: relative
is positioned relative to its normal flow. You can use properties like top
, left
, right
, and bottom
to move it without affecting other elements.
Theory:
The space the element originally occupies remains untouched in the document flow.
Useful for small adjustments while maintaining layout integrity.
Code Example:
Output:
- Box "Two" shifts 20px down and 30px to the right, but its original space remains reserved.
3. Absolute Positioning
An absolutely positioned element is removed from the document flow and positioned relative to its nearest positioned ancestor.
Theory:
If no ancestor has
position: relative
, the element is positioned relative to the<html>
(viewport).Other elements in the normal flow do not recognize its space.
Code Example:
Output:
- Box "One" is positioned at the top-left corner of its container, while Box "Two" is at the bottom-right.
4. Fixed Positioning
An element with position: fixed
is positioned relative to the viewport, and its position does not change even when scrolling.
Theory:
Useful for creating sticky headers, footers, or floating buttons.
Fixed elements are removed from the normal flow.
Code Example:
Output:
- The "Fixed" box stays in the bottom-right corner of the viewport regardless of scrolling.
5. Sticky Positioning
Sticky positioning toggles between relative
and fixed
based on the scroll position. The element behaves like relative
until it reaches a specific scroll threshold, where it becomes fixed
.
Theory:
Useful for creating navigation bars or section headers that remain visible during scrolling.
Requires a scrollable container for proper behavior.
Code Example:
Output:
- The "Sticky Header" stays at the top of the page while scrolling until the content beneath it is no longer visible.
Key Differences in Positioning:
Position | Relative to | Flow Impact | Use Cases |
static | Normal document flow | None | Default positioning |
relative | Element's normal position | Reserves space | Adjustments within layout |
absolute | Nearest positioned ancestor | Removed from flow | Pop-ups, tooltips, overlays |
fixed | Viewport | Removed from flow | Sticky navigation, floating buttons |
sticky | Scrollable ancestor boundaries | Depends on scroll behavior | Headers, sticky sections in layouts |
Differences Between Positioning Types
Static:
Default positioning; elements are in normal document flow.
Example: No special positioning applied.
Relative:
Positioned relative to its original position.
Example: Moves but leaves space in its original place.
Absolute:
Positioned relative to its nearest positioned ancestor (or the document if none exists).
Example: Removed from normal flow; other elements ignore it.
Fixed:
Positioned relative to the viewport; does not move during scrolling.
Example: Stays in the same place on the screen.
Sticky:
Toggles between relative and fixed based on scroll position.
Example: Moves with the document until a threshold, then becomes fixed.
3. Flexbox Basics
Flexbox, short for Flexible Box Layout, is a CSS layout model that provides an efficient way to distribute space and align items in a container. It allows developers to design complex layouts with simple code and less need for floating or positioning elements.
In this section, we will explore the key properties and how they work together to create flexible, responsive layouts.
a) display: flex
The display: flex
property is used to define a flex container. This enables the flex model for its direct child elements (also known as flex items).
Usage: When you apply
display: flex
to a container, all the direct children of that container become flex items.Example Code:
%[https://codepen.io/Rohit-Gawande/pen/EaYmxOM]
Explanation:
display: flex;
on the.container
makes it a flex container.The flex items (
.item
) are now aligned in a row by default (the default value offlex-direction
isrow
).
b) justify-content
The justify-content
property is used to align flex items along the main axis (which, by default, is horizontal in a row layout). It controls the spacing between and around the items.
Common values:
flex-start
: Align items to the start of the container.flex-end
: Align items to the end of the container.center
: Align items to the center of the container.space-between
: Distribute items with equal space between them.space-around
: Distribute items with equal space around them.space-evenly
: Distribute items with equal space between and around them.
Example Code:
Explanation:
justify-content: space-evenly;
ensures the three.item
elements are spaced evenly within the.container
.Each item is positioned with equal space between them, including at the start and end of the container.
c) align-items
The align-items
property is used to align the flex items along the cross axis (perpendicular to the main axis). By default, the cross axis is vertical when the flex container is set to row
.
