Building a Simple TCP Web Client in C
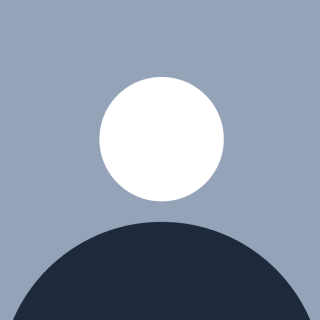
Introduction
In this post, we'll walk through building a simple TCP web client from scratch. Instead of posting the entire code upfront, we'll dive straight into the important sections, explaining the key concepts step by step.
Below here I have shown pseudo code for entire thing
// Import libraries for networking and error handling
// Set buffer size for response data
// Define function to handle errors and exit
// Check if correct arguments (IP & port) are provided
// Set up socket to connect using IP and port
// Try connecting to the server
// Send an HTTP GET request to the server
// Receive and print the response from the server
// Close the socket connection
Part of code Now I want to show is
... includes and Function Prototypes ...
int main(int argc, char *argv[])
{
.....
int socket_file_descriptor = socket(AF_INET, SOCK_STREAM, 0);
if (socket_file_descriptor < 0)
{
error_exit("Socket creation failed");
}
.....
close(socket_file_descriptor);
return 0;
}
void error_exit(const char *message)
{
perror(message);
exit(EXIT_FAILURE);
}
socket()
is a system call that creates a new socket for network communication.AF_INET
specifies that we're using the IPv4 protocol.SOCK_STREAM
tells the system we're creating a TCP connection (as opposed to a UDP connection).The last parameter (
0
) specifies the protocol to be used (here, the default TCP/IP stack).If
socket()
returns a negative value (usually-1
), it means the socket creation failed due to some error (like insufficient resources, invalid arguments, etc.).
Questions that came to my mind
What other socket types can you create besides
SOCK_STREAM
?What are the other address families available besides
AF_INET
(IPv4)?What are the different protocols you can specify when creating a socket?
Stay tuned as we dive deeper into networking :)
Subscribe to my newsletter
Read articles from Suriya Gnanamoorthy directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
Suriya Gnanamoorthy
Suriya Gnanamoorthy
🚀 Tech Explorer | Next.js Enthusiast | Blogging My Learning Journey Hey there! I'm Suriya, a developer passionate about building with Next.js, TypeScript, Tailwind CSS, and exploring databases. I document my journey in networking, operating systems, and databases—breaking down complex concepts into beginner-friendly insights for my brain to understand things.