Common values:
flex-start
: Align items to the start of the cross axis.flex-end
: Align items to the end of the cross axis.center
: Align items to the center of the cross axis.stretch
: Stretch items to fill the container (default behavior if no height is defined).baseline
: Align items along their baseline.
Example Code:
%[https://codepen.io/Rohit-Gawande/pen/LYwoBPW]
Explanation:
align-items: center;
ensures that the items are aligned vertically in the middle of the container.The container has a height of
300px
so the vertical alignment can be seen more clearly.
d) flex-direction
The flex-direction
property defines the direction of the main axis, affecting the layout of the flex items.
Common values:
row
: Default value. Flex items are laid out in a horizontal row.row-reverse
: Flex items are laid out in a reverse horizontal row.column
: Flex items are laid out in a vertical column.column-reverse
: Flex items are laid out in a reverse vertical column.
Example Code:
%[https://codepen.io/Rohit-Gawande/pen/rNXgKXV]
Explanation:
flex-direction: column;
arranges the items in a vertical column instead of a row.The items are aligned from top to bottom in the
.container
, andjustify-content: space-between
ensures equal space between the items.
These are the basic concepts of Flexbox, covering display: flex
, justify-content
, align-items
, and flex-direction
. By combining these properties, you can create responsive layouts that adapt to different screen sizes and provide better control over the positioning of elements.
4. Advanced Flexbox Concepts
Flexbox is a powerful tool for building responsive layouts, and understanding its advanced features can significantly enhance your ability to create flexible and dynamic designs. Let's explore some advanced concepts, including flex-wrap
, flex-grow
, flex-shrink
, align-self
, and order
. Each of these properties offers a unique way to manage the layout and positioning of items within a flex container.
a) Flex-Wrap: Controlling Item Wrapping
The flex-wrap
property is used to control whether flex items should wrap onto new lines when there isn't enough space in the container. By default, all flex items are laid out in a single line (no wrapping). If wrapping is enabled, the items will wrap to the next line as needed.
- Syntax:
flex-wrap: nowrap | wrap | wrap-reverse;
Values:
nowrap
: This is the default value, where flex items will not wrap and stay in a single line.wrap
: Allows items to wrap onto the next line when necessary.wrap-reverse
: Items will wrap in reverse order (from bottom to top).
Example of Flex-Wrap:
Explanation:
- In this example, when the screen size is smaller than the combined width of all the items, they will wrap into new rows due to the
flex-wrap: wrap;
rule.
b) Flex-Grow: Distributing Space Proportionally
The flex-grow
property defines the ability for an item to grow relative to the rest of the items in the flex container. By default, flex items will have a flex-grow
value of 0
, meaning they will not grow beyond their initial size. If set to a positive number, the item will grow to fill the available space proportionally.
- Syntax:
flex-grow: <number>;
Values:
0
: Default value, no growth.Any positive number: The item grows relative to others.
Example of Flex-Grow:
Explanation:
- All three
.item
elements will grow proportionally to fill the available space inside the container. Each item takes up an equal share of the remaining space because all of them haveflex-grow: 1
.
c) Flex-Shrink: Controlling Shrinking Behavior
The flex-shrink
property allows you to control how items shrink relative to one another when there is insufficient space in the flex container. By default, flex items can shrink if the container is too small to fit all items, with a default value of 1
. You can control this value to make certain items shrink more or less than others.
- Syntax:
flex-shrink: <number>;
Values:
0
: The item will not shrink, even if space is limited.Any positive number: The item will shrink according to the specified ratio.
Example of Flex-Shrink:
Explanation:
- All items are set to shrink if necessary. Since the container's total width is smaller than the total width of the items, they will shrink proportionally based on the
flex-shrink: 1
rule. If you setflex-shrink: 0
on any item, it won't shrink, causing other items to shrink more.
d) Align-Self: Overriding Default Alignment for Individual Items
The align-self
property allows you to override the default alignment of individual flex items. It works the same way as the align-items
property but applies to a single item instead of all items within the container. This property is especially useful when you need a specific item to align differently without affecting others.
- Syntax:
align-self: auto | flex-start | flex-end | center | baseline | stretch;
Values:
auto
: Default value, inherits fromalign-items
.flex-start
,flex-end
,center
,baseline
,stretch
: Custom alignment values for that specific item.
Example of Align-Self:
Explanation:
- The
.special
item will be aligned to the bottom of the flex container while other items are aligned to the center.
e) Order: Changing the Visual Order of Items
The order
property controls the visual order of flex items. By default, all items have an order value of 0
. If you assign a positive or negative integer to the order
property, you can change the display order without affecting the document order.
- Syntax:
order: <number>;
Values:
- Any integer value: Items are reordered based on this number. Items with a lower
order
value will be displayed first.
- Any integer value: Items are reordered based on this number. Items with a lower
Example of Order:
Explanation:
- The order of the items visually appears as: Item 2, Item 3, Item 1, even though they appear in the original order in the HTML. The
order
property allows us to change this visually while keeping the logical structure intact.
Conclusion
Mastering advanced Flexbox concepts such as flex-wrap
, flex-grow
, flex-shrink
, align-self
, and order
can greatly enhance your ability to design complex, responsive layouts. By applying these techniques in practical projects, you can create layouts that adapt to various screen sizes and offer a dynamic user experience. With Flexbox's powerful alignment and spacing features, the possibilities for creative design are endless.
c) Practical Differences Between align-items
and justify-content
Aspect | justify-content | align-items |
Axis | Aligns items on the main axis. | Aligns items on the cross axis. |
Values | center , space-between , etc. | center , stretch , etc. |
5. Practical Projects
In this section, we will explore three practical projects that will help you apply CSS positioning and Flexbox concepts. Each project uses different techniques and properties discussed in this guide. Let’s break them down step-by-step with complete code examples.
Project 1: Sticky Navbar with Flexbox
Objective:
Create a sticky navigation bar that stays at the top of the page when you scroll, using position: sticky
for the navbar and Flexbox to manage the layout.
Steps:
Use Flexbox to arrange the navigation items horizontally.
Apply
position: sticky
to keep the navbar at the top when scrolling.Style the navbar for better aesthetics and responsiveness.
Code Example:
Explanation:
position: sticky
on the<nav>
keeps the navbar at the top of the page when scrolling.Flexbox is used to arrange the logo and navigation links horizontally, and
justify-content: space-between
ensures they are spaced evenly across the navbar.z-index: 1000
ensures the navbar stays on top of other content while scrolling.
Project 2: Responsive Card Layout
Objective:
Create a responsive grid layout for cards using Flexbox. The cards should adjust their layout based on the screen size, using flex-wrap
to wrap the cards onto new lines when necessary.
Steps:
Use Flexbox to create a container for the cards.
Use
flex-wrap
to allow the cards to wrap to the next line when the screen size is reduced.Add media queries to make the layout responsive.
Code Example:
Explanation:
flex-wrap: wrap
in the.card-container
ensures that the cards wrap to the next line if there isn’t enough space.justify-content: space-around
spreads the cards evenly with equal space between them.Media query reduces the card size on smaller screens, ensuring the layout remains responsive.
Project 3: Tooltips with Absolute Positioning
Objective:
Create a tooltip that appears when hovering over an element. The tooltip should be positioned absolutely relative to the hovered element.
Steps:
Use
position: absolute
for the tooltip to place it precisely near the element.Use
position: relative
on the parent element to serve as the reference for absolute positioning.Add a hover effect to trigger the tooltip visibility.
Code Example:
Explanation:
position: relative
on.tooltip-container
creates a reference for the absolutely positioned.tooltip
.position: absolute
places the tooltip above the container (usingbottom: 100%
).Visibility and opacity are controlled by the
:hover
state, which shows the tooltip when the user hovers over the container.
Conclusion
These three projects demonstrate practical applications of CSS positioning and Flexbox in real-world scenarios. They cover:
A sticky navbar for persistent navigation.
A responsive card layout that adapts to screen sizes.
Tooltips that appear on hover, positioned absolutely.
By working through these projects, you’ll gain a deeper understanding of how to implement advanced CSS techniques for building responsive, user-friendly web pages.
6.Flexbox Froggy – Solved All Levels
I completed Flexbox Froggy, an interactive game designed to teach Flexbox CSS concepts. It helped me practice using the justify-content
, align-items
, and flex-direction
properties in real-world scenarios.
In this game, the goal was to move Froggy and other characters to their respective lilypads by using different Flexbox values. I successfully solved all 24 levels, each one progressively teaching me different Flexbox properties and how to manipulate them effectively.
Key Concepts Learned:
justify-content: Controls the horizontal alignment of items.
- Values:
flex-start
,flex-end
,center
,space-between
,space-around
- Values:
align-items: Controls the vertical alignment of items within the container.
- Values:
flex-start
,flex-end
,center
,baseline
,stretch
- Values:
flex-direction: Defines the direction in which the flex container’s items are arranged.
- Values:
row
,row-reverse
,column
,column-reverse
- Values:
flex-wrap: Defines whether the flex container is a single-line or multi-line layout.
- Values:
nowrap
,wrap
,wrap-reverse
- Values:
The game not only improved my understanding of Flexbox but also made learning more engaging. Now, I feel confident in using Flexbox for creating flexible, responsive layouts.
7. Conclusion
Mastering CSS positioning and Flexbox equips developers with powerful tools to create dynamic and responsive designs. By understanding key differences between position values and utilizing Flexbox for layout management, you can craft user-friendly and visually appealing web pages.
Experiment with real-world projects and refine your skills further! 🌟
Related Posts in My Series:
Chapter 1:Mastering the Web: A Journey Through HTML and Beyond
Other Series:
Connect with Me
Stay updated with my latest posts and projects by following me on social media:
LinkedIn: Connect with me for professional updates and insights.
GitHub: Explore my repository and contributions to various projects.
LeetCode: Check out my coding practice and challenges.
Your feedback and engagement are invaluable. Feel free to reach out with questions, comments, or suggestions. Happy coding!
Rohit Gawande
Full Stack Java Developer | Blogger | Coding Enthusiast
Subscribe to my newsletter
Read articles from Rohit Gawande directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
Rohit Gawande
Rohit Gawande
🚀 Tech Enthusiast | Full Stack Developer | System Design Explorer 💻 Passionate About Building Scalable Solutions and Sharing Knowledge Hi, I’m Rohit Gawande! 👋I am a Full Stack Java Developer with a deep interest in System Design, Data Structures & Algorithms, and building modern web applications. My goal is to empower developers with practical knowledge, best practices, and insights from real-world experiences. What I’m Currently Doing 🔹 Writing an in-depth System Design Series to help developers master complex design concepts.🔹 Sharing insights and projects from my journey in Full Stack Java Development, DSA in Java (Alpha Plus Course), and Full Stack Web Development.🔹 Exploring advanced Java concepts and modern web technologies. What You Can Expect Here ✨ Detailed technical blogs with examples, diagrams, and real-world use cases.✨ Practical guides on Java, System Design, and Full Stack Development.✨ Community-driven discussions to learn and grow together. Let’s Connect! 🌐 GitHub – Explore my projects and contributions.💼 LinkedIn – Connect for opportunities and collaborations.🏆 LeetCode – Check out my problem-solving journey. 💡 "Learning is a journey, not a destination. Let’s grow together!" Feel free to customize or add more based on your preferences! 😊